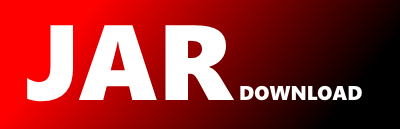
eu.europa.esig.dss.asic.validation.ASiCContainerWithCAdESValidator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of dss-asic-cades Show documentation
Show all versions of dss-asic-cades Show documentation
DSS ASiC with CAdES contains the code for the creation and validation of ASiC containers with CAdES signature(s).
package eu.europa.esig.dss.asic.validation;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import eu.europa.esig.dss.ASiCContainerType;
import eu.europa.esig.dss.DSSDocument;
import eu.europa.esig.dss.asic.ASiCUtils;
import eu.europa.esig.dss.asic.ASiCWithCAdESContainerExtractor;
import eu.europa.esig.dss.asic.AbstractASiCContainerExtractor;
import eu.europa.esig.dss.validation.DocumentValidator;
import eu.europa.esig.dss.validation.ManifestFile;
/**
* This class is an implementation to validate ASiC containers with CAdES signature(s)
*
*/
public class ASiCContainerWithCAdESValidator extends AbstractASiCContainerValidator {
private static final Logger LOG = LoggerFactory.getLogger(ASiCContainerWithCAdESValidator.class);
private ASiCContainerWithCAdESValidator() {
super(null);
}
public ASiCContainerWithCAdESValidator(final DSSDocument asicContainer) {
super(asicContainer);
analyseEntries();
}
@Override
public boolean isSupported(DSSDocument dssDocument) {
return ASiCUtils.isASiCContainer(dssDocument) && ASiCUtils.isArchiveContainsCorrectSignatureExtension(dssDocument, ".p7s");
}
@Override
AbstractASiCContainerExtractor getArchiveExtractor() {
return new ASiCWithCAdESContainerExtractor(document);
}
@Override
List getValidators() {
if (validators == null) {
validators = new ArrayList();
for (final DSSDocument signature : getSignatureDocuments()) {
CMSDocumentForASiCValidator cadesValidator = new CMSDocumentForASiCValidator(signature);
cadesValidator.setCertificateVerifier(certificateVerifier);
cadesValidator.setProcessExecutor(processExecutor);
cadesValidator.setSignaturePolicyProvider(signaturePolicyProvider);
cadesValidator.setValidationCertPool(validationCertPool);
cadesValidator.setDetachedContents(getSignedDocuments(signature));
validators.add(cadesValidator);
}
}
return validators;
}
private List getSignedDocuments(DSSDocument signature) {
ASiCContainerType type = getContainerType();
if (ASiCContainerType.ASiC_S == type) {
return getSignedDocuments(); // Collection size should be equals 1
} else if (ASiCContainerType.ASiC_E == type) {
// the manifest file is signed
// we need first to check the manifest file and its digests
ASiCEWithCAdESManifestValidator manifestValidator = new ASiCEWithCAdESManifestValidator(signature, getManifestDocuments(), getSignedDocuments());
DSSDocument linkedManifest = manifestValidator.getLinkedManifest();
if (linkedManifest != null) {
return Arrays.asList(linkedManifest);
} else {
return Collections.emptyList();
}
} else {
LOG.warn("Unknown asic container type (returns all signed documents)");
return getSignedDocuments();
}
}
@Override
protected List getManifestFilesDecriptions() {
List descriptions = new ArrayList();
List manifestDocuments = getManifestDocuments();
for (DSSDocument manifestDocument : manifestDocuments) {
ASiCEWithCAdESManifestParser parser = new ASiCEWithCAdESManifestParser(manifestDocument);
descriptions.add(parser.getDescription());
}
return descriptions;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy