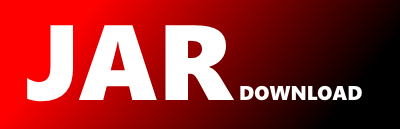
org.dmfs.httpessentials.HttpStatus Maven / Gradle / Ivy
Show all versions of http-client-essentials Show documentation
/*
* Copyright (C) 2016 Marten Gajda
*
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.dmfs.httpessentials;
import org.dmfs.httpessentials.status.NoneHttpStatus;
import org.dmfs.httpessentials.status.SimpleHttpStatus;
/**
* Interface of an HTTP status. Instances must be immutable. By convention, all instances MUST return the status code
* when {@link #hashCode()} is called.
*
* @author Marten Gajda
*/
public interface HttpStatus
{
/*
* Dummy HttpStatus for internal use.
*/
/**
* A dummy HTTP status which can be used to return "no status". This is the null
object.
*/
public final static HttpStatus NONE = new NoneHttpStatus();
/*
* 1xx Informational status codes, see http://tools.ietf.org/html/rfc7231#section-6.2
*/
/**
* HTTP status: 100 Continue
*/
public final static HttpStatus CONTINUE = new SimpleHttpStatus(100, "Continue");
/**
* HTTP status: 101 Switching Protocols
*/
public final static HttpStatus SWITCHING_PROTOCOLS = new SimpleHttpStatus(101, "Switching Protocols");
/**
* HTTP status: 102 Processing (WebDAV) Note that
* this has been removed from the WebDAV specification in RFC 4918, see RFC
* 4918, section 21.4
*/
public final static HttpStatus PROCESSING = new SimpleHttpStatus(102, "Processing");
/*
* 2xx Successful status codes, see: http://tools.ietf.org/html/rfc7231#section-6.3
*/
/**
* HTTP status: 200 OK
*/
public final static HttpStatus OK = new SimpleHttpStatus(200, "OK");
/**
* HTTP status: 201 Created
*/
public final static HttpStatus CREATED = new SimpleHttpStatus(201, "CREATED");
/**
* HTTP status: 202 Accepted
*/
public final static HttpStatus ACCEPTED = new SimpleHttpStatus(202, "Accepted");
/**
* HTTP status: 203 Non-Authoritative Information
*/
public final static HttpStatus NON_AUTHORITATIVE_INFORMATION = new SimpleHttpStatus(203,
"Non-Authoritative Information");
/**
* HTTP status: 204 No Content
*/
public final static HttpStatus NO_CONTENT = new SimpleHttpStatus(204, "No Content");
/**
* HTTP status: 205 Reset Content
*/
public final static HttpStatus RESET_CONTENT = new SimpleHttpStatus(205, "Reset Content");
/**
* HTTP status: 206 Partial Content
*/
public final static HttpStatus PARTIAL_CONTENT = new SimpleHttpStatus(206, "Partial Content");
/**
* HTTP status: 207 Multistatus (WebDAV)
*/
public final static HttpStatus MULTISTATUS = new SimpleHttpStatus(207, "Multistatus");
/*
* 3xx Redirection status codes, see: http://tools.ietf.org/html/rfc2068#section-10.3
*/
/**
* HTTP status: 300 Multiple Choices
*/
public final static HttpStatus MULTIPLE_CHOICES = new SimpleHttpStatus(300, "Multiple Choices");
/**
* HTTP status: 301 Moved Permanently
*/
public final static HttpStatus MOVED_PERMANENTLY = new SimpleHttpStatus(301, "Moved Permanently");
/**
* HTTP status: 302 Moved Temporarily
*/
public final static HttpStatus FOUND = new SimpleHttpStatus(302, "Found");
/**
* HTTP status: 303 See Other
*/
public final static HttpStatus SEE_OTHER = new SimpleHttpStatus(303, "See Other");
/**
* HTTP status: 304 Not Modified
*/
public final static HttpStatus NOT_MODIFIED = new SimpleHttpStatus(304, "Not Modified");
/**
* HTTP status: 305 Use Proxy
*/
public final static HttpStatus USE_PROXY = new SimpleHttpStatus(305, "Use Proxy");
/**
* HTTP status: 307 Temporary Redirect
*/
public final static HttpStatus TEMPORARY_REDIRECT = new SimpleHttpStatus(307, "Temporary Redirect");
/**
* HTTP status: 308 Permanent Redirect
*/
public final static HttpStatus PERMANENT_REDIRECT = new SimpleHttpStatus(308, "Permanent Redirect");
/*
* 4xx Client Error status codes, see: http://tools.ietf.org/html/rfc2068#section-10.4
*/
/**
* HTTP status: 400 Bad Request
*/
public final static HttpStatus BAD_REQUEST = new SimpleHttpStatus(400, "Bad Request");
/**
* HTTP status: 401 Unauthorized
*/
public final static HttpStatus UNAUTHORIZED = new SimpleHttpStatus(401, "Unauthorized");
/**
* HTTP status: 402 Payment Required
*/
public final static HttpStatus PAYMENT_REQUIRED = new SimpleHttpStatus(402, "Payment Required");
/**
* HTTP status: 403 Forbidden
*/
public final static HttpStatus FORBIDDEN = new SimpleHttpStatus(403, "Forbidden");
/**
* HTTP status: 404 Not Found
*/
public final static HttpStatus NOT_FOUND = new SimpleHttpStatus(404, "Not Found");
/**
* HTTP status: 405 Method Not Allowed
*/
public final static HttpStatus METHOD_NOT_ALLOWED = new SimpleHttpStatus(405, "Method Not Allowed");
/**
* HTTP status: 406 Not Acceptable
*/
public final static HttpStatus NOT_ACCEPTABLE = new SimpleHttpStatus(406, "Not Acceptable");
/**
* HTTP status: 407 Proxy Authentication Required
*/
public final static HttpStatus PROXY_AUTHENTICATION_REQUIRED = new SimpleHttpStatus(407,
"Proxy Authentication Required");
/**
* HTTP status: 408 Request Timeout
*/
public final static HttpStatus REQUEST_TIMEOUT = new SimpleHttpStatus(408, "Request Timeout");
/**
* HTTP status: 409 Conflict
*/
public final static HttpStatus CONFLICT = new SimpleHttpStatus(409, "Conflict");
/**
* HTTP status: 410 Gone
*/
public final static HttpStatus GONE = new SimpleHttpStatus(410, "Gone");
/**
* HTTP status: 411 Length Required
*/
public final static HttpStatus LENGTH_REQUIRED = new SimpleHttpStatus(411, "Length Required");
/**
* HTTP status: 412 Precondition Failed
*/
public final static HttpStatus PRECONDITION_FAILED = new SimpleHttpStatus(412, "Precondition Failed");
/**
* HTTP status: 413 Payload Too Large
*/
public final static HttpStatus PAYLOAD_TOO_LARGE = new SimpleHttpStatus(413, "Payload Too Large");
/**
* Old name of {@link #PAYLOAD_TOO_LARGE}.
*/
@Deprecated
public final static HttpStatus REQUEST_ENTITY_TOO_LARGE = PAYLOAD_TOO_LARGE;
/**
* HTTP status: 414 URI Too Long
*/
public final static HttpStatus URI_TOO_LONG = new SimpleHttpStatus(414, "URI Too Long");
/**
* Old name of {@link #URI_TOO_LONG}.
*/
@Deprecated
public final static HttpStatus REQUEST_URI_TOO_LONG = URI_TOO_LONG;
/**
* HTTP status: 415 Unsupported Media Type
*/
public final static HttpStatus UNSUPPORTED_MEDIA_TYPE = new SimpleHttpStatus(415, "Unsupported Media Type");
/**
* HTTP status: 417 Expectation Failed
*/
public final static HttpStatus EXPECTATION_FAILED = new SimpleHttpStatus(417, "Expectation Failed");
/**
* HTTP status: 422 Unprocessable Entity (WebDAV)
*/
public final static HttpStatus UNPROCESSABLE_ENTITY = new SimpleHttpStatus(422, "Unprocessable Entity");
/**
* HTTP status: 423 Locked (WebDAV)
*/
public final static HttpStatus LOCKED = new SimpleHttpStatus(423, "Locked");
/**
* HTTP status: 424 Failed Dependency (WebDAV)
*/
public final static HttpStatus FAILED_DEPENDENCY = new SimpleHttpStatus(424, "Failed Dependency");
/**
* HTTP status: 426 Upgrade Required
*/
public final static HttpStatus UPGRADE_REQUIRED = new SimpleHttpStatus(426, "Upgrade Required");
/*
* 5xx Server Error status codes, see: http://tools.ietf.org/html/rfc2068#section-10.5
*/
/**
* HTTP status: 500 Internal Server Error
*/
public final static HttpStatus INTERNAL_SERVER_ERROR = new SimpleHttpStatus(500, "Internal Server Error");
/**
* HTTP status: 501 Not Implemented
*/
public final static HttpStatus NOT_IMPLEMENTED = new SimpleHttpStatus(501, "Not Implemented");
/**
* HTTP status: 502 Bad Gateway
*/
public final static HttpStatus BAD_GATEWAY = new SimpleHttpStatus(502, "Bad Gateway");
/**
* HTTP status: 503 Service Unavailable
*/
public final static HttpStatus SERVICE_UNAVAILABLE = new SimpleHttpStatus(503, "Service Unavailable");
/**
* HTTP status: 504 Gateway Timeout
*/
public final static HttpStatus GATEWAY_TIMEOUT = new SimpleHttpStatus(504, "Gateway Timeout");
/**
* HTTP status: 505 HTTP Version Not Supported
*/
public final static HttpStatus HTTP_VERSION_NOT_SUPPORTED = new SimpleHttpStatus(505, "HTTP Version Not Supported");
/**
* HTTP status: 506 Variant Also Negotiates
* (experimental)
*/
public final static HttpStatus VARIANT_ALSO_NEGOTIATES = new SimpleHttpStatus(506, "Variant Also Negotiates");
/**
* HTTP status: 507 Insufficient Storage (WebDAV)
*/
public final static HttpStatus INSUFFICIENT_STORAGE = new SimpleHttpStatus(507, "Insufficient Storage");
/**
* Returns the status code.
*
* @return
*/
public int statusCode();
/**
* Returns the reason phrase of this status code.
Note: the reason phrase doesn't contain the
* status code itself.
*
* @return The reason phrase or null
if the status code is unknown.
*/
public String reason();
/**
* Returns a status line in the form:
*
*
* HTTP/VersionMajor.VersionMinor SP Status-Code SP Reason-Phrase
*
*
* @param httpVersionMajor
* The major version number, usually 1
* @param httpVersionMinor
* The minor version number, usually 1
*
* @return
*/
public String httpStatusLine(int httpVersionMajor, int httpVersionMinor);
/**
* Returns whether this represents an informational status code.
*
* @return true
if this represents an informational status code, false
otherwise.
*/
public boolean isInformational();
/**
* Returns whether this status represents a success status code.
*
* @return true
if this represents a success status code, false
otherwise.
*/
public boolean isSuccess();
/**
* Returns whether this status represents a redirection status code.
*
* @return true
if this represents a redirection status code, false
otherwise.
*/
public boolean isRedirect();
/**
* Returns whether this status represents a client error status code.
*
* @return true
if this represents a client error status code, false
otherwise.
*/
public boolean isClientError();
/**
* Returns whether this status represents a server error status code.
*
* @return true
if this represents a server error status code, false
otherwise.
*/
public boolean isServerError();
@Override
public int hashCode();
@Override
public boolean equals(final Object obj);
}