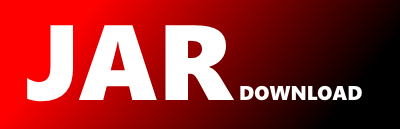
org.diirt.datasource.sample.MonitorChannel Maven / Gradle / Ivy
The newest version!
/**
* Copyright (C) 2010-18 diirt developers. See COPYRIGHT.TXT
* All rights reserved. Use is subject to license terms. See LICENSE.TXT
*/
package org.diirt.datasource.sample;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import org.diirt.datasource.PVManager;
import org.diirt.datasource.PVReader;
import org.diirt.datasource.PVReaderEvent;
import org.diirt.datasource.PVReaderListener;
import org.diirt.datasource.formula.ExpressionLanguage;
import java.time.Duration;
/**
* Prompts for a channel name, connects and shows the events.
*
* @author carcassi
*/
public class MonitorChannel {
public static void main(String[] args) throws Exception {
// Prompt for channel
System.out.print("Enter channel: ");
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
String channelName;
try {
channelName = br.readLine();
} catch (IOException ioe) {
System.out.println("IO error trying to read the channel name");
System.exit(1);
return;
}
System.out.println("Starting channel " + channelName);
PVReader> reader = PVManager.read(ExpressionLanguage.formula(channelName))
.readListener(new PVReaderListener
© 2015 - 2025 Weber Informatics LLC | Privacy Policy