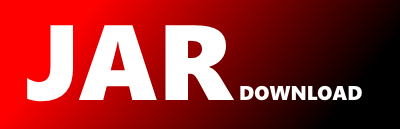
org.dominokit.domino.ui.menu.direction.DropDirection Maven / Gradle / Ivy
/*
* Copyright © 2019 Dominokit
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.dominokit.domino.ui.menu.direction;
import elemental2.dom.Element;
import elemental2.dom.Event;
import org.dominokit.domino.ui.style.CssClass;
/** DropDirection interface. */
public interface DropDirection {
/**
* init.
*
* @param event a {@link elemental2.dom.Event} object
* @return a {@link org.dominokit.domino.ui.menu.direction.DropDirection} object
*/
default DropDirection init(Event event) {
return this;
}
/**
* position.
*
* @param source a {@link elemental2.dom.Element} object
* @param target a {@link elemental2.dom.Element} object
*/
void position(Element source, Element target);
/**
* cleanup.
*
* @param source a {@link elemental2.dom.Element} object
*/
default void cleanup(Element source) {}
/** Constant BEST_FIT_SIDE
*/
DropDirection BEST_FIT_SIDE = new BestFitSideDropDirection();
/** Constant BEST_MIDDLE_SIDE
*/
DropDirection BEST_MIDDLE_SIDE = new BestMiddleSideDropDirection();
/** Constant BEST_MIDDLE_UP_DOWN
*/
DropDirection BEST_MIDDLE_UP_DOWN = new BestMiddleUpDownDropDirection();
/** Constant BEST_MIDDLE_DOWN_UP
*/
DropDirection BEST_MIDDLE_DOWN_UP = new BestMiddleDownUpDropDirection();
/** Constant BEST_SIDE_UP_DOWN
*/
DropDirection BEST_SIDE_UP_DOWN = new BestSideUpDownDropDirection();
/** Constant BOTTOM_LEFT
*/
DropDirection BOTTOM_LEFT = new BottomLeftDropDirection();
/** Constant BOTTOM_MIDDLE
*/
DropDirection BOTTOM_MIDDLE = new BottomMiddleDropDirection();
/** Constant BOTTOM_RIGHT
*/
DropDirection BOTTOM_RIGHT = new BottomRightDropDirection();
/** Constant LEFT_DOWN
*/
DropDirection LEFT_DOWN = new LeftDownDropDirection();
/** Constant LEFT_MIDDLE
*/
DropDirection LEFT_MIDDLE = new LeftMiddleDropDirection();
/** Constant LEFT_UP
*/
DropDirection LEFT_UP = new LeftUpDropDirection();
/** Constant MIDDLE_SCREEN
*/
DropDirection MIDDLE_SCREEN = new MiddleOfScreenDropDirection();
/** Constant BEST_MOUSE_FIT
*/
DropDirection BEST_MOUSE_FIT = new MouseBestFitDirection();
/** Constant RIGHT_DOWN
*/
DropDirection RIGHT_DOWN = new RightDownDropDirection();
/** Constant RIGHT_MIDDLE
*/
DropDirection RIGHT_MIDDLE = new RightMiddleDropDirection();
/** Constant RIGHT_UP
*/
DropDirection RIGHT_UP = new RightUpDropDirection();
/** Constant TOP_LEFT
*/
DropDirection TOP_LEFT = new TopLeftDropDirection();
/** Constant TOP_MIDDLE
*/
DropDirection TOP_MIDDLE = new TopMiddleDropDirection();
/** Constant TOP_RIGHT
*/
DropDirection TOP_RIGHT = new TopRightDropDirection();
/** Constant dui_dd_bottom_left
*/
CssClass dui_dd_bottom_left = () -> "dui-dd-bottom-left";
/** Constant dui_dd_bottom_middle
*/
CssClass dui_dd_bottom_middle = () -> "dui-dd-bottom-middle";
/** Constant dui_dd_bottom_right
*/
CssClass dui_dd_bottom_right = () -> "dui-dd-bottom-right";
/** Constant dui_dd_left_down
*/
CssClass dui_dd_left_down = () -> "dui-dd-left-down";
/** Constant dui_dd_left_middle
*/
CssClass dui_dd_left_middle = () -> "dui-dd-left-middle";
/** Constant dui_dd_left_up
*/
CssClass dui_dd_left_up = () -> "dui-dd-left-up";
/** Constant dui_dd_middle_screen
*/
CssClass dui_dd_middle_screen = () -> "dui-dd-middle-screen";
/** Constant dui_dd_best_mouse_fit
*/
CssClass dui_dd_best_mouse_fit = () -> "dui-dd-best-mouse-fit";
/** Constant dui_dd_right_down
*/
CssClass dui_dd_right_down = () -> "dui-dd-right-down";
/** Constant dui_dd_right_middle
*/
CssClass dui_dd_right_middle = () -> "dui-dd-right-middle";
/** Constant dui_dd_right_up
*/
CssClass dui_dd_right_up = () -> "dui-dd-right-up";
/** Constant dui_dd_top_left
*/
CssClass dui_dd_top_left = () -> "dui-dd-top-left";
/** Constant dui_dd_top_middle
*/
CssClass dui_dd_top_middle = () -> "dui-dd-top-middle";
/** Constant dui_dd_top_right
*/
CssClass dui_dd_top_right = () -> "dui-dd-top-right";
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy