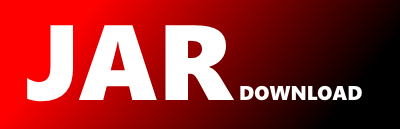
org.directwebremoting.proxy.scriptaculous.Effect Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of dwr Show documentation
Show all versions of dwr Show documentation
DWR is easy Ajax for Java. It makes it simple to call Java code directly from Javascript.
It gets rid of almost all the boiler plate code between the web browser and your Java code.
The newest version!
/*
* Copyright 2005 Joe Walker
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.directwebremoting.proxy.scriptaculous;
import java.util.Collection;
import org.directwebremoting.ScriptBuffer;
import org.directwebremoting.ScriptSession;
import org.directwebremoting.proxy.ScriptProxy;
/**
* A server side proxy the the Script.aculo.us Effect class
* @author Joe Walker [joe at getahead dot ltd dot uk]
* @author Mitch Gorman
* @deprecated Use org.directwebremoting.ui.scriptaculous.Effect
* @see org.directwebremoting.ui.scriptaculous.Effect
*/
@Deprecated
public class Effect extends ScriptProxy
{
/**
* Http thread constructor, that affects no browsers.
* Calls to {@link Effect#addScriptSession(ScriptSession)} or to
* {@link Effect#addScriptSessions(Collection)} will be needed
*/
public Effect()
{
}
/**
* Http thread constructor that alters a single browser
* @param scriptSession The browser to alter
*/
public Effect(ScriptSession scriptSession)
{
super(scriptSession);
}
/**
* Http thread constructor that alters a number of browsers
* @param scriptSessions A collection of ScriptSessions that we should act on.
*/
public Effect(Collection scriptSessions)
{
super(scriptSessions);
}
/**
* Call the script.aculo.us Effect.fade()
function.
* @param elementId The element to effect
*/
public void fade(String elementId)
{
callEffect(elementId, "Fade");
}
/**
* Call the script.aculo.us Effect.appear()
function.
* @param elementId The element to effect
*/
public void appear(String elementId)
{
callEffect(elementId, "Appear");
}
/**
* Call the script.aculo.us Effect.puff()
function.
* @param elementId The element to effect
*/
public void puff(String elementId)
{
callEffect(elementId, "Puff");
}
/**
* Call the script.aculo.us Effect.blindUp()
function.
* @param elementId The element to effect
*/
public void blindUp(String elementId)
{
callEffect(elementId, "BlindUp");
}
/**
* Call the script.aculo.us Effect.blindDown()
function.
* @param elementId The element to effect
*/
public void blindDown(String elementId)
{
callEffect(elementId, "BlindDown");
}
/**
* Call the script.aculo.us Effect.switchOff()
function.
* @param elementId The element to effect
*/
public void switchOff(String elementId)
{
callEffect(elementId, "SwitchOff");
}
/**
* Call the script.aculo.us Effect.dropOut()
function.
* @param elementId The element to effect
*/
public void dropOut(String elementId)
{
callEffect(elementId, "DropOut");
}
/**
* Call the script.aculo.us Effect.shake()
function.
* @param elementId The element to effect
*/
public void shake(String elementId)
{
callEffect(elementId, "Shake");
}
/**
* Call the script.aculo.us Effect.slideDown()
function.
* @param elementId The element to effect
*/
public void slideDown(String elementId)
{
callEffect(elementId, "SlideDown");
}
/**
* Call the script.aculo.us Effect.slideUp()
function.
* @param elementId The element to effect
*/
public void slideUp(String elementId)
{
callEffect(elementId, "SlideUp");
}
/**
* Call the script.aculo.us Effect.squish()
function.
* @param elementId The element to effect
*/
public void squish(String elementId)
{
callEffect(elementId, "Squish");
}
/**
* Call the script.aculo.us Effect.grow()
function.
* @param elementId The element to effect
*/
public void grow(String elementId)
{
callEffect(elementId, "Grow");
}
/**
* Call the script.aculo.us Effect.shrink()
function.
* @param elementId The element to effect
*/
public void shrink(String elementId)
{
callEffect(elementId, "Shrink");
}
/**
* Call the script.aculo.us Effect.pulsate()
function.
* @param elementId The element to effect
*/
public void pulsate(String elementId)
{
callEffect(elementId, "Pulsate");
}
/**
* Call the script.aculo.us Effect.fold()
function.
* @param elementId The element to effect
*/
public void fold(String elementId)
{
callEffect(elementId, "Fold");
}
/**
* Call the script.aculo.us Effect.Highlight()
function.
* @param elementId The element to effect
*/
public void highlight(String elementId)
{
callEffect(elementId, "Highlight");
}
/**
* Call the script.aculo.us Effect.Highlight()
function.
* @param elementId The element to affect
* @param options A string containing options to pass to the fade effect, as specified at http://script.aculo.us/
*/
public void highlight(String elementId, String options)
{
callEffect(elementId, "Highlight", options);
}
/**
* Call the script.aculo.us Effect.Fade()
function.
* @param elementId The element to affect
* @param options A string containing options to pass to the fade effect, as specified at http://script.aculo.us/
*/
public void fade(String elementId, String options)
{
callEffect(elementId, "Fade", options);
}
/**
* Call the script.aculo.us Effect.Appear()
function.
* @param elementId The element to affect
* @param options A string containing options to pass to the appear effect, as specified at http://script.aculo.us/
*/
public void appear(String elementId, String options)
{
callEffect(elementId, "Appear", options);
}
/**
* Call the script.aculo.us Effect.Puff()
function.
* @param elementId The element to affect
* @param options A string containing options to pass to the puff effect, as specified at http://script.aculo.us/
*/
public void puff(String elementId, String options)
{
callEffect(elementId, "Puff", options);
}
/**
* Call the script.aculo.us Effect.BlindUp()
function.
* @param elementId The element to affect
* @param options A string containing options to pass to the blindup effect, as specified at http://script.aculo.us/
*/
public void blindUp(String elementId, String options)
{
callEffect(elementId, "BlindUp", options);
}
/**
* Call the script.aculo.us Effect.BlindDown()
function.
* @param elementId The element to affect
* @param options A string containing options to pass to the blinddown effect, as specified at http://script.aculo.us/
*/
public void blindDown(String elementId, String options)
{
callEffect(elementId, "BlindDown", options);
}
/**
* Call the script.aculo.us Effect.SwitchOff()
function.
* @param elementId The element to affect
* @param options A string containing options to pass to the switchf effect, as specified at http://script.aculo.us/
*/
public void switchOff(String elementId, String options)
{
callEffect(elementId, "SwitchOff", options);
}
/**
* Call the script.aculo.us Effect.DropOut()
function.
* @param elementId The element to affect
* @param options A string containing options to pass to the dropout effect, as specified at http://script.aculo.us/
*/
public void dropOut(String elementId, String options)
{
callEffect(elementId, "DropOut", options);
}
/**
* Call the script.aculo.us Effect.Shake()
function.
* @param elementId The element to affect
* @param options A string containing options to pass to the shake effect, as specified at http://script.aculo.us/
*/
public void shake(String elementId, String options)
{
callEffect(elementId, "Shake", options);
}
/**
* Call the script.aculo.us Effect.SlideDown()
function.
* @param elementId The element to affect
* @param options A string containing options to pass to the slidedown effect, as specified at http://script.aculo.us/
*/
public void slideDown(String elementId, String options)
{
callEffect(elementId, "SlideDown", options);
}
/**
* Call the script.aculo.us Effect.SlideUp()
function.
* @param elementId The element to affect
* @param options A string containing options to pass to the slideup effect, as specified at http://script.aculo.us/
*/
public void slideUp(String elementId, String options)
{
callEffect(elementId, "SlideUp", options);
}
/**
* Call the script.aculo.us Effect.Squish()
function.
* @param elementId The element to affect
* @param options A string containing options to pass to the squish effect, as specified at http://script.aculo.us/
*/
public void squish(String elementId, String options)
{
callEffect(elementId, "Squish", options);
}
/**
* Call the script.aculo.us Effect.Grow()
function.
* @param elementId The element to affect
* @param options A string containing options to pass to the grow effect, as specified at http://script.aculo.us/
*/
public void grow(String elementId, String options)
{
callEffect(elementId, "Grow", options);
}
/**
* Call the script.aculo.us Effect.Shrink()
function.
* @param elementId The element to affect
* @param options A string containing options to pass to the shrink effect, as specified at http://script.aculo.us/
*/
public void shrink(String elementId, String options)
{
callEffect(elementId, "Shrink", options);
}
/**
* Call the script.aculo.us Effect.Pulsate()
function.
* @param elementId The element to affect
* @param options A string containing options to pass to the pulsate effect, as specified at http://script.aculo.us/
*/
public void pulsate(String elementId, String options)
{
callEffect(elementId, "Pulsate", options);
}
/**
* Call the script.aculo.us Effect.Fold()
function.
* @param elementId The element to affect
* @param options A string containing options to pass to the fold effect, as specified at http://script.aculo.us/
*/
public void fold(String elementId, String options)
{
callEffect(elementId, "Fold", options);
}
/**
* @param elementId The element to affect
* @param function The script.aculo.us effect to employ
*/
private void callEffect(String elementId, String function)
{
callEffect(elementId, function, null);
}
/**
* @param elementId The element to affect
* @param function The script.aculo.us effect to employ
* @param options A string containing options to pass to the script.aculo.us effect, as specified at http://script.aculo.us/
*/
private void callEffect(String elementId, String function, String options)
{
ScriptBuffer script = new ScriptBuffer();
script.appendScript("new Effect.").appendScript(function).appendScript("('").appendScript(elementId).appendScript("'");
if (options != null && options.length() > 0)
{
script.appendScript(", ").appendScript(options);
}
script.appendScript(");");
addScript(script);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy