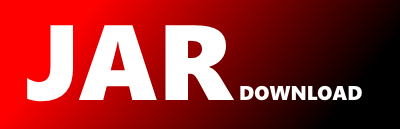
org.dishevelled.matrix.BitMatrix2D Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of dsh-matrix Show documentation
Show all versions of dsh-matrix Show documentation
long-addressable bit and typed object matrix implementations.
The newest version!
/*
dsh-matrix long-addressable bit and typed object matrix implementations.
Copyright (c) 2004-2012 held jointly by the individual authors.
This library is free software; you can redistribute it and/or modify it
under the terms of the GNU Lesser General Public License as published
by the Free Software Foundation; either version 3 of the License, or (at
your option) any later version.
This library is distributed in the hope that it will be useful, but WITHOUT
ANY WARRANTY; with out even the implied warranty of MERCHANTABILITY or
FITNESS FOR A PARTICULAR PURPOSE. See the GNU Lesser General Public
License for more details.
You should have received a copy of the GNU Lesser General Public License
along with this library; if not, write to the Free Software Foundation,
Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA.
> http://www.fsf.org/licensing/licenses/lgpl.html
> http://www.opensource.org/licenses/lgpl-license.php
*/
package org.dishevelled.matrix;
import org.dishevelled.bitset.MutableBitSet;
import org.dishevelled.functor.BinaryProcedure;
/**
* Fixed size bit matrix in two dimensions, indexed by long
s.
*
* @author Michael Heuer
* @version $Revision: 1065 $ $Date: 2012-01-04 22:01:07 -0600 (Wed, 04 Jan 2012) $
*/
public final class BitMatrix2D
{
/** Bit set. */
private final MutableBitSet bitset;
/** Number of rows. */
private final long rows;
/** Number of columns. */
private final long columns;
/** Size. */
private final long size;
/**
* Create a new 2D bit matrix with the specified number of rows and columns.
*
* @param rows number of rows, must be >= 0
* @param columns number of columns, must be >= 0
* @throws IllegalArgumentException if either rows
or
* columns
is negative
*/
public BitMatrix2D(final long rows, final long columns)
{
if (rows < 0)
{
throw new IllegalArgumentException("rows must be >= 0");
}
if (columns < 0)
{
throw new IllegalArgumentException("columns must be >= 0");
}
this.rows = rows;
this.columns = columns;
this.size = (rows * columns);
this.bitset = new MutableBitSet(this.size);
}
/**
* Return the size of this 2D bit matrix.
*
* @return the size of this 2D bit matrix
*/
public long size()
{
return size;
}
/**
* Return the number of rows in this 2D bit matrix.
*
* @return the number of rows in this 2D bit matrix
*/
public long rows()
{
return rows;
}
/**
* Return the number of columns in this 2D bit matrix.
*
* @return the number of columns in this 2D bit matrix
*/
public long columns()
{
return columns;
}
/**
* Return the cardinality of this 2D bit matrix, the
* number of bits set to true.
*
* @return the cardinality of this 2D bit matrix
*/
public long cardinality()
{
return bitset.cardinality();
}
/**
* Return true if the cardinality of this 2D bit matrix is zero.
*
* @return true if the cardinality of this 2D bit matrix is zero
*/
public boolean isEmpty()
{
return (0 == cardinality());
}
/**
* Clear all the values in this 2D bit matrix.
*/
public void clear()
{
for (long i = bitset.nextSetBit(0); i >= 0; i = bitset.nextSetBit(i + 1))
{
bitset.clear(i);
}
}
/**
* Return the bit value at the specified row and column.
*
* @param row row index, must be >= 0
and < rows()
* @param column column index, must be >= 0
and < columns()
* @return the bit value at the specified row and column
* @throws IndexOutOfBoundsException if row
or column
* is negative or if row
or column
is greater than
* or equal to rows()
or columns()
, respectively
*/
public boolean get(final long row, final long column)
{
if (row < 0)
{
throw new IndexOutOfBoundsException(row + " < 0");
}
if (row >= rows)
{
throw new IndexOutOfBoundsException(row + " >= " + rows);
}
if (column < 0)
{
throw new IndexOutOfBoundsException(column + " < 0");
}
if (column >= columns)
{
throw new IndexOutOfBoundsException(column + " >= " + columns);
}
return getQuick(row, column);
}
/**
* Return the bit value at the specified row and column without checking bounds.
*
* @param row row index
* @param column column index
* @return the bit value at the specified row and column
*/
public boolean getQuick(final long row, final long column)
{
return bitset.getQuick(index(row, column));
}
/**
* Set the bit value at the specified row and column to value
.
*
* @param row row index, must be >= 0
and < rows()
* @param column column index, must be >= 0
and < columns()
* @param value value
* @throws IndexOutOfBoundsException if row
or column
* is negative or if row
or column
is greater than
* or equal to rows()
or columns()
, respectively
*/
public void set(final long row, final long column, final boolean value)
{
if (row < 0)
{
throw new IndexOutOfBoundsException(row + " < 0");
}
if (row >= rows)
{
throw new IndexOutOfBoundsException(row + " >= " + rows);
}
if (column < 0)
{
throw new IndexOutOfBoundsException(column + " < 0");
}
if (column >= columns)
{
throw new IndexOutOfBoundsException(column + " >= " + columns);
}
setQuick(row, column, value);
}
/**
* Set the bit value at the specified row and column to value
without checking bounds.
*
* @param row row index
* @param column column index
* @param value value
*/
public void setQuick(final long row, final long column, final boolean value)
{
long index = index(row, column);
if (value)
{
bitset.setQuick(index);
}
else
{
bitset.clearQuick(index);
}
}
/**
* Set all of the bit values from (row0, column0
), inclusive,
* to (row1, column1
), exclusive, to the specified value.
*
* @param row0 first row, must be >= 0
and < rows()
* @param column0 first column, must be >= 0
and < columns()
* @param row1 second row, must be >= 0
, <= rows()
* and >= row0
* @param column1 second column, must be >= 0
, <= columns()
* and >= column0
* @param value value
* @throws IllegalArgumentException if either row1
or column1
* are less than row0
or column0
, respectively
* @throws IndexOutOfBoundsException if any of row0
, column0
,
* row1
, or column1
are negative or if either row0
* or column0
are greater than or equal to rows()
or
* columns()
, respectively, or if either row1
or
* column1
are strictly greater than rows()
or columns()
,
* respectively
*/
public void set(final long row0, final long column0,
final long row1, final long column1, final boolean value)
{
if (row0 < 0)
{
throw new IndexOutOfBoundsException(row0 + " < 0");
}
if (row0 >= rows)
{
throw new IndexOutOfBoundsException(row0 + " >= " + rows);
}
if (column0 < 0)
{
throw new IndexOutOfBoundsException(column0 + " < 0");
}
if (column0 >= columns)
{
throw new IndexOutOfBoundsException(column0 + " >= " + columns);
}
if (row1 < 0)
{
throw new IndexOutOfBoundsException(row1 + " < 0");
}
if (row1 > rows)
{
throw new IndexOutOfBoundsException(row1 + " > " + rows);
}
if (column1 < 0)
{
throw new IndexOutOfBoundsException(column1 + " < 0");
}
if (column1 > columns)
{
throw new IndexOutOfBoundsException(column1 + " > " + columns);
}
for (long row = row0; row < row1; row++)
{
for (long column = column0; column < column1; column++)
{
setQuick(row, column, value);
}
}
}
/**
* Set the bit value at the specified row and column to the complement
* of its current bit value.
*
* @param row row index, must be >= 0
and < rows()
* @param column column index, must be >= 0
and < columns()
* @throws IndexOutOfBoundsException if row
or column
* is negative or if row
or column
is greater than
* or equal to rows()
or columns()
, respectively
*/
public void flip(final long row, final long column)
{
if (row < 0)
{
throw new IndexOutOfBoundsException(row + " < 0");
}
if (row >= rows)
{
throw new IndexOutOfBoundsException(row + " >= " + rows);
}
if (column < 0)
{
throw new IndexOutOfBoundsException(column + " < 0");
}
if (column >= columns)
{
throw new IndexOutOfBoundsException(column + " >= " + columns);
}
flipQuick(row, column);
}
/**
* Set the bit value at the specified row and column to the complement
* of its current bit value without checking bounds.
*
* @param row row index
* @param column column index
*/
public void flipQuick(final long row, final long column)
{
bitset.flipQuick(index(row, column));
}
/**
* Set all of the bit values from (row0, column0
), inclusive,
* to (row1, column1
), exclusive, to the complement of their
* current bit values.
*
* @param row0 first row, must be >= 0
and < rows()
* @param column0 first column, must be >= 0
and < columns()
* @param row1 second row, must be >= 0
, <= rows()
* and >= row0
* @param column1 second column, must be >= 0
, <= columns()
* and >= column0
* @throws IllegalArgumentException if either row1
or column1
* are less than row0
or column0
, respectively
* @throws IndexOutOfBoundsException if any of row0
, column0
,
* row1
, or column1
are negative or if either row0
* or column0
are greater than or equal to rows()
or
* columns()
, respectively, or if either row1
or
* column1
are strictly greater than rows()
or columns()
,
* respectively
*/
public void flip(final long row0, final long column0,
final long row1, final long column1)
{
if (row0 < 0)
{
throw new IndexOutOfBoundsException(row0 + " < 0");
}
if (row0 >= rows)
{
throw new IndexOutOfBoundsException(row0 + " >= " + rows);
}
if (column0 < 0)
{
throw new IndexOutOfBoundsException(column0 + " < 0");
}
if (column0 >= columns)
{
throw new IndexOutOfBoundsException(column0 + " >= " + columns);
}
if (row1 < 0)
{
throw new IndexOutOfBoundsException(row1 + " < 0");
}
if (row1 > rows)
{
throw new IndexOutOfBoundsException(row1 + " > " + rows);
}
if (column1 < 0)
{
throw new IndexOutOfBoundsException(column1 + " < 0");
}
if (column1 > columns)
{
throw new IndexOutOfBoundsException(column1 + " > " + columns);
}
for (long row = row0; row < row1; row++)
{
for (long column = column0; column < column1; column++)
{
flipQuick(row, column);
}
}
}
/**
* Assign all values in this 2D bit matrix to value
.
*
* @param value value
* @return this 2D bit matrix, for convenience
*/
public BitMatrix2D assign(final boolean value)
{
if (size > 0)
{
set(0, 0, rows, columns, value);
}
return this;
}
/**
* Return true if the specified 2D bit matrix has any bits set
* to true that are also set to true in this 2D bit matrix.
*
* @param other other 2D bit matrix, must not be null and must
* have the same dimensions as this 2D bit matrix
* @return true if the specified 2D bit matrix has any bits set
* to true that are also set to true in this 2D bit matrix
*/
public boolean intersects(final BitMatrix2D other)
{
if (other == null)
{
throw new IllegalArgumentException("other must not be null");
}
if ((size != other.size()) || (rows != other.rows()) || (columns != other.columns()))
{
throw new IllegalArgumentException("this and other must have the same dimensions");
}
return bitset.intersects(other.bitset);
}
/**
* Perform a logical AND of this 2D bit matrix
* and the specified 2D bit matrix.
*
* @param other other 2D bit matrix, must not be null and must
* have the same dimensions as this 2D bit matrix
* @return this 2D bit matrix, for convenience
*/
public BitMatrix2D and(final BitMatrix2D other)
{
if (other == null)
{
throw new IllegalArgumentException("other must not be null");
}
if ((size != other.size()) || (rows != other.rows()) || (columns != other.columns()))
{
throw new IllegalArgumentException("this and other must have the same dimensions");
}
bitset.and(other.bitset);
return this;
}
/**
* Clear all the bits in this 2D bit matrix whose corresponding
* bit is set in the specified 2D bit matrix.
*
* @param other other 2D bit matrix, must not be null and must
* have the same dimensions as this 2D bit matrix
* @return this 2D bit matrix, for convenience
*/
public BitMatrix2D andNot(final BitMatrix2D other)
{
if (other == null)
{
throw new IllegalArgumentException("other must not be null");
}
if ((size != other.size()) || (rows != other.rows()) || (columns != other.columns()))
{
throw new IllegalArgumentException("this and other must have the same dimensions");
}
bitset.andNot(other.bitset);
return this;
}
/**
* Perform a logical OR of this 2D bit matrix
* and the specified 2D bit matrix.
*
* @param other other 2D bit matrix, must not be null and must
* have the same dimensions as this 2D bit matrix
* @return this 2D bit matrix, for convenience
*/
public BitMatrix2D or(final BitMatrix2D other)
{
if (other == null)
{
throw new IllegalArgumentException("other must not be null");
}
if ((size != other.size()) || (rows != other.rows()) || (columns != other.columns()))
{
throw new IllegalArgumentException("this and other must have the same dimensions");
}
bitset.or(other.bitset);
return this;
}
/**
* Perform a logical XOR of this 2D bit matrix
* and the specified 2D bit matrix.
*
* @param other other 2D bit matrix, must not be null and must
* have the same dimensions as this 2D bit matrix
* @return this 2D bit matrix, for convenience
*/
public BitMatrix2D xor(final BitMatrix2D other)
{
if (other == null)
{
throw new IllegalArgumentException("other must not be null");
}
if ((size != other.size()) || (rows != other.rows()) || (columns != other.columns()))
{
throw new IllegalArgumentException("this and other must have the same dimensions");
}
bitset.xor(other.bitset);
return this;
}
/**
* Apply the specified procedure to each row and column
* in this 2D bit matrix with a bit equal to the specified value.
*
* @param value value
* @param procedure procedure, must not be null
*/
public void forEach(final boolean value, final BinaryProcedure procedure)
{
if (procedure == null)
{
throw new IllegalArgumentException("procedure must not be null");
}
for (long row = 0; row < rows; row++)
{
for (long column = 0; column < columns; column++)
{
if (getQuick(row, column) == value)
{
procedure.run(Long.valueOf(row), Long.valueOf(column));
}
}
}
}
/** {@inheritDoc} */
public boolean equals(final Object o)
{
if (o == null)
{
return false;
}
if (!(o instanceof BitMatrix2D))
{
return false;
}
BitMatrix2D bm = (BitMatrix2D) o;
if ((size != bm.size()) || (rows != bm.rows()) || (columns != bm.columns()))
{
return false;
}
return bitset.equals(bm.bitset);
}
/** {@inheritDoc} */
public int hashCode()
{
int hashCode = 37;
hashCode = 17 * hashCode + (int) (size ^ (size >>> 32));
hashCode = 17 * hashCode + (int) (rows ^ (rows >>> 32));
hashCode = 17 * hashCode + (int) (columns ^ (columns >>> 32));
hashCode = 17 * hashCode + bitset.hashCode();
return hashCode;
}
/**
* Return the index for the specified row and column.
*
* @param row row index
* @param column column index
* @return the index for the specified row and column
*/
private long index(final long row, final long column)
{
return (columns * row) + column;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy