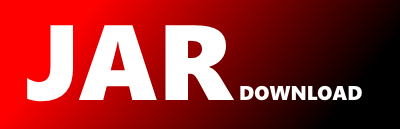
annotations.io.fabric8.openshift.api.model.OAuthClientFluentImpl Maven / Gradle / Ivy
package io.fabric8.openshift.api.model;
import io.fabric8.kubernetes.api.builder.BaseFluent;
import java.lang.String;
import java.util.List;
import com.fasterxml.jackson.annotation.JsonProperty;
import javax.validation.Valid;
import java.util.ArrayList;
import javax.validation.constraints.NotNull;
import io.fabric8.kubernetes.api.builder.VisitableBuilder;
import io.fabric8.kubernetes.api.model.validators.CheckObjectMeta;
import java.lang.Boolean;
import io.fabric8.kubernetes.api.model.ObjectMeta;
import java.lang.Object;
import io.fabric8.kubernetes.api.builder.Nested;
import io.fabric8.kubernetes.api.model.ObjectMetaFluentImpl;
import io.fabric8.kubernetes.api.model.ObjectMetaBuilder;
public class OAuthClientFluentImpl> extends BaseFluent implements OAuthClientFluent{
private List additionalSecrets = new ArrayList();
private String apiVersion;
private String grantMethod;
private String kind;
private VisitableBuilder extends ObjectMeta,?> metadata;
private List redirectURIs = new ArrayList();
private Boolean respondWithChallenges;
private List> scopeRestrictions = new ArrayList>();
private String secret;
public OAuthClientFluentImpl(){
}
public OAuthClientFluentImpl(OAuthClient instance){
this.withAdditionalSecrets(instance.getAdditionalSecrets());
this.withApiVersion(instance.getApiVersion());
this.withGrantMethod(instance.getGrantMethod());
this.withKind(instance.getKind());
this.withMetadata(instance.getMetadata());
this.withRedirectURIs(instance.getRedirectURIs());
this.withRespondWithChallenges(instance.getRespondWithChallenges());
this.withScopeRestrictions(instance.getScopeRestrictions());
this.withSecret(instance.getSecret());
}
public A addToAdditionalSecrets(String... items){
for (String item : items) {this.additionalSecrets.add(item);} return (A)this;
}
public A removeFromAdditionalSecrets(String... items){
for (String item : items) {this.additionalSecrets.remove(item);} return (A)this;
}
public List getAdditionalSecrets(){
return this.additionalSecrets;
}
public A withAdditionalSecrets(List additionalSecrets){
this.additionalSecrets.clear();
if (additionalSecrets != null) {for (String item : additionalSecrets){this.addToAdditionalSecrets(item);}} return (A) this;
}
public A withAdditionalSecrets(String... additionalSecrets){
this.additionalSecrets.clear(); if (additionalSecrets != null) {for (String item :additionalSecrets){ this.addToAdditionalSecrets(item);}} return (A) this;
}
public String getApiVersion(){
return this.apiVersion;
}
public A withApiVersion(String apiVersion){
this.apiVersion=apiVersion; return (A) this;
}
public String getGrantMethod(){
return this.grantMethod;
}
public A withGrantMethod(String grantMethod){
this.grantMethod=grantMethod; return (A) this;
}
public String getKind(){
return this.kind;
}
public A withKind(String kind){
this.kind=kind; return (A) this;
}
public ObjectMeta getMetadata(){
return this.metadata!=null?this.metadata.build():null;
}
public A withMetadata(ObjectMeta metadata){
if (metadata!=null){ this.metadata= new ObjectMetaBuilder(metadata); _visitables.add(this.metadata);} return (A) this;
}
public OAuthClientFluent.MetadataNested withNewMetadata(){
return new MetadataNestedImpl();
}
public OAuthClientFluent.MetadataNested withNewMetadataLike(ObjectMeta item){
return new MetadataNestedImpl(item);
}
public OAuthClientFluent.MetadataNested editMetadata(){
return withNewMetadataLike(getMetadata());
}
public A addToRedirectURIs(String... items){
for (String item : items) {this.redirectURIs.add(item);} return (A)this;
}
public A removeFromRedirectURIs(String... items){
for (String item : items) {this.redirectURIs.remove(item);} return (A)this;
}
public List getRedirectURIs(){
return this.redirectURIs;
}
public A withRedirectURIs(List redirectURIs){
this.redirectURIs.clear();
if (redirectURIs != null) {for (String item : redirectURIs){this.addToRedirectURIs(item);}} return (A) this;
}
public A withRedirectURIs(String... redirectURIs){
this.redirectURIs.clear(); if (redirectURIs != null) {for (String item :redirectURIs){ this.addToRedirectURIs(item);}} return (A) this;
}
public Boolean isRespondWithChallenges(){
return this.respondWithChallenges;
}
public A withRespondWithChallenges(Boolean respondWithChallenges){
this.respondWithChallenges=respondWithChallenges; return (A) this;
}
public A addToScopeRestrictions(ScopeRestriction... items){
for (ScopeRestriction item : items) {ScopeRestrictionBuilder builder = new ScopeRestrictionBuilder(item);_visitables.add(builder);this.scopeRestrictions.add(builder);} return (A)this;
}
public A removeFromScopeRestrictions(ScopeRestriction... items){
for (ScopeRestriction item : items) {ScopeRestrictionBuilder builder = new ScopeRestrictionBuilder(item);_visitables.remove(builder);this.scopeRestrictions.remove(builder);} return (A)this;
}
public List getScopeRestrictions(){
return build(scopeRestrictions);
}
public A withScopeRestrictions(List scopeRestrictions){
this.scopeRestrictions.clear();
if (scopeRestrictions != null) {for (ScopeRestriction item : scopeRestrictions){this.addToScopeRestrictions(item);}} return (A) this;
}
public A withScopeRestrictions(ScopeRestriction... scopeRestrictions){
this.scopeRestrictions.clear(); if (scopeRestrictions != null) {for (ScopeRestriction item :scopeRestrictions){ this.addToScopeRestrictions(item);}} return (A) this;
}
public OAuthClientFluent.ScopeRestrictionsNested addNewScopeRestriction(){
return new ScopeRestrictionsNestedImpl();
}
public OAuthClientFluent.ScopeRestrictionsNested addNewScopeRestrictionLike(ScopeRestriction item){
return new ScopeRestrictionsNestedImpl(item);
}
public String getSecret(){
return this.secret;
}
public A withSecret(String secret){
this.secret=secret; return (A) this;
}
public boolean equals(Object o){
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
if (!super.equals(o)) return false;
OAuthClientFluentImpl that = (OAuthClientFluentImpl) o;
if (additionalSecrets != null ? !additionalSecrets.equals(that.additionalSecrets) :that.additionalSecrets != null) return false;
if (apiVersion != null ? !apiVersion.equals(that.apiVersion) :that.apiVersion != null) return false;
if (grantMethod != null ? !grantMethod.equals(that.grantMethod) :that.grantMethod != null) return false;
if (kind != null ? !kind.equals(that.kind) :that.kind != null) return false;
if (metadata != null ? !metadata.equals(that.metadata) :that.metadata != null) return false;
if (redirectURIs != null ? !redirectURIs.equals(that.redirectURIs) :that.redirectURIs != null) return false;
if (respondWithChallenges != null ? !respondWithChallenges.equals(that.respondWithChallenges) :that.respondWithChallenges != null) return false;
if (scopeRestrictions != null ? !scopeRestrictions.equals(that.scopeRestrictions) :that.scopeRestrictions != null) return false;
if (secret != null ? !secret.equals(that.secret) :that.secret != null) return false;
return true;
}
public class MetadataNestedImpl extends ObjectMetaFluentImpl> implements OAuthClientFluent.MetadataNested,Nested{
private final ObjectMetaBuilder builder;
MetadataNestedImpl(){
this.builder = new ObjectMetaBuilder(this);
}
MetadataNestedImpl(ObjectMeta item){
this.builder = new ObjectMetaBuilder(this, item);
}
public N endMetadata(){
return and();
}
public N and(){
return (N) OAuthClientFluentImpl.this.withMetadata(builder.build());
}
}
public class ScopeRestrictionsNestedImpl extends ScopeRestrictionFluentImpl> implements OAuthClientFluent.ScopeRestrictionsNested,Nested{
private final ScopeRestrictionBuilder builder;
ScopeRestrictionsNestedImpl(){
this.builder = new ScopeRestrictionBuilder(this);
}
ScopeRestrictionsNestedImpl(ScopeRestriction item){
this.builder = new ScopeRestrictionBuilder(this, item);
}
public N endScopeRestriction(){
return and();
}
public N and(){
return (N) OAuthClientFluentImpl.this.addToScopeRestrictions(builder.build());
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy