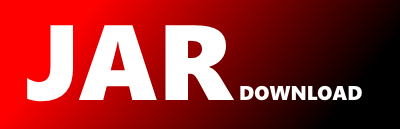
org.directwebremoting.spring.CreatorConfig Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of dwr Show documentation
Show all versions of dwr Show documentation
DWR is easy Ajax for Java. It makes it simple to call Java code directly from Javascript.
It gets rid of almost all the boiler plate code between the web browser and your Java code.
The newest version!
/*
* Copyright 2005 Joe Walker
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.directwebremoting.spring;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.directwebremoting.AjaxFilter;
import org.directwebremoting.extend.Creator;
/**
* The configuration for a creator.
* You can either specify the creator directly or specify one of the build in creator types,
* for instance "new".
*
* It allows the specification of the following optional configuration parameters:
*
* - includes - the list of method names to include
* - excludes - the list of method names to exclude
* - auth - the
Properties
object containing method names and corresponding
* required role
* - filters - the list of filter objects
*
*
* @see org.directwebremoting.extend.AccessControl#addIncludeRule(String, String)
* @see org.directwebremoting.extend.AccessControl#addExcludeRule(String, String)
* @see org.directwebremoting.extend.AccessControl#addRoleRestriction(String, String, String)
* @see org.directwebremoting.AjaxFilter
* @see org.directwebremoting.extend.AjaxFilterManager#addAjaxFilter(org.directwebremoting.AjaxFilter, String)
*
* @author Bram Smeets
* @author Joe Walker [joe at getahead dot ltd dot uk]
*/
public class CreatorConfig extends AbstractConfig
{
/**
* The creator type that will be used to create new objects for remoting
* @return Returns the creator type.
*/
public String getCreatorType()
{
return creatorType;
}
/**
* The creator that will be used to create new objects for remoting
* @param creatorType The creator type to set.
*/
public void setCreatorType(String creatorType)
{
this.creatorType = creatorType;
}
/**
* The creator that will be used to create new objects for remoting
* @return Returns the creator.
*/
public Creator getCreator()
{
return creator;
}
/**
* The creator type that will be used to create new objects for remoting
* @param creator The creator to set.
*/
public void setCreator(Creator creator)
{
this.creator = creator;
}
/**
* Sets the authentication parameters for this creator.
* @return the map containing the method name and the corrosponding required role
* @see org.directwebremoting.extend.AccessControl#addRoleRestriction(String, String, String)
*/
public Map> getAuth()
{
return auth;
}
/**
* Sets the authentication parameters for this creator.
* @param auth the map containing the method name and the corresponding required role
* @see org.directwebremoting.extend.AccessControl#addRoleRestriction(String, String, String)
*/
public void setAuth(Map> auth)
{
this.auth = auth;
}
/**
* Gets the list of all filters for this creator.
* @return the list containing all filters
* @see org.directwebremoting.AjaxFilter
* @see org.directwebremoting.extend.AjaxFilterManager#addAjaxFilter(org.directwebremoting.AjaxFilter, String)
*/
public List> getFilters()
{
return filters;
}
/**
* Sets the list of all filters for this creator.
* @param filters the list containing all filters
* @see org.directwebremoting.AjaxFilter
* @see org.directwebremoting.extend.AjaxFilterManager#addAjaxFilter(org.directwebremoting.AjaxFilter, String)
*/
public void setFilters(List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy