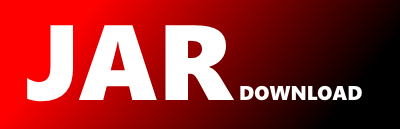
port.org.bouncycastle.asn1.LazyEncodedSequence Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of dss-model Show documentation
Show all versions of dss-model Show documentation
DSS Model contains the data model representation for DSS
package port.org.bouncycastle.asn1;
import java.io.IOException;
import java.util.Enumeration;
/**
* Note: this class is for processing DER/DL encoded sequences only.
*/
class LazyEncodedSequence extends ASN1Sequence {
private byte[] encoded;
LazyEncodedSequence(byte[] encoded) throws IOException {
this.encoded = encoded;
}
private void parse() {
Enumeration en = new LazyConstructionEnumeration(encoded);
while (en.hasMoreElements()) {
seq.addElement(en.nextElement());
}
encoded = null;
}
@Override
public synchronized ASN1Encodable getObjectAt(int index) {
if (encoded != null) {
parse();
}
return super.getObjectAt(index);
}
@Override
public synchronized Enumeration getObjects() {
if (encoded == null) {
return super.getObjects();
}
return new LazyConstructionEnumeration(encoded);
}
@Override
public synchronized int size() {
if (encoded != null) {
parse();
}
return super.size();
}
@Override
ASN1Primitive toDERObject() {
if (encoded != null) {
parse();
}
return super.toDERObject();
}
@Override
ASN1Primitive toDLObject() {
if (encoded != null) {
parse();
}
return super.toDLObject();
}
@Override
int encodedLength() throws IOException {
if (encoded != null) {
return 1 + StreamUtil.calculateBodyLength(encoded.length) + encoded.length;
} else {
return super.toDLObject().encodedLength();
}
}
@Override
void encode(ASN1OutputStream out) throws IOException {
if (encoded != null) {
out.writeEncoded(BERTags.SEQUENCE | BERTags.CONSTRUCTED, encoded);
} else {
super.toDLObject().encode(out);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy