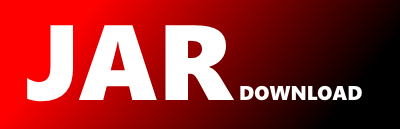
org.dmfs.httpessentials.HttpMethod Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of http-client-essentials Show documentation
Show all versions of http-client-essentials Show documentation
A lightweight http client model.
/*
* Copyright (C) 2016 Marten Gajda
*
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.dmfs.httpessentials;
import org.dmfs.httpessentials.methods.IdempotentMethod;
import org.dmfs.httpessentials.methods.Method;
import org.dmfs.httpessentials.methods.SafeMethod;
/**
* Represents an HTTP method and provides static members for HTTP methods defined in RFC 7231, section 4.3 and RFC 5789
*
* @author Marten Gajda
* @see RFC 7231, section 4
*/
public interface HttpMethod
{
/**
* HTTP Method GET
*
* @see RFC 7231, section 4.3.1
*/
public final static HttpMethod GET = new SafeMethod("GET", false);
/**
* HTTP Method HEAD
*
* @see RFC 7231, section 4.3.2
*/
public final static HttpMethod HEAD = new SafeMethod("HEAD", false);
/**
* HTTP Method POST
*
* @see RFC 7231, section 4.3.3
*/
public final static HttpMethod POST = new Method("POST", true);
/**
* HTTP Method PUT
*
* @see RFC 7231, section 4.3.4
*/
public final static HttpMethod PUT = new IdempotentMethod("PUT", true);
/**
* HTTP Method DELETE
*
* @see RFC 7231, section 4.3.5
*/
public final static HttpMethod DELETE = new IdempotentMethod("DELETE", false);
/**
* HTTP Method CONNECT
*
* @see RFC 7231, section 4.3.6
*/
public final static HttpMethod CONNECT = new Method("CONNECT", false);
/**
* HTTP Method OPTIONS
*
* @see RFC 7231, section 4.3.7
*/
public final static HttpMethod OPTIONS = new SafeMethod("OPTIONS", true);
/**
* HTTP Method TRACE
*
* @see RFC 7231, section 4.3.8
*/
public final static HttpMethod TRACE = new SafeMethod("TRACE", false);
/**
* HTTP Method PATCH
*
* @see RFC 5789
*/
public final static HttpMethod PATCH = new Method("PATCH", true);
/**
* Returns the HTTP verb of this method.
*
* @return A String containing the verb of the method.
*/
public String verb();
/**
* Returns if this request method is safe, which means that the request is not intended and not expected to change
* any state on the server. In effect the semantics are to be considered read-only.
*
* @see RFC 7231, Section 4.2.1
*/
public boolean isSafe();
/**
* Returns if this request method is idempotent, which means that sending multiple identical requests with that
* method has the same effect as sending one single request to the server.
*
* @see RFC 7231, Section 4.2.2
*/
public boolean isIdempotent();
/**
* Returns whether this {@link HttpMethod} allows to send a message body.
*
* Note that RFC 7231 does not explicitly forbid a message payload for some methods (in particular these are {@link
* #GET}, {@link #HEAD}, {@link #DELETE} and {@link #CONNECT}). Instead is says something like this:
*
*
* A payload within a XXX request message has no defined semantics;
* sending a payload body on a XXX request might cause some existing
* implementations to reject the request.
*
*
* The predefined methods in {@link HttpMethod} interpret this rather strict and return false
for such
* methods.
*
* @return true
if this method supports a message body, false
otherwise.
*/
public boolean supportsRequestPayload();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy