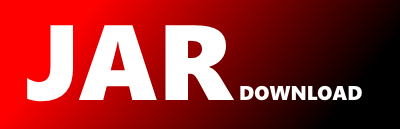
org.devocative.adroit.sql.SqlHelper Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of adroit Show documentation
Show all versions of adroit Show documentation
Utility classes specially used in Demeter project
package org.devocative.adroit.sql;
import com.thoughtworks.xstream.XStream;
import org.devocative.adroit.sql.plugin.INpsPlugin;
import org.devocative.adroit.sql.result.EColumnNameCase;
import org.devocative.adroit.sql.result.QueryVO;
import org.devocative.adroit.sql.result.ResultSetProcessor;
import org.devocative.adroit.xml.AdroitXStream;
import java.io.InputStream;
import java.sql.*;
import java.util.*;
import java.util.Date;
public class SqlHelper {
private final Connection connection;
private boolean ignoreExtraPassedParam = true;
private EColumnNameCase nameCase = EColumnNameCase.LOWER;
private Class extends Date> dateClassReplacement = java.sql.Date.class;
private Map xQueryMap = new HashMap<>();
// ------------------------------
public SqlHelper(Connection connection) {
this.connection = connection;
}
// ------------------------------
public SqlHelper setIgnoreExtraPassedParam(boolean ignoreExtraPassedParam) {
this.ignoreExtraPassedParam = ignoreExtraPassedParam;
return this;
}
public SqlHelper setNameCase(EColumnNameCase nameCase) {
this.nameCase = nameCase;
return this;
}
public SqlHelper setDateClassReplacement(Class extends Date> dateClassReplacement) {
this.dateClassReplacement = dateClassReplacement;
return this;
}
public SqlHelper setXMLQueryFile(InputStream in) {
XStream xStream = new AdroitXStream();
xStream.processAnnotations(XQuery.class);
List xQueries = (List) xStream.fromXML(in);
for (XQuery xQuery : xQueries) {
xQueryMap.put(xQuery.getName(), xQuery);
}
return this;
}
// ---------------
public NamedParameterStatement createNPS(String name) {
return createNPS(xQueryMap.get(name));
}
public NamedParameterStatement createNPS(XQuery sql) {
return new NamedParameterStatement(connection, sql.getSql())
.setIgnoreExtraPassedParam(ignoreExtraPassedParam)
.setDateClassReplacement(dateClassReplacement);
}
// ---------------
public Map twoCellsAsMap(String name) throws SQLException {
return twoCellsAsMap(name, new HashMap<>());
}
public Map twoCellsAsMap(String name, Map params, INpsPlugin... plugins) throws SQLException {
return twoCellsAsMap(xQueryMap.get(name), params, plugins);
}
public Map twoCellsAsMap(XQuery sql) throws SQLException {
return twoCellsAsMap(sql, new HashMap<>());
}
public Map twoCellsAsMap(XQuery sql, Map params, INpsPlugin... plugins) throws SQLException {
NamedParameterStatement nps = createNPS(sql, params, plugins);
ResultSet rs = nps.executeQuery();
ResultSetMetaData metaData = rs.getMetaData();
int col1Type = metaData.getColumnType(1);
int col2Type = metaData.getColumnType(2);
Map result = new LinkedHashMap<>();
while (rs.next()) {
K key = (K) ResultSetProcessor.getValue(rs, 1, col1Type);
V value = (V) ResultSetProcessor.getValue(rs, 2, col2Type);
result.put(key, value);
}
nps.close();
return result;
}
// -----
public List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy