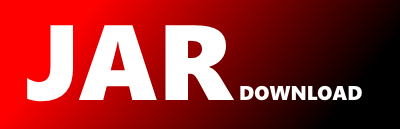
io.fabric8.openshift.api.model.ExecNewPodHookFluentImpl Maven / Gradle / Ivy
package io.fabric8.openshift.api.model;
import io.fabric8.kubernetes.api.builder.BaseFluent;
import java.lang.String;
import java.util.List;
import com.fasterxml.jackson.annotation.JsonProperty;
import javax.validation.Valid;
import java.util.ArrayList;
import io.fabric8.kubernetes.api.builder.VisitableBuilder;
import io.fabric8.kubernetes.api.model.EnvVar;
import java.lang.Object;
import io.fabric8.kubernetes.api.builder.Nested;
import io.fabric8.kubernetes.api.model.EnvVarFluentImpl;
import io.fabric8.kubernetes.api.model.EnvVarBuilder;
public class ExecNewPodHookFluentImpl> extends BaseFluent implements ExecNewPodHookFluent{
private List command = new ArrayList();
private String containerName;
private List> env = new ArrayList>();
private List volumes = new ArrayList();
public ExecNewPodHookFluentImpl(){
}
public ExecNewPodHookFluentImpl(ExecNewPodHook instance){
this.withCommand(instance.getCommand());
this.withContainerName(instance.getContainerName());
this.withEnv(instance.getEnv());
this.withVolumes(instance.getVolumes());
}
public A addToCommand(String... items){
for (String item : items) {this.command.add(item);} return (A)this;
}
public A removeFromCommand(String... items){
for (String item : items) {this.command.remove(item);} return (A)this;
}
public List getCommand(){
return this.command;
}
public A withCommand(List command){
this.command.clear();
if (command != null) {for (String item : command){this.addToCommand(item);}} return (A) this;
}
public A withCommand(String... command){
this.command.clear(); if (command != null) {for (String item :command){ this.addToCommand(item);}} return (A) this;
}
public String getContainerName(){
return this.containerName;
}
public A withContainerName(String containerName){
this.containerName=containerName; return (A) this;
}
public A addToEnv(EnvVar... items){
for (EnvVar item : items) {EnvVarBuilder builder = new EnvVarBuilder(item);_visitables.add(builder);this.env.add(builder);} return (A)this;
}
public A removeFromEnv(EnvVar... items){
for (EnvVar item : items) {EnvVarBuilder builder = new EnvVarBuilder(item);_visitables.remove(builder);this.env.remove(builder);} return (A)this;
}
public List getEnv(){
return build(env);
}
public A withEnv(List env){
this.env.clear();
if (env != null) {for (EnvVar item : env){this.addToEnv(item);}} return (A) this;
}
public A withEnv(EnvVar... env){
this.env.clear(); if (env != null) {for (EnvVar item :env){ this.addToEnv(item);}} return (A) this;
}
public ExecNewPodHookFluent.EnvNested addNewEnv(){
return new EnvNestedImpl();
}
public ExecNewPodHookFluent.EnvNested addNewEnvLike(EnvVar item){
return new EnvNestedImpl(item);
}
public A addToVolumes(String... items){
for (String item : items) {this.volumes.add(item);} return (A)this;
}
public A removeFromVolumes(String... items){
for (String item : items) {this.volumes.remove(item);} return (A)this;
}
public List getVolumes(){
return this.volumes;
}
public A withVolumes(List volumes){
this.volumes.clear();
if (volumes != null) {for (String item : volumes){this.addToVolumes(item);}} return (A) this;
}
public A withVolumes(String... volumes){
this.volumes.clear(); if (volumes != null) {for (String item :volumes){ this.addToVolumes(item);}} return (A) this;
}
public boolean equals(Object o){
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
if (!super.equals(o)) return false;
ExecNewPodHookFluentImpl that = (ExecNewPodHookFluentImpl) o;
if (command != null ? !command.equals(that.command) :that.command != null) return false;
if (containerName != null ? !containerName.equals(that.containerName) :that.containerName != null) return false;
if (env != null ? !env.equals(that.env) :that.env != null) return false;
if (volumes != null ? !volumes.equals(that.volumes) :that.volumes != null) return false;
return true;
}
public class EnvNestedImpl extends EnvVarFluentImpl> implements ExecNewPodHookFluent.EnvNested,Nested{
private final EnvVarBuilder builder;
EnvNestedImpl(){
this.builder = new EnvVarBuilder(this);
}
EnvNestedImpl(EnvVar item){
this.builder = new EnvVarBuilder(this, item);
}
public N endEnv(){
return and();
}
public N and(){
return (N) ExecNewPodHookFluentImpl.this.addToEnv(builder.build());
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy