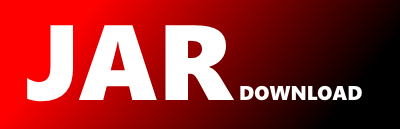
org.dominokit.domino.ui.utils.DominoElement Maven / Gradle / Ivy
/*
* Copyright © 2019 Dominokit
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.dominokit.domino.ui.utils;
import static elemental2.dom.DomGlobal.document;
import elemental2.dom.HTMLBodyElement;
import elemental2.dom.HTMLDivElement;
import elemental2.dom.HTMLElement;
import elemental2.dom.HTMLPictureElement;
import jsinterop.base.Js;
import org.jboss.elemento.Elements;
import org.jboss.elemento.IsElement;
/**
* A class that can wrap any HTMLElement as domino component
*
* @param the type of the wrapped element
*/
public class DominoElement extends BaseDominoElement> {
private final E wrappedElement;
/**
* @param element the {@link HTMLElement} E to wrap as a DominoElement
* @param extends from {@link HTMLElement}
* @return the {@link DominoElement} wrapping the provided element
*/
public static DominoElement of(E element) {
return new DominoElement<>(element);
}
/**
* @param element the {@link IsElement} E to wrap as a DominoElement
* @param extends from {@link HTMLElement}
* @return the {@link DominoElement} wrapping the provided element
*/
public static DominoElement of(IsElement element) {
return new DominoElement<>(element.element());
}
/** @return a {@link DominoElement} wrapping the document {@link HTMLBodyElement} */
public static DominoElement body() {
return new DominoElement<>(document.body);
}
/** @return a new {@link HTMLDivElement} wrapped as a {@link DominoElement} */
public static DominoElement div() {
return DominoElement.of(Elements.div());
}
/** @return a new {@link HTMLDivElement} wrapped as a {@link DominoElement} */
public static DominoElement picture() {
return DominoElement.of(
Js.uncheckedCast(document.createElement("picture")));
}
/** @param element the E element extending from {@link HTMLElement} */
public DominoElement(E element) {
this.wrappedElement = element;
init(this);
}
/**
* @return the E element that is extending from {@link HTMLElement} wrapped in this DominoElement
*/
@Override
public E element() {
return wrappedElement;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy