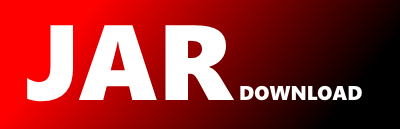
org.diirt.util.array.ListNumber Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of diirt-util Show documentation
Show all versions of diirt-util Show documentation
Basic Java utility classes to be shared across projects until
suitable replacements are available in the JDK.
The newest version!
/**
* Copyright (C) 2010-18 diirt developers. See COPYRIGHT.TXT
* All rights reserved. Use is subject to license terms. See LICENSE.TXT
*/
package org.diirt.util.array;
/**
* An ordered collection of numeric (primitive) elements.
*
* @author Gabriele Carcassi
*/
public interface ListNumber extends CollectionNumber {
/**
* Returns the element at the specified position in this list casted to a double.
*
* @param index position of the element to return
* @return the element at the specified position in this list
* @throws IndexOutOfBoundsException if the index is out of range
* (index < 0 || index >= size())
*/
double getDouble(int index);
/**
* Returns the element at the specified position in this list casted to a float.
*
* @param index position of the element to return
* @return the element at the specified position in this list
* @throws IndexOutOfBoundsException if the index is out of range
* (index < 0 || index >= size())
*/
float getFloat(int index);
/**
* Returns the element at the specified position in this list casted to a long.
*
* @param index position of the element to return
* @return the element at the specified position in this list
* @throws IndexOutOfBoundsException if the index is out of range
* (index < 0 || index >= size())
*/
long getLong(int index);
/**
* Returns the element at the specified position in this list casted to an int.
*
* @param index position of the element to return
* @return the element at the specified position in this list
* @throws IndexOutOfBoundsException if the index is out of range
* (index < 0 || index >= size())
*/
int getInt(int index);
/**
* Returns the element at the specified position in this list casted to a short.
*
* @param index position of the element to return
* @return the element at the specified position in this list
* @throws IndexOutOfBoundsException if the index is out of range
* (index < 0 || index >= size())
*/
short getShort(int index);
/**
* Returns the element at the specified position in this list casted to a byte.
*
* @param index position of the element to return
* @return the element at the specified position in this list
* @throws IndexOutOfBoundsException if the index is out of range
* (index < 0 || index >= size())
*/
byte getByte(int index);
/**
* Changes the element at the specified position, casting to the internal
* representation.
*
* @param index position of the element to change
* @param value the new value
* @throws IndexOutOfBoundsException if the index is out of range
* (index < 0 || index >= size())
*/
void setDouble(int index, double value);
/**
* Changes the element at the specified position, casting to the internal
* representation.
*
* @param index position of the element to change
* @param value the new value
* @throws IndexOutOfBoundsException if the index is out of range
* (index < 0 || index >= size())
*/
void setFloat(int index, float value);
/**
* Changes the element at the specified position, casting to the internal
* representation.
*
* @param index position of the element to change
* @param value the new value
* @throws IndexOutOfBoundsException if the index is out of range
* (index < 0 || index >= size())
*/
void setLong(int index, long value);
/**
* Changes the element at the specified position, casting to the internal
* representation.
*
* @param index position of the element to change
* @param value the new value
* @throws IndexOutOfBoundsException if the index is out of range
* (index < 0 || index >= size())
*/
void setInt(int index, int value);
/**
* Changes the element at the specified position, casting to the internal
* representation.
*
* @param index position of the element to change
* @param value the new value
* @throws IndexOutOfBoundsException if the index is out of range
* (index < 0 || index >= size())
*/
void setShort(int index, short value);
/**
* Changes the element at the specified position, casting to the internal
* representation.
*
* @param index position of the element to change
* @param value the new value
* @throws IndexOutOfBoundsException if the index is out of range
* (index < 0 || index >= size())
*/
void setByte(int index, byte value);
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy