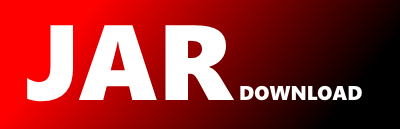
io.fabric8.kubernetes.api.model.PersistentVolumeSpecFluent Maven / Gradle / Ivy
package io.fabric8.kubernetes.api.model;
import io.fabric8.kubernetes.api.builder.Fluent;
import java.lang.String;
import java.util.List;
import com.fasterxml.jackson.annotation.JsonProperty;
import javax.validation.Valid;
import java.util.Map;
import java.lang.Object;
import com.fasterxml.jackson.annotation.JsonIgnore;
import java.lang.Integer;
import java.lang.Boolean;
import io.fabric8.kubernetes.api.builder.Nested;
public interface PersistentVolumeSpecFluent> extends Fluent{
public A addToAccessModes(String... items);
public A removeFromAccessModes(String... items);
public List getAccessModes();
public A withAccessModes(List accessModes);
public A withAccessModes(String... accessModes);
public AWSElasticBlockStoreVolumeSource getAwsElasticBlockStore();
public A withAwsElasticBlockStore(AWSElasticBlockStoreVolumeSource awsElasticBlockStore);
public PersistentVolumeSpecFluent.AwsElasticBlockStoreNested withNewAwsElasticBlockStore();
public PersistentVolumeSpecFluent.AwsElasticBlockStoreNested withNewAwsElasticBlockStoreLike(AWSElasticBlockStoreVolumeSource item);
public PersistentVolumeSpecFluent.AwsElasticBlockStoreNested editAwsElasticBlockStore();
public A withNewAwsElasticBlockStore(String fsType,Integer partition,Boolean readOnly,String volumeID);
public AzureFileVolumeSource getAzureFile();
public A withAzureFile(AzureFileVolumeSource azureFile);
public PersistentVolumeSpecFluent.AzureFileNested withNewAzureFile();
public PersistentVolumeSpecFluent.AzureFileNested withNewAzureFileLike(AzureFileVolumeSource item);
public PersistentVolumeSpecFluent.AzureFileNested editAzureFile();
public A withNewAzureFile(Boolean readOnly,String secretName,String shareName);
public A addToCapacity(String key,Quantity value);
public A addToCapacity(Map map);
public A removeFromCapacity(String key);
public A removeFromCapacity(Map map);
public Map getCapacity();
public A withCapacity(Map capacity);
public CephFSVolumeSource getCephfs();
public A withCephfs(CephFSVolumeSource cephfs);
public PersistentVolumeSpecFluent.CephfsNested withNewCephfs();
public PersistentVolumeSpecFluent.CephfsNested withNewCephfsLike(CephFSVolumeSource item);
public PersistentVolumeSpecFluent.CephfsNested editCephfs();
public CinderVolumeSource getCinder();
public A withCinder(CinderVolumeSource cinder);
public PersistentVolumeSpecFluent.CinderNested withNewCinder();
public PersistentVolumeSpecFluent.CinderNested withNewCinderLike(CinderVolumeSource item);
public PersistentVolumeSpecFluent.CinderNested editCinder();
public A withNewCinder(String fsType,Boolean readOnly,String volumeID);
public ObjectReference getClaimRef();
public A withClaimRef(ObjectReference claimRef);
public PersistentVolumeSpecFluent.ClaimRefNested withNewClaimRef();
public PersistentVolumeSpecFluent.ClaimRefNested withNewClaimRefLike(ObjectReference item);
public PersistentVolumeSpecFluent.ClaimRefNested editClaimRef();
public FCVolumeSource getFc();
public A withFc(FCVolumeSource fc);
public PersistentVolumeSpecFluent.FcNested withNewFc();
public PersistentVolumeSpecFluent.FcNested withNewFcLike(FCVolumeSource item);
public PersistentVolumeSpecFluent.FcNested editFc();
public FlexVolumeSource getFlexVolume();
public A withFlexVolume(FlexVolumeSource flexVolume);
public PersistentVolumeSpecFluent.FlexVolumeNested withNewFlexVolume();
public PersistentVolumeSpecFluent.FlexVolumeNested withNewFlexVolumeLike(FlexVolumeSource item);
public PersistentVolumeSpecFluent.FlexVolumeNested editFlexVolume();
public FlockerVolumeSource getFlocker();
public A withFlocker(FlockerVolumeSource flocker);
public PersistentVolumeSpecFluent.FlockerNested withNewFlocker();
public PersistentVolumeSpecFluent.FlockerNested withNewFlockerLike(FlockerVolumeSource item);
public PersistentVolumeSpecFluent.FlockerNested editFlocker();
public A withNewFlocker(String datasetName);
public GCEPersistentDiskVolumeSource getGcePersistentDisk();
public A withGcePersistentDisk(GCEPersistentDiskVolumeSource gcePersistentDisk);
public PersistentVolumeSpecFluent.GcePersistentDiskNested withNewGcePersistentDisk();
public PersistentVolumeSpecFluent.GcePersistentDiskNested withNewGcePersistentDiskLike(GCEPersistentDiskVolumeSource item);
public PersistentVolumeSpecFluent.GcePersistentDiskNested editGcePersistentDisk();
public A withNewGcePersistentDisk(String fsType,Integer partition,String pdName,Boolean readOnly);
public GlusterfsVolumeSource getGlusterfs();
public A withGlusterfs(GlusterfsVolumeSource glusterfs);
public PersistentVolumeSpecFluent.GlusterfsNested withNewGlusterfs();
public PersistentVolumeSpecFluent.GlusterfsNested withNewGlusterfsLike(GlusterfsVolumeSource item);
public PersistentVolumeSpecFluent.GlusterfsNested editGlusterfs();
public A withNewGlusterfs(String endpoints,String path,Boolean readOnly);
public HostPathVolumeSource getHostPath();
public A withHostPath(HostPathVolumeSource hostPath);
public PersistentVolumeSpecFluent.HostPathNested withNewHostPath();
public PersistentVolumeSpecFluent.HostPathNested withNewHostPathLike(HostPathVolumeSource item);
public PersistentVolumeSpecFluent.HostPathNested editHostPath();
public A withNewHostPath(String path);
public ISCSIVolumeSource getIscsi();
public A withIscsi(ISCSIVolumeSource iscsi);
public PersistentVolumeSpecFluent.IscsiNested withNewIscsi();
public PersistentVolumeSpecFluent.IscsiNested withNewIscsiLike(ISCSIVolumeSource item);
public PersistentVolumeSpecFluent.IscsiNested editIscsi();
public NFSVolumeSource getNfs();
public A withNfs(NFSVolumeSource nfs);
public PersistentVolumeSpecFluent.NfsNested withNewNfs();
public PersistentVolumeSpecFluent.NfsNested withNewNfsLike(NFSVolumeSource item);
public PersistentVolumeSpecFluent.NfsNested editNfs();
public A withNewNfs(String path,Boolean readOnly,String server);
public String getPersistentVolumeReclaimPolicy();
public A withPersistentVolumeReclaimPolicy(String persistentVolumeReclaimPolicy);
public RBDVolumeSource getRbd();
public A withRbd(RBDVolumeSource rbd);
public PersistentVolumeSpecFluent.RbdNested withNewRbd();
public PersistentVolumeSpecFluent.RbdNested withNewRbdLike(RBDVolumeSource item);
public PersistentVolumeSpecFluent.RbdNested editRbd();
public VsphereVirtualDiskVolumeSource getVsphereVolume();
public A withVsphereVolume(VsphereVirtualDiskVolumeSource vsphereVolume);
public PersistentVolumeSpecFluent.VsphereVolumeNested withNewVsphereVolume();
public PersistentVolumeSpecFluent.VsphereVolumeNested withNewVsphereVolumeLike(VsphereVirtualDiskVolumeSource item);
public PersistentVolumeSpecFluent.VsphereVolumeNested editVsphereVolume();
public A withNewVsphereVolume(String fsType,String volumePath);
public interface AwsElasticBlockStoreNested extends Nested,AWSElasticBlockStoreVolumeSourceFluent>{
public N endAwsElasticBlockStore(); public N and();
}
public interface AzureFileNested extends Nested,AzureFileVolumeSourceFluent>{
public N endAzureFile(); public N and();
}
public interface CephfsNested extends Nested,CephFSVolumeSourceFluent>{
public N endCephfs(); public N and();
}
public interface CinderNested extends Nested,CinderVolumeSourceFluent>{
public N endCinder(); public N and();
}
public interface ClaimRefNested extends Nested,ObjectReferenceFluent>{
public N endClaimRef(); public N and();
}
public interface FcNested extends Nested,FCVolumeSourceFluent>{
public N endFc(); public N and();
}
public interface FlexVolumeNested extends Nested,FlexVolumeSourceFluent>{
public N endFlexVolume(); public N and();
}
public interface FlockerNested extends Nested,FlockerVolumeSourceFluent>{
public N endFlocker(); public N and();
}
public interface GcePersistentDiskNested extends Nested,GCEPersistentDiskVolumeSourceFluent>{
public N endGcePersistentDisk(); public N and();
}
public interface GlusterfsNested extends Nested,GlusterfsVolumeSourceFluent>{
public N endGlusterfs(); public N and();
}
public interface HostPathNested extends Nested,HostPathVolumeSourceFluent>{
public N endHostPath(); public N and();
}
public interface IscsiNested extends Nested,ISCSIVolumeSourceFluent>{
public N endIscsi(); public N and();
}
public interface NfsNested extends Nested,NFSVolumeSourceFluent>{
public N endNfs(); public N and();
}
public interface RbdNested extends Nested,RBDVolumeSourceFluent>{
public N endRbd(); public N and();
}
public interface VsphereVolumeNested extends Nested,VsphereVirtualDiskVolumeSourceFluent>{
public N endVsphereVolume(); public N and();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy