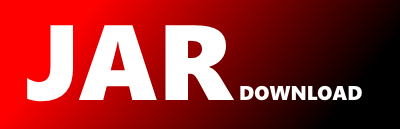
org.dishevelled.matrix.Matrix2D Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of dsh-matrix Show documentation
Show all versions of dsh-matrix Show documentation
long-addressable bit and typed object matrix implementations.
The newest version!
/*
dsh-matrix long-addressable bit and typed object matrix implementations.
Copyright (c) 2004-2012 held jointly by the individual authors.
This library is free software; you can redistribute it and/or modify it
under the terms of the GNU Lesser General Public License as published
by the Free Software Foundation; either version 3 of the License, or (at
your option) any later version.
This library is distributed in the hope that it will be useful, but WITHOUT
ANY WARRANTY; with out even the implied warranty of MERCHANTABILITY or
FITNESS FOR A PARTICULAR PURPOSE. See the GNU Lesser General Public
License for more details.
You should have received a copy of the GNU Lesser General Public License
along with this library; if not, write to the Free Software Foundation,
Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA.
> http://www.fsf.org/licensing/licenses/lgpl.html
> http://www.opensource.org/licenses/lgpl-license.php
*/
package org.dishevelled.matrix;
import java.util.Iterator;
import org.dishevelled.functor.UnaryFunction;
import org.dishevelled.functor.UnaryPredicate;
import org.dishevelled.functor.UnaryProcedure;
import org.dishevelled.functor.BinaryFunction;
import org.dishevelled.functor.TernaryPredicate;
import org.dishevelled.functor.TernaryProcedure;
/**
* Typed fixed size matrix of objects in two dimensions, indexed
* by long
s.
*
* @param type of this 2D matrix
* @author Michael Heuer
* @version $Revision: 1059 $ $Date: 2012-01-03 14:03:02 -0600 (Tue, 03 Jan 2012) $
*/
public interface Matrix2D
extends Iterable
{
/**
* Return the size of this 2D matrix.
*
* @return the size of this 2D matrix
*/
long size();
/**
* Return the number of rows in this 2D matrix.
*
* @return the number of rows in this 2D matrix
*/
long rows();
/**
* Return the number of columns in this 2D matrix.
*
* @return the number of columns in this 2D matrix
*/
long columns();
/**
* Return the cardinality of this 2D matrix, the number
* of non-null values.
*
* @return the cardinality of this 2D matrix
*/
long cardinality();
/**
* Return true if the cardinality of this 2D matrix is zero.
*
* @return true if the cardinality of this 2D matrix is zero
*/
boolean isEmpty();
/**
* Clear all the values in this 2D matrix (optional operation).
*
* @throws UnsupportedOperationException if the clear
operation
* is not supported by this 2D matrix
*/
void clear();
/**
* Return the value at the specified row and column.
*
* @param row row index, must be >= 0
and < rows()
* @param column column index, must be >= 0
and < columns()
* @return the value at the specified row and column
* @throws IndexOutOfBoundsException if row
or column
* is negative or if row
or column
is greater than
* or equal to rows()
or columns()
, respectively
*/
E get(long row, long column);
/**
* Return the value at the specified row and column without
* checking bounds.
*
* @param row row index, should be >= 0
and < rows()
* (unchecked)
* @param column column index, should be >= 0
and < columns()
* (unchecked)
* @return the value at the specified row and column without
* checking bounds
*/
E getQuick(long row, long column);
/**
* Set the value at the specified row and column to e
(optional
* operation).
*
* @param row row index, must be >= 0
and < rows()
* @param column column index, must be >= 0
and < columns()
* @param e value
* @throws IndexOutOfBoundsException if row
or column
* is negative or if row
or column
is greater than
* or equal to rows()
or columns()
, respectively
* @throws UnsupportedOperationException if the set
operation
* is not supported by this 2D matrix
*/
void set(long row, long column, E e);
/**
* Set the value at the specified row and column to
* e
without checking bounds (optional operation).
*
* @param row row index, should be >= 0
and < rows()
* (unchecked)
* @param column column index, should be >= 0
and < columns()
* (unchecked)
* @param e value
* @throws UnsupportedOperationException if the setQuick
operation
* is not supported by this 2D matrix
*/
void setQuick(long row, long column, E e);
/**
* Return an iterator over the values in this 2D matrix, including
* null
s.
*
* @return an iterator over the values in this 2D matrix, including
* null
s
*/
Iterator iterator();
/**
* Assign all values in this 2D matrix to e
(optional operation).
*
* @param e value
* @return this 2D matrix, for convenience
* @throws UnsupportedOperationException if this assign
operation
* is not supported by this 2D matrix
*/
Matrix2D assign(E e);
/**
* Assign the result of the specified function to each value
* in this 2D matrix (optional operation).
*
* @param function function, must not be null
* @return this 2D matrix, for convenience
* @throws UnsupportedOperationException if this assign
operation
* is not supported by this 2D matrix
*/
Matrix2D assign(UnaryFunction function);
/**
* Assign all values in this 2D matrix to the values in the
* specified matrix (optional operation).
*
* @param other other 2D matrix, must not be null and must
* have the same dimensions as this 2D matrix
* @return this 2D matrix, for convenience
* @throws UnsupportedOperationException if this assign
operation
* is not supported by this 2D matrix
*/
Matrix2D assign(Matrix2D extends E> other);
/**
* Assign the result of the specified function of a value from
* this 2D matrix and the specified matrix to each value in this
* 2D matrix (optional operation).
*
* @param other other 2D matrix, must not be null and must
* have the same dimensions as this 2D matrix
* @param function function, must not be null
* @return this 2D matrix, for convenience
* @throws UnsupportedOperationException if this assign
operation
* is not supported by this 2D matrix
*/
Matrix2D assign(Matrix2D extends E> other,
BinaryFunction function);
/**
* Apply a function to each value in this 2D matrix and aggregate
* the result.
*
* @param aggr aggregate function, must not be null
* @param function function, must not be null
* @return the aggregate result
*/
E aggregate(BinaryFunction aggr, UnaryFunction function);
/**
* Apply a function to each value in this 2D matrix and the specified
* matrix and aggregate the result.
*
* @param other other 2D matrix, must not be null and must
* have the same dimensions as this 2D matrix
* @param aggr aggregate function, must not be null
* @param function function, must not be null
* @return the aggregate result
*/
E aggregate(Matrix2D extends E> other,
BinaryFunction aggr,
BinaryFunction function);
/**
* Return a new 1D matrix slice view of the specified row. The view
* is backed by this matrix, so changes made to the returned view
* are reflected in this matrix, and vice-versa.
*
* @param row row index, must be >= 0
and < rows()
* @return a new 1D matrix slice view of the specified row
* @throws IndexOutOfBoundsException if row
is negative or if
* row
is greater than or equal to rows()
*/
Matrix1D viewRow(long row);
/**
* Return a new 1D matrix slice view of the specified column. The view
* is backed by this matrix, so changes made to the returned view
* are reflected in this matrix, and vice-versa.
*
* @param column column index, must be >= 0
and < columns()
* @return a new 1D matrix slice view of the specified column
* @throws IndexOutOfBoundsException if column
is negative or if
* column
is greater than or equal to columns()
*/
Matrix1D viewColumn(long column);
/**
* Return a new 2D matrix dice (transposition) view. The view has
* both dimensions exchanged; what used to be columns become rows, what used
* to be rows become columns. In other words:
* view.get(row, column) == this.get(column, row)
.
* The view is backed by this matrix, so changes made to the returned
* view are reflected in this matrix, and vice-versa.
*
* @return a new 2D matrix dice (transposition) view
*/
Matrix2D viewDice();
/**
* Return a new 2D matrix flip view along the row axis.
* What used to be row 0
is now row rows() - 1
,
* ..., what used to be row rows() - 1
is now row 0
.
* The view is backed by this matrix, so changes made to the returned
* view are reflected in this matrix, and vice-versa.
*
* @return a new 2D matrix flip view along the row axis
*/
Matrix2D viewRowFlip();
/**
* Return a new 2D matrix flip view along the column axis.
* What used to be column 0
is now column columns() - 1
,
* ..., what used to be column columns() - 1
is now column
* 0
. The view is backed by this matrix, so changes made to the
* returned view are reflected in this matrix, and vice-versa.
*
* @return a new 2D matrix flip view along the column axis
*/
Matrix2D viewColumnFlip();
/**
* Return a new 2D matrix sub-range view that contains only those values
* from (row, column)
to
* (row + height - 1, column + width - 1)
.
* The view is backed by this matrix, so changes made to the returned
* view are reflected in this matrix, and vice-versa.
*
* @param row row index, must be >= 0
and < rows()
* @param column column index, must be >= 0
and < columns()
* @param height height
* @param width width
* @return a new 2D matrix that contains only those values
* from (row, column)
to
* (row + height - 1, column + width - 1)
.
*/
Matrix2D viewPart(long row, long column, long height, long width);
/**
* Return a new 2D matrix selection view that contains only those values at the
* specified indices. The view is backed by this matrix, so changes made
* to the returned view are reflected in this matrix, and vice-versa.
*
* @param rowIndices row indices
* @param columnIndices column indices
* @return a new 2D matrix selection view that contains only those values at the
* specified indices
*/
Matrix2D viewSelection(long[] rowIndices, long[] columnIndices);
/**
* Return a new 2D matrix selection view that contains only those rows
* selected by the specified predicate. The view is backed by this
* matrix, so changes made to the returned view are reflected in
* this matrix, and vice-versa.
*
* @param predicate predicate, must not be null
* @return a new 2D matrix view that contains only those rows
* selected by the specified predicate
*/
Matrix2D viewSelection(UnaryPredicate> predicate);
/**
* Return a new 2D matrix selection view that contains only those values
* at the indices present in the specified bit mask. The view is backed by this matrix, so
* changes made to the returned view are reflected in this matrix, and vice-versa.
*
* @param mask 2D bit mask, must not be null
* @return a new 2D matrix selection view that contains only those values
* at the indices present in the specified mask
*/
Matrix2D viewSelection(BitMatrix2D mask);
/**
* Return a new 2D matrix stride view which is a sub matrix consisting
* of every rowStride
-th row and every columnStride
-th
* column. The view is backed by this matrix, so changes made to the returned view
* are reflected in this matrix, and vice-versa.
*
* @param rowStride row stride, must be > 0
* @param columnStride column stride, must be > 0
* @return a new 2D matrix stride view which is a sub matrix consisting
* of every rowStride
-th row and every columnStride
-th
* column
* @throws IndexOutOfBoundsException if either rowStride
* or columnStride
are negative or zero
*/
Matrix2D viewStrides(long rowStride, long columnStride);
/**
* Apply the specified procedure to each value in this 2D matrix.
*
* For example:
*
* Matrix2D<String> m;
* m.forEach(new UnaryProcedure<String>()
* {
* public void run(final String value)
* {
* System.out.println(value);
* }
* });
*
*
* @param procedure procedure, must not be null
*/
void forEach(UnaryProcedure super E> procedure);
/**
* Apply the specified procedure to each value in this 2D matrix
* accepted by the specified predicate.
*
* For example:
*
* Matrix2D<String> m;
* m.forEach(new UnaryPredicate<String>()
* {
* public boolean test(final String value)
* {
* return (value != null);
* }
* }, new UnaryProcedure<String>()
* {
* public void run(final String value)
* {
* System.out.println(value);
* }
* });
*
*
* @param predicate predicate, must not be null
* @param procedure procedure, must not be null
*/
void forEach(UnaryPredicate super E> predicate,
UnaryProcedure super E> procedure);
/**
* Apply the specified procedure to each non-null value in this 2D matrix.
*
* For example:
*
* Matrix2D<String> m;
* m.forEachNonNull(new UnaryProcedure<String>()
* {
* public void run(final String value)
* {
* System.out.println(value);
* }
* });
*
*
* @param procedure procedure, must not be null
*/
void forEachNonNull(UnaryProcedure super E> procedure);
/**
* Apply the specified procedures to each row and column and
* to each value in this 2D matrix.
*
* For example:
*
* Matrix2D<String> m;
* m.forEach(new TernaryProcedure<Long, Long, String>()
* {
* public void run(final Long row, final Long column, final String value)
* {
* System.out.print("m[" + row + ", " + column + "]=" + value);
* }
* });
*
*
* @param procedure procedure, must not be null
*/
void forEach(TernaryProcedure procedure);
/**
* Apply the specified procedures to each row and column
* and to each value in this 2D matrix accepted by the specified predicate.
*
* For example:
*
* Matrix2D<String> m;
* m.forEach(new TernaryPredicate<Long, Long, String>()
* {
* public boolean test(final Long row, final Long column, final String value)
* {
* return (value != null);
* }
* }, new TernaryProcedure<Long, Long, String>()
* {
* public void run(final Long row, final Long column, final String value)
* {
* System.out.print("m[" + row + ", " + column + "]=" + value);
* }
* });
*
*
* @param predicate predicate, must not be null
* @param procedure procedure, must not be null
*/
void forEach(TernaryPredicate predicate,
TernaryProcedure procedure);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy