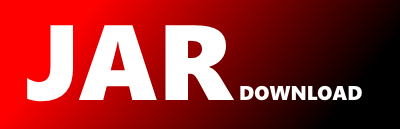
io.fabric8.kubernetes.api.model.ObjectMetaFluentImpl Maven / Gradle / Ivy
package io.fabric8.kubernetes.api.model;
import io.fabric8.kubernetes.api.builder.BaseFluent;
import java.lang.String;
import java.util.Map;
import com.fasterxml.jackson.annotation.JsonProperty;
import javax.validation.Valid;
import java.util.LinkedHashMap;
import java.lang.Long;
import java.util.List;
import java.util.ArrayList;
import javax.validation.constraints.Pattern;
import javax.validation.constraints.Size;
import io.fabric8.kubernetes.api.builder.VisitableBuilder;
import java.lang.Boolean;
import java.lang.Object;
import io.fabric8.kubernetes.api.builder.Nested;
public class ObjectMetaFluentImpl> extends BaseFluent implements ObjectMetaFluent{
private Map annotations = new LinkedHashMap();
private String creationTimestamp;
private Long deletionGracePeriodSeconds;
private String deletionTimestamp;
private List finalizers = new ArrayList();
private String generateName;
private Long generation;
private Map labels = new LinkedHashMap();
private String name;
private String namespace;
private List> ownerReferences = new ArrayList>();
private String resourceVersion;
private String selfLink;
private String uid;
public ObjectMetaFluentImpl(){
}
public ObjectMetaFluentImpl(ObjectMeta instance){
this.withAnnotations(instance.getAnnotations());
this.withCreationTimestamp(instance.getCreationTimestamp());
this.withDeletionGracePeriodSeconds(instance.getDeletionGracePeriodSeconds());
this.withDeletionTimestamp(instance.getDeletionTimestamp());
this.withFinalizers(instance.getFinalizers());
this.withGenerateName(instance.getGenerateName());
this.withGeneration(instance.getGeneration());
this.withLabels(instance.getLabels());
this.withName(instance.getName());
this.withNamespace(instance.getNamespace());
this.withOwnerReferences(instance.getOwnerReferences());
this.withResourceVersion(instance.getResourceVersion());
this.withSelfLink(instance.getSelfLink());
this.withUid(instance.getUid());
}
public A addToAnnotations(String key,String value){
if(key != null && value != null) {this.annotations.put(key, value);} return (A)this;
}
public A addToAnnotations(Map map){
if(map != null) { this.annotations.putAll(map);} return (A)this;
}
public A removeFromAnnotations(String key){
if(key != null) {this.annotations.remove(key);} return (A)this;
}
public A removeFromAnnotations(Map map){
if(map != null) { for(Object key : map.keySet()) {this.annotations.remove(key);}} return (A)this;
}
public Map getAnnotations(){
return this.annotations;
}
public A withAnnotations(Map annotations){
this.annotations.clear();
if (annotations != null) {this.annotations.putAll(annotations);} return (A) this;
}
public String getCreationTimestamp(){
return this.creationTimestamp;
}
public A withCreationTimestamp(String creationTimestamp){
this.creationTimestamp=creationTimestamp; return (A) this;
}
public Long getDeletionGracePeriodSeconds(){
return this.deletionGracePeriodSeconds;
}
public A withDeletionGracePeriodSeconds(Long deletionGracePeriodSeconds){
this.deletionGracePeriodSeconds=deletionGracePeriodSeconds; return (A) this;
}
public String getDeletionTimestamp(){
return this.deletionTimestamp;
}
public A withDeletionTimestamp(String deletionTimestamp){
this.deletionTimestamp=deletionTimestamp; return (A) this;
}
public A addToFinalizers(String... items){
for (String item : items) {this.finalizers.add(item);} return (A)this;
}
public A removeFromFinalizers(String... items){
for (String item : items) {this.finalizers.remove(item);} return (A)this;
}
public List getFinalizers(){
return this.finalizers;
}
public A withFinalizers(List finalizers){
this.finalizers.clear();
if (finalizers != null) {for (String item : finalizers){this.addToFinalizers(item);}} return (A) this;
}
public A withFinalizers(String... finalizers){
this.finalizers.clear(); if (finalizers != null) {for (String item :finalizers){ this.addToFinalizers(item);}} return (A) this;
}
public String getGenerateName(){
return this.generateName;
}
public A withGenerateName(String generateName){
this.generateName=generateName; return (A) this;
}
public Long getGeneration(){
return this.generation;
}
public A withGeneration(Long generation){
this.generation=generation; return (A) this;
}
public A addToLabels(String key,String value){
if(key != null && value != null) {this.labels.put(key, value);} return (A)this;
}
public A addToLabels(Map map){
if(map != null) { this.labels.putAll(map);} return (A)this;
}
public A removeFromLabels(String key){
if(key != null) {this.labels.remove(key);} return (A)this;
}
public A removeFromLabels(Map map){
if(map != null) { for(Object key : map.keySet()) {this.labels.remove(key);}} return (A)this;
}
public Map getLabels(){
return this.labels;
}
public A withLabels(Map labels){
this.labels.clear();
if (labels != null) {this.labels.putAll(labels);} return (A) this;
}
public String getName(){
return this.name;
}
public A withName(String name){
this.name=name; return (A) this;
}
public String getNamespace(){
return this.namespace;
}
public A withNamespace(String namespace){
this.namespace=namespace; return (A) this;
}
public A addToOwnerReferences(OwnerReference... items){
for (OwnerReference item : items) {OwnerReferenceBuilder builder = new OwnerReferenceBuilder(item);_visitables.add(builder);this.ownerReferences.add(builder);} return (A)this;
}
public A removeFromOwnerReferences(OwnerReference... items){
for (OwnerReference item : items) {OwnerReferenceBuilder builder = new OwnerReferenceBuilder(item);_visitables.remove(builder);this.ownerReferences.remove(builder);} return (A)this;
}
public List getOwnerReferences(){
return build(ownerReferences);
}
public A withOwnerReferences(List ownerReferences){
this.ownerReferences.clear();
if (ownerReferences != null) {for (OwnerReference item : ownerReferences){this.addToOwnerReferences(item);}} return (A) this;
}
public A withOwnerReferences(OwnerReference... ownerReferences){
this.ownerReferences.clear(); if (ownerReferences != null) {for (OwnerReference item :ownerReferences){ this.addToOwnerReferences(item);}} return (A) this;
}
public ObjectMetaFluent.OwnerReferencesNested addNewOwnerReference(){
return new OwnerReferencesNestedImpl();
}
public ObjectMetaFluent.OwnerReferencesNested addNewOwnerReferenceLike(OwnerReference item){
return new OwnerReferencesNestedImpl(item);
}
public A addNewOwnerReference(String apiVersion,Boolean controller,String kind,String name,String uid){
return (A)addToOwnerReferences(new OwnerReference(apiVersion, controller, kind, name, uid));
}
public String getResourceVersion(){
return this.resourceVersion;
}
public A withResourceVersion(String resourceVersion){
this.resourceVersion=resourceVersion; return (A) this;
}
public String getSelfLink(){
return this.selfLink;
}
public A withSelfLink(String selfLink){
this.selfLink=selfLink; return (A) this;
}
public String getUid(){
return this.uid;
}
public A withUid(String uid){
this.uid=uid; return (A) this;
}
public boolean equals(Object o){
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
if (!super.equals(o)) return false;
ObjectMetaFluentImpl that = (ObjectMetaFluentImpl) o;
if (annotations != null ? !annotations.equals(that.annotations) :that.annotations != null) return false;
if (creationTimestamp != null ? !creationTimestamp.equals(that.creationTimestamp) :that.creationTimestamp != null) return false;
if (deletionGracePeriodSeconds != null ? !deletionGracePeriodSeconds.equals(that.deletionGracePeriodSeconds) :that.deletionGracePeriodSeconds != null) return false;
if (deletionTimestamp != null ? !deletionTimestamp.equals(that.deletionTimestamp) :that.deletionTimestamp != null) return false;
if (finalizers != null ? !finalizers.equals(that.finalizers) :that.finalizers != null) return false;
if (generateName != null ? !generateName.equals(that.generateName) :that.generateName != null) return false;
if (generation != null ? !generation.equals(that.generation) :that.generation != null) return false;
if (labels != null ? !labels.equals(that.labels) :that.labels != null) return false;
if (name != null ? !name.equals(that.name) :that.name != null) return false;
if (namespace != null ? !namespace.equals(that.namespace) :that.namespace != null) return false;
if (ownerReferences != null ? !ownerReferences.equals(that.ownerReferences) :that.ownerReferences != null) return false;
if (resourceVersion != null ? !resourceVersion.equals(that.resourceVersion) :that.resourceVersion != null) return false;
if (selfLink != null ? !selfLink.equals(that.selfLink) :that.selfLink != null) return false;
if (uid != null ? !uid.equals(that.uid) :that.uid != null) return false;
return true;
}
public class OwnerReferencesNestedImpl extends OwnerReferenceFluentImpl> implements ObjectMetaFluent.OwnerReferencesNested,Nested{
private final OwnerReferenceBuilder builder;
OwnerReferencesNestedImpl(){
this.builder = new OwnerReferenceBuilder(this);
}
OwnerReferencesNestedImpl(OwnerReference item){
this.builder = new OwnerReferenceBuilder(this, item);
}
public N endOwnerReference(){
return and();
}
public N and(){
return (N) ObjectMetaFluentImpl.this.addToOwnerReferences(builder.build());
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy