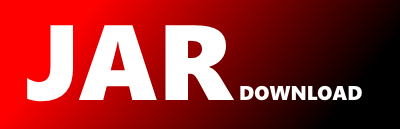
org.dihedron.patterns.functional.Functional Maven / Gradle / Ivy
/**
* Copyright (c) 2012-2014, Andrea Funto'. All rights reserved.
*
* This file is part of the Dihedron Common Utilities library ("Commons").
*
* "Commons" is free software: you can redistribute it and/or modify it under
* the terms of the GNU Lesser General Public License as published by the Free
* Software Foundation, either version 3 of the License, or (at your option)
* any later version.
*
* "Commons" is distributed in the hope that it will be useful, but WITHOUT ANY
* WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS FOR
* A PARTICULAR PURPOSE. See the GNU Lesser General Public License for more
* details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with "Commons". If not, see .
*/
package org.dihedron.patterns.functional;
import java.util.List;
import java.util.Map;
import java.util.Map.Entry;
import java.util.Set;
/**
* @author Andrea Funto'
*/
public abstract class Functional {
/**
* Returns a functional wrapper for the input list, providing a way to apply
* a function on all its elements in a functional style.
*
* @param list
* the list to which the functor will be applied.
* @return
* a list that also extends this abstract class.
*/
public static final Functional functionalList(List list) {
return new FunctionalList(list);
}
/**
* Returns a functional wrapper for the input set, providing a way to apply
* a function on all its elements in a functional style.
*
* @param set
* the set to which the functor will be applied.
* @return
* a set that also extends this abstract class.
*/
public static final Functional functionalSet(Set set) {
return new FunctionalSet(set);
}
/**
* Returns a functional wrapper for the input map, providing a way to apply
* a function on all its entries in a functional style.
*
* @param map
* the map to which the functor will be applied.
* @return
* a map that also extends this abstract class.
*/
public static final Functional functionalMap(Map map) {
return new FunctionalMap(map);
}
/**
* Applies the given functor to all elements in the input list.
*
* @param list
* the list to which the functor will be applied.
* @param state
* an state variable, that will be used as the return vale for the iteration.
* @param functor
* a function to be applied to all elements in the list.
* @return
* the state object after the processing.
*/
public static final S forEach(List list, S state, Fx functor) {
return new FunctionalList(list).forEach(state, functor);
}
/**
* Applies the given functor to all elements in the input set.
*
* @param set
* the set to which the functor will be applied.
* @param state
* an state variable, that will be used as the return vale for the iteration.
* @param functor
* a function to be applied to all elements in the set.
* @return
* the state object after the processing.
*/
public static final S forEach(Set set, S state, Fx functor) {
return new FunctionalSet(set).forEach(state, functor);
}
/**
* Applies the given functor to all entries in the input map.
*
* @param map
* the map to which the functor will be applied.
* @param state
* an state variable, that will be used as the return vale for the iteration.
* @param functor
* a function to be applied to all entries in the map.
* @return
* the state object after the processing.
*/
public static final S forEach(Map map, S state, Fx> functor) {
return new FunctionalMap(map).forEach(state, functor);
}
/**
* Iterates over the collection elements or entries and passing each of them
* to the given implementation of the functor interface; if state needs to be
* propagated, it can be instantiated and returned by the first invocation of
* the functor and it will be passed along the next elements of the collection.
*
* @param functor
* an implementation of the {@code $} functor interface.
* @return
* the result of the iteration.
*/
public S forEach(Fx functor) {
return forEach(null, functor);
}
/**
* Iterates over the collection elements or entries and passing each of them
* to the given implementation of the functor interface; if state is provided
* it will be it will be passed along the elements of the collection to the
* functor.
*
* @param state
* a state variable to be used during the processing.
* @param functor
* an implementation of the {@code $} functor interface.
* @return
* the result of the iteration.
*/
public abstract S forEach(S state, Fx functor);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy