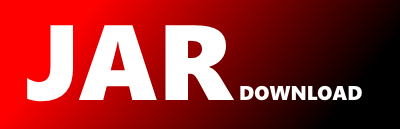
ch.randelshofer.quaqua.jaguar.filechooser.QuaquaJaguarFileChooserPanel Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of Quaqua Show documentation
Show all versions of Quaqua Show documentation
A Mavenisation of the Quaqua Mac OSX Swing Look and Feel (Java library)
Quaqua Look and Feel (C) 2003-2010, Werner Randelshofer.
Mavenisation by Matt Gumbley, DevZendo.org - for problems with
Mavenisation, see Matt; for issues with Quaqua, see the Quaqua home page.
For full license details, see http://randelshofer.ch/quaqua/license.html
The newest version!
/*
* @(#)TestQuaquaFileChooserPanel.java 1.0.2 2006-04-23
*
* Copyright (c) 2003-2010 Werner Randelshofer, Immensee, Switzerland.
* http://www.randelshofer.ch
* All rights reserved.
*
* You may not use, copy or modify this file, except in compliance with the
* license agreement you entered into with Werner Randelshofer.
* For details see accompanying license terms.
*/
package ch.randelshofer.quaqua.jaguar.filechooser;
import ch.randelshofer.quaqua.*;
import ch.randelshofer.quaqua.util.*;
import javax.swing.*;
/**
* QuaquaFileChooserPanel (This class is needed only to design the UI of the
* QuaquaFileChooserUI in the NetBeans form editor).
*
* @author Werner Randelshofer
* @version 1.0.2 2006-04-23 Labels are now directly retrieved from UIManager.
*
1.0.1 2005-11-07 Get "Labels" resource bundle from UIManager.
*
1.0 July 24, 2003 Created.
*/
public class QuaquaJaguarFileChooserPanel extends javax.swing.JPanel {
/** Creates a new instance. */
public QuaquaJaguarFileChooserPanel() {
initComponents();
}
public static void main(String[] args) {
JFrame f = new JFrame("Open Frame");
f.getContentPane().add(new QuaquaJaguarFileChooserPanel());
f.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
f.setVisible(true);
JDialog d = new JDialog(f, "Open Dialog");
//d.getContentPane().add(new QuaquaJaguarFileChooserPanel());
JPanel p = new JPanel();
QuaquaJaguarFileChooserPanel fp;
p.add(fp = new QuaquaJaguarFileChooserPanel());
d.getContentPane().add(p);
d.getRootPane().setDefaultButton(fp.approveButton);
d.setVisible(true);
}
/** This method is called from within the constructor to
* initialize the form.
* WARNING: Do NOT modify this code. The content of this method is
* always regenerated by the Form Editor.
*/
// //GEN-BEGIN:initComponents
private void initComponents() {
java.awt.GridBagConstraints gridBagConstraints;
fc = new javax.swing.JPanel();
fromPanel = new javax.swing.JPanel();
fileNameLabel = new javax.swing.JLabel();
fileNameTextField = new javax.swing.JTextField();
strutPanel1 = new javax.swing.JPanel();
lookInLabel = new javax.swing.JLabel();
directoryComboBox = new javax.swing.JComboBox();
strutPanel2 = new javax.swing.JPanel();
separatorPanel1 = new javax.swing.JPanel();
separatorPanel2 = new javax.swing.JPanel();
browserScrollPane = new javax.swing.JScrollPane();
browser = new ch.randelshofer.quaqua.JBrowser();
newFolderButton = new javax.swing.JButton();
separatorPanel = new javax.swing.JPanel();
formatPanel = new javax.swing.JPanel();
formatPanel2 = new javax.swing.JPanel();
filesOfTypeLabel = new javax.swing.JLabel();
filterComboBox = new javax.swing.JComboBox();
accessoryPanel = new javax.swing.JPanel();
buttonPanel = new javax.swing.JPanel();
cancelButton = new javax.swing.JButton();
approveButton = new javax.swing.JButton();
setLayout(new java.awt.BorderLayout());
fc.setLayout(new java.awt.GridBagLayout());
fromPanel.setLayout(new java.awt.GridBagLayout());
fileNameLabel.setText(UIManager.getString("FileChooser.fileNameLabelText"));
gridBagConstraints = new java.awt.GridBagConstraints();
gridBagConstraints.gridx = 0;
gridBagConstraints.gridy = 0;
gridBagConstraints.gridwidth = 2;
gridBagConstraints.anchor = java.awt.GridBagConstraints.EAST;
gridBagConstraints.insets = new java.awt.Insets(0, 0, 14, 0);
fromPanel.add(fileNameLabel, gridBagConstraints);
gridBagConstraints = new java.awt.GridBagConstraints();
gridBagConstraints.gridx = 2;
gridBagConstraints.gridy = 0;
gridBagConstraints.fill = java.awt.GridBagConstraints.HORIZONTAL;
gridBagConstraints.weightx = 1.0;
gridBagConstraints.insets = new java.awt.Insets(0, 2, 14, 0);
fromPanel.add(fileNameTextField, gridBagConstraints);
strutPanel1.setLayout(null);
gridBagConstraints = new java.awt.GridBagConstraints();
gridBagConstraints.gridx = 0;
gridBagConstraints.gridy = 1;
gridBagConstraints.ipadx = 40;
gridBagConstraints.ipady = 5;
fromPanel.add(strutPanel1, gridBagConstraints);
lookInLabel.setText(UIManager.getString("FileChooser.fromLabelText"));
gridBagConstraints = new java.awt.GridBagConstraints();
gridBagConstraints.gridx = 1;
gridBagConstraints.gridy = 1;
gridBagConstraints.gridheight = 2;
gridBagConstraints.anchor = java.awt.GridBagConstraints.EAST;
gridBagConstraints.insets = new java.awt.Insets(0, 1, 0, 0);
fromPanel.add(lookInLabel, gridBagConstraints);
gridBagConstraints = new java.awt.GridBagConstraints();
gridBagConstraints.gridx = 2;
gridBagConstraints.gridy = 1;
gridBagConstraints.gridheight = 2;
gridBagConstraints.fill = java.awt.GridBagConstraints.HORIZONTAL;
gridBagConstraints.anchor = java.awt.GridBagConstraints.WEST;
gridBagConstraints.weightx = 1.0;
gridBagConstraints.insets = new java.awt.Insets(0, 2, 0, 0);
fromPanel.add(directoryComboBox, gridBagConstraints);
strutPanel2.setLayout(new java.awt.BorderLayout());
gridBagConstraints = new java.awt.GridBagConstraints();
gridBagConstraints.gridx = 3;
gridBagConstraints.gridy = 1;
gridBagConstraints.ipadx = 40;
gridBagConstraints.ipady = 5;
fromPanel.add(strutPanel2, gridBagConstraints);
separatorPanel1.setLayout(new java.awt.BorderLayout());
separatorPanel1.setBackground(javax.swing.UIManager.getDefaults().getColor("Separator.foreground"));
gridBagConstraints = new java.awt.GridBagConstraints();
gridBagConstraints.gridx = 0;
gridBagConstraints.gridy = 2;
gridBagConstraints.fill = java.awt.GridBagConstraints.HORIZONTAL;
gridBagConstraints.ipadx = 40;
gridBagConstraints.ipady = 1;
gridBagConstraints.weightx = 1.0E-4;
fromPanel.add(separatorPanel1, gridBagConstraints);
separatorPanel2.setLayout(new java.awt.BorderLayout());
separatorPanel2.setBackground(javax.swing.UIManager.getDefaults().getColor("Separator.foreground"));
gridBagConstraints = new java.awt.GridBagConstraints();
gridBagConstraints.gridx = 3;
gridBagConstraints.gridy = 2;
gridBagConstraints.ipadx = 40;
gridBagConstraints.ipady = 1;
fromPanel.add(separatorPanel2, gridBagConstraints);
gridBagConstraints = new java.awt.GridBagConstraints();
gridBagConstraints.gridy = 0;
gridBagConstraints.gridwidth = 2;
gridBagConstraints.fill = java.awt.GridBagConstraints.HORIZONTAL;
gridBagConstraints.insets = new java.awt.Insets(14, 0, 0, 0);
fc.add(fromPanel, gridBagConstraints);
browserScrollPane.setHorizontalScrollBarPolicy(javax.swing.ScrollPaneConstants.HORIZONTAL_SCROLLBAR_ALWAYS);
browserScrollPane.setVerticalScrollBarPolicy(javax.swing.ScrollPaneConstants.VERTICAL_SCROLLBAR_NEVER);
browserScrollPane.setViewportView(browser);
gridBagConstraints = new java.awt.GridBagConstraints();
gridBagConstraints.gridy = 1;
gridBagConstraints.gridwidth = 2;
gridBagConstraints.fill = java.awt.GridBagConstraints.BOTH;
gridBagConstraints.weightx = 1.0;
gridBagConstraints.weighty = 1.0;
gridBagConstraints.insets = new java.awt.Insets(8, 23, 0, 23);
fc.add(browserScrollPane, gridBagConstraints);
newFolderButton.setText(UIManager.getString("FileChooser.newFolderButtonText"));
gridBagConstraints = new java.awt.GridBagConstraints();
gridBagConstraints.gridx = 0;
gridBagConstraints.gridy = 2;
gridBagConstraints.gridwidth = 2;
gridBagConstraints.insets = new java.awt.Insets(4, 0, 0, 0);
fc.add(newFolderButton, gridBagConstraints);
separatorPanel.setLayout(new java.awt.BorderLayout());
separatorPanel.setBackground(javax.swing.UIManager.getDefaults().getColor("Separator.foreground"));
gridBagConstraints = new java.awt.GridBagConstraints();
gridBagConstraints.gridx = 0;
gridBagConstraints.gridy = 3;
gridBagConstraints.fill = java.awt.GridBagConstraints.HORIZONTAL;
gridBagConstraints.ipady = 1;
gridBagConstraints.weightx = 1.0;
gridBagConstraints.insets = new java.awt.Insets(14, 0, 0, 0);
fc.add(separatorPanel, gridBagConstraints);
formatPanel.setLayout(new java.awt.GridBagLayout());
formatPanel2.setLayout(new java.awt.BorderLayout(2, 0));
filesOfTypeLabel.setText(UIManager.getString("FileChooser.filesOfTypeLabelText"));
formatPanel2.add(filesOfTypeLabel, java.awt.BorderLayout.WEST);
formatPanel2.add(filterComboBox, java.awt.BorderLayout.CENTER);
gridBagConstraints = new java.awt.GridBagConstraints();
gridBagConstraints.gridx = 0;
gridBagConstraints.gridy = 0;
gridBagConstraints.insets = new java.awt.Insets(0, 40, 0, 40);
formatPanel.add(formatPanel2, gridBagConstraints);
gridBagConstraints = new java.awt.GridBagConstraints();
gridBagConstraints.gridx = 0;
gridBagConstraints.gridy = 4;
gridBagConstraints.gridwidth = 2;
gridBagConstraints.fill = java.awt.GridBagConstraints.HORIZONTAL;
gridBagConstraints.insets = new java.awt.Insets(14, 0, 0, 0);
fc.add(formatPanel, gridBagConstraints);
accessoryPanel.setLayout(new java.awt.BorderLayout());
gridBagConstraints = new java.awt.GridBagConstraints();
gridBagConstraints.gridx = 0;
gridBagConstraints.gridy = 5;
gridBagConstraints.gridwidth = 2;
gridBagConstraints.fill = java.awt.GridBagConstraints.BOTH;
gridBagConstraints.insets = new java.awt.Insets(14, 20, 0, 20);
fc.add(accessoryPanel, gridBagConstraints);
buttonPanel.setLayout(new java.awt.GridBagLayout());
cancelButton.setText(UIManager.getString("FileChooser.cancelButtonText"));
gridBagConstraints = new java.awt.GridBagConstraints();
gridBagConstraints.gridx = 0;
gridBagConstraints.gridy = 0;
gridBagConstraints.anchor = java.awt.GridBagConstraints.EAST;
gridBagConstraints.weightx = 1.0;
gridBagConstraints.insets = new java.awt.Insets(0, 18, 16, 0);
buttonPanel.add(cancelButton, gridBagConstraints);
approveButton.setText(UIManager.getString("FileChooser.openButtonText"));
gridBagConstraints = new java.awt.GridBagConstraints();
gridBagConstraints.gridx = 1;
gridBagConstraints.gridy = 0;
gridBagConstraints.insets = new java.awt.Insets(0, 6, 16, 22);
buttonPanel.add(approveButton, gridBagConstraints);
gridBagConstraints = new java.awt.GridBagConstraints();
gridBagConstraints.gridy = 6;
gridBagConstraints.gridwidth = java.awt.GridBagConstraints.REMAINDER;
gridBagConstraints.fill = java.awt.GridBagConstraints.BOTH;
gridBagConstraints.insets = new java.awt.Insets(14, 0, 0, 0);
fc.add(buttonPanel, gridBagConstraints);
add(fc, java.awt.BorderLayout.CENTER);
}// //GEN-END:initComponents
// Variables declaration - do not modify//GEN-BEGIN:variables
private javax.swing.JPanel accessoryPanel;
private javax.swing.JButton approveButton;
private ch.randelshofer.quaqua.JBrowser browser;
private javax.swing.JScrollPane browserScrollPane;
private javax.swing.JPanel buttonPanel;
private javax.swing.JButton cancelButton;
private javax.swing.JComboBox directoryComboBox;
private javax.swing.JPanel fc;
private javax.swing.JLabel fileNameLabel;
private javax.swing.JTextField fileNameTextField;
private javax.swing.JLabel filesOfTypeLabel;
private javax.swing.JComboBox filterComboBox;
private javax.swing.JPanel formatPanel;
private javax.swing.JPanel formatPanel2;
private javax.swing.JPanel fromPanel;
private javax.swing.JLabel lookInLabel;
private javax.swing.JButton newFolderButton;
private javax.swing.JPanel separatorPanel;
private javax.swing.JPanel separatorPanel1;
private javax.swing.JPanel separatorPanel2;
private javax.swing.JPanel strutPanel1;
private javax.swing.JPanel strutPanel2;
// End of variables declaration//GEN-END:variables
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy