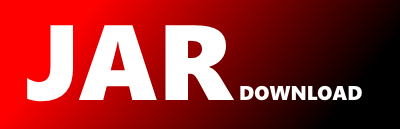
io.fabric8.openshift.api.model.RouteSpecFluentImpl Maven / Gradle / Ivy
The newest version!
package io.fabric8.openshift.api.model;
import io.fabric8.kubernetes.api.builder.BaseFluent;
import io.fabric8.kubernetes.api.builder.VisitableBuilder;
import java.util.List;
import com.fasterxml.jackson.annotation.JsonProperty;
import javax.validation.Valid;
import java.util.ArrayList;
import java.lang.String;
import java.lang.Integer;
import java.lang.Object;
import io.fabric8.kubernetes.api.builder.Nested;
public class RouteSpecFluentImpl> extends BaseFluent implements RouteSpecFluent{
private List> alternateBackends = new ArrayList>();
private String host;
private String path;
private VisitableBuilder extends RoutePort,?> port;
private VisitableBuilder extends TLSConfig,?> tls;
private VisitableBuilder extends RouteTargetReference,?> to;
public RouteSpecFluentImpl(){
}
public RouteSpecFluentImpl(RouteSpec instance){
this.withAlternateBackends(instance.getAlternateBackends());
this.withHost(instance.getHost());
this.withPath(instance.getPath());
this.withPort(instance.getPort());
this.withTls(instance.getTls());
this.withTo(instance.getTo());
}
public A addToAlternateBackends(RouteTargetReference... items){
for (RouteTargetReference item : items) {RouteTargetReferenceBuilder builder = new RouteTargetReferenceBuilder(item);_visitables.add(builder);this.alternateBackends.add(builder);} return (A)this;
}
public A removeFromAlternateBackends(RouteTargetReference... items){
for (RouteTargetReference item : items) {RouteTargetReferenceBuilder builder = new RouteTargetReferenceBuilder(item);_visitables.remove(builder);this.alternateBackends.remove(builder);} return (A)this;
}
public List getAlternateBackends(){
return build(alternateBackends);
}
public A withAlternateBackends(List alternateBackends){
this.alternateBackends.clear();
if (alternateBackends != null) {for (RouteTargetReference item : alternateBackends){this.addToAlternateBackends(item);}} return (A) this;
}
public A withAlternateBackends(RouteTargetReference... alternateBackends){
this.alternateBackends.clear(); if (alternateBackends != null) {for (RouteTargetReference item :alternateBackends){ this.addToAlternateBackends(item);}} return (A) this;
}
public RouteSpecFluent.AlternateBackendsNested addNewAlternateBackend(){
return new AlternateBackendsNestedImpl();
}
public RouteSpecFluent.AlternateBackendsNested addNewAlternateBackendLike(RouteTargetReference item){
return new AlternateBackendsNestedImpl(item);
}
public A addNewAlternateBackend(String kind,String name,Integer weight){
return (A)addToAlternateBackends(new RouteTargetReference(kind, name, weight));
}
public String getHost(){
return this.host;
}
public A withHost(String host){
this.host=host; return (A) this;
}
public String getPath(){
return this.path;
}
public A withPath(String path){
this.path=path; return (A) this;
}
public RoutePort getPort(){
return this.port!=null?this.port.build():null;
}
public A withPort(RoutePort port){
if (port!=null){ this.port= new RoutePortBuilder(port); _visitables.add(this.port);} return (A) this;
}
public RouteSpecFluent.PortNested withNewPort(){
return new PortNestedImpl();
}
public RouteSpecFluent.PortNested withNewPortLike(RoutePort item){
return new PortNestedImpl(item);
}
public RouteSpecFluent.PortNested editPort(){
return withNewPortLike(getPort());
}
public TLSConfig getTls(){
return this.tls!=null?this.tls.build():null;
}
public A withTls(TLSConfig tls){
if (tls!=null){ this.tls= new TLSConfigBuilder(tls); _visitables.add(this.tls);} return (A) this;
}
public RouteSpecFluent.TlsNested withNewTls(){
return new TlsNestedImpl();
}
public RouteSpecFluent.TlsNested withNewTlsLike(TLSConfig item){
return new TlsNestedImpl(item);
}
public RouteSpecFluent.TlsNested editTls(){
return withNewTlsLike(getTls());
}
public RouteTargetReference getTo(){
return this.to!=null?this.to.build():null;
}
public A withTo(RouteTargetReference to){
if (to!=null){ this.to= new RouteTargetReferenceBuilder(to); _visitables.add(this.to);} return (A) this;
}
public RouteSpecFluent.ToNested withNewTo(){
return new ToNestedImpl();
}
public RouteSpecFluent.ToNested withNewToLike(RouteTargetReference item){
return new ToNestedImpl(item);
}
public RouteSpecFluent.ToNested editTo(){
return withNewToLike(getTo());
}
public A withNewTo(String kind,String name,Integer weight){
return (A)withTo(new RouteTargetReference(kind, name, weight));
}
public boolean equals(Object o){
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
if (!super.equals(o)) return false;
RouteSpecFluentImpl that = (RouteSpecFluentImpl) o;
if (alternateBackends != null ? !alternateBackends.equals(that.alternateBackends) :that.alternateBackends != null) return false;
if (host != null ? !host.equals(that.host) :that.host != null) return false;
if (path != null ? !path.equals(that.path) :that.path != null) return false;
if (port != null ? !port.equals(that.port) :that.port != null) return false;
if (tls != null ? !tls.equals(that.tls) :that.tls != null) return false;
if (to != null ? !to.equals(that.to) :that.to != null) return false;
return true;
}
public class AlternateBackendsNestedImpl extends RouteTargetReferenceFluentImpl> implements RouteSpecFluent.AlternateBackendsNested,Nested{
private final RouteTargetReferenceBuilder builder;
AlternateBackendsNestedImpl(RouteTargetReference item){
this.builder = new RouteTargetReferenceBuilder(this, item);
}
AlternateBackendsNestedImpl(){
this.builder = new RouteTargetReferenceBuilder(this);
}
public N endAlternateBackend(){
return and();
}
public N and(){
return (N) RouteSpecFluentImpl.this.addToAlternateBackends(builder.build());
}
}
public class PortNestedImpl extends RoutePortFluentImpl> implements RouteSpecFluent.PortNested,Nested{
private final RoutePortBuilder builder;
PortNestedImpl(){
this.builder = new RoutePortBuilder(this);
}
PortNestedImpl(RoutePort item){
this.builder = new RoutePortBuilder(this, item);
}
public N endPort(){
return and();
}
public N and(){
return (N) RouteSpecFluentImpl.this.withPort(builder.build());
}
}
public class TlsNestedImpl extends TLSConfigFluentImpl> implements RouteSpecFluent.TlsNested,Nested{
private final TLSConfigBuilder builder;
TlsNestedImpl(){
this.builder = new TLSConfigBuilder(this);
}
TlsNestedImpl(TLSConfig item){
this.builder = new TLSConfigBuilder(this, item);
}
public N endTls(){
return and();
}
public N and(){
return (N) RouteSpecFluentImpl.this.withTls(builder.build());
}
}
public class ToNestedImpl extends RouteTargetReferenceFluentImpl> implements RouteSpecFluent.ToNested,Nested{
private final RouteTargetReferenceBuilder builder;
ToNestedImpl(RouteTargetReference item){
this.builder = new RouteTargetReferenceBuilder(this, item);
}
ToNestedImpl(){
this.builder = new RouteTargetReferenceBuilder(this);
}
public N endTo(){
return and();
}
public N and(){
return (N) RouteSpecFluentImpl.this.withTo(builder.build());
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy