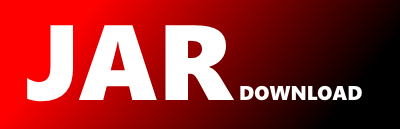
io.fabric8.openshift.api.model.BuildStatusFluentImpl Maven / Gradle / Ivy
package io.fabric8.openshift.api.model;
import io.fabric8.kubernetes.api.builder.BaseFluent;
import java.lang.Boolean;
import com.fasterxml.jackson.annotation.JsonProperty;
import java.lang.String;
import io.fabric8.kubernetes.api.builder.VisitableBuilder;
import javax.validation.Valid;
import java.lang.Long;
import io.fabric8.kubernetes.api.model.ObjectReference;
import java.lang.Object;
import io.fabric8.kubernetes.api.builder.Nested;
import io.fabric8.kubernetes.api.model.ObjectReferenceFluentImpl;
import io.fabric8.kubernetes.api.model.ObjectReferenceBuilder;
public class BuildStatusFluentImpl> extends BaseFluent implements BuildStatusFluent{
private Boolean cancelled;
private String completionTimestamp;
private VisitableBuilder extends ObjectReference,?> config;
private Long duration;
private String message;
private String outputDockerImageReference;
private String phase;
private String reason;
private String startTimestamp;
public BuildStatusFluentImpl(){
}
public BuildStatusFluentImpl(BuildStatus instance){
this.withCancelled(instance.getCancelled());
this.withCompletionTimestamp(instance.getCompletionTimestamp());
this.withConfig(instance.getConfig());
this.withDuration(instance.getDuration());
this.withMessage(instance.getMessage());
this.withOutputDockerImageReference(instance.getOutputDockerImageReference());
this.withPhase(instance.getPhase());
this.withReason(instance.getReason());
this.withStartTimestamp(instance.getStartTimestamp());
}
public Boolean isCancelled(){
return this.cancelled;
}
public A withCancelled(Boolean cancelled){
this.cancelled=cancelled; return (A) this;
}
public String getCompletionTimestamp(){
return this.completionTimestamp;
}
public A withCompletionTimestamp(String completionTimestamp){
this.completionTimestamp=completionTimestamp; return (A) this;
}
public ObjectReference getConfig(){
return this.config!=null?this.config.build():null;
}
public A withConfig(ObjectReference config){
if (config!=null){ this.config= new ObjectReferenceBuilder(config); _visitables.add(this.config);} return (A) this;
}
public BuildStatusFluent.ConfigNested withNewConfig(){
return new ConfigNestedImpl();
}
public BuildStatusFluent.ConfigNested withNewConfigLike(ObjectReference item){
return new ConfigNestedImpl(item);
}
public BuildStatusFluent.ConfigNested editConfig(){
return withNewConfigLike(getConfig());
}
public Long getDuration(){
return this.duration;
}
public A withDuration(Long duration){
this.duration=duration; return (A) this;
}
public String getMessage(){
return this.message;
}
public A withMessage(String message){
this.message=message; return (A) this;
}
public String getOutputDockerImageReference(){
return this.outputDockerImageReference;
}
public A withOutputDockerImageReference(String outputDockerImageReference){
this.outputDockerImageReference=outputDockerImageReference; return (A) this;
}
public String getPhase(){
return this.phase;
}
public A withPhase(String phase){
this.phase=phase; return (A) this;
}
public String getReason(){
return this.reason;
}
public A withReason(String reason){
this.reason=reason; return (A) this;
}
public String getStartTimestamp(){
return this.startTimestamp;
}
public A withStartTimestamp(String startTimestamp){
this.startTimestamp=startTimestamp; return (A) this;
}
public boolean equals(Object o){
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
if (!super.equals(o)) return false;
BuildStatusFluentImpl that = (BuildStatusFluentImpl) o;
if (cancelled != null ? !cancelled.equals(that.cancelled) :that.cancelled != null) return false;
if (completionTimestamp != null ? !completionTimestamp.equals(that.completionTimestamp) :that.completionTimestamp != null) return false;
if (config != null ? !config.equals(that.config) :that.config != null) return false;
if (duration != null ? !duration.equals(that.duration) :that.duration != null) return false;
if (message != null ? !message.equals(that.message) :that.message != null) return false;
if (outputDockerImageReference != null ? !outputDockerImageReference.equals(that.outputDockerImageReference) :that.outputDockerImageReference != null) return false;
if (phase != null ? !phase.equals(that.phase) :that.phase != null) return false;
if (reason != null ? !reason.equals(that.reason) :that.reason != null) return false;
if (startTimestamp != null ? !startTimestamp.equals(that.startTimestamp) :that.startTimestamp != null) return false;
return true;
}
public class ConfigNestedImpl extends ObjectReferenceFluentImpl> implements BuildStatusFluent.ConfigNested,Nested{
private final ObjectReferenceBuilder builder;
ConfigNestedImpl(){
this.builder = new ObjectReferenceBuilder(this);
}
ConfigNestedImpl(ObjectReference item){
this.builder = new ObjectReferenceBuilder(this, item);
}
public N endConfig(){
return and();
}
public N and(){
return (N) BuildStatusFluentImpl.this.withConfig(builder.build());
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy