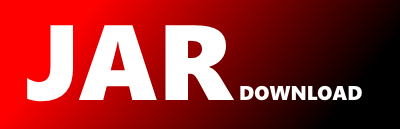
org.diirt.graphene.profile.impl.ProfileScatterGraph2D Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of graphene-profile Show documentation
Show all versions of graphene-profile Show documentation
Tools to profile the graph library.
/**
* Copyright (C) 2010-14 diirt developers. See COPYRIGHT.TXT
* All rights reserved. Use is subject to license terms. See LICENSE.TXT
*/
package org.diirt.graphene.profile.impl;
import org.diirt.graphene.InterpolationScheme;
import org.diirt.graphene.ScatterGraph2DRendererUpdate;
import org.diirt.graphene.ScatterGraph2DRenderer;
import org.diirt.graphene.Point2DDataset;
import org.diirt.graphene.Graph2DRendererUpdate;
import java.awt.Graphics2D;
import java.util.HashMap;
import java.util.LinkedHashMap;
import java.util.Map;
import org.diirt.graphene.profile.ProfileGraph2D;
import org.diirt.graphene.profile.utils.DatasetFactory;
/**
* Handles profiling for ScatterGraph2DRenderer
.
* Takes a Point2DDataset
dataset and repeatedly renders through a ScatterGraph2DRenderer
.
*
* @author asbarber
*/
public class ProfileScatterGraph2D extends ProfileGraph2D{
/**
* Gets a set of random Gaussian 2D point data.
* @return the appropriate Point2DDataset
data
*/
@Override
protected Point2DDataset getDataset() {
return DatasetFactory.makePoint2DGaussianRandomData(getNumDataPoints());
}
/**
* Returns the renderer used in the render loop.
* The 2D point is rendered by a ScatterGraph2DRenderer
.
* @param imageWidth width of rendered image in pixels
* @param imageHeight height of rendered image in pixels
* @return a scatter graph to draw the data
*/
@Override
protected ScatterGraph2DRenderer getRenderer(int imageWidth, int imageHeight) {
return new ScatterGraph2DRenderer(imageWidth, imageHeight);
}
/**
* Draws the 2D point data in a scatter graph.
* Primary method in the render loop.
* @param graphics where image draws to
* @param renderer what draws the image
* @param data the 2D point data being drawn
*/
@Override
protected void render(Graphics2D graphics, ScatterGraph2DRenderer renderer, Point2DDataset data) {
renderer.draw(graphics, data);
}
/**
* Returns the name of the graph being profiled.
* @return ScatterGraph2DRenderer
title
*/
@Override
public String getGraphTitle() {
return "ScatterGraph2D";
}
/**
* Gets the updates associated with the renderer in a map, linking a
* description of the update to the update object.
* @return map with description of update paired with an update
*/
@Override
public LinkedHashMap getVariations() {
LinkedHashMap map = new LinkedHashMap<>();
map.put("None", null);
map.put("Linear Interpolation", new ScatterGraph2DRendererUpdate().interpolation(InterpolationScheme.LINEAR));
map.put("Cubic Interpolation", new ScatterGraph2DRendererUpdate().interpolation(InterpolationScheme.CUBIC));
return map;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy