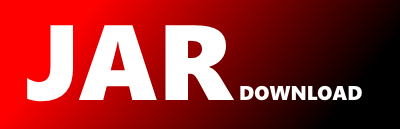
org.dominokit.domino.ui.utils.ElementsFactory Maven / Gradle / Ivy
/*
* Copyright © 2019 Dominokit
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.dominokit.domino.ui.utils;
import static org.dominokit.domino.ui.utils.Domino.*;
import elemental2.dom.Element;
import elemental2.dom.Text;
import elemental2.svg.SVGElement;
import java.util.Optional;
import org.dominokit.domino.ui.IsElement;
import org.dominokit.domino.ui.elements.*;
import org.dominokit.domino.ui.elements.svg.CircleElement;
import org.dominokit.domino.ui.elements.svg.LineElement;
/**
* A factory interface for creating various HTML elements in a fluent manner.
*
* Example usage:
*
*
java BodyElement body = ElementsFactory.elements.body(); DivElement div =
* ElementsFactory.elements.div(); AnchorElement anchor = ElementsFactory.elements.a();
*/
public interface ElementsFactory {
/** A default instance of the ElementsFactory interface. */
ElementsFactory elements = new ElementsFactory() {};
/**
* Provides access to the delegate that implements the ElementsFactory interface.
*
* @return An instance of ElementsFactoryDelegate.
*/
default ElementsFactoryDelegate delegate() {
return DominoUIConfig.CONFIG.getElementsFactory();
}
/**
* Retrieves an element by its unique identifier.
*
* @param id The unique identifier of the element to retrieve.
* @return An optional containing the element, or empty if not found.
*/
default Optional> byId(String id) {
return delegate().byId(id);
}
/**
* Creates a new HTML element of the specified type.
*
* @param element The HTML element name (e.g., "div", "span").
* @param type The Java class representing the element type.
* @param The element type.
* @return A new instance of the specified element type.
*/
default E create(String element, Class type) {
return delegate().create(element, type);
}
/**
* Wraps an existing element in a DominoElement.
*
* @param element The existing HTML element to wrap.
* @param The element type.
* @return A DominoElement wrapping the existing element.
*/
default DominoElement elementOf(E element) {
return delegate().elementOf(element);
}
/**
* Creates a DominoElement of the specified IsElement type.
*
* @param element The IsElement to wrap.
* @param The element type.
* @param The IsElement type.
* @return A DominoElement wrapping the IsElement.
*/
default > DominoElement elementOf(E element) {
return delegate().elementOf(element);
}
/**
* Generates a unique identifier.
*
* @return A unique identifier.
*/
default String getUniqueId() {
return delegate().getUniqueId();
}
/**
* Generates a unique identifier with the specified prefix.
*
* @param prefix The prefix to use in the generated identifier.
* @return A unique identifier with the specified prefix.
*/
default String getUniqueId(String prefix) {
return delegate().getUniqueId(prefix);
}
/**
* Creates a <body> element.
*
* @return A new BodyElement.
*/
default BodyElement body() {
return delegate().body();
}
/**
* Creates a <picture> element.
*
* @return A new PictureElement.
*/
default PictureElement picture() {
return delegate().picture();
}
/**
* Creates an <address> element.
*
* @return A new AddressElement.
*/
default AddressElement address() {
return delegate().address();
}
/**
* Creates an <article> element.
*
* @return A new ArticleElement.
*/
default ArticleElement article() {
return delegate().article();
}
/**
* Creates an <aside> element.
*
* @return A new AsideElement.
*/
default AsideElement aside() {
return delegate().aside();
}
/**
* Creates a <footer> element.
*
* @return A new FooterElement.
*/
default FooterElement footer() {
return delegate().footer();
}
/**
* Creates a <h> (heading) element with the specified level.
*
* @param n The heading level (e.g., 1 for <h1>, 2 for <h2>, etc.).
* @return A new HeadingElement with the specified level.
*/
default HeadingElement h(int n) {
return delegate().h(n);
}
/**
* Creates a <header> element.
*
* @return A new HeaderElement.
*/
default HeaderElement header() {
return delegate().header();
}
/**
* Creates an <hgroup> element.
*
* @return A new HGroupElement.
*/
default HGroupElement hgroup() {
return delegate().hgroup();
}
/**
* Creates a <nav> element.
*
* @return A new NavElement.
*/
default NavElement nav() {
return delegate().nav();
}
/**
* Creates a <section> element.
*
* @return A new SectionElement.
*/
default SectionElement section() {
return delegate().section();
}
/**
* Creates a <blockquote> element.
*
* @return A new BlockquoteElement.
*/
default BlockquoteElement blockquote() {
return delegate().blockquote();
}
/**
* Creates a <dd> element.
*
* @return A new DDElement.
*/
default DDElement dd() {
return delegate().dd();
}
/**
* Creates a <div> element.
*
* @return A new DivElement.
*/
default DivElement div() {
return delegate().div();
}
/**
* Creates a <dl> (definition list) element.
*
* @return A new DListElement.
*/
default DListElement dl() {
return delegate().dl();
}
/**
* Creates a <dt> (definition term) element.
*
* @return A new DTElement.
*/
default DTElement dt() {
return delegate().dt();
}
/**
* Creates a <figcaption> element.
*
* @return A new FigCaptionElement.
*/
default FigCaptionElement figcaption() {
return delegate().figcaption();
}
/**
* Creates a <figure> element.
*
* @return A new FigureElement.
*/
default FigureElement figure() {
return delegate().figure();
}
/**
* Creates a <hr> (horizontal rule) element.
*
* @return A new HRElement.
*/
default HRElement hr() {
return delegate().hr();
}
/**
* Creates a <li> (list item) element.
*
* @return A new LIElement.
*/
default LIElement li() {
return delegate().li();
}
/**
* Creates a <main> element.
*
* @return A new MainElement.
*/
@SuppressWarnings("all")
default MainElement main() {
return delegate().main();
}
/**
* Creates an <ol> (ordered list) element.
*
* @return A new OListElement.
*/
default OListElement ol() {
return delegate().ol();
}
/**
* Creates a <p> (paragraph) element.
*
* @return A new ParagraphElement.
*/
default ParagraphElement p() {
return delegate().p();
}
/**
* Creates a <p> (paragraph) element with the specified text content.
*
* @param text The text content to set in the paragraph.
* @return A new ParagraphElement with the specified text content.
*/
default ParagraphElement p(String text) {
return delegate().p(text);
}
/**
* Creates a <pre> (preformatted text) element.
*
* @return A new PreElement.
*/
default PreElement pre() {
return delegate().pre();
}
/**
* Creates a <ul> (unordered list) element.
*
* @return A new UListElement.
*/
default UListElement ul() {
return delegate().ul();
}
/**
* Creates an <a> (anchor) element.
*
* @return A new AnchorElement.
*/
default AnchorElement a() {
return delegate().a();
}
/**
* Creates an <a> (anchor) element with the specified href.
*
* @param href The URL to set in the anchor's href attribute.
* @return A new AnchorElement with the specified href.
*/
default AnchorElement a(String href) {
return delegate().a(href);
}
/**
* Creates an <a> (anchor) element with the specified href and target.
*
* @param href The URL to set in the anchor's href attribute.
* @param target The target attribute value to set.
* @return A new AnchorElement with the specified href and target.
*/
default AnchorElement a(String href, String target) {
return delegate().a(href, target);
}
/**
* Creates an <abbr> element.
*
* @return A new ABBRElement.
*/
default ABBRElement abbr() {
return delegate().abbr();
}
/**
* Creates a <b> (bold) element.
*
* @return A new BElement.
*/
default BElement b() {
return delegate().b();
}
/**
* Creates a <br> (line break) element.
*
* @return A new BRElement.
*/
default BRElement br() {
return delegate().br();
}
/**
* Creates a <cite> element.
*
* @return A new CiteElement.
*/
default CiteElement cite() {
return delegate().cite();
}
/**
* Creates a <code> element.
*
* @return A new CodeElement.
*/
default CodeElement code() {
return delegate().code();
}
/**
* Creates a <dfn> element.
*
* @return A new DFNElement.
*/
default DFNElement dfn() {
return delegate().dfn();
}
/**
* Creates an <em> (emphasis) element.
*
* @return A new EMElement.
*/
default EMElement em() {
return delegate().em();
}
/**
* Creates an <i> (italic) element.
*
* @return A new IElement.
*/
default IElement i() {
return delegate().i();
}
/**
* Creates a <kbd> (keyboard input) element.
*
* @return A new KBDElement.
*/
default KBDElement kbd() {
return delegate().kbd();
}
/**
* Creates a <mark> element.
*
* @return A new MarkElement.
*/
default MarkElement mark() {
return delegate().mark();
}
/**
* Creates a <q> (quotation) element.
*
* @return A new QuoteElement.
*/
default QuoteElement q() {
return delegate().q();
}
/**
* Creates a <small> element.
*
* @return A new SmallElement.
*/
default SmallElement small() {
return delegate().small();
}
/**
* Creates a <span> element.
*
* @return A new SpanElement.
*/
default SpanElement span() {
return delegate().span();
}
/**
* Creates a <strong> (strong emphasis) element.
*
* @return A new StrongElement.
*/
default StrongElement strong() {
return delegate().strong();
}
/**
* Creates a <sub> (subscript) element.
*
* @return A new SubElement.
*/
default SubElement sub() {
return delegate().sub();
}
/**
* Creates a <sup> (superscript) element.
*
* @return A new SupElement.
*/
default SupElement sup() {
return delegate().sup();
}
/**
* Creates a <time> element.
*
* @return A new TimeElement.
*/
default TimeElement time() {
return delegate().time();
}
/**
* Creates a <u> (underline) element.
*
* @return A new UElement.
*/
default UElement u() {
return delegate().u();
}
/**
* Creates a <var> (variable) element.
*
* @return A new VarElement.
*/
default VarElement var() {
return delegate().var();
}
/**
* Creates a <wbr> (word break opportunity) element.
*
* @return A new WBRElement.
*/
default WBRElement wbr() {
return delegate().wbr();
}
/**
* Creates an <area> element.
*
* @return A new AreaElement.
*/
default AreaElement area() {
return delegate().area();
}
/**
* Creates an <audio> element.
*
* @return A new AudioElement.
*/
default AudioElement audio() {
return delegate().audio();
}
/**
* Creates an <img> (image) element.
*
* @return A new ImageElement.
*/
default ImageElement img() {
return delegate().img();
}
/**
* Creates an <img> (image) element with the specified source URL.
*
* @param src The URL of the image source.
* @return A new ImageElement with the specified source URL.
*/
default ImageElement img(String src) {
return delegate().img(src);
}
/**
* Creates a <map> element.
*
* @return A new MapElement.
*/
default MapElement map() {
return delegate().map();
}
/**
* Creates a <track> element.
*
* @return A new TrackElement.
*/
default TrackElement track() {
return delegate().track();
}
/**
* Creates a <video> element.
*
* @return A new VideoElement.
*/
default VideoElement video() {
return delegate().video();
}
/**
* Creates a <canvas> element.
*
* @return A new CanvasElement.
*/
default CanvasElement canvas() {
return delegate().canvas();
}
/**
* Creates an <embed> element.
*
* @return A new EmbedElement.
*/
default EmbedElement embed() {
return delegate().embed();
}
/**
* Creates an <iframe> element.
*
* @return A new IFrameElement.
*/
default IFrameElement iframe() {
return delegate().iframe();
}
/**
* Creates an <iframe> element with the specified source URL.
*
* @param src The URL of the iframe source.
* @return A new IFrameElement with the specified source URL.
*/
default IFrameElement iframe(String src) {
return delegate().iframe(src);
}
/**
* Creates an <object> element.
*
* @return A new ObjectElement.
*/
default ObjectElement object() {
return delegate().object();
}
/**
* Creates a <param> element.
*
* @return A new ParamElement.
*/
default ParamElement param() {
return delegate().param();
}
/**
* Creates a <source> element.
*
* @return A new SourceElement.
*/
default SourceElement source() {
return delegate().source();
}
/**
* Creates a <noscript> element.
*
* @return A new NoScriptElement.
*/
default NoScriptElement noscript() {
return delegate().noscript();
}
/**
* Creates a <script> element.
*
* @return A new ScriptElement.
*/
default ScriptElement script() {
return delegate().script();
}
/**
* Creates a <del> (deleted text) element.
*
* @return A new DelElement.
*/
default DelElement del() {
return delegate().del();
}
/**
* Creates an <ins> (inserted text) element.
*
* @return A new InsElement.
*/
default InsElement ins() {
return delegate().ins();
}
/**
* Creates a <caption> (table caption) element.
*
* @return A new TableCaptionElement.
*/
default TableCaptionElement caption() {
return delegate().caption();
}
/**
* Creates a <col> element.
*
* @return A new ColElement.
*/
default ColElement col() {
return delegate().col();
}
/**
* Creates a <colgroup> element.
*
* @return A new ColGroupElement.
*/
default ColGroupElement colgroup() {
return delegate().colgroup();
}
/**
* Creates a <table> element.
*
* @return A new TableElement.
*/
default TableElement table() {
return delegate().table();
}
/**
* Creates a <tbody> element.
*
* @return A new TBodyElement.
*/
default TBodyElement tbody() {
return delegate().tbody();
}
/**
* Creates a <td> (table cell) element.
*
* @return A new TDElement.
*/
default TDElement td() {
return delegate().td();
}
/**
* Creates a <tfoot> element.
*
* @return A new TFootElement.
*/
default TFootElement tfoot() {
return delegate().tfoot();
}
/**
* Creates a <th> (table header cell) element.
*
* @return A new THElement.
*/
default THElement th() {
return delegate().th();
}
/**
* Creates a <thead> element.
*
* @return A new THeadElement.
*/
default THeadElement thead() {
return delegate().thead();
}
/**
* Creates a <tr> (table row) element.
*
* @return A new TableRowElement.
*/
default TableRowElement tr() {
return delegate().tr();
}
/**
* Creates a <button> element.
*
* @return A new ButtonElement.
*/
default ButtonElement button() {
return delegate().button();
}
/**
* Creates a <datalist> element.
*
* @return A new DataListElement.
*/
default DataListElement datalist() {
return delegate().datalist();
}
/**
* Creates a <fieldset> element.
*
* @return A new FieldSetElement.
*/
default FieldSetElement fieldset() {
return delegate().fieldset();
}
/**
* Creates a <form> element.
*
* @return A new FormElement.
*/
default FormElement form() {
return delegate().form();
}
/**
* Creates an <input> element with the specified input type.
*
* @param type The input type (e.g., "text", "checkbox", "radio", etc.).
* @return A new InputElement with the specified input type.
*/
default InputElement input(InputType type) {
return delegate().input(type);
}
/**
* Creates an <input> element with the specified input type.
*
* @param type The input type (e.g., "text", "checkbox", "radio", etc.).
* @return A new InputElement with the specified input type.
*/
default InputElement input(String type) {
return delegate().input(type);
}
/**
* Creates a <label> element.
*
* @return A new LabelElement.
*/
default LabelElement label() {
return delegate().label();
}
/**
* Creates a <label> element with the specified text.
*
* @param text The text content to set in the label.
* @return A new LabelElement with the specified text content.
*/
default LabelElement label(String text) {
return delegate().label(text);
}
/**
* Creates a <legend> element.
*
* @return A new LegendElement.
*/
default LegendElement legend() {
return delegate().legend();
}
/**
* Creates a <meter> element.
*
* @return A new MeterElement.
*/
default MeterElement meter() {
return delegate().meter();
}
/**
* Creates an <optgroup> element.
*
* @return A new OptGroupElement.
*/
default OptGroupElement optgroup() {
return delegate().optgroup();
}
/**
* Creates an <option> element.
*
* @return A new OptionElement.
*/
default OptionElement option() {
return delegate().option();
}
/**
* Creates an <output> element.
*
* @return A new OutputElement.
*/
default OutputElement output() {
return delegate().output();
}
/**
* Creates a <progress> element.
*
* @return A new ProgressElement.
*/
default ProgressElement progress() {
return delegate().progress();
}
/**
* Creates a <select> element.
*
* @return A new SelectElement.
*/
default SelectElement select_() {
return delegate().select_();
}
/**
* Creates a <textarea> element.
*
* @return A new TextAreaElement.
*/
default TextAreaElement textarea() {
return delegate().textarea();
}
/**
* Creates an <svg> element.
*
* @return A new SvgElement.
*/
default SvgElement svg() {
return delegate().svg();
}
/**
* Creates an <svg> element.
*
* @param The actual type of the svg element being created
* @param tag The string tag name for the svg element.
* @param type The concrete type for the svg element
* @return A new SvgElement of the specified type.
*/
default T svg(String tag, Class type) {
return delegate().svg(tag, type);
}
/**
* Creates a <circle> element with the specified attributes.
*
* @param cx The x-coordinate of the center of the circle.
* @param cy The y-coordinate of the center of the circle.
* @param r The radius of the circle.
* @return A new CircleElement with the specified attributes.
*/
default CircleElement circle(double cx, double cy, double r) {
return delegate().circle(cx, cy, r);
}
/**
* Creates a <line> element with the specified attributes.
*
* @param x1 The x-coordinate of the start point of the line.
* @param y1 The y-coordinate of the start point of the line.
* @param x2 The x-coordinate of the end point of the line.
* @param y2 The y-coordinate of the end point of the line.
* @return A new LineElement with the specified attributes.
*/
default LineElement line(double x1, double y1, double x2, double y2) {
return delegate().line(x1, y1, x2, y2);
}
/**
* Creates a <text> element.
*
* @return A new Text element.
*/
default Text text() {
return delegate().text();
}
/**
* Creates a <text> element with the specified content.
*
* @param content The text content to set in the text element.
* @return A new Text element with the specified content.
*/
default Text text(String content) {
return delegate().text(content);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy