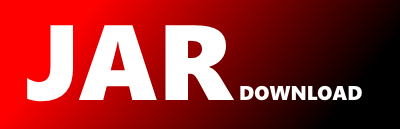
test.CheckBoxTest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of Quaqua Show documentation
Show all versions of Quaqua Show documentation
A Mavenisation of the Quaqua Mac OSX Swing Look and Feel (Java library)
Quaqua Look and Feel (C) 2003-2010, Werner Randelshofer.
Mavenisation by Matt Gumbley, DevZendo.org - for problems with
Mavenisation, see Matt; for issues with Quaqua, see the Quaqua home page.
For full license details, see http://randelshofer.ch/quaqua/license.html
The newest version!
/*
* @(#)CheckBoxTest.java 1.0 13 February 2005
*
* Copyright (c) 2004 Werner Randelshofer, Immensee, Switzerland.
* All rights reserved.
*
* You may not use, copy or modify this file, except in compliance with the
* license agreement you entered into with Werner Randelshofer.
* For details see accompanying license terms.
*/
package test;
import ch.randelshofer.quaqua.*;
import java.awt.*;
import javax.swing.*;
import javax.swing.border.*;
/**
* CheckBoxTest.
*
* @author Werner Randelshofer
* @version 1.0 13 February 2005 Created.
*/
public class CheckBoxTest extends javax.swing.JPanel {
/** Creates new form. */
public CheckBoxTest() {
initComponents();
// Leopard properties
if (QuaquaManager.getDesign() == QuaquaManager.LEOPARD) {
checkBox3.putClientProperty("JComponent.sizeVariant","small");
checkBox4.putClientProperty("JComponent.sizeVariant","small");
}
}
public static void main(String args[]) {
try {
UIManager.setLookAndFeel(QuaquaManager.getLookAndFeelClassName());
} catch (Exception e) {
e.printStackTrace();
}
JFrame f = new JFrame("Quaqua CheckBox Test");
f.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
f.getContentPane().add(new CheckBoxTest());
((JComponent) f.getContentPane()).setBorder(new EmptyBorder(9,17,17,17));
f.pack();
f.setVisible(true);
}
/** This method is called from within the constructor to
* initialize the form.
* WARNING: Do NOT modify this code. The content of this method is
* always regenerated by the Form Editor.
*/
// //GEN-BEGIN:initComponents
private void initComponents() {
java.awt.GridBagConstraints gridBagConstraints;
checkBox1 = new javax.swing.JCheckBox();
enabledLabel = new javax.swing.JLabel();
checkBox2 = new javax.swing.JCheckBox();
disabledLabel = new javax.swing.JLabel();
separator = new javax.swing.JSeparator();
smallLabel = new javax.swing.JLabel();
checkBox3 = new javax.swing.JCheckBox();
checkBox4 = new javax.swing.JCheckBox();
jSeparator1 = new javax.swing.JSeparator();
jCheckBox5 = new javax.swing.JCheckBox();
largeLabel = new javax.swing.JLabel();
setBorder(javax.swing.BorderFactory.createEmptyBorder(16, 17, 17, 17));
setLayout(new java.awt.GridBagLayout());
checkBox1.setSelected(true);
checkBox1.setText("Ångström H");
gridBagConstraints = new java.awt.GridBagConstraints();
gridBagConstraints.gridx = 0;
gridBagConstraints.anchor = java.awt.GridBagConstraints.WEST;
add(checkBox1, gridBagConstraints);
enabledLabel.setText("Enabled");
gridBagConstraints = new java.awt.GridBagConstraints();
gridBagConstraints.gridx = 1;
gridBagConstraints.gridy = 0;
gridBagConstraints.anchor = java.awt.GridBagConstraints.WEST;
gridBagConstraints.insets = new java.awt.Insets(0, 20, 0, 0);
add(enabledLabel, gridBagConstraints);
checkBox2.setSelected(true);
checkBox2.setText("Ångström H");
checkBox2.setEnabled(false);
gridBagConstraints = new java.awt.GridBagConstraints();
gridBagConstraints.gridx = 0;
gridBagConstraints.anchor = java.awt.GridBagConstraints.WEST;
add(checkBox2, gridBagConstraints);
disabledLabel.setText("Disabled");
gridBagConstraints = new java.awt.GridBagConstraints();
gridBagConstraints.gridx = 1;
gridBagConstraints.anchor = java.awt.GridBagConstraints.WEST;
gridBagConstraints.insets = new java.awt.Insets(0, 20, 0, 0);
add(disabledLabel, gridBagConstraints);
gridBagConstraints = new java.awt.GridBagConstraints();
gridBagConstraints.gridy = 3;
gridBagConstraints.gridwidth = 2;
gridBagConstraints.fill = java.awt.GridBagConstraints.HORIZONTAL;
gridBagConstraints.insets = new java.awt.Insets(12, 0, 12, 0);
add(separator, gridBagConstraints);
smallLabel.setFont(new java.awt.Font("Lucida Grande", 0, 11));
smallLabel.setText("Small Size");
gridBagConstraints = new java.awt.GridBagConstraints();
gridBagConstraints.gridx = 1;
gridBagConstraints.gridy = 4;
gridBagConstraints.anchor = java.awt.GridBagConstraints.WEST;
gridBagConstraints.insets = new java.awt.Insets(0, 20, 0, 0);
add(smallLabel, gridBagConstraints);
checkBox3.setFont(new java.awt.Font("Lucida Grande", 0, 11));
checkBox3.setSelected(true);
checkBox3.setText("Ångström H");
gridBagConstraints = new java.awt.GridBagConstraints();
gridBagConstraints.gridx = 0;
gridBagConstraints.anchor = java.awt.GridBagConstraints.WEST;
add(checkBox3, gridBagConstraints);
checkBox4.setFont(new java.awt.Font("Lucida Grande", 0, 11));
checkBox4.setSelected(true);
checkBox4.setText("Ångström H");
checkBox4.setEnabled(false);
gridBagConstraints = new java.awt.GridBagConstraints();
gridBagConstraints.gridx = 0;
gridBagConstraints.anchor = java.awt.GridBagConstraints.WEST;
add(checkBox4, gridBagConstraints);
gridBagConstraints = new java.awt.GridBagConstraints();
gridBagConstraints.gridx = 0;
gridBagConstraints.gridwidth = java.awt.GridBagConstraints.REMAINDER;
gridBagConstraints.fill = java.awt.GridBagConstraints.HORIZONTAL;
gridBagConstraints.insets = new java.awt.Insets(12, 0, 12, 0);
add(jSeparator1, gridBagConstraints);
jCheckBox5.setFont(new java.awt.Font("Lucida Grande", 0, 24)); // NOI18N
jCheckBox5.setText("Ångström H");
gridBagConstraints = new java.awt.GridBagConstraints();
gridBagConstraints.anchor = java.awt.GridBagConstraints.NORTHWEST;
gridBagConstraints.weighty = 1.0;
add(jCheckBox5, gridBagConstraints);
largeLabel.setText("Large Size");
gridBagConstraints = new java.awt.GridBagConstraints();
gridBagConstraints.gridx = 1;
gridBagConstraints.anchor = java.awt.GridBagConstraints.NORTHWEST;
gridBagConstraints.insets = new java.awt.Insets(0, 20, 0, 0);
add(largeLabel, gridBagConstraints);
}// //GEN-END:initComponents
// Variables declaration - do not modify//GEN-BEGIN:variables
private javax.swing.JCheckBox checkBox1;
private javax.swing.JCheckBox checkBox2;
private javax.swing.JCheckBox checkBox3;
private javax.swing.JCheckBox checkBox4;
private javax.swing.JLabel disabledLabel;
private javax.swing.JLabel enabledLabel;
private javax.swing.JCheckBox jCheckBox5;
private javax.swing.JSeparator jSeparator1;
private javax.swing.JLabel largeLabel;
private javax.swing.JSeparator separator;
private javax.swing.JLabel smallLabel;
// End of variables declaration//GEN-END:variables
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy