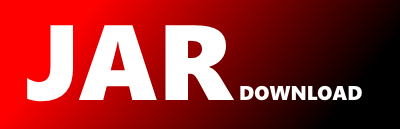
org.diirt.datasource.extra.DynamicGroupFunction Maven / Gradle / Ivy
/**
* Copyright (C) 2010-14 diirt developers. See COPYRIGHT.TXT
* All rights reserved. Use is subject to license terms. See LICENSE.TXT
*/
package org.diirt.datasource.extra;
import java.util.ArrayList;
import java.util.List;
import org.diirt.datasource.ReadFunction;
/**
* Function that implements the dynamic group.
*
* @author carcassi
*/
class DynamicGroupFunction implements ReadFunction> {
// Guarded by this
private final List> arguments = new ArrayList>();
// Guarded by this
private List exceptions = new ArrayList();
// Gaurded by this
private List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy