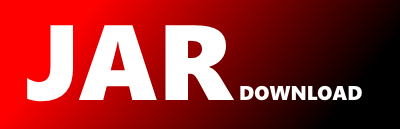
test.ActivationTest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of Quaqua Show documentation
Show all versions of Quaqua Show documentation
A Mavenisation of the Quaqua Mac OSX Swing Look and Feel (Java library)
Quaqua Look and Feel (C) 2003-2010, Werner Randelshofer.
Mavenisation by Matt Gumbley, DevZendo.org - for problems with
Mavenisation, see Matt; for issues with Quaqua, see the Quaqua home page.
For full license details, see http://randelshofer.ch/quaqua/license.html
The newest version!
/*
* @(#)ActivationTest.java 1.0 2009-10-13
*
* Copyright (c) 2009 Werner Randelshofer, Immensee, Switzerland.
* All rights reserved.
*
* You may not use, copy or modify this file, except in compliance with the
* license agreement you entered into with Werner Randelshofer.
* For details see accompanying license terms.
*/
package test;
import java.awt.FileDialog;
import java.beans.PropertyChangeEvent;
import java.beans.PropertyChangeListener;
import javax.swing.*;
import javax.swing.border.EmptyBorder;
/**
* ActivationTest.
*
* @author Werner Randelshofer
* @version 1.0 2009-10-13 Created.
*/
public class ActivationTest extends javax.swing.JFrame {
/** Creates new form ActivationTest */
public ActivationTest() {
getRootPane().putClientProperty("apple.awt.brushMetalLook", Boolean.TRUE);
initComponents();
((JComponent) getContentPane()).setBorder(new EmptyBorder(18, 20, 22, 20));
helpButton.putClientProperty("JButton.buttonType", "help");
helpButton.putClientProperty("Quaqua.Button.style", "help");
// Display the active state of the window in a JLabel
stateLabel.addPropertyChangeListener(new PropertyChangeListener() {
public void propertyChange(PropertyChangeEvent evt) {
if (evt.getPropertyName() == "Frame.active") {
stateLabel.setText(((Boolean) evt.getNewValue()).booleanValue() ? "Active" : "Inactive");
}
}
});
setSize(400, 300);
}
/** This method is called from within the constructor to
* initialize the form.
* WARNING: Do NOT modify this code. The content of this method is
* always regenerated by the Form Editor.
*/
// //GEN-BEGIN:initComponents
private void initComponents() {
java.awt.GridBagConstraints gridBagConstraints;
stateLabel = new javax.swing.JLabel();
helpButton = new javax.swing.JButton();
dialogButton = new javax.swing.JButton();
FormListener formListener = new FormListener();
setDefaultCloseOperation(javax.swing.WindowConstants.EXIT_ON_CLOSE);
getContentPane().setLayout(new java.awt.GridBagLayout());
stateLabel.setHorizontalAlignment(javax.swing.SwingConstants.CENTER);
stateLabel.setText("Active");
gridBagConstraints = new java.awt.GridBagConstraints();
gridBagConstraints.gridwidth = java.awt.GridBagConstraints.REMAINDER;
gridBagConstraints.weightx = 1.0;
gridBagConstraints.weighty = 1.0;
getContentPane().add(stateLabel, gridBagConstraints);
getContentPane().add(helpButton, new java.awt.GridBagConstraints());
dialogButton.setText("Open File Dialog");
dialogButton.addActionListener(formListener);
gridBagConstraints = new java.awt.GridBagConstraints();
gridBagConstraints.anchor = java.awt.GridBagConstraints.EAST;
getContentPane().add(dialogButton, gridBagConstraints);
pack();
}
// Code for dispatching events from components to event handlers.
private class FormListener implements java.awt.event.ActionListener {
FormListener() {}
public void actionPerformed(java.awt.event.ActionEvent evt) {
if (evt.getSource() == dialogButton) {
ActivationTest.this.openFileDialog(evt);
}
}
}// //GEN-END:initComponents
private void openFileDialog(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_openFileDialog
new FileDialog(this).setVisible(true);
}//GEN-LAST:event_openFileDialog
/**
* @param args the command line arguments
*/
public static void main(String args[]) throws Exception {
UIManager.setLookAndFeel("ch.randelshofer.quaqua.QuaquaLookAndFeel");
java.awt.EventQueue.invokeLater(new Runnable() {
public void run() {
new ActivationTest().setVisible(true);
}
});
}
// Variables declaration - do not modify//GEN-BEGIN:variables
private javax.swing.JButton dialogButton;
private javax.swing.JButton helpButton;
private javax.swing.JLabel stateLabel;
// End of variables declaration//GEN-END:variables
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy