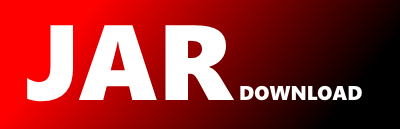
org.docx4j.model.styles.Tree Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of docx4j Show documentation
Show all versions of docx4j Show documentation
docx4j is a library which helps you to work with the Office Open
XML file format as used in docx
documents, pptx presentations, and xlsx spreadsheets.
package org.docx4j.model.styles;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Set;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.docx4j.model.PropertyResolver;
import org.docx4j.openpackaging.packages.WordprocessingMLPackage;
import org.docx4j.wml.Style;
import org.docx4j.wml.Styles;
/**
* Represents a Tree of Objects of generic type T. The Tree is represented as
* a single rootElement which points to a List> of children. There is
* no restriction on the number of children that a particular node may have.
* This Tree provides a method to serialize the Tree into a List by doing a
* pre-order traversal. It has several methods to allow easy updation of Nodes
* in the Tree.
*
* Original code from http://sujitpal.blogspot.com/2006/05/java-data-structure-generic-tree.html
*/
public class Tree {
private static Logger log = LoggerFactory.getLogger(Tree.class);
private Node rootElement;
// private Map> nodes = new HashMap>(); // weird compile errors on put with this
protected Map nodes = new HashMap();
/**
* Quick access to any node in the tree.
* @param name
* @return
*/
public Node get(String name) {
// if (log.isDebugEnabled()) {
// Node result = (Node)nodes.get(name);
// if (result==null) {
// log.warn("Null result for " + name);
// }
// return result;
// }
return (Node)nodes.get(name);
}
/**
* Return the root Node of the tree.
* @return the root element.
*/
public Node getRootElement() {
return this.rootElement;
}
/**
* Set the root Element for the tree.
* @param rootElement the root element to set.
*/
public void setRootElement(Node rootElement) {
this.rootElement = rootElement;
nodes.put(rootElement.name, rootElement);
}
/**
* Returns the Tree as a List of Node objects. The elements of the
* List are generated from a pre-order traversal of the tree.
* @return a List>.
*/
public List> toList() {
List> list = new ArrayList>();
walk(rootElement, list);
return list;
}
/**
* Returns a String representation of the Tree. The elements are generated
* from a pre-order traversal of the Tree.
* @return the String representation of the Tree.
*/
public String toString() {
StringBuffer sb = new StringBuffer();
for (Node n : toList() ) {
sb.append(n.name + "\n");
}
return sb.toString();
}
/**
* Walks the Tree in pre-order style. This is a recursive method, and is
* called from the toList() method with the root element as the first
* argument. It appends to the second argument, which is passed by reference
* as it recurses down the tree.
* @param element the starting element.
* @param list the output of the walk.
*/
private void walk(Node element, List> list) {
if (element==null) {return;}
list.add(element);
for (Node data : element.getChildren()) {
walk(data, list);
}
}
public List> climb(Node n) {
List> list = new ArrayList>();
climb(n, list);
return list;
}
private List> climb(Node n, List> list) {
list.add(n);
if (n.equals(this.rootElement)) {
return list;
} else {
Node parent = n.getParent();
if (parent==null) { // no basedOn
return list;
}
climb(parent, list);
}
return list;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy