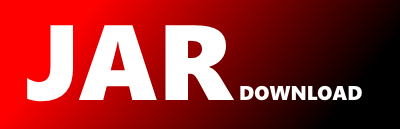
org.docstr.gwt.AbstractBaseOptions Maven / Gradle / Ivy
Show all versions of gwt-gradle-plugin Show documentation
/**
* Copyright (C) 2024 Document Node Pty Ltd
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.docstr.gwt;
import org.gradle.api.file.DirectoryProperty;
import org.gradle.api.provider.ListProperty;
import org.gradle.api.provider.Property;
/**
* Base options for GWT compiler and dev mode
*/
public abstract class AbstractBaseOptions {
/**
* Minimum heap size for the JVM
* @return The minimum heap size
*/
public abstract Property getMinHeapSize();
/**
* Maximum heap size for the JVM
* @return The maximum heap size
*/
public abstract Property getMaxHeapSize();
/**
* -logLevel
* The level of logging detail: ERROR, WARN, INFO, TRACE, DEBUG, SPAM
* or ALL (defaults to INFO)
* @return The log level
*/
public abstract Property getLogLevel();
/**
* -workDir
* The compiler's working directory for internal use (must be writeable;
* defaults to a system temp dir)
* @return The working directory
*/
public abstract DirectoryProperty getWorkDir();
/**
* -gen
*
Debugging: causes normally-transient generated types to be saved
* in the specified directory
*
* @return The generated types directory
*/
public abstract DirectoryProperty getGen();
/**
* -war
* The directory into which deployable output files will be written
* (defaults to 'war')
*
* @return The war directory
*/
public abstract DirectoryProperty getWar();
/**
* -deploy
* The directory into which deployable but not servable output files
* will be written (defaults to 'WEB-INF/deploy' under the -war
* directory/jar, and may be the same as the -extra directory/jar)
*
* @return The deploy directory
*/
public abstract DirectoryProperty getDeploy();
/**
* -extra
* The directory into which extra files, not intended for
* deployment, will be written
*
* @return The extra directory
*/
public abstract DirectoryProperty getExtra();
/**
* -Dgwt.persistentunitcachedir=[YourCacheDir]
* The directory to use for the persistent unit cache
*
* @return The cache directory
*/
public abstract DirectoryProperty getCacheDir();
/**
* -[no]generateJsInteropExports
* Generate exports for JsInterop purposes. If no
* -includeJsInteropExport/-excludeJsInteropExport provided, generates
* all exports. (defaults to OFF)
* @return The generate JsInterop exports flag
*/
public abstract Property getGenerateJsInteropExports();
/**
* -includeJsInteropExports/excludeJsInteropExports
* Include/exclude members and classes while generating JsInterop
* exports. Flag could be set multiple times to expand the pattern.
* (The flag has only effect if exporting is enabled
* via -generateJsInteropExports)
* @return The include/exclude JsInterop exports
*/
public abstract ListProperty getIncludeJsInteropExports();
/**
* -includeJsInteropExports/excludeJsInteropExports
* Include/exclude members and classes while generating JsInterop
* exports. Flag could be set multiple times to expand the pattern.
* (The flag has only effect if exporting is enabled
* via -generateJsInteropExports)
* @return The include/exclude JsInterop exports
*/
public abstract ListProperty getExcludeJsInteropExports();
/**
* -XmethodNameDisplayMode
* EXPERIMENTAL: Specifies method display name mode for chrome devtools:
* NONE, ONLY_METHOD_NAME, ABBREVIATED or FULL (defaults to NONE)
* @return The method name display mode
*/
public abstract Property getMethodNameDisplayMode();
/**
* -sourceLevel
* Specifies Java source level (defaults to 1.8)
* @return The source level
*/
public abstract Property getSourceLevel();
/**
* -[no]incremental
* Compiles faster by reusing data from the previous compile.
* (defaults to OFF)
* @return The incremental flag
*/
public abstract Property getIncremental();
/**
* -style
* Script output style: DETAILED, OBFUSCATED or PRETTY
* (defaults to OBFUSCATED)
* @return The style
*/
public abstract Property getStyle();
/**
* -[no]failOnError
* Fail compilation if any input file contains an error. (defaults to OFF)
* @return The fail on error flag
*/
public abstract Property getFailOnError();
/**
* -setProperty
* Set the values of a property in the form of
* propertyName=value1[,value2...].
* Example: -setProperties = ["user.agent=safari", "locale=default"]
* would add the parameters -setProperty user.agent=safari -setProperty locale=default
* @return The set properties
*/
public abstract ListProperty getSetProperty();
/**
* module[s]
* Specifies the name(s) of the module(s) to host
* @return The modules
*/
public abstract ListProperty getModules();
}