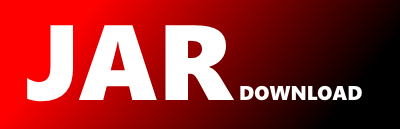
org.dizitart.no2.transaction.Transaction Maven / Gradle / Ivy
package org.dizitart.no2.transaction;
import org.dizitart.no2.collection.NitriteCollection;
import org.dizitart.no2.repository.ObjectRepository;
import org.dizitart.no2.repository.EntityDecorator;
import org.dizitart.no2.index.IndexOptions;
/**
* Represents a transaction in Nitrite database. It provides methods to perform
* transactional operations on Nitrite database collections and repositories.
*
* A transaction can be committed or rolled back. Once a transaction is
* committed, all changes made during the transaction are persisted to the
* underlying store. If a transaction is rolled back, all changes made during
* the transaction are discarded.
*
*
* NOTE: Certain operations are auto-committed in Nitrite database. Those
* operations are not part of a transaction and cannot be rolled back. The
* following operations are auto-committed:
*
*
* - {@link NitriteCollection#createIndex(String...)}
* - {@link NitriteCollection#createIndex(IndexOptions, String...)}
* - {@link NitriteCollection#rebuildIndex(String...)}
* - {@link NitriteCollection#dropIndex(String...)}
* - {@link NitriteCollection#dropAllIndices()}
* - {@link NitriteCollection#clear()}
* - {@link NitriteCollection#drop()}
* - {@link NitriteCollection#close()}
*
* - {@link ObjectRepository#createIndex(String...)}
* - {@link ObjectRepository#createIndex(IndexOptions, String...)}
* - {@link ObjectRepository#rebuildIndex(String...)}
* - {@link ObjectRepository#dropIndex(String...)}
* - {@link ObjectRepository#dropAllIndices()}
* - {@link ObjectRepository#clear()}
* - {@link ObjectRepository#drop()}
* - {@link ObjectRepository#close()}
*
*
* @author Anindya Chatterjee
* @since 4.0
*/
public interface Transaction extends AutoCloseable {
/**
* Gets the unique identifier of the transaction.
*
* @return the unique identifier of the transaction.
*/
String getId();
/**
* Returns the current state of the transaction.
*
* @return the current state of the transaction.
*/
TransactionState getState();
/**
* Gets a {@link NitriteCollection} to perform transactional operations on it.
*
* @param name the name
* @return the collection
*/
NitriteCollection getCollection(String name);
/**
* Gets an {@link ObjectRepository} to perform transactional operations on it.
*
* @param the type parameter
* @param type the type
* @return the repository
*/
ObjectRepository getRepository(Class type);
/**
* Gets an {@link ObjectRepository} to perform transactional operations on it.
*
* @param the type parameter
* @param type the type
* @param key the key
* @return the repository
*/
ObjectRepository getRepository(Class type, String key);
/**
* Gets an {@link ObjectRepository} to perform transactional operations on it.
*
* @param the type parameter
* @param entityDecorator the entityDecorator
* @return the repository
*/
ObjectRepository getRepository(EntityDecorator entityDecorator);
/**
* Gets an {@link ObjectRepository} to perform transactional operations on it.
*
* @param the type parameter
* @param entityDecorator the entityDecorator
* @param key the key
* @return the repository
*/
ObjectRepository getRepository(EntityDecorator entityDecorator, String key);
/**
* Completes the transaction and commits the data to the underlying store.
*/
void commit();
/**
* Rolls back the transaction, discarding any changes made during the
* transaction.
*/
void rollback();
/**
* Closes this {@link Transaction}.
*/
void close();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy