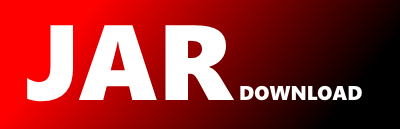
eu.europa.esig.xmldsig.XSDAbstractUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of specs-xmldsig Show documentation
Show all versions of specs-xmldsig Show documentation
Generated sources from the xmldsig XSD
Please wait ...
© 2015 - 2025 Weber Informatics LLC | Privacy Policy