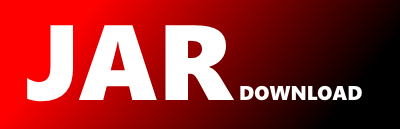
org.drizzle.jdbc.CommonDatabaseMetaData Maven / Gradle / Ivy
Show all versions of drizzle-jdbc Show documentation
/*
* Drizzle-JDBC
*
* Copyright (c) 2009-2011, Marcus Eriksson
*
* All rights reserved.
*
* Redistribution and use in source and binary forms, with or without modification, are permitted provided that the following
* conditions are met:
*
* Redistributions of source code must retain the above copyright notice, this list of conditions and the following disclaimer.
* Redistributions in binary form must reproduce the above copyright notice, this list of conditions and the following
* disclaimer in the documentation and/or other materials provided with the distribution.
* Neither the name of the driver nor the names of its contributors may be used to endorse or promote products derived
* from this software without specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING,
* BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO
* EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR
* CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR
* PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR
* TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
*/
package org.drizzle.jdbc;
import org.drizzle.jdbc.internal.common.SupportedDatabases;
import org.drizzle.jdbc.internal.common.Utils;
import org.drizzle.jdbc.internal.SQLExceptionMapper;
import java.sql.Connection;
import java.sql.DatabaseMetaData;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.sql.Statement;
import java.sql.Types;
import java.util.logging.Logger;
/**
* TODO: complete it! User: marcuse Date: Jan 31, 2009 Time: 8:59:57 PM
*/
public abstract class CommonDatabaseMetaData implements DatabaseMetaData {
private final String version;
private final String url;
private final String username;
private final Connection connection;
private final static Logger log = Logger.getLogger(CommonDatabaseMetaData.class.getName());
private final String databaseProductName;
/**
* Retrieves whether the current user can call all the procedures returned by the method
* getProcedures
.
*
* @return true
if so; false
otherwise
* @throws java.sql.SQLException if a database access error occurs
*/
public boolean allProceduresAreCallable() throws SQLException {
return false;
}
/**
* Retrieves whether the current user can use all the tables returned by the method getTables
in a
* SELECT
statement.
*
* @return true
if so; false
otherwise
* @throws java.sql.SQLException if a database access error occurs
*/
public boolean allTablesAreSelectable() throws SQLException {
return true;
}
/**
* Retrieves the URL for this DBMS.
*
* @return the URL for this DBMS or null
if it cannot be generated
* @throws java.sql.SQLException if a database access error occurs
*/
public String getURL() throws SQLException {
return url;
}
/**
* Retrieves the user name as known to this database.
*
* @return the database user name
* @throws java.sql.SQLException if a database access error occurs
*/
public String getUserName() throws SQLException {
return username;
}
/**
* Retrieves whether this database is in read-only mode.
*
* @return true
if so; false
otherwise
* @throws java.sql.SQLException if a database access error occurs
*/
public boolean isReadOnly() throws SQLException {
return false;
}
/**
* Retrieves whether NULL
values are sorted high. Sorted high means that NULL
values sort
* higher than any other value in a domain. In an ascending order, if this method returns true
,
* NULL
values will appear at the end. By contrast, the method nullsAreSortedAtEnd
* indicates whether NULL
values are sorted at the end regardless of sort order.
*
* @return true
if so; false
otherwise
* @throws java.sql.SQLException if a database access error occurs
*/
public boolean nullsAreSortedHigh() throws SQLException {
return false;
}
/**
* Retrieves whether NULL
values are sorted low. Sorted low means that NULL
values sort
* lower than any other value in a domain. In an ascending order, if this method returns true
,
* NULL
values will appear at the beginning. By contrast, the method nullsAreSortedAtStart
* indicates whether NULL
values are sorted at the beginning regardless of sort order.
*
* @return true
if so; false
otherwise
* @throws java.sql.SQLException if a database access error occurs
*/
public boolean nullsAreSortedLow() throws SQLException {
return !nullsAreSortedHigh();
}
/**
* Retrieves whether NULL
values are sorted at the start regardless of sort order.
*
* @return true
if so; false
otherwise
* @throws java.sql.SQLException if a database access error occurs
*/
public boolean nullsAreSortedAtStart() throws SQLException {
return false;
}
/**
* Retrieves whether NULL
values are sorted at the end regardless of sort order.
*
* @return true
if so; false
otherwise
* @throws java.sql.SQLException if a database access error occurs
*/
public boolean nullsAreSortedAtEnd() throws SQLException {
return !nullsAreSortedAtStart();
}
/**
* Retrieves the name of this database product.
*
* @return database product name
* @throws java.sql.SQLException if a database access error occurs
*/
public String getDatabaseProductName() throws SQLException {
return databaseProductName; // TODO: get from constants file
}
/**
* Retrieves the version number of this database product.
*
* @return database version number
* @throws java.sql.SQLException if a database access error occurs
*/
public String getDatabaseProductVersion() throws SQLException {
return version;
}
/**
* Retrieves the name of this JDBC driver.
*
* @return JDBC driver name
* @throws java.sql.SQLException if a database access error occurs
*/
public String getDriverName() throws SQLException {
return "Drizzle-JDBC"; // TODO: get from constants file
}
/**
* Retrieves the version number of this JDBC driver as a String
.
*
* @return JDBC driver version
* @throws java.sql.SQLException if a database access error occurs
*/
public String getDriverVersion() throws SQLException {
return "0.1"; // TODO: get from constants file
}
/**
* Retrieves this JDBC driver's major version number.
*
* @return JDBC driver major version
*/
public int getDriverMajorVersion() {
return 0;
}
/**
* Retrieves this JDBC driver's minor version number.
*
* @return JDBC driver minor version number
*/
public int getDriverMinorVersion() {
return 3;
}
/**
* Retrieves whether this database stores tables in a local file.
*
* @return true
if so; false
otherwise
* @throws java.sql.SQLException if a database access error occurs
*/
public boolean usesLocalFiles() throws SQLException {
return false;
}
/**
* Retrieves whether this database uses a file for each table.
*
* @return true
if this database uses a local file for each table; false
otherwise
* @throws java.sql.SQLException if a database access error occurs
*/
public boolean usesLocalFilePerTable() throws SQLException {
return false;
}
/**
* Retrieves whether this database treats mixed case unquoted SQL identifiers as case sensitive and as a result
* stores them in mixed case.
*
* @return true
if so; false
otherwise
* @throws java.sql.SQLException if a database access error occurs
*/
public boolean supportsMixedCaseIdentifiers() throws SQLException {
return true;
}
/**
* Retrieves whether this database treats mixed case unquoted SQL identifiers as case insensitive and stores them in
* upper case.
*
* @return true
if so; false
otherwise
* @throws java.sql.SQLException if a database access error occurs
*/
public boolean storesUpperCaseIdentifiers() throws SQLException {
return false;
}
/**
* Retrieves whether this database treats mixed case unquoted SQL identifiers as case insensitive and stores them in
* lower case.
*
* @return true
if so; false
otherwise
* @throws java.sql.SQLException if a database access error occurs
*/
public boolean storesLowerCaseIdentifiers() throws SQLException {
return !storesUpperCaseIdentifiers();
}
/**
* Retrieves whether this database treats mixed case unquoted SQL identifiers as case insensitive and stores them in
* mixed case.
*
* @return true
if so; false
otherwise
* @throws java.sql.SQLException if a database access error occurs
*/
public boolean storesMixedCaseIdentifiers() throws SQLException {
return false;
}
/**
* Retrieves whether this database treats mixed case quoted SQL identifiers as case sensitive and as a result stores
* them in mixed case.
*
* @return true
if so; false
otherwise
* @throws java.sql.SQLException if a database access error occurs
*/
public boolean supportsMixedCaseQuotedIdentifiers() throws SQLException {
return false;
}
/**
* Retrieves whether this database treats mixed case quoted SQL identifiers as case insensitive and stores them in
* upper case.
*
* @return true
if so; false
otherwise
* @throws java.sql.SQLException if a database access error occurs
*/
public boolean storesUpperCaseQuotedIdentifiers() throws SQLException {
return !storesMixedCaseQuotedIdentifiers();
}
/**
* Retrieves whether this database treats mixed case quoted SQL identifiers as case insensitive and stores them in
* lower case.
*
* @return true
if so; false
otherwise
* @throws java.sql.SQLException if a database access error occurs
*/
public boolean storesLowerCaseQuotedIdentifiers() throws SQLException {
return false;
}
/**
* Retrieves whether this database treats mixed case quoted SQL identifiers as case insensitive and stores them in
* mixed case.
*
* @return true
if so; false
otherwise
* @throws java.sql.SQLException if a database access error occurs
*/
public boolean storesMixedCaseQuotedIdentifiers() throws SQLException {
return false;
}
/**
* Retrieves the string used to quote SQL identifiers. This method returns a space " " if identifier quoting is not
* supported.
*
* @return the quoting string or a space if quoting is not supported
* @throws java.sql.SQLException if a database access error occurs
*/
public String getIdentifierQuoteString() throws SQLException {
return "`";
}
/**
* Retrieves a comma-separated list of all of this database's SQL keywords that are NOT also SQL:2003 keywords.
*
* @return the list of this database's keywords that are not also SQL:2003 keywords
* @throws java.sql.SQLException if a database access error occurs
*/
public String getSQLKeywords() throws SQLException {
return "";
}
/**
* Retrieves a comma-separated list of math functions available with this database. These are the Open /Open CLI
* math function names used in the JDBC function escape clause.
*
* @return the list of math functions supported by this database
* @throws java.sql.SQLException if a database access error occurs
*/
public String getNumericFunctions() throws SQLException {
return ""; //todo: add
}
/**
* Retrieves a comma-separated list of string functions available with this database. These are the Open Group CLI
* string function names used in the JDBC function escape clause.
*
* @return the list of string functions supported by this database
* @throws java.sql.SQLException if a database access error occurs
*/
public String getStringFunctions() throws SQLException {
return ""; //Todo: add
}
/**
* Retrieves a comma-separated list of system functions available with this database. These are the Open Group CLI
* system function names used in the JDBC function escape clause.
*
* @return a list of system functions supported by this database
* @throws java.sql.SQLException if a database access error occurs
*/
public String getSystemFunctions() throws SQLException {
return "DATABASE,USER,SYSTEM_USER,SESSION_USER,LAST_INSERT_ID,VERSION";
}
/**
* Retrieves a comma-separated list of the time and date functions available with this database.
*
* @return the list of time and date functions supported by this database
* @throws java.sql.SQLException if a database access error occurs
*/
public String getTimeDateFunctions() throws SQLException {
return "";
}
/**
* Retrieves the string that can be used to escape wildcard characters. This is the string that can be used to
* escape '_' or '%' in the catalog search parameters that are a pattern (and therefore use one of the wildcard
* characters).
*
* The '_' character represents any single character; the '%' character represents any sequence of zero or more
* characters.
*
* @return the string used to escape wildcard characters
* @throws java.sql.SQLException if a database access error occurs
*/
public String getSearchStringEscape() throws SQLException {
return "\\";
}
/**
* Retrieves all the "extra" characters that can be used in unquoted identifier names (those beyond a-z, A-Z, 0-9
* and _).
*
* @return the string containing the extra characters
* @throws java.sql.SQLException if a database access error occurs
*/
public String getExtraNameCharacters() throws SQLException {
return "#@";
}
/**
* Retrieves whether this database supports ALTER TABLE
with add column.
*
* @return true
if so; false
otherwise
* @throws java.sql.SQLException if a database access error occurs
*/
public boolean supportsAlterTableWithAddColumn() throws SQLException {
return true;
}
/**
* Retrieves whether this database supports ALTER TABLE
with drop column.
*
* @return true
if so; false
otherwise
* @throws java.sql.SQLException if a database access error occurs
*/
public boolean supportsAlterTableWithDropColumn() throws SQLException {
return true;
}
/**
* Retrieves whether this database supports column aliasing.
*
* If so, the SQL AS clause can be used to provide names for computed columns or to provide alias names for
* columns as required.
*
* @return true
if so; false
otherwise
* @throws java.sql.SQLException if a database access error occurs
*/
public boolean supportsColumnAliasing() throws SQLException {
return true;
}
/**
* Retrieves whether this database supports concatenations between NULL
and non-NULL
* values being NULL
.
*
* @return true
if so; false
otherwise
* @throws java.sql.SQLException if a database access error occurs
*/
public boolean nullPlusNonNullIsNull() throws SQLException {
return true;
}
/**
* Retrieves whether this database supports the JDBC scalar function CONVERT
for the conversion of one
* JDBC type to another. The JDBC types are the generic SQL data types defined in java.sql.Types
.
*
* @return true
if so; false
otherwise
* @throws java.sql.SQLException if a database access error occurs
*/
public boolean supportsConvert() throws SQLException {
return false; //wrong
}
/**
* Retrieves whether this database supports the JDBC scalar function CONVERT
for conversions between
* the JDBC types fromType and toType. The JDBC types are the generic SQL data types defined in
* java.sql.Types
.
*
* @param fromType the type to convert from; one of the type codes from the class java.sql.Types
* @param toType the type to convert to; one of the type codes from the class java.sql.Types
* @return true
if so; false
otherwise
* @throws java.sql.SQLException if a database access error occurs
* @see java.sql.Types
*/
public boolean supportsConvert(final int fromType, final int toType) throws SQLException {
return false; // TODO: it does...
}
/**
* Retrieves whether this database supports table correlation names.
*
* @return true
if so; false
otherwise
* @throws java.sql.SQLException if a database access error occurs
*/
public boolean supportsTableCorrelationNames() throws SQLException {
return true;
}
/**
* Retrieves whether, when table correlation names are supported, they are restricted to being different from the
* names of the tables.
*
* @return true
if so; false
otherwise
* @throws java.sql.SQLException if a database access error occurs
*/
public boolean supportsDifferentTableCorrelationNames() throws SQLException {
return true;
}
/**
* Retrieves whether this database supports expressions in ORDER BY
lists.
*
* @return true
if so; false
otherwise
* @throws java.sql.SQLException if a database access error occurs
*/
public boolean supportsExpressionsInOrderBy() throws SQLException {
return true;
}
/**
* Retrieves whether this database supports using a column that is not in the SELECT
statement in an
* ORDER BY
clause.
*
* @return true
if so; false
otherwise
* @throws java.sql.SQLException if a database access error occurs
*/
public boolean supportsOrderByUnrelated() throws SQLException {
return false;
}
/**
* Retrieves whether this database supports some form of GROUP BY
clause.
*
* @return true
if so; false
otherwise
* @throws java.sql.SQLException if a database access error occurs
*/
public boolean supportsGroupBy() throws SQLException {
return true;
}
/**
* Retrieves whether this database supports using a column that is not in the SELECT
statement in a
* GROUP BY
clause.
*
* @return true
if so; false
otherwise
* @throws java.sql.SQLException if a database access error occurs
*/
public boolean supportsGroupByUnrelated() throws SQLException {
return true;
}
/**
* Retrieves whether this database supports using columns not included in the SELECT
statement in a
* GROUP BY
clause provided that all of the columns in the SELECT
statement are included
* in the GROUP BY
clause.
*
* @return true
if so; false
otherwise
* @throws java.sql.SQLException if a database access error occurs
*/
public boolean supportsGroupByBeyondSelect() throws SQLException {
return true;
}
/**
* Retrieves whether this database supports specifying a LIKE
escape clause.
*
* @return true
if so; false
otherwise
* @throws java.sql.SQLException if a database access error occurs
*/
public boolean supportsLikeEscapeClause() throws SQLException {
return true;
}
/**
* Retrieves whether this database supports getting multiple ResultSet
objects from a single call to
* the method execute
.
*
* @return true
if so; false
otherwise
* @throws java.sql.SQLException if a database access error occurs
*/
public boolean supportsMultipleResultSets() throws SQLException {
return false;
}
/**
* Retrieves whether this database allows having multiple transactions open at once (on different connections).
*
* @return true
if so; false
otherwise
* @throws java.sql.SQLException if a database access error occurs
*/
public boolean supportsMultipleTransactions() throws SQLException {
return true;
}
/**
* Retrieves whether columns in this database may be defined as non-nullable.
*
* @return true
if so; false
otherwise
* @throws java.sql.SQLException if a database access error occurs
*/
public boolean supportsNonNullableColumns() throws SQLException {
return true;
}
/**
* Retrieves whether this database supports the ODBC Minimum SQL grammar.
*
* @return true
if so; false
otherwise
* @throws java.sql.SQLException if a database access error occurs
*/
public boolean supportsMinimumSQLGrammar() throws SQLException {
return true;
}
/**
* Retrieves whether this database supports the ODBC Core SQL grammar.
*
* @return true
if so; false
otherwise
* @throws java.sql.SQLException if a database access error occurs
*/
public boolean supportsCoreSQLGrammar() throws SQLException {
return true;
}
/**
* Retrieves whether this database supports the ODBC Extended SQL grammar.
*
* @return true
if so; false
otherwise
* @throws java.sql.SQLException if a database access error occurs
*/
public boolean supportsExtendedSQLGrammar() throws SQLException {
return false;
}
/**
* Retrieves whether this database supports the ANSI92 entry level SQL grammar.
*
* @return true
if so; false
otherwise
* @throws java.sql.SQLException if a database access error occurs
*/
public boolean supportsANSI92EntryLevelSQL() throws SQLException {
return true;
}
/**
* Retrieves whether this database supports the ANSI92 intermediate SQL grammar supported.
*
* @return true
if so; false
otherwise
* @throws java.sql.SQLException if a database access error occurs
*/
public boolean supportsANSI92IntermediateSQL() throws SQLException {
return false;
}
/**
* Retrieves whether this database supports the ANSI92 full SQL grammar supported.
*
* @return true
if so; false
otherwise
* @throws java.sql.SQLException if a database access error occurs
*/
public boolean supportsANSI92FullSQL() throws SQLException {
return false;
}
/**
* Retrieves whether this database supports the SQL Integrity Enhancement Facility.
*
* @return true
if so; false
otherwise
* @throws java.sql.SQLException if a database access error occurs
*/
public boolean supportsIntegrityEnhancementFacility() throws SQLException {
return false; //Todo: verify
}
/**
* Retrieves whether this database supports some form of outer join.
*
* @return true
if so; false
otherwise
* @throws java.sql.SQLException if a database access error occurs
*/
public boolean supportsOuterJoins() throws SQLException {
return true;
}
/**
* Retrieves whether this database supports full nested outer joins.
*
* @return true
if so; false
otherwise
* @throws java.sql.SQLException if a database access error occurs
*/
public boolean supportsFullOuterJoins() throws SQLException {
return false;
}
/**
* Retrieves whether this database provides limited support for outer joins. (This will be true
if the
* method supportsFullOuterJoins
returns true
).
*
* @return true
if so; false
otherwise
* @throws java.sql.SQLException if a database access error occurs
*/
public boolean supportsLimitedOuterJoins() throws SQLException {
return true;
}
/**
* Retrieves the database vendor's preferred term for "schema".
*
* @return the vendor term for "schema"
* @throws java.sql.SQLException if a database access error occurs
*/
public String getSchemaTerm() throws SQLException {
return "database";
}
/**
* Retrieves the database vendor's preferred term for "procedure".
*
* @return the vendor term for "procedure"
* @throws java.sql.SQLException if a database access error occurs
*/
public String getProcedureTerm() throws SQLException {
return "";
}
/**
* Retrieves the database vendor's preferred term for "catalog".
*
* @return the vendor term for "catalog"
* @throws java.sql.SQLException if a database access error occurs
*/
public String getCatalogTerm() throws SQLException {
return "";
}
/**
* Retrieves whether a catalog appears at the start of a fully qualified table name. If not, the catalog appears at
* the end.
*
* @return true
if the catalog name appears at the beginning of a fully qualified table name;
* false
otherwise
* @throws java.sql.SQLException if a database access error occurs
*/
public boolean isCatalogAtStart() throws SQLException {
return true;
}
/**
* Retrieves the String
that this database uses as the separator between a catalog and table name.
*
* @return the separator string
* @throws java.sql.SQLException if a database access error occurs
*/
public String getCatalogSeparator() throws SQLException {
return ".";
}
/**
* Retrieves whether a schema name can be used in a data manipulation statement.
*
* @return true
if so; false
otherwise
* @throws java.sql.SQLException if a database access error occurs
*/
public boolean supportsSchemasInDataManipulation() throws SQLException {
return true;
}
/**
* Retrieves whether a schema name can be used in a procedure call statement.
*
* @return true
if so; false
otherwise
* @throws java.sql.SQLException if a database access error occurs
*/
public boolean supportsSchemasInProcedureCalls() throws SQLException {
return false;
}
/**
* Retrieves whether a schema name can be used in a table definition statement.
*
* @return true
if so; false
otherwise
* @throws java.sql.SQLException if a database access error occurs
*/
public boolean supportsSchemasInTableDefinitions() throws SQLException {
return true;
}
/**
* Retrieves whether a schema name can be used in an index definition statement.
*
* @return true
if so; false
otherwise
* @throws java.sql.SQLException if a database access error occurs
*/
public boolean supportsSchemasInIndexDefinitions() throws SQLException {
return true;
}
/**
* Retrieves whether a schema name can be used in a privilege definition statement.
*
* @return true
if so; false
otherwise
* @throws java.sql.SQLException if a database access error occurs
*/
public boolean supportsSchemasInPrivilegeDefinitions() throws SQLException {
return true;
}
/**
* Retrieves whether a catalog name can be used in a data manipulation statement.
*
* @return true
if so; false
otherwise
* @throws java.sql.SQLException if a database access error occurs
*/
public boolean supportsCatalogsInDataManipulation() throws SQLException {
return false;
}
/**
* Retrieves whether a catalog name can be used in a procedure call statement.
*
* @return true
if so; false
otherwise
* @throws java.sql.SQLException if a database access error occurs
*/
public boolean supportsCatalogsInProcedureCalls() throws SQLException {
return false;
}
/**
* Retrieves whether a catalog name can be used in a table definition statement.
*
* @return true
if so; false
otherwise
* @throws java.sql.SQLException if a database access error occurs
*/
public boolean supportsCatalogsInTableDefinitions() throws SQLException {
return false;
}
/**
* Retrieves whether a catalog name can be used in an index definition statement.
*
* @return true
if so; false
otherwise
* @throws java.sql.SQLException if a database access error occurs
*/
public boolean supportsCatalogsInIndexDefinitions() throws SQLException {
return false;
}
/**
* Retrieves whether a catalog name can be used in a privilege definition statement.
*
* @return true
if so; false
otherwise
* @throws java.sql.SQLException if a database access error occurs
*/
public boolean supportsCatalogsInPrivilegeDefinitions() throws SQLException {
return false;
}
/**
* Retrieves whether this database supports positioned DELETE
statements.
*
* @return true
if so; false
otherwise
* @throws java.sql.SQLException if a database access error occurs
*/
public boolean supportsPositionedDelete() throws SQLException {
return false;
}
/**
* Retrieves whether this database supports positioned UPDATE
statements.
*
* @return true
if so; false
otherwise
* @throws java.sql.SQLException if a database access error occurs
*/
public boolean supportsPositionedUpdate() throws SQLException {
return false;
}
/**
* Retrieves whether this database supports SELECT FOR UPDATE
statements.
*
* @return true
if so; false
otherwise
* @throws java.sql.SQLException if a database access error occurs
*/
public boolean supportsSelectForUpdate() throws SQLException {
return true;
}
/**
* Retrieves whether this database supports stored procedure calls that use the stored procedure escape syntax.
*
* @return true
if so; false
otherwise
* @throws java.sql.SQLException if a database access error occurs
*/
public boolean supportsStoredProcedures() throws SQLException {
return false;
}
/**
* Retrieves whether this database supports subqueries in comparison expressions.
*
* @return true
if so; false
otherwise
* @throws java.sql.SQLException if a database access error occurs
*/
public boolean supportsSubqueriesInComparisons() throws SQLException {
return true;
}
/**
* Retrieves whether this database supports subqueries in EXISTS
expressions.
*
* @return true
if so; false
otherwise
* @throws java.sql.SQLException if a database access error occurs
*/
public boolean supportsSubqueriesInExists() throws SQLException {
return true;
}
/**
* Retrieves whether this database supports subqueries in IN
expressions.
*
* @return true
if so; false
otherwise
* @throws java.sql.SQLException if a database access error occurs
*/
public boolean supportsSubqueriesInIns() throws SQLException {
return true;
}
/**
* Retrieves whether this database supports subqueries in quantified expressions.
*
* @return true
if so; false
otherwise
* @throws java.sql.SQLException if a database access error occurs
*/
public boolean supportsSubqueriesInQuantifieds() throws SQLException {
return true;
}
/**
* Retrieves whether this database supports correlated subqueries.
*
* @return true
if so; false
otherwise
* @throws java.sql.SQLException if a database access error occurs
*/
public boolean supportsCorrelatedSubqueries() throws SQLException {
return true;
}
/**
* Retrieves whether this database supports SQL UNION
.
*
* @return true
if so; false
otherwise
* @throws java.sql.SQLException if a database access error occurs
*/
public boolean supportsUnion() throws SQLException {
return true;
}
/**
* Retrieves whether this database supports SQL UNION ALL
.
*
* @return true
if so; false
otherwise
* @throws java.sql.SQLException if a database access error occurs
*/
public boolean supportsUnionAll() throws SQLException {
return true;
}
/**
* Retrieves whether this database supports keeping cursors open across commits.
*
* @return true
if cursors always remain open; false
if they might not remain open
* @throws java.sql.SQLException if a database access error occurs
*/
public boolean supportsOpenCursorsAcrossCommit() throws SQLException {
return true;
}
/**
* Retrieves whether this database supports keeping cursors open across rollbacks.
*
* @return true
if cursors always remain open; false
if they might not remain open
* @throws java.sql.SQLException if a database access error occurs
*/
public boolean supportsOpenCursorsAcrossRollback() throws SQLException {
return true;
}
/**
* Retrieves whether this database supports keeping statements open across commits.
*
* @return true
if statements always remain open; false
if they might not remain open
* @throws java.sql.SQLException if a database access error occurs
*/
public boolean supportsOpenStatementsAcrossCommit() throws SQLException {
return true;
}
/**
* Retrieves whether this database supports keeping statements open across rollbacks.
*
* @return true
if statements always remain open; false
if they might not remain open
* @throws java.sql.SQLException if a database access error occurs
*/
public boolean supportsOpenStatementsAcrossRollback() throws SQLException {
return true;
}
/**
* Retrieves the maximum number of hex characters this database allows in an inline binary literal.
*
* @return max the maximum length (in hex characters) for a binary literal; a result of zero means that there is no
* limit or the limit is not known
* @throws java.sql.SQLException if a database access error occurs
*/
public int getMaxBinaryLiteralLength() throws SQLException {
return 16777208;
}
/**
* Retrieves the maximum number of characters this database allows for a character literal.
*
* @return the maximum number of characters allowed for a character literal; a result of zero means that there is no
* limit or the limit is not known
* @throws java.sql.SQLException if a database access error occurs
*/
public int getMaxCharLiteralLength() throws SQLException {
return 16777208;
}
/**
* Retrieves the maximum number of characters this database allows for a column name.
*
* @return the maximum number of characters allowed for a column name; a result of zero means that there is no limit
* or the limit is not known
* @throws java.sql.SQLException if a database access error occurs
*/
public int getMaxColumnNameLength() throws SQLException {
return 64;
}
/**
* Retrieves the maximum number of columns this database allows in a GROUP BY
clause.
*
* @return the maximum number of columns allowed; a result of zero means that there is no limit or the limit is not
* known
* @throws java.sql.SQLException if a database access error occurs
*/
public int getMaxColumnsInGroupBy() throws SQLException {
return 64;
}
/**
* Retrieves the maximum number of columns this database allows in an index.
*
* @return the maximum number of columns allowed; a result of zero means that there is no limit or the limit is not
* known
* @throws java.sql.SQLException if a database access error occurs
*/
public int getMaxColumnsInIndex() throws SQLException {
return 16;
}
/**
* Retrieves the maximum number of columns this database allows in an ORDER BY
clause.
*
* @return the maximum number of columns allowed; a result of zero means that there is no limit or the limit is not
* known
* @throws java.sql.SQLException if a database access error occurs
*/
public int getMaxColumnsInOrderBy() throws SQLException {
return 64;
}
/**
* Retrieves the maximum number of columns this database allows in a SELECT
list.
*
* @return the maximum number of columns allowed; a result of zero means that there is no limit or the limit is not
* known
* @throws java.sql.SQLException if a database access error occurs
*/
public int getMaxColumnsInSelect() throws SQLException {
return 256;
}
/**
* Retrieves the maximum number of columns this database allows in a table.
*
* @return the maximum number of columns allowed; a result of zero means that there is no limit or the limit is not
* known
* @throws java.sql.SQLException if a database access error occurs
*/
public int getMaxColumnsInTable() throws SQLException {
return 0;
}
/**
* Retrieves the maximum number of concurrent connections to this database that are possible.
*
* @return the maximum number of active connections possible at one time; a result of zero means that there is no
* limit or the limit is not known
* @throws java.sql.SQLException if a database access error occurs
*/
public int getMaxConnections() throws SQLException {
return 0;
}
/**
* Retrieves the maximum number of characters that this database allows in a cursor name.
*
* @return the maximum number of characters allowed in a cursor name; a result of zero means that there is no limit
* or the limit is not known
* @throws java.sql.SQLException if a database access error occurs
*/
public int getMaxCursorNameLength() throws SQLException {
return 0;
}
/**
* Retrieves the maximum number of bytes this database allows for an index, including all of the parts of the
* index.
*
* @return the maximum number of bytes allowed; this limit includes the composite of all the constituent parts of
* the index; a result of zero means that there is no limit or the limit is not known
* @throws java.sql.SQLException if a database access error occurs
*/
public int getMaxIndexLength() throws SQLException {
return 256;
}
/**
* Retrieves the maximum number of characters that this database allows in a schema name.
*
* @return the maximum number of characters allowed in a schema name; a result of zero means that there is no limit
* or the limit is not known
* @throws java.sql.SQLException if a database access error occurs
*/
public int getMaxSchemaNameLength() throws SQLException {
return 32;
}
/**
* Retrieves the maximum number of characters that this database allows in a procedure name.
*
* @return the maximum number of characters allowed in a procedure name; a result of zero means that there is no
* limit or the limit is not known
* @throws java.sql.SQLException if a database access error occurs
*/
public int getMaxProcedureNameLength() throws SQLException {
return 0;
}
/**
* Retrieves the maximum number of characters that this database allows in a catalog name.
*
* @return the maximum number of characters allowed in a catalog name; a result of zero means that there is no limit
* or the limit is not known
* @throws java.sql.SQLException if a database access error occurs
*/
public int getMaxCatalogNameLength() throws SQLException {
return 0;
}
/**
* Retrieves the maximum number of bytes this database allows in a single row.
*
* @return the maximum number of bytes allowed for a row; a result of zero means that there is no limit or the limit
* is not known
* @throws java.sql.SQLException if a database access error occurs
*/
public int getMaxRowSize() throws SQLException {
return 0;
}
/**
* Retrieves whether the return value for the method getMaxRowSize
includes the SQL data types
* LONGVARCHAR
and LONGVARBINARY
.
*
* @return true
if so; false
otherwise
* @throws java.sql.SQLException if a database access error occurs
*/
public boolean doesMaxRowSizeIncludeBlobs() throws SQLException {
return false;
}
/**
* Retrieves the maximum number of characters this database allows in an SQL statement.
*
* @return the maximum number of characters allowed for an SQL statement; a result of zero means that there is no
* limit or the limit is not known
* @throws java.sql.SQLException if a database access error occurs
*/
public int getMaxStatementLength() throws SQLException {
return 0;
}
/**
* Retrieves the maximum number of active statements to this database that can be open at the same time.
*
* @return the maximum number of statements that can be open at one time; a result of zero means that there is no
* limit or the limit is not known
* @throws java.sql.SQLException if a database access error occurs
*/
public int getMaxStatements() throws SQLException {
return 0;
}
/**
* Retrieves the maximum number of characters this database allows in a table name.
*
* @return the maximum number of characters allowed for a table name; a result of zero means that there is no limit
* or the limit is not known
* @throws java.sql.SQLException if a database access error occurs
*/
public int getMaxTableNameLength() throws SQLException {
return 64;
}
/**
* Retrieves the maximum number of tables this database allows in a SELECT
statement.
*
* @return the maximum number of tables allowed in a SELECT
statement; a result of zero means that
* there is no limit or the limit is not known
* @throws java.sql.SQLException if a database access error occurs
*/
public int getMaxTablesInSelect() throws SQLException {
return 256;
}
/**
* Retrieves the maximum number of characters this database allows in a user name.
*
* @return the maximum number of characters allowed for a user name; a result of zero means that there is no limit
* or the limit is not known
* @throws java.sql.SQLException if a database access error occurs
*/
public int getMaxUserNameLength() throws SQLException {
return 16;
}
/**
* Retrieves this database's default transaction isolation level. The possible values are defined in
* java.sql.Connection
.
*
* @return the default isolation level
* @throws java.sql.SQLException if a database access error occurs
* @see java.sql.Connection
*/
public int getDefaultTransactionIsolation() throws SQLException {
return Connection.TRANSACTION_REPEATABLE_READ;
}
/**
* Retrieves whether this database supports transactions. If not, invoking the method commit
is a noop,
* and the isolation level is TRANSACTION_NONE
.
*
* @return true
if transactions are supported; false
otherwise
* @throws java.sql.SQLException if a database access error occurs
*/
public boolean supportsTransactions() throws SQLException {
return true;
}
/**
* Retrieves whether this database supports the given transaction isolation level.
*
* @param level one of the transaction isolation levels defined in java.sql.Connection
* @return true
if so; false
otherwise
* @throws java.sql.SQLException if a database access error occurs
* @see java.sql.Connection
*/
public boolean supportsTransactionIsolationLevel(final int level) throws SQLException {
switch (level) {
case Connection.TRANSACTION_READ_UNCOMMITTED:
case Connection.TRANSACTION_READ_COMMITTED:
case Connection.TRANSACTION_REPEATABLE_READ:
case Connection.TRANSACTION_SERIALIZABLE:
return true;
default:
return false;
}
}
/**
* Retrieves whether this database supports both data definition and data manipulation statements within a
* transaction.
*
* @return true
if so; false
otherwise
* @throws java.sql.SQLException if a database access error occurs
*/
public boolean supportsDataDefinitionAndDataManipulationTransactions() throws SQLException {
return true;
}
/**
* Retrieves whether this database supports only data manipulation statements within a transaction.
*
* @return true
if so; false
otherwise
* @throws java.sql.SQLException if a database access error occurs
*/
public boolean supportsDataManipulationTransactionsOnly() throws SQLException {
return false;
}
/**
* Retrieves whether a data definition statement within a transaction forces the transaction to commit.
*
* @return true
if so; false
otherwise
* @throws java.sql.SQLException if a database access error occurs
*/
public boolean dataDefinitionCausesTransactionCommit() throws SQLException {
return true;
}
/**
* Retrieves whether this database ignores a data definition statement within a transaction.
*
* @return true
if so; false
otherwise
* @throws java.sql.SQLException if a database access error occurs
*/
public boolean dataDefinitionIgnoredInTransactions() throws SQLException {
return false;
}
/**
* Retrieves a description of the stored procedures available in the given catalog.
*
* Only procedure descriptions matching the schema and procedure name criteria are returned. They are ordered by
* PROCEDURE_CAT
, PROCEDURE_SCHEM
, PROCEDURE_NAME
and SPECIFIC_
* NAME
.
*
* Each procedure description has the the following columns:
- PROCEDURE_CAT String => procedure
* catalog (may be
null
) - PROCEDURE_SCHEM String => procedure schema (may be
*
null
) - PROCEDURE_NAME String => procedure name
- reserved for future use
- reserved
* for future use
- reserved for future use
- REMARKS String => explanatory comment on the procedure
*
- PROCEDURE_TYPE short => kind of procedure:
- procedureResultUnknown - Cannot determine if a
* return value will be returned
- procedureNoResult - Does not return a return value
- procedureReturnsResult
* - Returns a return value
- SPECIFIC_NAME String => The name which uniquely identifies this
* procedure within its schema.
*
* A user may not have permissions to execute any of the procedures that are returned by getProcedures
*
* @param catalog a catalog name; must match the catalog name as it is stored in the database; ""
* retrieves those without a catalog; null
means that the catalog name
* should not be used to narrow the search
* @param schemaPattern a schema name pattern; must match the schema name as it is stored in the database; ""
* retrieves those without a schema; null
means that the schema name should
* not be used to narrow the search
* @param procedureNamePattern a procedure name pattern; must match the procedure name as it is stored in the
* database
* @return ResultSet
- each row is a procedure description
* @throws java.sql.SQLException if a database access error occurs
* @see #getSearchStringEscape
*/
public ResultSet getProcedures(final String catalog, final String schemaPattern, final String procedureNamePattern)
throws SQLException {
log.info("getting empty result set, procedures");
return getEmptyResultSet();
}
/**
* Retrieves a description of the given catalog's stored procedure parameter and result columns.
*
* Only descriptions matching the schema, procedure and parameter name criteria are returned. They are ordered
* by PROCEDURE_CAT, PROCEDURE_SCHEM, PROCEDURE_NAME and SPECIFIC_NAME. Within this, the return value, if any, is
* first. Next are the parameter descriptions in call order. The column descriptions follow in column number order.
*
* Each row in the ResultSet
is a parameter description or column description with the following
* fields:
- PROCEDURE_CAT String => procedure catalog (may be
null
)
* - PROCEDURE_SCHEM String => procedure schema (may be
null
) - PROCEDURE_NAME String
* => procedure name
- COLUMN_NAME String => column/parameter name
- COLUMN_TYPE Short => kind of
* column/parameter:
- procedureColumnUnknown - nobody knows
- procedureColumnIn - IN parameter
-
* procedureColumnInOut - INOUT parameter
- procedureColumnOut - OUT parameter
- procedureColumnReturn -
* procedure return value
- procedureColumnResult - result column in
ResultSet
* - DATA_TYPE int => SQL type from java.sql.Types
- TYPE_NAME String => SQL type name, for a UDT
* type the type name is fully qualified
- PRECISION int => precision
- LENGTH int => length in
* bytes of data
- SCALE short => scale - null is returned for data types where SCALE is not applicable.
*
- RADIX short => radix
- NULLABLE short => can it contain NULL.
- procedureNoNulls -
* does not allow NULL values
- procedureNullable - allows NULL values
- procedureNullableUnknown -
* nullability unknown
- REMARKS String => comment describing parameter/column
- COLUMN_DEF
* String => default value for the column, which should be interpreted as a string when the value is enclosed in
* single quotes (may be
null
) - The string NULL (not enclosed in quotes) - if NULL was
* specified as the default value
- TRUNCATE (not enclosed in quotes) - if the specified default value
* cannot be represented without truncation
- NULL - if a default value was
* not specified
- SQL_DATA_TYPE int => reserved for future use
- SQL_DATETIME_SUB int =>
* reserved for future use
- CHAR_OCTET_LENGTH int => the maximum length of binary and character based
* columns. For any other datatype the returned value is a NULL
- ORDINAL_POSITION int => the ordinal
* position, starting from 1, for the input and output parameters for a procedure. A value of 0 is returned if this
* row describes the procedure's return value. For result set columns, it is the ordinal position of the column in
* the result set starting from 1. If there are multiple result sets, the column ordinal positions are
* implementation defined.
- IS_NULLABLE String => ISO rules are used to determine the nullability for a
* column.
- YES --- if the parameter can include NULLs
- NO --- if the parameter
* cannot include NULLs
- empty string --- if the nullability for the parameter is unknown
* - SPECIFIC_NAME String => the name which uniquely identifies this procedure within its schema.
*
* Note: Some databases may not return the column descriptions for a procedure.
*
* The PRECISION column represents the specified column size for the given column. For numeric data, this is the
* maximum precision. For character data, this is the length in characters. For datetime datatypes, this is the
* length in characters of the String representation (assuming the maximum allowed precision of the fractional
* seconds component). For binary data, this is the length in bytes. For the ROWID datatype, this is the length in
* bytes. Null is returned for data types where the column size is not applicable.
*
* @param catalog a catalog name; must match the catalog name as it is stored in the database; ""
* retrieves those without a catalog; null
means that the catalog name
* should not be used to narrow the search
* @param schemaPattern a schema name pattern; must match the schema name as it is stored in the database; ""
* retrieves those without a schema; null
means that the schema name should
* not be used to narrow the search
* @param procedureNamePattern a procedure name pattern; must match the procedure name as it is stored in the
* database
* @param columnNamePattern a column name pattern; must match the column name as it is stored in the database
* @return ResultSet
- each row describes a stored procedure parameter or column
* @throws java.sql.SQLException if a database access error occurs
* @see #getSearchStringEscape
*/
public ResultSet getProcedureColumns(final String catalog,
final String schemaPattern,
final String procedureNamePattern,
final String columnNamePattern) throws SQLException {
log.info("getting empty result set, proc columns");
return getEmptyResultSet();
}
/**
* Retrieves a description of the tables available in the given catalog. Only table descriptions matching the
* catalog, schema, table name and type criteria are returned. They are ordered by TABLE_TYPE
,
* TABLE_CAT
, TABLE_SCHEM
and TABLE_NAME
.
*
* Each table description has the following columns: - TABLE_CAT String => table catalog (may be
*
null
) - TABLE_SCHEM String => table schema (may be
null
) - TABLE_NAME
* String => table name
- TABLE_TYPE String => table type. Typical types are "TABLE", "VIEW", "SYSTEM
* TABLE", "GLOBAL TEMPORARY", "LOCAL TEMPORARY", "ALIAS", "SYNONYM".
- REMARKS String => explanatory
* comment on the table
- TYPE_CAT String => the types catalog (may be
null
)
* - TYPE_SCHEM String => the types schema (may be
null
) - TYPE_NAME String => type
* name (may be
null
) - SELF_REFERENCING_COL_NAME String => name of the designated
* "identifier" column of a typed table (may be
null
) - REF_GENERATION String => specifies how
* values in SELF_REFERENCING_COL_NAME are created. Values are "SYSTEM", "USER", "DERIVED". (may be
*
null
)
*
* Note: Some databases may not return information for all tables.
*
* @param catalog a catalog name; must match the catalog name as it is stored in the database; "" retrieves
* those without a catalog; null
means that the catalog name should not be used
* to narrow the search
* @param schemaPattern a schema name pattern; must match the schema name as it is stored in the database; ""
* retrieves those without a schema; null
means that the schema name should not
* be used to narrow the search
* @param tableNamePattern a table name pattern; must match the table name as it is stored in the database
* @param types a list of table types, which must be from the list of table types returned from {@link
* #getTableTypes},to include; null
returns all types
* @return ResultSet
- each row is a table description
* @throws java.sql.SQLException if a database access error occurs
* @see #getSearchStringEscape
*/
public abstract ResultSet getTables(final String catalog, final String schemaPattern, final String tableNamePattern, final String[] types) throws SQLException;
/**
* returns a schema name pattern, restricts the search to the current database
* @param schemaPattern the pattern, not used if null.
* @return an AND clause
*/
protected String getSchemaPattern(String schemaPattern) {
if(schemaPattern != null) {
return " AND table_schema LIKE \"" + schemaPattern + "\"";
} else {
return " AND table_schema LIKE IFNULL(database(), \"%\")";
}
}
/**
* Retrieves the schema names available in this database. The results are ordered by TABLE_CATALOG
and
* TABLE_SCHEM
.
*
* The schema columns are:
- TABLE_SCHEM String => schema name
- TABLE_CATALOG String =>
* catalog name (may be
null
)
*
* @return a ResultSet
object in which each row is a schema description
* @throws java.sql.SQLException if a database access error occurs
*/
public ResultSet getSchemas() throws SQLException {
final Statement stmt = connection.createStatement();
return stmt.executeQuery("SELECT schema_name table_schem, catalog_name table_catalog " +
"FROM information_schema.schemata");
}
/**
* Retrieves the catalog names available in this database. The results are ordered by catalog name.
*
* The catalog column is:
- TABLE_CAT String => catalog name
*
* @return a ResultSet
object in which each row has a single String
column that is a
* catalog name
* @throws java.sql.SQLException if a database access error occurs
*/
public ResultSet getCatalogs() throws SQLException {
final Statement stmt = connection.createStatement();
return stmt.executeQuery("SELECT null as table_cat");
}
/**
* Retrieves the table types available in this database. The results are ordered by table type.
*
* The table type is:
- TABLE_TYPE String => table type. Typical types are "TABLE", "VIEW",
* "SYSTEM TABLE", "GLOBAL TEMPORARY", "LOCAL TEMPORARY", "ALIAS", "SYNONYM".
*
* @return a ResultSet
object in which each row has a single String
column that is a table
* type
* @throws java.sql.SQLException if a database access error occurs
*/
public ResultSet getTableTypes() throws SQLException {
final Statement stmt = connection.createStatement();
return stmt.executeQuery("SELECT DISTINCT(table_type) FROM information_schema.tables");
}
/**
* Retrieves a description of table columns available in the specified catalog.
*
* Only column descriptions matching the catalog, schema, table and column name criteria are returned. They are
* ordered by TABLE_CAT
,TABLE_SCHEM
, TABLE_NAME
, and
* ORDINAL_POSITION
.
*
* Each column description has the following columns:
*
*
*
* - TABLE_CAT String => table catalog (may be
null
) - TABLE_SCHEM String => table
* schema (may be
null
) - TABLE_NAME String => table name
- COLUMN_NAME String =>
* column name
- DATA_TYPE int => SQL type from java.sql.Types
- TYPE_NAME String => Data source
* dependent type name, for a UDT the type name is fully qualified
- COLUMN_SIZE int => column size.
*
- BUFFER_LENGTH is not used.
- DECIMAL_DIGITS int => the number of fractional digits. Null is
* returned for data types where DECIMAL_DIGITS is not applicable.
- NUM_PREC_RADIX int => Radix (typically
* either 10 or 2)
- NULLABLE int => is NULL allowed.
- columnNoNulls - might not allow
*
NULL
values - columnNullable - definitely allows
NULL
values -
* columnNullableUnknown - nullability unknown
- REMARKS String => comment describing column (may be
*
null
) - COLUMN_DEF String => default value for the column, which should be interpreted as a
* string when the value is enclosed in single quotes (may be
null
) - SQL_DATA_TYPE int =>
* unused
- SQL_DATETIME_SUB int => unused
- CHAR_OCTET_LENGTH int => for char types the maximum
* number of bytes in the column
- ORDINAL_POSITION int => index of column in table (starting at 1)
*
- IS_NULLABLE String => ISO rules are used to determine the nullability for a column.
- YES ---
* if the parameter can include NULLs
- NO --- if the parameter cannot include NULLs
- empty string
* --- if the nullability for the parameter is unknown
- SCOPE_CATLOG String => catalog of table
* that is the scope of a reference attribute (
null
if DATA_TYPE isn't REF) - SCOPE_SCHEMA
* String => schema of table that is the scope of a reference attribute (
null
if the DATA_TYPE isn't
* REF) - SCOPE_TABLE String => table name that this the scope of a reference attribure (
null
* if the DATA_TYPE isn't REF) - SOURCE_DATA_TYPE short => source type of a distinct type or user-generated
* Ref type, SQL type from java.sql.Types (
null
if DATA_TYPE isn't DISTINCT or user-generated REF)
* - IS_AUTOINCREMENT String => Indicates whether this column is auto incremented
- YES
* --- if the column is auto incremented
- NO --- if the column is not auto incremented
- empty
* string --- if it cannot be determined whether the column is auto incremented parameter is unknown
*
* The COLUMN_SIZE column the specified column size for the given column. For numeric data, this is the maximum
* precision. For character data, this is the length in characters. For datetime datatypes, this is the length in
* characters of the String representation (assuming the maximum allowed precision of the fractional seconds
* component). For binary data, this is the length in bytes. For the ROWID datatype, this is the length in bytes.
* Null is returned for data types where the column size is not applicable.
*
* @param catalog a catalog name; must match the catalog name as it is stored in the database; ""
* retrieves those without a catalog; null
means that the catalog name should
* not be used to narrow the search
* @param schemaPattern a schema name pattern; must match the schema name as it is stored in the database; ""
* retrieves those without a schema; null
means that the schema name should
* not be used to narrow the search
* @param tableNamePattern a table name pattern; must match the table name as it is stored in the database
* @param columnNamePattern a column name pattern; must match the column name as it is stored in the database
* @return ResultSet
- each row is a column description
* @throws java.sql.SQLException if a database access error occurs
* @see #getSearchStringEscape
*/
public abstract ResultSet getColumns(final String catalog, final String schemaPattern, final String tableNamePattern, final String columnNamePattern)
throws SQLException;
/**
* Retrieves a description of the access rights for a table's columns.
*
* Only privileges matching the column name criteria are returned. They are ordered by COLUMN_NAME and
* PRIVILEGE.
*
* Each privilige description has the following columns:
- TABLE_CAT String => table catalog (may
* be
null
) - TABLE_SCHEM String => table schema (may be
null
)
* - TABLE_NAME String => table name
- COLUMN_NAME String => column name
- GRANTOR String
* => grantor of access (may be
null
) - GRANTEE String => grantee of access
*
- PRIVILEGE String => name of access (SELECT, INSERT, UPDATE, REFRENCES, ...)
- IS_GRANTABLE
* String => "YES" if grantee is permitted to grant to others; "NO" if not;
null
if unknown
*
* @param catalog a catalog name; must match the catalog name as it is stored in the database; ""
* retrieves those without a catalog; null
means that the catalog name should
* not be used to narrow the search
* @param schema a schema name; must match the schema name as it is stored in the database; "" retrieves
* those without a schema; null
means that the schema name should not be used
* to narrow the search
* @param table a table name; must match the table name as it is stored in the database
* @param columnNamePattern a column name pattern; must match the column name as it is stored in the database
* @return ResultSet
- each row is a column privilege description
* @throws java.sql.SQLException if a database access error occurs
* @see #getSearchStringEscape
*/
public ResultSet getColumnPrivileges(final String catalog, final String schema, final String table, final String columnNamePattern)
throws SQLException {
log.info("getting empty result set, column privileges");
return getEmptyResultSet();
}
/**
* Retrieves a description of the access rights for each table available in a catalog. Note that a table privilege
* applies to one or more columns in the table. It would be wrong to assume that this privilege applies to all
* columns (this may be true for some systems but is not true for all.)
*
* Only privileges matching the schema and table name criteria are returned. They are ordered by
* TABLE_CAT
, TABLE_SCHEM
, TABLE_NAME
, and PRIVILEGE
.
*
* Each privilige description has the following columns:
- TABLE_CAT String => table catalog (may
* be
null
) - TABLE_SCHEM String => table schema (may be
null
)
* - TABLE_NAME String => table name
- GRANTOR String => grantor of access (may be
*
null
) - GRANTEE String => grantee of access
- PRIVILEGE String => name of access
* (SELECT, INSERT, UPDATE, REFRENCES, ...)
- IS_GRANTABLE String => "YES" if grantee is permitted to grant
* to others; "NO" if not;
null
if unknown
*
* @param catalog a catalog name; must match the catalog name as it is stored in the database; "" retrieves
* those without a catalog; null
means that the catalog name should not be used
* to narrow the search
* @param schemaPattern a schema name pattern; must match the schema name as it is stored in the database; ""
* retrieves those without a schema; null
means that the schema name should not
* be used to narrow the search
* @param tableNamePattern a table name pattern; must match the table name as it is stored in the database
* @return ResultSet
- each row is a table privilege description
* @throws java.sql.SQLException if a database access error occurs
* @see #getSearchStringEscape
*/
public ResultSet getTablePrivileges(final String catalog, final String schemaPattern, final String tableNamePattern)
throws SQLException {
final Statement stmt = connection.createStatement();
final String query = "SELECT null table_cat, " +
"table_schema table_schem, " +
"table_name, " +
"null grantor, " +
"user() grantee, " +
"'update' privilege, " +
"'yes' is_grantable " +
"FROM information_schema.columns " +
"WHERE table_schema LIKE '" + ((schemaPattern == null) ? "%" : schemaPattern) + "'" +
" AND table_name LIKE '" + ((tableNamePattern == null) ? "%" : tableNamePattern) + "'";
return stmt.executeQuery(query);
}
/**
* Retrieves a description of a table's optimal set of columns that uniquely identifies a row. They are ordered by
* SCOPE.
*
* Each column description has the following columns:
- SCOPE short => actual scope of result
* - bestRowTemporary - very temporary, while using row
- bestRowTransaction - valid for remainder of current
* transaction
- bestRowSession - valid for remainder of current session
- COLUMN_NAME String =>
* column name
- DATA_TYPE int => SQL data type from java.sql.Types
- TYPE_NAME String => Data
* source dependent type name, for a UDT the type name is fully qualified
- COLUMN_SIZE int => precision
*
- BUFFER_LENGTH int => not used
- DECIMAL_DIGITS short => scale - Null is returned for data
* types where DECIMAL_DIGITS is not applicable.
- PSEUDO_COLUMN short => is this a pseudo column like an
* Oracle ROWID
- bestRowUnknown - may or may not be pseudo column
- bestRowNotPseudo - is NOT a pseudo
* column
- bestRowPseudo - is a pseudo column
*
* The COLUMN_SIZE column represents the specified column size for the given column. For numeric data, this is
* the maximum precision. For character data, this is the length in characters. For datetime datatypes, this is the
* length in characters of the String representation (assuming the maximum allowed precision of the fractional
* seconds component). For binary data, this is the length in bytes. For the ROWID datatype, this is the length in
* bytes. Null is returned for data types where the column size is not applicable.
*
* @param catalog a catalog name; must match the catalog name as it is stored in the database; "" retrieves those
* without a catalog; null
means that the catalog name should not be used to narrow the search
* @param schema a schema name; must match the schema name as it is stored in the database; "" retrieves those
* without a schema; null
means that the schema name should not be used to narrow the search
* @param table a table name; must match the table name as it is stored in the database
* @param scope the scope of interest; use same values as SCOPE
* @param nullable include columns that are nullable.
* @return ResultSet
- each row is a column description
* @throws java.sql.SQLException if a database access error occurs
*/
protected final String dataTypeClause =
" CASE data_type" +
" WHEN 'int' THEN " + Types.INTEGER +
" WHEN 'mediumint' THEN "+Types.INTEGER +
" WHEN 'varchar' THEN " + Types.VARCHAR +
" WHEN 'datetime' THEN " + Types.TIMESTAMP +
" WHEN 'date' THEN " + Types.DATE +
" WHEN 'time' THEN " + Types.TIME +
" WHEN 'text' THEN " + Types.VARCHAR +
" WHEN 'bigint' THEN " + Types.BIGINT +
" WHEN 'varbinary' THEN " + Types.VARBINARY +
" WHEN 'timestamp' THEN " + Types.TIMESTAMP +
" WHEN 'double' THEN " + Types.DOUBLE +
" WHEN 'bit' THEN " + Types.BIT +
" END";
public abstract ResultSet getBestRowIdentifier(final String catalog, final String schema, final String table, final int scope, final boolean nullable)
throws SQLException;
/**
* Retrieves a description of a table's columns that are automatically updated when any value in a row is updated.
* They are unordered.
*
* Each column description has the following columns:
- SCOPE short => is not used
*
- COLUMN_NAME String => column name
- DATA_TYPE int => SQL data type from
*
java.sql.Types
- TYPE_NAME String => Data source-dependent type name
- COLUMN_SIZE
* int => precision
- BUFFER_LENGTH int => length of column value in bytes
- DECIMAL_DIGITS short
* => scale - Null is returned for data types where DECIMAL_DIGITS is not applicable.
- PSEUDO_COLUMN short
* => whether this is pseudo column like an Oracle ROWID
- versionColumnUnknown - may or may not be pseudo
* column
- versionColumnNotPseudo - is NOT a pseudo column
- versionColumnPseudo - is a pseudo column
*
*
* The COLUMN_SIZE column represents the specified column size for the given column. For numeric data, this is
* the maximum precision. For character data, this is the length in characters. For datetime datatypes, this is the
* length in characters of the String representation (assuming the maximum allowed precision of the fractional
* seconds component). For binary data, this is the length in bytes. For the ROWID datatype, this is the length in
* bytes. Null is returned for data types where the column size is not applicable.
*
* @param catalog a catalog name; must match the catalog name as it is stored in the database; "" retrieves those
* without a catalog; null
means that the catalog name should not be used to narrow the
* search
* @param schema a schema name; must match the schema name as it is stored in the database; "" retrieves those
* without a schema; null
means that the schema name should not be used to narrow the
* search
* @param table a table name; must match the table name as it is stored in the database
* @return a ResultSet
object in which each row is a column description
* @throws java.sql.SQLException if a database access error occurs
*/
public ResultSet getVersionColumns(final String catalog, final String schema, final String table) throws SQLException {
//TODO: check this!
log.info("getting empty result set, version columns");
return getEmptyResultSet();
}
/**
* Retrieves a description of the given table's primary key columns. They are ordered by COLUMN_NAME.
*
* Each primary key column description has the following columns:
- TABLE_CAT String => table
* catalog (may be
null
) - TABLE_SCHEM String => table schema (may be
null
)
* - TABLE_NAME String => table name
- COLUMN_NAME String => column name
- KEY_SEQ short
* => sequence number within primary key( a value of 1 represents the first column of the primary key, a value of 2
* would represent the second column within the primary key).
- PK_NAME String => primary key name (may be
*
null
)
*
* @param catalog a catalog name; must match the catalog name as it is stored in the database; "" retrieves those
* without a catalog; null
means that the catalog name should not be used to narrow the
* search
* @param schema a schema name; must match the schema name as it is stored in the database; "" retrieves those
* without a schema; null
means that the schema name should not be used to narrow the
* search
* @param table a table name; must match the table name as it is stored in the database
* @return ResultSet
- each row is a primary key column description
* @throws java.sql.SQLException if a database access error occurs
*/
public abstract ResultSet getPrimaryKeys(final String catalog, final String schema, final String table) throws SQLException;
/**
* Retrieves a description of the primary key columns that are referenced by the given table's foreign key columns
* (the primary keys imported by a table). They are ordered by PKTABLE_CAT, PKTABLE_SCHEM, PKTABLE_NAME, and
* KEY_SEQ.
*
* Each primary key column description has the following columns:
s - PKTABLE_CAT String => primary
* key table catalog being imported (may be
null
) - PKTABLE_SCHEM String => primary key table
* schema being imported (may be
null
) - PKTABLE_NAME String => primary key table name being
* imported
- PKCOLUMN_NAME String => primary key column name being imported
- FKTABLE_CAT String
* => foreign key table catalog (may be
null
) - FKTABLE_SCHEM String => foreign key table
* schema (may be
null
) - FKTABLE_NAME String => foreign key table name
*
- FKCOLUMN_NAME String => foreign key column name
- KEY_SEQ short => sequence number within a
* foreign key( a value of 1 represents the first column of the foreign key, a value of 2 would represent the second
* column within the foreign key).
- UPDATE_RULE short => What happens to a foreign key when the primary
* key is updated:
- importedNoAction - do not allow update of primary key if it has been imported
-
* importedKeyCascade - change imported key to agree with primary key update
- importedKeySetNull - change
* imported key to
NULL
if its primary key has been updated - importedKeySetDefault - change
* imported key to default values if its primary key has been updated
- importedKeyRestrict - same as
* importedKeyNoAction (for ODBC 2.x compatibility)
- DELETE_RULE short => What happens to the
* foreign key when primary is deleted.
- importedKeyNoAction - do not allow delete of primary key if it has
* been imported
- importedKeyCascade - delete rows that import a deleted key
- importedKeySetNull - change
* imported key to NULL if its primary key has been deleted
- importedKeyRestrict - same as importedKeyNoAction
* (for ODBC 2.x compatibility)
- importedKeySetDefault - change imported key to default if its primary key has
* been deleted
- FK_NAME String => foreign key name (may be
null
) - PK_NAME
* String => primary key name (may be
null
) - DEFERRABILITY short => can the evaluation of
* foreign key constraints be deferred until commit
- importedKeyInitiallyDeferred - see SQL92 for
* definition
- importedKeyInitiallyImmediate - see SQL92 for definition
- importedKeyNotDeferrable - see
* SQL92 for definition
*
* @param catalog a catalog name; must match the catalog name as it is stored in the database; "" retrieves those
* without a catalog; null
means that the catalog name should not be used to narrow the
* search
* @param schema a schema name; must match the schema name as it is stored in the database; "" retrieves those
* without a schema; null
means that the schema name should not be used to narrow the
* search
* @param table a table name; must match the table name as it is stored in the database
* @return ResultSet
- each row is a primary key column description
* @throws java.sql.SQLException if a database access error occurs
* @see #getExportedKeys
*/
public abstract ResultSet getImportedKeys(final String catalog, final String schema, final String table) throws SQLException;
/**
* Retrieves a description of the foreign key columns that reference the given table's primary key columns (the
* foreign keys exported by a table). They are ordered by FKTABLE_CAT, FKTABLE_SCHEM, FKTABLE_NAME, and KEY_SEQ.
*
* THE QUERY: SELECT null PKTABLE_CAT, kcu.referenced_table_schema PKTABLE_SCHEM, kcu.referenced_table_name
* PKTABLE_NAME, kcu.referenced_column_name PKCOLUMN_NAME, null FKTABLE_CAT, kcu.table_schema FKTABLE_SCHEM,
* kcu.table_name FKTABLE_NAME, kcu.column_name FKCOLUMN_NAME, kcu.position_in_unique_constraint KEY_SEQ, CASE
* update_rule WHEN 'RESTRICT' THEN 1 WHEN 'NO ACTION' THEN 3 WHEN 'CASCADE' THEN 0 WHEN 'SET NULL' THEN 2 WHEN 'SET
* DEFAULT' THEN 4 END UPDATE_RULE, CASE delete_rule WHEN 'RESTRICT' THEN 1 WHEN 'NO ACTION' THEN 3 WHEN 'CASCADE'
* THEN 0 WHEN 'SET NULL' THEN 2 WHEN 'SET DEFAULT' THEN 4 END UPDATE_RULE, rc.constraint_name FK_NAME, null
* PK_NAME, 6 DEFERRABILITY FROM information_schema.key_column_usage kcu INNER JOIN
* information_schema.referential_constraints rc ON kcu.constraint_schema=rc.constraint_schema AND
* kcu.constraint_name=rc.constraint_name WHERE kcu.table_schema='key_tests' AND kcu.referenced_table_name='a' ORDER
* BY FKTABLE_CAT, FKTABLE_SCHEM, FKTABLE_NAME, KEY_SEQ
*
*
* Each foreign key column description has the following columns:
- PKTABLE_CAT String => primary
* key table catalog (may be
null
) - PKTABLE_SCHEM String => primary key table schema (may be
*
null
) - PKTABLE_NAME String => primary key table name
- PKCOLUMN_NAME String =>
* primary key column name
- FKTABLE_CAT String => foreign key table catalog (may be
null
)
* being exported (may be null
) - FKTABLE_SCHEM String => foreign key table schema (may be
*
null
) being exported (may be null
) - FKTABLE_NAME String => foreign key table
* name being exported
- FKCOLUMN_NAME String => foreign key column name being exported
- KEY_SEQ
* short => sequence number within foreign key( a value of 1 represents the first column of the foreign key, a value
* of 2 would represent the second column within the foreign key).
- UPDATE_RULE short => What happens to
* foreign key when primary is updated:
- importedNoAction - do not allow update of primary key if it has
* been imported
- importedKeyCascade - change imported key to agree with primary key update
-
* importedKeySetNull - change imported key to
NULL
if its primary key has been updated -
* importedKeySetDefault - change imported key to default values if its primary key has been updated
-
* importedKeyRestrict - same as importedKeyNoAction (for ODBC 2.x compatibility)
- DELETE_RULE short
* => What happens to the foreign key when primary is deleted.
- importedKeyNoAction - do not allow delete
* of primary key if it has been imported
- importedKeyCascade - delete rows that import a deleted key
-
* importedKeySetNull - change imported key to
NULL
if its primary key has been deleted -
* importedKeyRestrict - same as importedKeyNoAction (for ODBC 2.x compatibility)
- importedKeySetDefault -
* change imported key to default if its primary key has been deleted
- FK_NAME String => foreign key
* name (may be
null
) - PK_NAME String => primary key name (may be
null
)
* - DEFERRABILITY short => can the evaluation of foreign key constraints be deferred until commit
* - importedKeyInitiallyDeferred - see SQL92 for definition
- importedKeyInitiallyImmediate - see SQL92 for
* definition
- importedKeyNotDeferrable - see SQL92 for definition
*
* @param catalog a catalog name; must match the catalog name as it is stored in this database; "" retrieves those
* without a catalog; null
means that the catalog name should not be used to narrow the
* search
* @param schema a schema name; must match the schema name as it is stored in the database; "" retrieves those
* without a schema; null
means that the schema name should not be used to narrow the
* search
* @param table a table name; must match the table name as it is stored in this database
* @return a ResultSet
object in which each row is a foreign key column description
* @throws java.sql.SQLException if a database access error occurs
* @see #getImportedKeys
*/
public abstract ResultSet getExportedKeys(final String catalog, final String schema, final String table) throws SQLException;
/**
* Retrieves a description of the foreign key columns in the given foreign key table that reference the primary key
* or the columns representing a unique constraint of the parent table (could be the same or a different table).
* The number of columns returned from the parent table must match the number of columns that make up the foreign
* key. They are ordered by FKTABLE_CAT, FKTABLE_SCHEM, FKTABLE_NAME, and KEY_SEQ.
*
* Each foreign key column description has the following columns:
- PKTABLE_CAT String => parent
* key table catalog (may be
null
) - PKTABLE_SCHEM String => parent key table schema (may be
*
null
) - PKTABLE_NAME String => parent key table name
- PKCOLUMN_NAME String =>
* parent key column name
- FKTABLE_CAT String => foreign key table catalog (may be
null
)
* being exported (may be null
) - FKTABLE_SCHEM String => foreign key table schema (may be
*
null
) being exported (may be null
) - FKTABLE_NAME String => foreign key table
* name being exported
- FKCOLUMN_NAME String => foreign key column name being exported
- KEY_SEQ
* short => sequence number within foreign key( a value of 1 represents the first column of the foreign key, a value
* of 2 would represent the second column within the foreign key).
- UPDATE_RULE short => What happens to
* foreign key when parent key is updated:
- importedNoAction - do not allow update of parent key if it has
* been imported
- importedKeyCascade - change imported key to agree with parent key update
-
* importedKeySetNull - change imported key to
NULL
if its parent key has been updated -
* importedKeySetDefault - change imported key to default values if its parent key has been updated
-
* importedKeyRestrict - same as importedKeyNoAction (for ODBC 2.x compatibility)
- DELETE_RULE short
* => What happens to the foreign key when parent key is deleted.
- importedKeyNoAction - do not allow
* delete of parent key if it has been imported
- importedKeyCascade - delete rows that import a deleted key
-
* importedKeySetNull - change imported key to
NULL
if its primary key has been deleted -
* importedKeyRestrict - same as importedKeyNoAction (for ODBC 2.x compatibility)
- importedKeySetDefault -
* change imported key to default if its parent key has been deleted
- FK_NAME String => foreign key
* name (may be
null
) - PK_NAME String => parent key name (may be
null
)
* - DEFERRABILITY short => can the evaluation of foreign key constraints be deferred until commit
* - importedKeyInitiallyDeferred - see SQL92 for definition
- importedKeyInitiallyImmediate - see SQL92 for
* definition
- importedKeyNotDeferrable - see SQL92 for definition
*
* @param parentCatalog a catalog name; must match the catalog name as it is stored in the database; "" retrieves
* those without a catalog; null
means drop catalog name from the selection
* criteria
* @param parentSchema a schema name; must match the schema name as it is stored in the database; "" retrieves
* those without a schema; null
means drop schema name from the selection
* criteria
* @param parentTable the name of the table that exports the key; must match the table name as it is stored in
* the database
* @param foreignCatalog a catalog name; must match the catalog name as it is stored in the database; "" retrieves
* those without a catalog; null
means drop catalog name from the selection
* criteria
* @param foreignSchema a schema name; must match the schema name as it is stored in the database; "" retrieves
* those without a schema; null
means drop schema name from the selection
* criteria
* @param foreignTable the name of the table that imports the key; must match the table name as it is stored in
* the database
* @return ResultSet
- each row is a foreign key column description
* @throws java.sql.SQLException if a database access error occurs
* @see #getImportedKeys
*/
public ResultSet getCrossReference(final String parentCatalog,
final String parentSchema,
final String parentTable,
final String foreignCatalog,
final String foreignSchema,
final String foreignTable) throws SQLException {
//TODO: FIX
log.info("getting empty result set, cross ref");
return getEmptyResultSet();
}
/**
* Retrieves a description of all the data types supported by this database. They are ordered by DATA_TYPE and then
* by how closely the data type maps to the corresponding JDBC SQL type.
*
* If the database supports SQL distinct types, then getTypeInfo() will return a single row with a TYPE_NAME of
* DISTINCT and a DATA_TYPE of Types.DISTINCT. If the database supports SQL structured types, then getTypeInfo()
* will return a single row with a TYPE_NAME of STRUCT and a DATA_TYPE of Types.STRUCT.
*
* If SQL distinct or structured types are supported, then information on the individual types may be obtained
* from the getUDTs() method.
*
*
*
* Each type description has the following columns:
- TYPE_NAME String => Type name
*
- DATA_TYPE int => SQL data type from java.sql.Types
- PRECISION int => maximum precision
*
- LITERAL_PREFIX String => prefix used to quote a literal (may be
null
)
* - LITERAL_SUFFIX String => suffix used to quote a literal (may be
null
)
* - CREATE_PARAMS String => parameters used in creating the type (may be
null
)
* - NULLABLE short => can you use NULL for this type.
- typeNoNulls - does not allow NULL values
*
- typeNullable - allows NULL values
- typeNullableUnknown - nullability unknown
* - CASE_SENSITIVE boolean=> is it case sensitive.
- SEARCHABLE short => can you use "WHERE"
* based on this type:
- typePredNone - No support
- typePredChar - Only supported with WHERE .. LIKE
*
- typePredBasic - Supported except for WHERE .. LIKE
- typeSearchable - Supported for all WHERE ..
* - UNSIGNED_ATTRIBUTE boolean => is it unsigned.
- FIXED_PREC_SCALE boolean => can it be a money
* value.
- AUTO_INCREMENT boolean => can it be used for an auto-increment value.
*
- LOCAL_TYPE_NAME String => localized version of type name (may be
null
)
* - MINIMUM_SCALE short => minimum scale supported
- MAXIMUM_SCALE short => maximum scale
* supported
- SQL_DATA_TYPE int => unused
- SQL_DATETIME_SUB int => unused
*
- NUM_PREC_RADIX int => usually 2 or 10
*
* The PRECISION column represents the maximum column size that the server supports for the given datatype. For
* numeric data, this is the maximum precision. For character data, this is the length in characters. For datetime
* datatypes, this is the length in characters of the String representation (assuming the maximum allowed precision
* of the fractional seconds component). For binary data, this is the length in bytes. For the ROWID datatype, this
* is the length in bytes. Null is returned for data types where the column size is not applicable.
*
* @return a ResultSet
object in which each row is an SQL type description
* @throws java.sql.SQLException if a database access error occurs
*/
public ResultSet getTypeInfo() throws SQLException {
//TODO: FIX
log.info("getting empty result set, type info");
return getEmptyResultSet();
}
/**
* Retrieves a description of the given table's indices and statistics. They are ordered by NON_UNIQUE, TYPE,
* INDEX_NAME, and ORDINAL_POSITION.
*
* Each index column description has the following columns:
- TABLE_CAT String => table catalog
* (may be
null
) - TABLE_SCHEM String => table schema (may be
null
)
* - TABLE_NAME String => table name
- NON_UNIQUE boolean => Can index values be non-unique. false
* when TYPE is tableIndexStatistic
- INDEX_QUALIFIER String => index catalog (may be
null
);
* null
when TYPE is tableIndexStatistic - INDEX_NAME String => index name;
null
* when TYPE is tableIndexStatistic - TYPE short => index type:
- tableIndexStatistic - this
* identifies table statistics that are returned in conjuction with a table's index descriptions
-
* tableIndexClustered - this is a clustered index
- tableIndexHashed - this is a hashed index
-
* tableIndexOther - this is some other style of index
- ORDINAL_POSITION short => column sequence
* number within index; zero when TYPE is tableIndexStatistic
- COLUMN_NAME String => column name;
*
null
when TYPE is tableIndexStatistic - ASC_OR_DESC String => column sort sequence, "A" =>
* ascending, "D" => descending, may be
null
if sort sequence is not supported; null
when
* TYPE is tableIndexStatistic - CARDINALITY int => When TYPE is tableIndexStatistic, then this is the
* number of rows in the table; otherwise, it is the number of unique values in the index.
- PAGES int =>
* When TYPE is tableIndexStatisic then this is the number of pages used for the table, otherwise it is the number
* of pages used for the current index.
- FILTER_CONDITION String => Filter condition, if any. (may be
*
null
)
*
* @param catalog a catalog name; must match the catalog name as it is stored in this database; "" retrieves
* those without a catalog; null
means that the catalog name should not be used to
* narrow the search
* @param schema a schema name; must match the schema name as it is stored in this database; "" retrieves those
* without a schema; null
means that the schema name should not be used to narrow
* the search
* @param table a table name; must match the table name as it is stored in this database
* @param unique when true, return only indices for unique values; when false, return indices regardless of
* whether unique or not
* @param approximate when true, result is allowed to reflect approximate or out of data values; when false, results
* are requested to be accurate
* @return ResultSet
- each row is an index column description
* @throws java.sql.SQLException if a database access error occurs
*/
public ResultSet getIndexInfo(final String catalog, final String schema, final String table, final boolean unique, final boolean approximate)
throws SQLException {
final String query = "SELECT null table_cat," +
" table_schema table_schem," +
" table_name," +
" non_unique," +
" table_schema index_qualifier," +
" index_name," +
" " + tableIndexOther + " type," +
" seq_in_index ordinal_position," +
" column_name," +
" collation asc_or_desc," +
" cardinality," +
" null as pages," +
" null as filter_condition" +
" FROM information_schema.statistics" +
" WHERE table_name='" + table + "' " +
((schema != null) ? (" AND table_schema like '" + schema + "' ") : "") +
(unique ? " AND NON_UNIQUE = 0" : "") +
" ORDER BY NON_UNIQUE, TYPE, INDEX_NAME, ORDINAL_POSITION";
final Statement stmt = connection.createStatement();
return stmt.executeQuery(query);
}
/**
* Retrieves whether this database supports the given result set type.
*
* @param type defined in java.sql.ResultSet
* @return true
if so; false
otherwise
* @throws java.sql.SQLException if a database access error occurs
* @see java.sql.Connection
* @since 1.2
*/
public boolean supportsResultSetType(final int type) throws SQLException {
return true;
}
/**
* Retrieves whether this database supports the given concurrency type in combination with the given result set
* type.
*
* @param type defined in java.sql.ResultSet
* @param concurrency type defined in java.sql.ResultSet
* @return true
if so; false
otherwise
* @throws java.sql.SQLException if a database access error occurs
* @see java.sql.Connection
* @since 1.2
*/
public boolean supportsResultSetConcurrency(final int type, final int concurrency) throws SQLException {
return concurrency == ResultSet.CONCUR_READ_ONLY;
}
/**
* Retrieves whether for the given type of ResultSet
object, the result set's own updates are visible.
*
* @param type the ResultSet
type; one of ResultSet.TYPE_FORWARD_ONLY
,
* ResultSet.TYPE_SCROLL_INSENSITIVE
, or ResultSet.TYPE_SCROLL_SENSITIVE
* @return true
if updates are visible for the given result set type; false
otherwise
* @throws java.sql.SQLException if a database access error occurs
* @since 1.2
*/
public boolean ownUpdatesAreVisible(final int type) throws SQLException {
return false;
}
/**
* Retrieves whether a result set's own deletes are visible.
*
* @param type the ResultSet
type; one of ResultSet.TYPE_FORWARD_ONLY
,
* ResultSet.TYPE_SCROLL_INSENSITIVE
, or ResultSet.TYPE_SCROLL_SENSITIVE
* @return true
if deletes are visible for the given result set type; false
otherwise
* @throws java.sql.SQLException if a database access error occurs
* @since 1.2
*/
public boolean ownDeletesAreVisible(final int type) throws SQLException {
return false;
}
/**
* Retrieves whether a result set's own inserts are visible.
*
* @param type the ResultSet
type; one of ResultSet.TYPE_FORWARD_ONLY
,
* ResultSet.TYPE_SCROLL_INSENSITIVE
, or ResultSet.TYPE_SCROLL_SENSITIVE
* @return true
if inserts are visible for the given result set type; false
otherwise
* @throws java.sql.SQLException if a database access error occurs
* @since 1.2
*/
public boolean ownInsertsAreVisible(final int type) throws SQLException {
return false;
}
/**
* Retrieves whether updates made by others are visible.
*
* @param type the ResultSet
type; one of ResultSet.TYPE_FORWARD_ONLY
,
* ResultSet.TYPE_SCROLL_INSENSITIVE
, or ResultSet.TYPE_SCROLL_SENSITIVE
* @return true
if updates made by others are visible for the given result set type; false
* otherwise
* @throws java.sql.SQLException if a database access error occurs
* @since 1.2
*/
public boolean othersUpdatesAreVisible(final int type) throws SQLException {
return false;
}
/**
* Retrieves whether deletes made by others are visible.
*
* @param type the ResultSet
type; one of ResultSet.TYPE_FORWARD_ONLY
,
* ResultSet.TYPE_SCROLL_INSENSITIVE
, or ResultSet.TYPE_SCROLL_SENSITIVE
* @return true
if deletes made by others are visible for the given result set type; false
* otherwise
* @throws java.sql.SQLException if a database access error occurs
* @since 1.2
*/
public boolean othersDeletesAreVisible(final int type) throws SQLException {
return false;
}
/**
* Retrieves whether inserts made by others are visible.
*
* @param type the ResultSet
type; one of ResultSet.TYPE_FORWARD_ONLY
,
* ResultSet.TYPE_SCROLL_INSENSITIVE
, or ResultSet.TYPE_SCROLL_SENSITIVE
* @return true
if inserts made by others are visible for the given result set type; false
* otherwise
* @throws java.sql.SQLException if a database access error occurs
* @since 1.2
*/
public boolean othersInsertsAreVisible(final int type) throws SQLException {
return false;
}
/**
* Retrieves whether or not a visible row update can be detected by calling the method
* ResultSet.rowUpdated
.
*
* @param type the ResultSet
type; one of ResultSet.TYPE_FORWARD_ONLY
,
* ResultSet.TYPE_SCROLL_INSENSITIVE
, or ResultSet.TYPE_SCROLL_SENSITIVE
* @return true
if changes are detected by the result set type; false
otherwise
* @throws java.sql.SQLException if a database access error occurs
* @since 1.2
*/
public boolean updatesAreDetected(final int type) throws SQLException {
return false;
}
/**
* Retrieves whether or not a visible row delete can be detected by calling the method
* ResultSet.rowDeleted
. If the method deletesAreDetected
returns false
, it
* means that deleted rows are removed from the result set.
*
* @param type the ResultSet
type; one of ResultSet.TYPE_FORWARD_ONLY
,
* ResultSet.TYPE_SCROLL_INSENSITIVE
, or ResultSet.TYPE_SCROLL_SENSITIVE
* @return true
if deletes are detected by the given result set type; false
otherwise
* @throws java.sql.SQLException if a database access error occurs
* @since 1.2
*/
public boolean deletesAreDetected(final int type) throws SQLException {
return false;
}
/**
* Retrieves whether or not a visible row insert can be detected by calling the method
* ResultSet.rowInserted
.
*
* @param type the ResultSet
type; one of ResultSet.TYPE_FORWARD_ONLY
,
* ResultSet.TYPE_SCROLL_INSENSITIVE
, or ResultSet.TYPE_SCROLL_SENSITIVE
* @return true
if changes are detected by the specified result set type; false
otherwise
* @throws java.sql.SQLException if a database access error occurs
* @since 1.2
*/
public boolean insertsAreDetected(final int type) throws SQLException {
return false;
}
/**
* Retrieves whether this database supports batch updates.
*
* @return true
if this database supports batch upcates; false
otherwise
* @throws java.sql.SQLException if a database access error occurs
* @since 1.2
*/
public boolean supportsBatchUpdates() throws SQLException {
return true;
}
/**
* Retrieves a description of the user-defined types (UDTs) defined in a particular schema. Schema-specific UDTs
* may have type JAVA_OBJECT
, STRUCT
, or DISTINCT
.
*
* Only types matching the catalog, schema, type name and type criteria are returned. They are ordered by
* DATA_TYPE
, TYPE_CAT
, TYPE_SCHEM
and TYPE_NAME
. The type
* name parameter may be a fully-qualified name. In this case, the catalog and schemaPattern parameters are
* ignored.
*
* Each type description has the following columns:
- TYPE_CAT String => the type's catalog (may be
*
null
) - TYPE_SCHEM String => type's schema (may be
null
) - TYPE_NAME
* String => type name
- CLASS_NAME String => Java class name
- DATA_TYPE int => type value
* defined in java.sql.Types. One of JAVA_OBJECT, STRUCT, or DISTINCT
- REMARKS String => explanatory
* comment on the type
- BASE_TYPE short => type code of the source type of a DISTINCT type or the type
* that implements the user-generated reference type of the SELF_REFERENCING_COLUMN of a structured type as defined
* in java.sql.Types (
null
if DATA_TYPE is not DISTINCT or not STRUCT with REFERENCE_GENERATION =
* USER_DEFINED)
*
* Note: If the driver does not support UDTs, an empty result set is returned.
*
* @param catalog a catalog name; must match the catalog name as it is stored in the database; "" retrieves
* those without a catalog; null
means that the catalog name should not be used
* to narrow the search
* @param schemaPattern a schema pattern name; must match the schema name as it is stored in the database; ""
* retrieves those without a schema; null
means that the schema name should not
* be used to narrow the search
* @param typeNamePattern a type name pattern; must match the type name as it is stored in the database; may be a
* fully qualified name
* @param types a list of user-defined types (JAVA_OBJECT, STRUCT, or DISTINCT) to include;
* null
returns all types
* @return ResultSet
object in which each row describes a UDT
* @throws java.sql.SQLException if a database access error occurs
* @see #getSearchStringEscape
* @since 1.2
*/
public ResultSet getUDTs(final String catalog, final String schemaPattern, final String typeNamePattern, final int[] types)
throws SQLException {
log.info("getting empty result set, get UTDs");
return getEmptyResultSet();
}
private ResultSet getEmptyResultSet() throws SQLException {
final Statement stmt = connection.createStatement();
return stmt.executeQuery("select * from information_schema.statistics where 1=2");
}
/**
* Retrieves the connection that produced this metadata object.
*
*
* @return the connection that produced this metadata object
* @throws java.sql.SQLException if a database access error occurs
* @since 1.2
*/
public Connection getConnection() throws SQLException {
return connection;
}
/**
* Retrieves whether this database supports savepoints.
*
* @return true
if savepoints are supported; false
otherwise
* @throws java.sql.SQLException if a database access error occurs
* @since 1.4
*/
public boolean supportsSavepoints() throws SQLException {
return true;
}
/**
* Retrieves whether this database supports named parameters to callable statements.
*
* @return true
if named parameters are supported; false
otherwise
* @throws java.sql.SQLException if a database access error occurs
* @since 1.4
*/
public boolean supportsNamedParameters() throws SQLException {
return false;
}
/**
* Retrieves whether it is possible to have multiple ResultSet
objects returned from a
* CallableStatement
object simultaneously.
*
* @return true
if a CallableStatement
object can return multiple ResultSet
* objects simultaneously; false
otherwise
* @throws java.sql.SQLException if a datanase access error occurs
* @since 1.4
*/
public boolean supportsMultipleOpenResults() throws SQLException {
return false;
}
/**
* Retrieves whether auto-generated keys can be retrieved after a statement has been executed.
*
* @return true
if auto-generated keys can be retrieved after a statement has executed;
* false
otherwise If true
is returned, the JDBC driver must support the
* returning of auto-generated keys for at least SQL INSERT statements
*
* @throws java.sql.SQLException if a database access error occurs
* @since 1.4
*/
public boolean supportsGetGeneratedKeys() throws SQLException {
return true;
}
/**
* Retrieves a description of the user-defined type (UDT) hierarchies defined in a particular schema in this
* database. Only the immediate super type/ sub type relationship is modeled.
*
* Only supertype information for UDTs matching the catalog, schema, and type name is returned. The type name
* parameter may be a fully-qualified name. When the UDT name supplied is a fully-qualified name, the catalog and
* schemaPattern parameters are ignored.
*
* If a UDT does not have a direct super type, it is not listed here. A row of the ResultSet
object
* returned by this method describes the designated UDT and a direct supertype. A row has the following columns:
* - TYPE_CAT String => the UDT's catalog (may be
null
) - TYPE_SCHEM String =>
* UDT's schema (may be
null
) - TYPE_NAME String => type name of the UDT
*
- SUPERTYPE_CAT String => the direct super type's catalog (may be
null
)
* - SUPERTYPE_SCHEM String => the direct super type's schema (may be
null
)
* - SUPERTYPE_NAME String => the direct super type's name
*
* Note: If the driver does not support type hierarchies, an empty result set is returned.
*
* @param catalog a catalog name; "" retrieves those without a catalog; null
means drop catalog
* name from the selection criteria
* @param schemaPattern a schema name pattern; "" retrieves those without a schema
* @param typeNamePattern a UDT name pattern; may be a fully-qualified name
* @return a ResultSet
object in which a row gives information about the designated UDT
* @throws java.sql.SQLException if a database access error occurs
* @see #getSearchStringEscape
* @since 1.4
*/
public ResultSet getSuperTypes(final String catalog, final String schemaPattern, final String typeNamePattern) throws SQLException {
log.info("getting empty result set, get super types");
return getEmptyResultSet();
}
/**
* Retrieves a description of the table hierarchies defined in a particular schema in this database.
*
* Only supertable information for tables matching the catalog, schema and table name are returned. The table
* name parameter may be a fully- qualified name, in which case, the catalog and schemaPattern parameters are
* ignored. If a table does not have a super table, it is not listed here. Supertables have to be defined in the
* same catalog and schema as the sub tables. Therefore, the type description does not need to include this
* information for the supertable.
*
* Each type description has the following columns:
- TABLE_CAT String => the type's catalog (may
* be
null
) - TABLE_SCHEM String => type's schema (may be
null
)
* - TABLE_NAME String => type name
- SUPERTABLE_NAME String => the direct super type's name
*
*
* Note: If the driver does not support type hierarchies, an empty result set is returned.
*
* @param catalog a catalog name; "" retrieves those without a catalog; null
means drop
* catalog name from the selection criteria
* @param schemaPattern a schema name pattern; "" retrieves those without a schema
* @param tableNamePattern a table name pattern; may be a fully-qualified name
* @return a ResultSet
object in which each row is a type description
* @throws java.sql.SQLException if a database access error occurs
* @see #getSearchStringEscape
* @since 1.4
*/
public ResultSet getSuperTables(final String catalog, final String schemaPattern, final String tableNamePattern) throws SQLException {
log.info("getting empty result set, get super tables");
return getEmptyResultSet();
}
/**
* Retrieves a description of the given attribute of the given type for a user-defined type (UDT) that is available
* in the given schema and catalog.
*
* Descriptions are returned only for attributes of UDTs matching the catalog, schema, type, and attribute name
* criteria. They are ordered by TYPE_CAT
, TYPE_SCHEM
, TYPE_NAME
and
* ORDINAL_POSITION
. This description does not contain inherited attributes.
*
* The ResultSet
object that is returned has the following columns: - TYPE_CAT String =>
* type catalog (may be
null
) - TYPE_SCHEM String => type schema (may be
null
)
* - TYPE_NAME String => type name
- ATTR_NAME String => attribute name
- DATA_TYPE int
* => attribute type SQL type from java.sql.Types
- ATTR_TYPE_NAME String => Data source dependent type
* name. For a UDT, the type name is fully qualified. For a REF, the type name is fully qualified and represents the
* target type of the reference type.
- ATTR_SIZE int => column size. For char or date types this is the
* maximum number of characters; for numeric or decimal types this is precision.
- DECIMAL_DIGITS int =>
* the number of fractional digits. Null is returned for data types where DECIMAL_DIGITS is not applicable.
*
- NUM_PREC_RADIX int => Radix (typically either 10 or 2)
- NULLABLE int => whether NULL is
* allowed
- attributeNoNulls - might not allow NULL values
- attributeNullable - definitely allows NULL
* values
- attributeNullableUnknown - nullability unknown
- REMARKS String => comment describing
* column (may be
null
) - ATTR_DEF String => default value (may be
null
)
* - SQL_DATA_TYPE int => unused
- SQL_DATETIME_SUB int => unused
- CHAR_OCTET_LENGTH int
* => for char types the maximum number of bytes in the column
- ORDINAL_POSITION int => index of the
* attribute in the UDT (starting at 1)
- IS_NULLABLE String => ISO rules are used to determine the
* nullability for a attribute.
- YES --- if the attribute can include NULLs
- NO --- if the
* attribute cannot include NULLs
- empty string --- if the nullability for the attribute is unknown
* - SCOPE_CATALOG String => catalog of table that is the scope of a reference attribute (
null
* if DATA_TYPE isn't REF) - SCOPE_SCHEMA String => schema of table that is the scope of a reference
* attribute (
null
if DATA_TYPE isn't REF) - SCOPE_TABLE String => table name that is the
* scope of a reference attribute (
null
if the DATA_TYPE isn't REF) - SOURCE_DATA_TYPE short
* => source type of a distinct type or user-generated Ref type,SQL type from java.sql.Types (
null
if
* DATA_TYPE isn't DISTINCT or user-generated REF)
*
* @param catalog a catalog name; must match the catalog name as it is stored in the database; ""
* retrieves those without a catalog; null
means that the catalog name
* should not be used to narrow the search
* @param schemaPattern a schema name pattern; must match the schema name as it is stored in the database; ""
* retrieves those without a schema; null
means that the schema name should
* not be used to narrow the search
* @param typeNamePattern a type name pattern; must match the type name as it is stored in the database
* @param attributeNamePattern an attribute name pattern; must match the attribute name as it is declared in the
* database
* @return a ResultSet
object in which each row is an attribute description
* @throws java.sql.SQLException if a database access error occurs
* @see #getSearchStringEscape
* @since 1.4
*/
public ResultSet getAttributes(final String catalog,
final String schemaPattern,
final String typeNamePattern,
final String attributeNamePattern) throws SQLException {
log.info("getting empty result set, get attributes");
return getEmptyResultSet();
}
/**
* Retrieves whether this database supports the given result set holdability.
*
* @param holdability one of the following constants: ResultSet.HOLD_CURSORS_OVER_COMMIT
or
* ResultSet.CLOSE_CURSORS_AT_COMMIT
* @return true
if so; false
otherwise
* @throws java.sql.SQLException if a database access error occurs
* @see java.sql.Connection
* @since 1.4
*/
public boolean supportsResultSetHoldability(final int holdability) throws SQLException {
return holdability == ResultSet.HOLD_CURSORS_OVER_COMMIT;
}
/**
* Retrieves this database's default holdability for ResultSet
objects.
*
* @return the default holdability; either ResultSet.HOLD_CURSORS_OVER_COMMIT
or
* ResultSet.CLOSE_CURSORS_AT_COMMIT
* @throws java.sql.SQLException if a database access error occurs
* @since 1.4
*/
public int getResultSetHoldability() throws SQLException {
return ResultSet.HOLD_CURSORS_OVER_COMMIT;
}
/**
* Retrieves the major version number of the underlying database.
*
* @return the underlying database's major version
* @throws java.sql.SQLException if a database access error occurs
* @since 1.4
*/
public int getDatabaseMajorVersion() throws SQLException {
return 0;
}
/**
* Retrieves the minor version number of the underlying database.
*
* @return underlying database's minor version
* @throws java.sql.SQLException if a database access error occurs
* @since 1.4
*/
public int getDatabaseMinorVersion() throws SQLException {
return 1;
}
/**
* Retrieves the major JDBC version number for this driver.
*
* @return JDBC version major number
* @throws java.sql.SQLException if a database access error occurs
* @since 1.4
*/
public int getJDBCMajorVersion() throws SQLException {
return 4;
}
/**
* Retrieves the minor JDBC version number for this driver.
*
* @return JDBC version minor number
* @throws java.sql.SQLException if a database access error occurs
* @since 1.4
*/
public int getJDBCMinorVersion() throws SQLException {
return 0;
}
/**
* Indicates whether the SQLSTATE returned by SQLException.getSQLState
is X/Open (now known as Open
* Group) SQL CLI or SQL:2003.
*
* @return the type of SQLSTATE; one of: sqlStateXOpen or sqlStateSQL
* @throws java.sql.SQLException if a database access error occurs
* @since 1.4
*/
public int getSQLStateType() throws SQLException {
if (Utils.isJava5()) {
return sqlStateXOpen;
} else {
return sqlStateSQL;
}
}
/**
* Indicates whether updates made to a LOB are made on a copy or directly to the LOB.
*
* @return true
if updates are made to a copy of the LOB; false
if updates are made
* directly to the LOB
* @throws java.sql.SQLException if a database access error occurs
* @since 1.4
*/
public boolean locatorsUpdateCopy() throws SQLException {
return false;
}
/**
* Retrieves whether this database supports statement pooling.
*
* @return true
if so; false
otherwise
* @throws SQLException if a database access error occurs
* @since 1.4
*/
public boolean supportsStatementPooling() throws SQLException {
return false;
}
/**
* Indicates whether or not this data source supports the SQL ROWID
type, and if so the lifetime for
* which a RowId
object remains valid.
*
* The returned int values have the following relationship:
*
* ROWID_UNSUPPORTED < ROWID_VALID_OTHER < ROWID_VALID_TRANSACTION
* < ROWID_VALID_SESSION < ROWID_VALID_FOREVER
*
* so conditional logic such as
*
* if (metadata.getRowIdLifetime() > DatabaseMetaData.ROWID_VALID_TRANSACTION)
*
* can be used. Valid Forever means valid across all Sessions, and valid for a Session means valid across all its
* contained Transactions.
*
* @return the status indicating the lifetime of a RowId
* @throws java.sql.SQLException if a database access error occurs
* @since 1.6
*/
public java.sql.RowIdLifetime getRowIdLifetime() throws SQLException {
return java.sql.RowIdLifetime.ROWID_UNSUPPORTED;
}
/**
* Retrieves the schema names available in this database. The results are ordered by TABLE_CATALOG
and
* TABLE_SCHEM
.
*
* The schema columns are:
- TABLE_SCHEM String => schema name
- TABLE_CATALOG String =>
* catalog name (may be
null
)
*
* @param catalog a catalog name; must match the catalog name as it is stored in the database;"" retrieves
* those without a catalog; null means catalog name should not be used to narrow down the
* search.
* @param schemaPattern a schema name; must match the schema name as it is stored in the database; null means schema
* name should not be used to narrow down the search.
* @return a ResultSet
object in which each row is a schema description
* @throws java.sql.SQLException if a database access error occurs
* @see #getSearchStringEscape
* @since 1.6
*/
public ResultSet getSchemas(final String catalog, final String schemaPattern) throws SQLException {
final String query = "SELECT schema_name table_schem, " +
"null table_catalog " +
"FROM information_schema.schemata " +
(schemaPattern != null ? "WHERE schema_name like '" + schemaPattern + "'" : "") +
" ORDER BY table_schem";
final Statement stmt = connection.createStatement();
return stmt.executeQuery(query);
}
/**
* Retrieves whether this database supports invoking user-defined or vendor functions using the stored procedure
* escape syntax.
*
* @return true
if so; false
otherwise
* @throws java.sql.SQLException if a database access error occurs
* @since 1.6
*/
public boolean supportsStoredFunctionsUsingCallSyntax() throws SQLException {
return false;
}
/**
* Retrieves whether a SQLException
while autoCommit is true
inidcates that all open
* ResultSets are closed, even ones that are holdable. When a SQLException
occurs while autocommit is
* true
, it is vendor specific whether the JDBC driver responds with a commit operation, a rollback
* operation, or by doing neither a commit nor a rollback. A potential result of this difference is in whether or
* not holdable ResultSets are closed.
*
* @return true
if so; false
otherwise
* @throws java.sql.SQLException if a database access error occurs
* @since 1.6
*/
public boolean autoCommitFailureClosesAllResultSets() throws SQLException {
return false; //TODO: look into this
}
/**
* Retrieves a list of the client info properties that the driver supports. The result set contains the following
* columns
*
* - NAME String=> The name of the client info property
- MAX_LEN int=> The maximum
* length of the value for the property
- DEFAULT_VALUE String=> The default value of the property
* - DESCRIPTION String=> A description of the property. This will typically contain information as to
* where this property is stored in the database.
*
* The ResultSet
is sorted by the NAME column
*
*
* @return A ResultSet
object; each row is a supported client info property
*
* @throws java.sql.SQLException if a database access error occurs
*
* @since 1.6
*/
public ResultSet getClientInfoProperties() throws SQLException {
log.info("getting empty result set, client info properties");
return getEmptyResultSet(); //TODO: look into this
}
/**
* Retrieves a description of the system and user functions available in the given catalog.
*
* Only system and user function descriptions matching the schema and function name criteria are returned. They are
* ordered by FUNCTION_CAT
, FUNCTION_SCHEM
, FUNCTION_NAME
and SPECIFIC_
* NAME
.
*
* Each function description has the the following columns:
- FUNCTION_CAT String => function
* catalog (may be
null
) - FUNCTION_SCHEM String => function schema (may be
null
)
* - FUNCTION_NAME String => function name. This is the name used to invoke the function
*
- REMARKS String => explanatory comment on the function
- FUNCTION_TYPE short => kind of
* function:
- functionResultUnknown - Cannot determine if a return value or table will be returned
-
* functionNoTable- Does not return a table
- functionReturnsTable - Returns a table
* - SPECIFIC_NAME String => the name which uniquely identifies this function within its schema. This is
* a user specified, or DBMS generated, name that may be different then the
FUNCTION_NAME
for example
* with overload functions
*
* A user may not have permission to execute any of the functions that are returned by getFunctions
*
* @param catalog a catalog name; must match the catalog name as it is stored in the database; ""
* retrieves those without a catalog; null
means that the catalog name
* should not be used to narrow the search
* @param schemaPattern a schema name pattern; must match the schema name as it is stored in the database; ""
* retrieves those without a schema; null
means that the schema name should
* not be used to narrow the search
* @param functionNamePattern a function name pattern; must match the function name as it is stored in the database
* @return ResultSet
- each row is a function description
* @throws java.sql.SQLException if a database access error occurs
* @see #getSearchStringEscape
* @since 1.6
*/
public ResultSet getFunctions(final String catalog, final String schemaPattern, final String functionNamePattern)
throws SQLException {
log.info("getting empty result set, functions");
return getEmptyResultSet(); //TODO: fix
}
/**
* Retrieves a description of the given catalog's system or user function parameters and return type.
*
* Only descriptions matching the schema, function and parameter name criteria are returned. They are ordered by
* FUNCTION_CAT
, FUNCTION_SCHEM
, FUNCTION_NAME
and SPECIFIC_
* NAME
. Within this, the return value, if any, is first. Next are the parameter descriptions in call order.
* The column descriptions follow in column number order.
*
* Each row in the ResultSet
is a parameter description, column description or return type
* description with the following fields:
- FUNCTION_CAT String => function catalog (may be
*
null
) - FUNCTION_SCHEM String => function schema (may be
null
)
* - FUNCTION_NAME String => function name. This is the name used to invoke the function
*
- COLUMN_NAME String => column/parameter name
- COLUMN_TYPE Short => kind of column/parameter:
*
- functionColumnUnknown - nobody knows
- functionColumnIn - IN parameter
- functionColumnInOut -
* INOUT parameter
- functionColumnOut - OUT parameter
- functionColumnReturn - function return value
-
* functionColumnResult - Indicates that the parameter or column is a column in the
ResultSet
* - DATA_TYPE int => SQL type from java.sql.Types
- TYPE_NAME String => SQL type name, for a UDT
* type the type name is fully qualified
- PRECISION int => precision
- LENGTH int => length in
* bytes of data
- SCALE short => scale - null is returned for data types where SCALE is not applicable.
*
- RADIX short => radix
- NULLABLE short => can it contain NULL.
- functionNoNulls -
* does not allow NULL values
- functionNullable - allows NULL values
- functionNullableUnknown - nullability
* unknown
- REMARKS String => comment describing column/parameter
- CHAR_OCTET_LENGTH int
* => the maximum length of binary and character based parameters or columns. For any other datatype the returned
* value is a NULL
- ORDINAL_POSITION int => the ordinal position, starting from 1, for the input and
* output parameters. A value of 0 is returned if this row describes the function's return value. For result set
* columns, it is the ordinal position of the column in the result set starting from 1.
- IS_NULLABLE
* String => ISO rules are used to determine the nullability for a parameter or column.
- YES ---
* if the parameter or column can include NULLs
- NO --- if the parameter or column cannot include
* NULLs
- empty string --- if the nullability for the parameter or column is unknown
* - SPECIFIC_NAME String => the name which uniquely identifies this function within its schema. This is
* a user specified, or DBMS generated, name that may be different then the
FUNCTION_NAME
for example
* with overload functions
*
* The PRECISION column represents the specified column size for the given parameter or column. For numeric data,
* this is the maximum precision. For character data, this is the length in characters. For datetime datatypes,
* this is the length in characters of the String representation (assuming the maximum allowed precision of the
* fractional seconds component). For binary data, this is the length in bytes. For the ROWID datatype, this is the
* length in bytes. Null is returned for data types where the column size is not applicable.
*
* @param catalog a catalog name; must match the catalog name as it is stored in the database; ""
* retrieves those without a catalog; null
means that the catalog name
* should not be used to narrow the search
* @param schemaPattern a schema name pattern; must match the schema name as it is stored in the database; ""
* retrieves those without a schema; null
means that the schema name should
* not be used to narrow the search
* @param functionNamePattern a procedure name pattern; must match the function name as it is stored in the
* database
* @param columnNamePattern a parameter name pattern; must match the parameter or column name as it is stored in
* the database
* @return ResultSet
- each row describes a user function parameter, column or return type
* @throws java.sql.SQLException if a database access error occurs
* @see #getSearchStringEscape
* @since 1.6
*/
public ResultSet getFunctionColumns(final String catalog,
final String schemaPattern,
final String functionNamePattern,
final String columnNamePattern) throws SQLException {
throw SQLExceptionMapper.getSQLException("uh7");
}
/**
* Returns an object that implements the given interface to allow access to non-standard methods, or standard
* methods not exposed by the proxy.
*
* If the receiver implements the interface then the result is the receiver or a proxy for the receiver. If the
* receiver is a wrapper and the wrapped object implements the interface then the result is the wrapped object or a
* proxy for the wrapped object. Otherwise return the the result of calling unwrap
recursively on the
* wrapped object or a proxy for that result. If the receiver is not a wrapper and does not implement the interface,
* then an SQLException
is thrown.
*
* @param iface A Class defining an interface that the result must implement.
* @return an object that implements the interface. May be a proxy for the actual implementing object.
* @throws java.sql.SQLException If no object found that implements the interface
* @since 1.6
*/
public T unwrap(final Class iface) throws SQLException {
return null;
}
/**
* Returns true if this either implements the interface argument or is directly or indirectly a wrapper for an
* object that does. Returns false otherwise. If this implements the interface then return true, else if this is a
* wrapper then return the result of recursively calling isWrapperFor
on the wrapped object. If this
* does not implement the interface and is not a wrapper, return false. This method should be implemented as a
* low-cost operation compared to unwrap
so that callers can use this method to avoid expensive
* unwrap
calls that may fail. If this method returns true then calling unwrap
with the
* same argument should succeed.
*
* @param iface a Class defining an interface.
* @return true if this implements the interface or directly or indirectly wraps an object that does.
* @throws java.sql.SQLException if an error occurs while determining whether this is a wrapper for an object with
* the given interface.
* @since 1.6
*/
public boolean isWrapperFor(final Class> iface) throws SQLException {
return false;
}
public static final class Builder {
private String version;
private String url;
private String username;
private final Connection connection;
private final SupportedDatabases database;
private String databaseProductName;
public Builder(SupportedDatabases database, final Connection connection) {
this.connection = connection;
this.database = database;
}
public Builder version(final String version) {
this.version = version;
return this;
}
public Builder url(final String url) {
this.url = url;
return this;
}
public Builder username(final String username) {
this.username = username;
return this;
}
public Builder databaseProductName(final String name) {
this.databaseProductName = name;
return this;
}
public CommonDatabaseMetaData build() {
switch(database) {
case DRIZZLE: return new DrizzleDataBaseMetaData(this);
case MYSQL: return new MySQLDatabaseMetaData(this);
}
//return new CommonDatabaseMetaData(this);
throw new IllegalArgumentException("Unsupported database "+database);
}
}
protected CommonDatabaseMetaData(final Builder builder) {
this.version = builder.version;
this.url = builder.url;
this.username = builder.username;
this.connection = builder.connection;
this.databaseProductName = builder.databaseProductName;
}
}