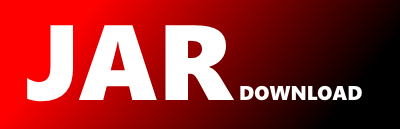
test.ToolBarTest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of Quaqua Show documentation
Show all versions of Quaqua Show documentation
A Mavenisation of the Quaqua Mac OSX Swing Look and Feel (Java library)
Quaqua Look and Feel (C) 2003-2010, Werner Randelshofer.
Mavenisation by Matt Gumbley, DevZendo.org - for problems with
Mavenisation, see Matt; for issues with Quaqua, see the Quaqua home page.
For full license details, see http://randelshofer.ch/quaqua/license.html
The newest version!
/*
* @(#)ToolBarTest.java
*
* Copyright (c) 2004-2010 Werner Randelshofer, Immensee, Switzerland.
* All rights reserved.
*
* You may not use, copy or modify this file, except in compliance with the
* license agreement you entered into with Werner Randelshofer.
* For details see accompanying license terms.
*/
package test;
import java.awt.BorderLayout;
import java.awt.Window;
import java.awt.event.*;
import java.awt.peer.FramePeer;
import javax.swing.*;
/**
* ToolBarTest.
*
* @author Werner Randelshofer
* @version $Id: ToolBarTest.java 363 2010-11-21 17:41:04Z wrandelshofer $
*/
public class ToolBarTest extends javax.swing.JPanel {
private static JToolBar unifiedToolBar;
private static JToolBar unifiedStatusBar;
/** Creates new form. */
public ToolBarTest() {
initComponents();
setOpaque(true);
// toolBar.putClientProperty("JToolBar.isRollover", Boolean.TRUE);
if (unifiedToolBar != null) {
unifiedToolBarBox.setSelected(true);
toolBarPanel.remove(toolBar);
toolBar = unifiedToolBar;
}
if (unifiedStatusBar != null) {
unifiedStatusBarBox.setSelected(true);
remove(statusBar);
statusBar = unifiedStatusBar;
}
}
/** This method is called from within the constructor to
* initialize the form.
* WARNING: Do NOT modify this code. The content of this method is
* always regenerated by the Form Editor.
*/
// //GEN-BEGIN:initComponents
private void initComponents() {
java.awt.GridBagConstraints gridBagConstraints;
statusBar = new javax.swing.JToolBar();
statusLabel = new javax.swing.JLabel();
toolBarPanel = new javax.swing.JPanel();
toolBar = new javax.swing.JToolBar();
folderButton = new javax.swing.JButton();
fileButton = new javax.swing.JButton();
jPanel2 = new javax.swing.JPanel();
unifiedToolBarBox = new javax.swing.JCheckBox();
unifiedStatusBarBox = new javax.swing.JCheckBox();
setLayout(new java.awt.BorderLayout());
statusBar.setFloatable(false);
statusLabel.setText("A status bar");
statusBar.add(statusLabel);
add(statusBar, java.awt.BorderLayout.SOUTH);
toolBarPanel.setLayout(new java.awt.BorderLayout());
toolBar.setName("ToolBar with Title"); // NOI18N
folderButton.setIcon(UIManager.getIcon("FileView.directoryIcon"));
folderButton.setText("Folder");
folderButton.setFocusable(false);
folderButton.setHorizontalTextPosition(javax.swing.SwingConstants.CENTER);
folderButton.setVerticalTextPosition(javax.swing.SwingConstants.BOTTOM);
toolBar.add(folderButton);
fileButton.setIcon(UIManager.getIcon("FileView.fileIcon"));
fileButton.setText("File");
fileButton.setFocusable(false);
fileButton.setHorizontalTextPosition(javax.swing.SwingConstants.CENTER);
fileButton.setVerticalTextPosition(javax.swing.SwingConstants.BOTTOM);
toolBar.add(fileButton);
toolBarPanel.add(toolBar, java.awt.BorderLayout.NORTH);
jPanel2.setLayout(new java.awt.GridBagLayout());
unifiedToolBarBox.setText("Unified 'title' tool bar");
unifiedToolBarBox.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
unifiedToolBarPerformed(evt);
}
});
gridBagConstraints = new java.awt.GridBagConstraints();
gridBagConstraints.anchor = java.awt.GridBagConstraints.WEST;
jPanel2.add(unifiedToolBarBox, gridBagConstraints);
unifiedStatusBarBox.setText("Unified 'bottom' tool bar");
unifiedStatusBarBox.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
unifiedStatusBarPerformed(evt);
}
});
gridBagConstraints = new java.awt.GridBagConstraints();
gridBagConstraints.gridx = 0;
gridBagConstraints.anchor = java.awt.GridBagConstraints.WEST;
jPanel2.add(unifiedStatusBarBox, gridBagConstraints);
toolBarPanel.add(jPanel2, java.awt.BorderLayout.CENTER);
add(toolBarPanel, java.awt.BorderLayout.CENTER);
}// //GEN-END:initComponents
private void unifiedToolBarPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_unifiedToolBarPerformed
JRootPane rp = SwingUtilities.getRootPane(this);
JPanel cp = (JPanel) rp.getContentPane();
if (unifiedToolBarBox.isSelected()) {
cp.add(toolBar, BorderLayout.NORTH);
toolBar.putClientProperty("Quaqua.ToolBar.style", "title");
unifiedToolBar = toolBar;
toolBar.setFloatable(false);
} else {
toolBarPanel.add(toolBar, BorderLayout.NORTH);
toolBar.putClientProperty("Quaqua.ToolBar.style", "plain");
toolBar.setFloatable(true);
unifiedToolBar = null;
}
cp.revalidate();
rp.putClientProperty("apple.awt.brushMetalLook",//
unifiedToolBarBox.isSelected()/* || unifiedStatusBarBox.isSelected()*/);
Window f=(Window)rp.getParent();
f.dispose();
f.setVisible(true);
}//GEN-LAST:event_unifiedToolBarPerformed
private void unifiedStatusBarPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_unifiedStatusBarPerformed
JRootPane rp = SwingUtilities.getRootPane(this);
JPanel cp = (JPanel) rp.getContentPane();
if (unifiedStatusBarBox.isSelected()) {
cp.add(statusBar, BorderLayout.SOUTH);
unifiedStatusBar = statusBar;
statusBar.putClientProperty("Quaqua.ToolBar.style", "bottom");
} else {
add(statusBar, BorderLayout.SOUTH);
statusBar.putClientProperty("Quaqua.ToolBar.style", "plain");
unifiedStatusBar = null;
}
cp.revalidate();
rp.putClientProperty("apple.awt.brushMetalLook",//
unifiedToolBarBox.isSelected() /*|| unifiedStatusBarBox.isSelected()*/);
Window f=(Window)rp.getParent();
f.dispose();
f.setVisible(true);
}//GEN-LAST:event_unifiedStatusBarPerformed
// Variables declaration - do not modify//GEN-BEGIN:variables
private javax.swing.JButton fileButton;
private javax.swing.JButton folderButton;
private javax.swing.JPanel jPanel2;
private javax.swing.JToolBar statusBar;
private javax.swing.JLabel statusLabel;
private javax.swing.JToolBar toolBar;
private javax.swing.JPanel toolBarPanel;
private javax.swing.JCheckBox unifiedStatusBarBox;
private javax.swing.JCheckBox unifiedToolBarBox;
// End of variables declaration//GEN-END:variables
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy