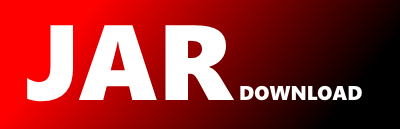
org.docstr.gwt.options.GwtTestOptions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gwt-gradle-plugin Show documentation
Show all versions of gwt-gradle-plugin Show documentation
Gradle plugin to support GWT (http://www.gwtproject.org/) related tasks.
/**
* Copyright (C) 2024 Document Node Pty Ltd
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package org.docstr.gwt.options;
import java.io.File;
import org.docstr.gwt.AbstractBaseOptions;
import org.gradle.api.file.DirectoryProperty;
import org.gradle.api.provider.ListProperty;
import org.gradle.api.provider.Property;
/**
* GWT test options
*/
public abstract class GwtTestOptions extends AbstractBaseOptions {
/**
* Builds the parameter string for the GWT test options 'gwt.args'
*
* @return The parameter string
*/
public String getParameterString() {
final StringBuilder builder = new StringBuilder();
dirArgIfSet(builder, "-war", getWar());
dirArgIfSet(builder, "-deploy", getDeploy());
dirArgIfSet(builder, "-extra", getExtra());
dirArgIfSet(builder, "-workDir", getWorkDir());
dirArgIfSet(builder, "-gen", getGen());
argIfSet(builder, "-logLevel", getLogLevel());
argIfSet(builder, "-sourceLevel", getSourceLevel());
argIfSet(builder, "-port", getPort());
argIfSet(builder, "-whitelist", getWhitelist());
argIfSet(builder, "-blacklist", getBlacklist());
dirArgIfSet(builder, "-logdir", getLogdir());
argIfSet(builder, "-codeServerPort", getCodeServerPort());
argIfSet(builder, "-style", getStyle());
argIfEnabled(builder, getEa(), "-ea");
argIfEnabled(builder, getDisableClassMetadata(), "-XdisableClassMetadata");
argIfEnabled(builder, getDisableCastChecking(), "-XdisableCastChecking");
argIfEnabled(builder, getDraftCompile(), "-draftCompile");
argIfSet(builder, "-localWorkers", getLocalWorkers());
argIfEnabled(builder, getProd(), "-prod");
argIfSet(builder, "-testMethodTimeout", getTestMethodTimeout());
argIfSet(builder, "-testBeginTimeout", getTestBeginTimeout());
argIfSet(builder, "-runStyle", getRunStyle());
argIfEnabled(builder, getNotHeadless(), "-notHeadless");
argIfEnabled(builder, getStandardsMode(), "-standardsMode");
argIfEnabled(builder, getQuirksMode(), "-quirksMode");
argIfSet(builder, "-Xtries", getTries());
argIfSet(builder, "-userAgents", getUserAgents());
return builder.toString();
}
private void argIfEnabled(
StringBuilder builder, Property condition, String arg) {
if (condition.isPresent() && Boolean.TRUE.equals(condition.get())) {
arg(builder, arg);
}
}
private void dirArgIfSet(
StringBuilder builder, String arg, DirectoryProperty dir) {
if (dir != null && dir.isPresent()) {
File dirAsFile = dir.getAsFile().get();
dirAsFile.mkdirs();
arg(builder, arg, dirAsFile);
}
}
private void argIfSet(
StringBuilder builder, String arg, Property value) {
if (value != null && value.isPresent()) {
arg(builder, arg, value.get());
}
}
private void arg(StringBuilder builder, Object... args) {
for (Object arg : args) {
if (builder.length() > 0) {
builder.append(' ');
}
builder.append(arg.toString());
}
}
/**
* -port
* Specifies the TCP port for the embedded web server (defaults to 8888)
*
* @return The port
*/
public abstract Property getPort();
/**
* -logdir
* Logs to a file in the given directory, as well as graphically
*
* @return The log directory
*/
public abstract DirectoryProperty getLogdir();
/**
* -whitelist
*
* @return The whitelist
*/
public abstract Property getWhitelist();
/**
* -blacklist
*
* @return The blacklist
*/
public abstract Property getBlacklist();
/**
* -codeServerPort
* Specifies the TCP port for the code server (defaults to 9997 for
* classic Dev Mode or 9876 for Super Dev Mode)
*
* @return The code server port
*/
public abstract Property getCodeServerPort();
/**
* -ea
*
* @return The ea flag
*/
public abstract Property getEa();
/**
* -XdisableClassMetadata
*
* @return The disable class metadata flag
*/
public abstract Property getDisableClassMetadata();
/**
* -XdisableCastChecking
*
* @return The disable cast checking flag
*/
public abstract Property getDisableCastChecking();
/**
* -draftCompile
*
* @return The draft compile flag
*/
public abstract Property getDraftCompile();
/**
* -localWorkers
*
* @return The local workers
*/
public abstract Property getLocalWorkers();
/**
* -prod
*
* @return The prod flag
*/
public abstract Property getProd();
/**
* -testMethodTimeout
*
* @return The test method timeout
*/
public abstract Property getTestMethodTimeout();
/**
* -testBeginTimeout
*
* @return The test begin timeout
*/
public abstract Property getTestBeginTimeout();
/**
* -runStyle
*
* @return The run style
*/
public abstract Property getRunStyle();
/**
* -notHeadless
*
* @return The not headless flag
*/
public abstract Property getNotHeadless();
/**
* -standardsMode
*
* @return The standards mode flag
*/
public abstract Property getStandardsMode();
/**
* -quirksMode
*
* @return The quirks mode flag
*/
public abstract Property getQuirksMode();
/**
* -Xtries
*
* @return The tries
*/
public abstract Property getTries();
/**
* -userAgents
*
* @return The user agents
*/
public abstract Property getUserAgents();
/**
* Names of the test tasks to configure for GWT.
*
* This defaults to an empty list.
* For backwards compatibility, an empty list is interpreted to mean all tasks of type {@link org.gradle.api.tasks.testing.Test Test}.
*
*
* To disable any test task configuration, this can be set to {@code null}.
*
*
* @return The test task names.
*/
public abstract ListProperty getTestTasks();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy