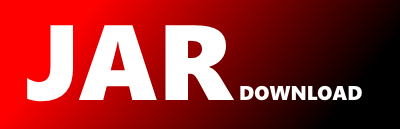
org.dominokit.domino.ui.utils.DomElements Maven / Gradle / Ivy
/*
* Copyright © 2019 Dominokit
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.dominokit.domino.ui.utils;
import static elemental2.dom.DomGlobal.document;
import static jsinterop.base.Js.cast;
import static org.dominokit.domino.ui.utils.Domino.*;
import elemental2.dom.*;
import elemental2.svg.SVGCircleElement;
import elemental2.svg.SVGElement;
import elemental2.svg.SVGLineElement;
/**
* The {@code DomElements} interface provides factory methods for creating various HTML and SVG
* elements as well as accessing commonly used HTML elements from the DOM.
*
* Example Usage:
*
*
* // Create an anchor element
* HTMLAnchorElement anchorElement = DomElements.dom.a();
*
* // Create an input element with a specific type
* HTMLInputElement inputElement = DomElements.dom.input(InputType.TEXT);
*
* // Access the document body element
* HTMLBodyElement bodyElement = DomElements.dom.body();
*
*/
public interface DomElements {
/** The XML namespace for SVG elements. */
String SVGNS = "http://www.w3.org/2000/svg";
/** An instance of the {@code DomElements} interface for convenience. */
DomElements dom = new DomElements() {};
/**
* Creates an HTML element of the specified type.
*
* @param element The name of the HTML element.
* @param type The class representing the HTML element.
* @return An instance of the created HTML element.
*/
default E create(String element, Class type) {
return cast(document.createElement(element));
}
/**
* Creates an SVG element of the specified type.
*
* @param element The name of the SVG element.
* @param type The class representing the SVG element.
* @return An instance of the created SVG element.
*/
default E createSVG(String element, Class type) {
return cast(document.createElementNS(SVGNS, element));
}
/**
* Accesses the document's body element.
*
* @return The HTML body element.
*/
default HTMLBodyElement body() {
return document.body;
}
/**
* Creates a {@code } element.
*
* @return The created picture element.
*/
default HTMLPictureElement picture() {
return create("picture", HTMLPictureElement.class);
}
/**
* Creates an {@code } element.
*
* @return The created address element.
*/
default HTMLElement address() {
return create("address", HTMLElement.class);
}
/**
* Creates an {@code } element.
*
* @return The created article element.
*/
default HTMLElement article() {
return create("article", HTMLElement.class);
}
/**
* Creates an {@code