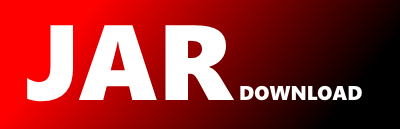
org.dishevelled.matrix.impl.SparseMatrixUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of dsh-matrix Show documentation
Show all versions of dsh-matrix Show documentation
long-addressable bit and typed object matrix implementations.
The newest version!
/*
dsh-matrix long-addressable bit and typed object matrix implementations.
Copyright (c) 2004-2012 held jointly by the individual authors.
This library is free software; you can redistribute it and/or modify it
under the terms of the GNU Lesser General Public License as published
by the Free Software Foundation; either version 3 of the License, or (at
your option) any later version.
This library is distributed in the hope that it will be useful, but WITHOUT
ANY WARRANTY; with out even the implied warranty of MERCHANTABILITY or
FITNESS FOR A PARTICULAR PURPOSE. See the GNU Lesser General Public
License for more details.
You should have received a copy of the GNU Lesser General Public License
along with this library; if not, write to the Free Software Foundation,
Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA.
> http://www.fsf.org/licensing/licenses/lgpl.html
> http://www.opensource.org/licenses/lgpl-license.php
*/
package org.dishevelled.matrix.impl;
import org.dishevelled.matrix.Matrix1D;
import org.dishevelled.matrix.Matrix2D;
import org.dishevelled.matrix.Matrix3D;
/**
* Static utility methods on sparse matrices.
*
* @author Michael Heuer
* @version $Revision$ $Date$
*/
public final class SparseMatrixUtils
{
/**
* Private no-arg constructor.
*/
private SparseMatrixUtils()
{
// empty
}
/**
* Create and return a new sparse 1D matrix with the specified size.
*
* @param 1D matrix type
* @param size size, must be >= 0
* @throws IllegalArgumentException if size
is negative
* @return a new sparse 1D matrix with the specified size
*/
public static Matrix1D createSparseMatrix1D(final long size)
{
return new SparseMatrix1D(size);
}
/**
* Create and return a new sparse 1D matrix with the specified size,
* initial capacity, and load factor.
*
* @param 1D matrix type
* @param size size, must be >= 0
* @param initialCapacity initial capacity, must be >= 0
* @param loadFactor load factor, must be > 0
* @return a new sparse 1D matrix with the specified size,
* initial capacity, and load factor
*/
public static Matrix1D createSparseMatrix1D(final long size,
final int initialCapacity,
final float loadFactor)
{
return new SparseMatrix1D(size, initialCapacity, loadFactor);
}
/**
* Create and return a new sparse 2D matrix with the specified number
* of rows and columns.
*
* @param 2D matrix type
* @param rows rows, must be >= 0
* @param columns columns, must be >= 0
* @throws IllegalArgumentException if either rows
* or columns
is negative
* @return a new sparse 2D matrix with the specified number
* of rows and columns
*/
public static Matrix2D createSparseMatrix2D(final long rows, final long columns)
{
return new SparseMatrix2D(rows, columns);
}
/**
* Create and return a new sparse 2D matrix with the specified number
* of rows and columns, initial capacity, and load factor.
*
* @param 2D matrix type
* @param rows rows, must be >= 0
* @param columns columns, must be >= 0
* @param initialCapacity initial capacity, must be >= 0
* @param loadFactor load factor, must be > 0
* @return a new sparse 2D matrix with the specified number
* of rows and columns, initial capacity, and load factor
*/
public static Matrix2D createSparseMatrix2D(final long rows,
final long columns,
final int initialCapacity,
final float loadFactor)
{
return new SparseMatrix2D(rows, columns, initialCapacity, loadFactor);
}
/**
* Create and return a new sparse 3D matrix with the specified number
* of slices, rows, and columns.
*
* @param 3D matrix type
* @param slices slices, must be >= 0
* @param rows rows, must be >= 0
* @param columns columns, must be >= 0
* @throws IllegalArgumentException if any of slices
,
* rows
, or columns
is negative
* @return a new sparse 3D matrix with the specified number
* of slices, rows, and columns
*/
public static Matrix3D createSparseMatrix3D(final long slices, final long rows, final long columns)
{
return new SparseMatrix3D(slices, rows, columns);
}
/**
* Create and return a new sparse 3D matrix with the specified number
* of slices, rows, and columns, initial capacity, and load factor.
*
* @param 3D matrix type
* @param slices slices, must be >= 0
* @param rows rows, must be >= 0
* @param columns columns, must be >= 0
* @param initialCapacity initial capacity, must be >= 0
* @param loadFactor load factor, must be > 0
* @return a new sparse 3D matrix with the specified number
* of slices, rows, and columns, initial capacity, and load factor
*/
public static Matrix3D createSparseMatrix3D(final long slices,
final long rows,
final long columns,
final int initialCapacity,
final float loadFactor)
{
return new SparseMatrix3D(slices, rows, columns, initialCapacity, loadFactor);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy