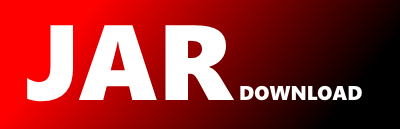
annotations.io.fabric8.openshift.api.model.DockerConfigFluentImpl Maven / Gradle / Ivy
package io.fabric8.openshift.api.model;
import io.fabric8.kubernetes.api.builder.BaseFluent;
import java.lang.Boolean;
import com.fasterxml.jackson.annotation.JsonProperty;
import java.lang.String;
import java.util.List;
import javax.validation.Valid;
import java.util.ArrayList;
import java.lang.Long;
import java.lang.Object;
import java.util.Map;
import java.util.LinkedHashMap;
public class DockerConfigFluentImpl> extends BaseFluent implements DockerConfigFluent{
private Boolean attachStderr;
private Boolean attachStdin;
private Boolean attachStdout;
private List cmd = new ArrayList();
private Long cpuShares;
private String cpuset;
private List dns = new ArrayList();
private String domainname;
private List entrypoint = new ArrayList();
private List env = new ArrayList();
private Map exposedPorts = new LinkedHashMap();
private String hostname;
private String image;
private Map labels = new LinkedHashMap();
private Long memory;
private Long memorySwap;
private Boolean networkDisabled;
private List onBuild = new ArrayList();
private Boolean openStdin;
private List portSpecs = new ArrayList();
private List securityOpts = new ArrayList();
private Boolean stdinOnce;
private Boolean tty;
private String user;
private Map volumes = new LinkedHashMap();
private String volumesFrom;
private String workingDir;
public DockerConfigFluentImpl(){
}
public DockerConfigFluentImpl(DockerConfig instance){
this.withAttachStderr(instance.getAttachStderr());
this.withAttachStdin(instance.getAttachStdin());
this.withAttachStdout(instance.getAttachStdout());
this.withCmd(instance.getCmd());
this.withCpuShares(instance.getCpuShares());
this.withCpuset(instance.getCpuset());
this.withDns(instance.getDns());
this.withDomainname(instance.getDomainname());
this.withEntrypoint(instance.getEntrypoint());
this.withEnv(instance.getEnv());
this.withExposedPorts(instance.getExposedPorts());
this.withHostname(instance.getHostname());
this.withImage(instance.getImage());
this.withLabels(instance.getLabels());
this.withMemory(instance.getMemory());
this.withMemorySwap(instance.getMemorySwap());
this.withNetworkDisabled(instance.getNetworkDisabled());
this.withOnBuild(instance.getOnBuild());
this.withOpenStdin(instance.getOpenStdin());
this.withPortSpecs(instance.getPortSpecs());
this.withSecurityOpts(instance.getSecurityOpts());
this.withStdinOnce(instance.getStdinOnce());
this.withTty(instance.getTty());
this.withUser(instance.getUser());
this.withVolumes(instance.getVolumes());
this.withVolumesFrom(instance.getVolumesFrom());
this.withWorkingDir(instance.getWorkingDir());
}
public Boolean isAttachStderr(){
return this.attachStderr;
}
public A withAttachStderr(Boolean attachStderr){
this.attachStderr=attachStderr; return (A) this;
}
public Boolean isAttachStdin(){
return this.attachStdin;
}
public A withAttachStdin(Boolean attachStdin){
this.attachStdin=attachStdin; return (A) this;
}
public Boolean isAttachStdout(){
return this.attachStdout;
}
public A withAttachStdout(Boolean attachStdout){
this.attachStdout=attachStdout; return (A) this;
}
public A addToCmd(String... items){
for (String item : items) {this.cmd.add(item);} return (A)this;
}
public A removeFromCmd(String... items){
for (String item : items) {this.cmd.remove(item);} return (A)this;
}
public List getCmd(){
return this.cmd;
}
public A withCmd(List cmd){
this.cmd.clear();
if (cmd != null) {for (String item : cmd){this.addToCmd(item);}} return (A) this;
}
public A withCmd(String... cmd){
this.cmd.clear(); if (cmd != null) {for (String item :cmd){ this.addToCmd(item);}} return (A) this;
}
public Long getCpuShares(){
return this.cpuShares;
}
public A withCpuShares(Long cpuShares){
this.cpuShares=cpuShares; return (A) this;
}
public String getCpuset(){
return this.cpuset;
}
public A withCpuset(String cpuset){
this.cpuset=cpuset; return (A) this;
}
public A addToDns(String... items){
for (String item : items) {this.dns.add(item);} return (A)this;
}
public A removeFromDns(String... items){
for (String item : items) {this.dns.remove(item);} return (A)this;
}
public List getDns(){
return this.dns;
}
public A withDns(List dns){
this.dns.clear();
if (dns != null) {for (String item : dns){this.addToDns(item);}} return (A) this;
}
public A withDns(String... dns){
this.dns.clear(); if (dns != null) {for (String item :dns){ this.addToDns(item);}} return (A) this;
}
public String getDomainname(){
return this.domainname;
}
public A withDomainname(String domainname){
this.domainname=domainname; return (A) this;
}
public A addToEntrypoint(String... items){
for (String item : items) {this.entrypoint.add(item);} return (A)this;
}
public A removeFromEntrypoint(String... items){
for (String item : items) {this.entrypoint.remove(item);} return (A)this;
}
public List getEntrypoint(){
return this.entrypoint;
}
public A withEntrypoint(List entrypoint){
this.entrypoint.clear();
if (entrypoint != null) {for (String item : entrypoint){this.addToEntrypoint(item);}} return (A) this;
}
public A withEntrypoint(String... entrypoint){
this.entrypoint.clear(); if (entrypoint != null) {for (String item :entrypoint){ this.addToEntrypoint(item);}} return (A) this;
}
public A addToEnv(String... items){
for (String item : items) {this.env.add(item);} return (A)this;
}
public A removeFromEnv(String... items){
for (String item : items) {this.env.remove(item);} return (A)this;
}
public List getEnv(){
return this.env;
}
public A withEnv(List env){
this.env.clear();
if (env != null) {for (String item : env){this.addToEnv(item);}} return (A) this;
}
public A withEnv(String... env){
this.env.clear(); if (env != null) {for (String item :env){ this.addToEnv(item);}} return (A) this;
}
public A addToExposedPorts(String key,Object value){
if(key != null && value != null) {this.exposedPorts.put(key, value);} return (A)this;
}
public A addToExposedPorts(Map map){
if(map != null) { this.exposedPorts.putAll(map);} return (A)this;
}
public A removeFromExposedPorts(String key){
if(key != null) {this.exposedPorts.remove(key);} return (A)this;
}
public A removeFromExposedPorts(Map map){
if(map != null) { for(Object key : map.keySet()) {this.exposedPorts.remove(key);}} return (A)this;
}
public Map getExposedPorts(){
return this.exposedPorts;
}
public A withExposedPorts(Map exposedPorts){
this.exposedPorts.clear();
if (exposedPorts != null) {this.exposedPorts.putAll(exposedPorts);} return (A) this;
}
public String getHostname(){
return this.hostname;
}
public A withHostname(String hostname){
this.hostname=hostname; return (A) this;
}
public String getImage(){
return this.image;
}
public A withImage(String image){
this.image=image; return (A) this;
}
public A addToLabels(String key,String value){
if(key != null && value != null) {this.labels.put(key, value);} return (A)this;
}
public A addToLabels(Map map){
if(map != null) { this.labels.putAll(map);} return (A)this;
}
public A removeFromLabels(String key){
if(key != null) {this.labels.remove(key);} return (A)this;
}
public A removeFromLabels(Map map){
if(map != null) { for(Object key : map.keySet()) {this.labels.remove(key);}} return (A)this;
}
public Map getLabels(){
return this.labels;
}
public A withLabels(Map labels){
this.labels.clear();
if (labels != null) {this.labels.putAll(labels);} return (A) this;
}
public Long getMemory(){
return this.memory;
}
public A withMemory(Long memory){
this.memory=memory; return (A) this;
}
public Long getMemorySwap(){
return this.memorySwap;
}
public A withMemorySwap(Long memorySwap){
this.memorySwap=memorySwap; return (A) this;
}
public Boolean isNetworkDisabled(){
return this.networkDisabled;
}
public A withNetworkDisabled(Boolean networkDisabled){
this.networkDisabled=networkDisabled; return (A) this;
}
public A addToOnBuild(String... items){
for (String item : items) {this.onBuild.add(item);} return (A)this;
}
public A removeFromOnBuild(String... items){
for (String item : items) {this.onBuild.remove(item);} return (A)this;
}
public List getOnBuild(){
return this.onBuild;
}
public A withOnBuild(List onBuild){
this.onBuild.clear();
if (onBuild != null) {for (String item : onBuild){this.addToOnBuild(item);}} return (A) this;
}
public A withOnBuild(String... onBuild){
this.onBuild.clear(); if (onBuild != null) {for (String item :onBuild){ this.addToOnBuild(item);}} return (A) this;
}
public Boolean isOpenStdin(){
return this.openStdin;
}
public A withOpenStdin(Boolean openStdin){
this.openStdin=openStdin; return (A) this;
}
public A addToPortSpecs(String... items){
for (String item : items) {this.portSpecs.add(item);} return (A)this;
}
public A removeFromPortSpecs(String... items){
for (String item : items) {this.portSpecs.remove(item);} return (A)this;
}
public List getPortSpecs(){
return this.portSpecs;
}
public A withPortSpecs(List portSpecs){
this.portSpecs.clear();
if (portSpecs != null) {for (String item : portSpecs){this.addToPortSpecs(item);}} return (A) this;
}
public A withPortSpecs(String... portSpecs){
this.portSpecs.clear(); if (portSpecs != null) {for (String item :portSpecs){ this.addToPortSpecs(item);}} return (A) this;
}
public A addToSecurityOpts(String... items){
for (String item : items) {this.securityOpts.add(item);} return (A)this;
}
public A removeFromSecurityOpts(String... items){
for (String item : items) {this.securityOpts.remove(item);} return (A)this;
}
public List getSecurityOpts(){
return this.securityOpts;
}
public A withSecurityOpts(List securityOpts){
this.securityOpts.clear();
if (securityOpts != null) {for (String item : securityOpts){this.addToSecurityOpts(item);}} return (A) this;
}
public A withSecurityOpts(String... securityOpts){
this.securityOpts.clear(); if (securityOpts != null) {for (String item :securityOpts){ this.addToSecurityOpts(item);}} return (A) this;
}
public Boolean isStdinOnce(){
return this.stdinOnce;
}
public A withStdinOnce(Boolean stdinOnce){
this.stdinOnce=stdinOnce; return (A) this;
}
public Boolean isTty(){
return this.tty;
}
public A withTty(Boolean tty){
this.tty=tty; return (A) this;
}
public String getUser(){
return this.user;
}
public A withUser(String user){
this.user=user; return (A) this;
}
public A addToVolumes(String key,Object value){
if(key != null && value != null) {this.volumes.put(key, value);} return (A)this;
}
public A addToVolumes(Map map){
if(map != null) { this.volumes.putAll(map);} return (A)this;
}
public A removeFromVolumes(String key){
if(key != null) {this.volumes.remove(key);} return (A)this;
}
public A removeFromVolumes(Map map){
if(map != null) { for(Object key : map.keySet()) {this.volumes.remove(key);}} return (A)this;
}
public Map getVolumes(){
return this.volumes;
}
public A withVolumes(Map volumes){
this.volumes.clear();
if (volumes != null) {this.volumes.putAll(volumes);} return (A) this;
}
public String getVolumesFrom(){
return this.volumesFrom;
}
public A withVolumesFrom(String volumesFrom){
this.volumesFrom=volumesFrom; return (A) this;
}
public String getWorkingDir(){
return this.workingDir;
}
public A withWorkingDir(String workingDir){
this.workingDir=workingDir; return (A) this;
}
public boolean equals(Object o){
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
if (!super.equals(o)) return false;
DockerConfigFluentImpl that = (DockerConfigFluentImpl) o;
if (attachStderr != null ? !attachStderr.equals(that.attachStderr) :that.attachStderr != null) return false;
if (attachStdin != null ? !attachStdin.equals(that.attachStdin) :that.attachStdin != null) return false;
if (attachStdout != null ? !attachStdout.equals(that.attachStdout) :that.attachStdout != null) return false;
if (cmd != null ? !cmd.equals(that.cmd) :that.cmd != null) return false;
if (cpuShares != null ? !cpuShares.equals(that.cpuShares) :that.cpuShares != null) return false;
if (cpuset != null ? !cpuset.equals(that.cpuset) :that.cpuset != null) return false;
if (dns != null ? !dns.equals(that.dns) :that.dns != null) return false;
if (domainname != null ? !domainname.equals(that.domainname) :that.domainname != null) return false;
if (entrypoint != null ? !entrypoint.equals(that.entrypoint) :that.entrypoint != null) return false;
if (env != null ? !env.equals(that.env) :that.env != null) return false;
if (exposedPorts != null ? !exposedPorts.equals(that.exposedPorts) :that.exposedPorts != null) return false;
if (hostname != null ? !hostname.equals(that.hostname) :that.hostname != null) return false;
if (image != null ? !image.equals(that.image) :that.image != null) return false;
if (labels != null ? !labels.equals(that.labels) :that.labels != null) return false;
if (memory != null ? !memory.equals(that.memory) :that.memory != null) return false;
if (memorySwap != null ? !memorySwap.equals(that.memorySwap) :that.memorySwap != null) return false;
if (networkDisabled != null ? !networkDisabled.equals(that.networkDisabled) :that.networkDisabled != null) return false;
if (onBuild != null ? !onBuild.equals(that.onBuild) :that.onBuild != null) return false;
if (openStdin != null ? !openStdin.equals(that.openStdin) :that.openStdin != null) return false;
if (portSpecs != null ? !portSpecs.equals(that.portSpecs) :that.portSpecs != null) return false;
if (securityOpts != null ? !securityOpts.equals(that.securityOpts) :that.securityOpts != null) return false;
if (stdinOnce != null ? !stdinOnce.equals(that.stdinOnce) :that.stdinOnce != null) return false;
if (tty != null ? !tty.equals(that.tty) :that.tty != null) return false;
if (user != null ? !user.equals(that.user) :that.user != null) return false;
if (volumes != null ? !volumes.equals(that.volumes) :that.volumes != null) return false;
if (volumesFrom != null ? !volumesFrom.equals(that.volumesFrom) :that.volumesFrom != null) return false;
if (workingDir != null ? !workingDir.equals(that.workingDir) :that.workingDir != null) return false;
return true;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy