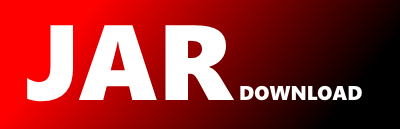
annotations.io.fabric8.kubernetes.api.model.ServiceAccountFluentImpl Maven / Gradle / Ivy
package io.fabric8.kubernetes.api.model;
import io.fabric8.kubernetes.api.builder.BaseFluent;
import java.lang.String;
import javax.validation.constraints.NotNull;
import com.fasterxml.jackson.annotation.JsonProperty;
import io.fabric8.kubernetes.api.builder.VisitableBuilder;
import java.util.List;
import javax.validation.Valid;
import java.util.ArrayList;
import io.fabric8.kubernetes.api.model.validators.CheckObjectMeta;
import java.lang.Object;
import io.fabric8.kubernetes.api.builder.Nested;
public class ServiceAccountFluentImpl> extends BaseFluent implements ServiceAccountFluent{
private String apiVersion;
private List> imagePullSecrets = new ArrayList>();
private String kind;
private VisitableBuilder extends ObjectMeta,?> metadata;
private List> secrets = new ArrayList>();
public ServiceAccountFluentImpl(){
}
public ServiceAccountFluentImpl(ServiceAccount instance){
this.withApiVersion(instance.getApiVersion());
this.withImagePullSecrets(instance.getImagePullSecrets());
this.withKind(instance.getKind());
this.withMetadata(instance.getMetadata());
this.withSecrets(instance.getSecrets());
}
public String getApiVersion(){
return this.apiVersion;
}
public A withApiVersion(String apiVersion){
this.apiVersion=apiVersion; return (A) this;
}
public A addToImagePullSecrets(LocalObjectReference... items){
for (LocalObjectReference item : items) {LocalObjectReferenceBuilder builder = new LocalObjectReferenceBuilder(item);_visitables.add(builder);this.imagePullSecrets.add(builder);} return (A)this;
}
public A removeFromImagePullSecrets(LocalObjectReference... items){
for (LocalObjectReference item : items) {LocalObjectReferenceBuilder builder = new LocalObjectReferenceBuilder(item);_visitables.remove(builder);this.imagePullSecrets.remove(builder);} return (A)this;
}
public List getImagePullSecrets(){
return build(imagePullSecrets);
}
public A withImagePullSecrets(List imagePullSecrets){
this.imagePullSecrets.clear();
if (imagePullSecrets != null) {for (LocalObjectReference item : imagePullSecrets){this.addToImagePullSecrets(item);}} return (A) this;
}
public A withImagePullSecrets(LocalObjectReference... imagePullSecrets){
this.imagePullSecrets.clear(); if (imagePullSecrets != null) {for (LocalObjectReference item :imagePullSecrets){ this.addToImagePullSecrets(item);}} return (A) this;
}
public ServiceAccountFluent.ImagePullSecretsNested addNewImagePullSecret(){
return new ImagePullSecretsNestedImpl();
}
public ServiceAccountFluent.ImagePullSecretsNested addNewImagePullSecretLike(LocalObjectReference item){
return new ImagePullSecretsNestedImpl(item);
}
public A addNewImagePullSecret(String name){
return (A)addToImagePullSecrets(new LocalObjectReference(name));
}
public String getKind(){
return this.kind;
}
public A withKind(String kind){
this.kind=kind; return (A) this;
}
public ObjectMeta getMetadata(){
return this.metadata!=null?this.metadata.build():null;
}
public A withMetadata(ObjectMeta metadata){
if (metadata!=null){ this.metadata= new ObjectMetaBuilder(metadata); _visitables.add(this.metadata);} return (A) this;
}
public ServiceAccountFluent.MetadataNested withNewMetadata(){
return new MetadataNestedImpl();
}
public ServiceAccountFluent.MetadataNested withNewMetadataLike(ObjectMeta item){
return new MetadataNestedImpl(item);
}
public ServiceAccountFluent.MetadataNested editMetadata(){
return withNewMetadataLike(getMetadata());
}
public A addToSecrets(ObjectReference... items){
for (ObjectReference item : items) {ObjectReferenceBuilder builder = new ObjectReferenceBuilder(item);_visitables.add(builder);this.secrets.add(builder);} return (A)this;
}
public A removeFromSecrets(ObjectReference... items){
for (ObjectReference item : items) {ObjectReferenceBuilder builder = new ObjectReferenceBuilder(item);_visitables.remove(builder);this.secrets.remove(builder);} return (A)this;
}
public List getSecrets(){
return build(secrets);
}
public A withSecrets(List secrets){
this.secrets.clear();
if (secrets != null) {for (ObjectReference item : secrets){this.addToSecrets(item);}} return (A) this;
}
public A withSecrets(ObjectReference... secrets){
this.secrets.clear(); if (secrets != null) {for (ObjectReference item :secrets){ this.addToSecrets(item);}} return (A) this;
}
public ServiceAccountFluent.SecretsNested addNewSecret(){
return new SecretsNestedImpl();
}
public ServiceAccountFluent.SecretsNested addNewSecretLike(ObjectReference item){
return new SecretsNestedImpl(item);
}
public boolean equals(Object o){
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
if (!super.equals(o)) return false;
ServiceAccountFluentImpl that = (ServiceAccountFluentImpl) o;
if (apiVersion != null ? !apiVersion.equals(that.apiVersion) :that.apiVersion != null) return false;
if (imagePullSecrets != null ? !imagePullSecrets.equals(that.imagePullSecrets) :that.imagePullSecrets != null) return false;
if (kind != null ? !kind.equals(that.kind) :that.kind != null) return false;
if (metadata != null ? !metadata.equals(that.metadata) :that.metadata != null) return false;
if (secrets != null ? !secrets.equals(that.secrets) :that.secrets != null) return false;
return true;
}
public class ImagePullSecretsNestedImpl extends LocalObjectReferenceFluentImpl> implements ServiceAccountFluent.ImagePullSecretsNested,Nested{
private final LocalObjectReferenceBuilder builder;
ImagePullSecretsNestedImpl(){
this.builder = new LocalObjectReferenceBuilder(this);
}
ImagePullSecretsNestedImpl(LocalObjectReference item){
this.builder = new LocalObjectReferenceBuilder(this, item);
}
public N endImagePullSecret(){
return and();
}
public N and(){
return (N) ServiceAccountFluentImpl.this.addToImagePullSecrets(builder.build());
}
}
public class MetadataNestedImpl extends ObjectMetaFluentImpl> implements ServiceAccountFluent.MetadataNested,Nested{
private final ObjectMetaBuilder builder;
MetadataNestedImpl(){
this.builder = new ObjectMetaBuilder(this);
}
MetadataNestedImpl(ObjectMeta item){
this.builder = new ObjectMetaBuilder(this, item);
}
public N endMetadata(){
return and();
}
public N and(){
return (N) ServiceAccountFluentImpl.this.withMetadata(builder.build());
}
}
public class SecretsNestedImpl extends ObjectReferenceFluentImpl> implements ServiceAccountFluent.SecretsNested,Nested{
private final ObjectReferenceBuilder builder;
SecretsNestedImpl(){
this.builder = new ObjectReferenceBuilder(this);
}
SecretsNestedImpl(ObjectReference item){
this.builder = new ObjectReferenceBuilder(this, item);
}
public N endSecret(){
return and();
}
public N and(){
return (N) ServiceAccountFluentImpl.this.addToSecrets(builder.build());
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy