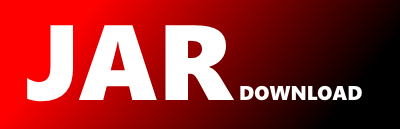
org.devzendo.xplp.UnixScriptLauncherCreator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of CrossPlatformLauncherPlugin Show documentation
Show all versions of CrossPlatformLauncherPlugin Show documentation
A Maven plugin that creates launchers for Windows
(using Janel), Mac OS X (creating a .app structure, or tree with shell
script) or Linux (using a shell script).
(Apache License v2) 2008-2013 Matt Gumbley, DevZendo.org
The newest version!
/**
* Copyright (C) 2008-2010 Matt Gumbley, DevZendo.org
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
/**
*
*/
package org.devzendo.xplp;
import java.io.File;
import java.io.IOException;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import java.util.Properties;
import java.util.Set;
import org.apache.maven.artifact.Artifact;
import org.apache.maven.plugin.AbstractMojo;
import org.codehaus.plexus.util.StringUtils;
/**
* Create a UNIX script launcher directory structure.
* @author matt
*
*/
public class UnixScriptLauncherCreator extends LauncherCreator {
private final String mOsOutputSubDirectoryName;
/**
* @param mojo the parent mojo class
* @param outputDirectory where to create the .app structure
* @param osOutputSubDirectoryName the name of the directory under the
* output directory in which this lancher structure will be created, e.g
* linux or macosx
* @param mainClassName the main class
* @param applicationName the name of the application
* @param libraryDirectory where the libraries are stored
* @param transitiveArtifacts the set of transitive artifact dependencies
* @param resourceDirectories the project's resource directories
* @param parameterProperties the plugin configuration parameters, as properties
* @param systemProperties an array of name=value system properties
* @param vmArguments an array of arguments to the VM
* @param narClassifierTypes an array of NAR classifier:types
*/
public UnixScriptLauncherCreator(final AbstractMojo mojo,
final File outputDirectory,
final String osOutputSubDirectoryName,
final String mainClassName,
final String applicationName,
final String libraryDirectory,
final Set transitiveArtifacts,
final Set resourceDirectories,
final Properties parameterProperties,
final String[] systemProperties,
final String[] vmArguments,
final String[] narClassifierTypes) {
super(mojo, outputDirectory, mainClassName,
applicationName, libraryDirectory,
transitiveArtifacts, resourceDirectories, parameterProperties,
systemProperties, vmArguments, narClassifierTypes);
mOsOutputSubDirectoryName = osOutputSubDirectoryName;
}
/**
* {@inheritDoc}
*/
@Override
public void createLauncher() throws IOException {
final File osOutputDir = new File(getOutputDirectory(), mOsOutputSubDirectoryName);
final File binDir = new File(osOutputDir, "bin");
final File libDir = new File(osOutputDir, "lib");
osOutputDir.mkdirs();
binDir.mkdirs();
libDir.mkdirs();
final boolean allDirsOK = osOutputDir.exists()
&& binDir.exists() && libDir.exists();
if (!allDirsOK) {
throw new IOException("Could not create required directories under " + getOutputDirectory().getAbsolutePath());
}
final List jvmArgs = new ArrayList();
jvmArgs.addAll(systemPropertiesAsJVMArgs(getSystemProperties()));
jvmArgs.addAll(vmArgumentsAsJVMArgs(getVmArguments()));
final StringBuilder jvmArgsString = new StringBuilder();
for (final String jvmArg : jvmArgs) {
jvmArgsString.append(jvmArg);
jvmArgsString.append(' '); // a space at the end is needed
}
getParameterProperties().put("xplp.linuxjvmargs", jvmArgsString.toString());
getParameterProperties().put("xplp.linuxclasspatharray", transitiveArtifactsAsClassPathArray(getTransitiveArtifacts()));
final File outputRunScript = new File(binDir, getApplicationName());
copyInterpolatedPluginResource("linux/launcher.sh", outputRunScript);
makeExecutable(outputRunScript);
copyTransitiveArtifacts(libDir);
}
private List vmArgumentsAsJVMArgs(final String[] vmArguments) {
return Arrays.asList(vmArguments);
}
private List systemPropertiesAsJVMArgs(final String[] systemProperties) {
final List addDList = new ArrayList();
for (final String sysProp : systemProperties) {
addDList.add("-D" + sysProp);
}
return addDList;
}
private String transitiveArtifactsAsClassPathArray(final Set transitiveArtifacts) {
final ArrayList libsAsArtifacts = new ArrayList();
final Set transitiveArtifactFileNames = getTransitiveJarOrNarArtifactFileNames(transitiveArtifacts);
for (final String fileName : transitiveArtifactFileNames) {
libsAsArtifacts.add("$progdir/../lib/" + fileName);
}
return StringUtils.join(libsAsArtifacts.iterator(), ":");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy