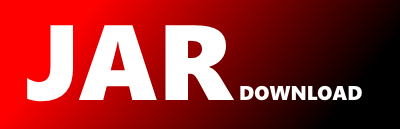
org.diirt.datasource.extra.DynamicGroup Maven / Gradle / Ivy
/**
* Copyright (C) 2010-14 diirt developers. See COPYRIGHT.TXT
* All rights reserved. Use is subject to license terms. See LICENSE.TXT
*/
package org.diirt.datasource.extra;
import java.util.ArrayList;
import java.util.List;
import org.diirt.datasource.ReadRecipe;
import org.diirt.datasource.DataSource;
import org.diirt.datasource.expression.DesiredRateExpression;
import org.diirt.datasource.expression.DesiredRateExpressionImpl;
import org.diirt.datasource.ExceptionHandler;
import org.diirt.datasource.PVManager;
import org.diirt.datasource.expression.DesiredRateExpressionListImpl;
/**
* A expression that returns the result of a dynamically managed group.
* Once the group is created, any {@link DesiredRateExpression} can be
* added dynamically. The exceptions eventually generated by those
* expressions can be obtained through {@link #lastExceptions() }.
*
* @author carcassi
*/
public class DynamicGroup extends DesiredRateExpressionImpl> {
private final DataSource dataSource = PVManager.getDefaultDataSource();
private final List recipes = new ArrayList();
/**
* Creates a new group.
*/
public DynamicGroup() {
super(new DesiredRateExpressionListImpl
© 2015 - 2025 Weber Informatics LLC | Privacy Policy