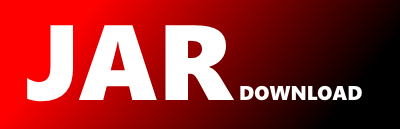
org.dizitart.no2.common.streams.DistinctStream Maven / Gradle / Ivy
/*
* Copyright (c) 2017-2021 Nitrite author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
*/
package org.dizitart.no2.common.streams;
import org.dizitart.no2.collection.Document;
import org.dizitart.no2.collection.NitriteId;
import org.dizitart.no2.common.RecordStream;
import org.dizitart.no2.common.tuples.Pair;
import java.util.*;
/**
* @author Anindya Chatterjee
* @since 4.0
*/
public class DistinctStream implements RecordStream> {
private final RecordStream> rawStream;
public DistinctStream(RecordStream> rawStream) {
this.rawStream = rawStream;
}
@Override
public Iterator> iterator() {
Iterator> iterator = rawStream == null ? Collections.emptyIterator()
: rawStream.iterator();
return new DistinctStreamIterator(iterator);
}
private static class DistinctStreamIterator implements Iterator> {
private final Iterator> iterator;
private final Set scannedIds;
private Pair nextPair;
private boolean nextPairSet = false;
public DistinctStreamIterator(Iterator> iterator) {
this.iterator = iterator;
this.scannedIds = new HashSet<>(); // fastest lookup for ids - O(1)
}
@Override
public boolean hasNext() {
return nextPairSet || setNextId();
}
@Override
public Pair next() {
if (!nextPairSet && !setNextId()) {
throw new NoSuchElementException();
}
nextPairSet = false;
return nextPair;
}
private boolean setNextId() {
while (iterator.hasNext()) {
final Pair pair = iterator.next();
if (!scannedIds.contains(pair.getFirst())) {
scannedIds.add(pair.getFirst());
nextPair = pair;
nextPairSet = true;
return true;
}
}
return false;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy