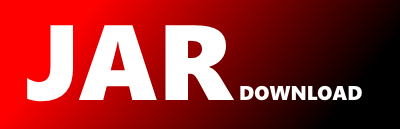
org.dizitart.no2.common.util.Iterables Maven / Gradle / Ivy
/*
* Copyright (c) 2017-2020. Nitrite author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.dizitart.no2.common.util;
import java.lang.reflect.Array;
import java.util.*;
/**
* @author Anindya Chatterjee.
* @since 1.0
*/
public class Iterables {
private Iterables() {}
public static T firstOrNull(Iterable iterable) {
if (iterable == null) return null;
Iterator iterator = iterable.iterator();
if (iterator.hasNext()) {
return iterator.next();
}
return null;
}
public static List toList(Iterable iterable) {
if (iterable instanceof List) return (List) iterable;
List list = new ArrayList<>();
for (T item : iterable) {
list.add(item);
}
return list;
}
public static Set toSet(Iterable iterable) {
if (iterable instanceof Set) return (Set) iterable;
Set set = new LinkedHashSet<>();
for (T item : iterable) {
set.add(item);
}
return set;
}
@SuppressWarnings("unchecked")
public static T[] toArray(Iterable iterable, Class type) {
T[] dummy = (T[]) Array.newInstance(type, 0);
if (iterable instanceof Collection) {
return ((Collection) iterable).toArray(dummy);
} else {
List list = new ArrayList<>();
for (T item : iterable) {
list.add(item);
}
return list.toArray(dummy);
}
}
public static boolean arrayContains(T[] array, T element) {
for (T item : array) {
if (item.equals(element)) return true;
}
return false;
}
@SafeVarargs
public static List listOf(T... items) {
if (items != null) {
return Arrays.asList(items);
}
return Collections.emptyList();
}
@SafeVarargs
public static Set setOf(T... items) {
Set set = new HashSet<>();
if (items != null) {
set.addAll(Arrays.asList(items));
}
return set;
}
public static long size(Iterable> iterable) {
if (iterable instanceof Collection) {
return ((Collection>) iterable).size();
}
long count = 0;
for (Object ignored : iterable) {
count++;
}
return count;
}
@SuppressWarnings({"unchecked", "rawtypes"})
static Object[] toArray(Iterable iterable) {
if (iterable instanceof Collection) {
return ((Collection) iterable).toArray();
} else {
List list = new ArrayList();
for (Object item : iterable) {
list.add(item);
}
return list.toArray();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy