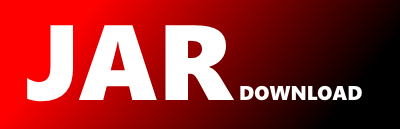
org.directwebremoting.Container Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of dwr Show documentation
Show all versions of dwr Show documentation
DWR is easy Ajax for Java. It makes it simple to call Java code directly from Javascript.
It gets rid of almost all the boiler plate code between the web browser and your Java code.
The newest version!
/*
* Copyright 2005 Joe Walker
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.directwebremoting;
import java.util.Collection;
/**
* A very basic IoC container.
* See ContainerUtil for information on how to setup a {@link Container}
* @author Joe Walker [joe at getahead dot ltd dot uk]
*/
public interface Container
{
/**
* Get the contained instance of a bean/setting of a given name.
* @param id The type to get an instance of
* @return The object of the given type, or null if the object does not exist
*/
Object getBean(String id);
/**
* Get the contained instance of a bean of a given type
* @param type The type to get an instance of
* @return The object of the given type, or null if the object does not exist
*/
T getBean(Class type);
/**
* Get a list of all the available beans.
* Implementation of this method is optional so it is valid for this method
* to return an empty collection, but to return Objects when queried
* directly using {@link #getBean(String)}. This method should only be used
* for debugging purposes.
* @return A collection containing all the available bean names.
*/
Collection getBeanNames();
/**
* Closes down all parts of DWR in a timely way, stops threads,
* and performs tidy-up.
*/
void destroy();
/**
* Sometimes we need to create a bean as a one-off object and have it
* injected with settings by the container.
* This does not make the object part of the container.
* @param type The type to get an instance of
*/
T newInstance(Class type) throws InstantiationException, IllegalAccessException;
/**
* Sometimes we need to take a bean not created by the container, and inject
* it with the data that it would contain if it was created by the
* container.
* This does not make the object part of the container.
* @param object The object to inject.
*/
void initializeBean(Object object);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy