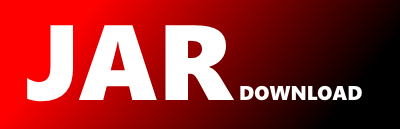
test.JLazyPanel Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of Quaqua Show documentation
Show all versions of Quaqua Show documentation
A Mavenisation of the Quaqua Mac OSX Swing Look and Feel (Java library)
Quaqua Look and Feel (C) 2003-2010, Werner Randelshofer.
Mavenisation by Matt Gumbley, DevZendo.org - for problems with
Mavenisation, see Matt; for issues with Quaqua, see the Quaqua home page.
For full license details, see http://randelshofer.ch/quaqua/license.html
The newest version!
/*
* @(#)JLazyPanel.java
*
* Copyright (c) 2005-2009 Werner Randelshofer, Immensee, Switzerland.
* All rights reserved.
*
* You may not use, copy or modify this file, except in compliance with the
* license agreement you entered into with Werner Randelshofer.
* For details see accompanying license terms.
*/
package test;
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
/**
* A JLazyPanel creates its child component only when it becomes visible or
* when it is painted.
* JLazyPanel is intended for use as a child of a JTabbedPane.
*
* @author Werner Randelshofer
* @version $Id: JLazyPanel.java 363 2010-11-21 17:41:04Z wrandelshofer $
*/
public class JLazyPanel extends javax.swing.JPanel {
private String childClassName;
/**
* Creates a new instance.
*/
public JLazyPanel() {
initComponents();
addComponentListener(new ComponentAdapter() {
public void componentShown(ComponentEvent e) {
instantiateChild();
JLazyPanel.this.removeComponentListener(this);
}
});
}
public JLazyPanel(String childClassName) {
this();
setChildClassName(childClassName);
}
public void setChildClassName(String childClassName) {
this.childClassName = childClassName;
}
private void instantiateChild() {
if (childClassName != null) {
long start = System.currentTimeMillis();
try {
Class childClass = Class.forName(childClassName);
Component child = (Component) childClass.newInstance();
add(child);
} catch (Throwable e) {
add(new JLabel("Unable to instantiate "+childClassName));
e.printStackTrace();
}
long end = System.currentTimeMillis();
System.out.println("create "+childClassName+" "+(end-start));
childClassName = null;
start = end;
applyComponentOrientation(SwingUtilities.getRoot(this).getComponentOrientation());
validate();
end = System.currentTimeMillis();
System.out.println("validate "+(end-start));
}
}
/** This method is called from within the constructor to
* initialize the form.
* WARNING: Do NOT modify this code. The content of this method is
* always regenerated by the Form Editor.
*/
// //GEN-BEGIN:initComponents
private void initComponents() {
setLayout(new java.awt.BorderLayout());
}// //GEN-END:initComponents
@Override
public void paint(Graphics g) {
super.paint(g);
if (childClassName != null) {
SwingUtilities.invokeLater(new Runnable() {
public void run() { instantiateChild(); }
});
}
}
// Variables declaration - do not modify//GEN-BEGIN:variables
// End of variables declaration//GEN-END:variables
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy