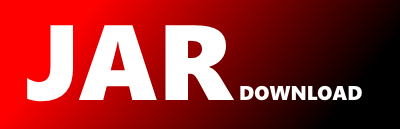
org.diirt.util.config.Configuration Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of diirt-util Show documentation
Show all versions of diirt-util Show documentation
Basic Java utility classes to be shared across projects until
suitable replacements are available in the JDK.
The newest version!
/**
* Copyright (C) 2010-18 diirt developers. See COPYRIGHT.TXT
* All rights reserved. Use is subject to license terms. See LICENSE.TXT
*/
package org.diirt.util.config;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.util.logging.Level;
import java.util.logging.Logger;
/**
* Entry point for all configuration in diirt.
*
* The configuration directory used is given by:
*
* - The Java property diirt.home if set. It can either be
* set when creating the JVM using -D or programmatically using
*
System.setProperty
. When set programmatically, one
* must make sure that it is set before any call to {@link #configurationDirectory()},
* since the property is only read once and then cached.
* - The environment variable DIIRT_HOME if set.
* - The default $USER_HOME/.diirt
*
*
* @author carcassi
*/
public class Configuration {
private static Logger log = Logger.getLogger(Configuration.class.getName());
private static File configurationDirectory = configurationDirectory();
private static File configurationDirectory() {
// First look for java property
String diirtHome = System.getProperty("diirt.home");
// Second look for environment variable
if (diirtHome == null) {
diirtHome = System.getenv("DIIRT_HOME");
}
File dir;
if (diirtHome != null) {
dir = new File(diirtHome);
} else {
// Third use default in home directory
dir = new File(System.getProperty("user.home"), ".diirt");
}
dir.mkdirs();
return dir;
}
public static synchronized File getDirectory() {
return configurationDirectory;
}
/**
* A temporary method added to allow the mapping of osgi preferences to java
* system properties. This is needed due to the limited options in
* controlling the startup order of java declarative services and osgi
* services
*/
public static synchronized void reset() {
configurationDirectory = configurationDirectory();
}
public static File getFile(String relativeFilePath, Object obj, String defaultResource) throws IOException {
File file = new File(Configuration.getDirectory(), relativeFilePath);
if (!file.exists()) {
file.getParentFile().mkdirs();
try (InputStream input = obj.getClass().getResourceAsStream(defaultResource);
OutputStream output = new FileOutputStream(file)) {
byte[] buffer = new byte[8 * 1024];
int bytesRead;
while ((bytesRead = input.read(buffer)) != -1) {
output.write(buffer, 0, bytesRead);
}
}
log.log(Level.INFO, "Initializing configuration file " + file);
}
log.log(Level.INFO, "Loading " + file);
return file;
}
public static InputStream getFileAsStream(String relativeFilePath, Object obj, String defaultResource) throws IOException {
return new FileInputStream(getFile(relativeFilePath, obj, defaultResource));
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy