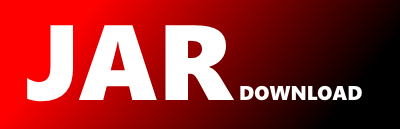
eu.agrosense.api.drmcrop.PhytophthoraFungicide Maven / Gradle / Ivy
//
// This file was generated by the JavaTM Architecture for XML Binding(JAXB) Reference Implementation, v2.2.6
// See http://java.sun.com/xml/jaxb
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2014.08.07 at 06:37:52 PM CEST
//
package eu.agrosense.api.drmcrop;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlType;
import org.jvnet.jaxb2_commons.lang.JAXBToStringStrategy;
import org.jvnet.jaxb2_commons.lang.ToString;
import org.jvnet.jaxb2_commons.lang.ToStringStrategy;
import org.jvnet.jaxb2_commons.locator.ObjectLocator;
/**
* Definition.
* A Fungicide which can be used for Phytophthora control.
*
* Java class for PhytophthoraFungicide complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="PhytophthoraFungicide">
* <complexContent>
* <extension base="{http://www.drmCrop.org/schemas/drmCrop}Fungicide">
* <sequence>
* <element name="RecommendedDose" type="{http://www.drmCrop.org/schemas/DataTypes}RateType"/>
* <element name="LeafBligthEffectiviness" type="{http://www.drmCrop.org/schemas/DataTypes}NumberValueType"/>
* <element name="NewGrowthEffectiviness" type="{http://www.drmCrop.org/schemas/DataTypes}NumberValueType"/>
* <element name="StemBlightEffectiviness" type="{http://www.drmCrop.org/schemas/DataTypes}NumberValueType"/>
* <element name="TuberBlightEffectiviness" type="{http://www.drmCrop.org/schemas/DataTypes}NumberValueType"/>
* <element name="Protectant" type="{http://www.drmCrop.org/schemas/DataTypes}NumberValueType"/>
* <element name="Curative" type="{http://www.drmCrop.org/schemas/DataTypes}NumberValueType"/>
* <element name="AntiSporulant" type="{http://www.drmCrop.org/schemas/DataTypes}NumberValueType"/>
* <element name="Rainfastness" type="{http://www.drmCrop.org/schemas/DataTypes}NumberValueType"/>
* <element name="MobilityInThePlant" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="PhytophthoraProtectionCategory" type="{http://www.drmCrop.org/schemas/drmCrop}PhytophthoraProtectionCategory"/>
* </sequence>
* </extension>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "PhytophthoraFungicide", propOrder = {
"recommendedDose",
"leafBligthEffectiviness",
"newGrowthEffectiviness",
"stemBlightEffectiviness",
"tuberBlightEffectiviness",
"protectant",
"curative",
"antiSporulant",
"rainfastness",
"mobilityInThePlant",
"phytophthoraProtectionCategory"
})
public class PhytophthoraFungicide
extends Fungicide
implements ToString
{
@XmlElement(name = "RecommendedDose", required = true)
protected RateType recommendedDose;
@XmlElement(name = "LeafBligthEffectiviness", required = true)
protected NumberValueType leafBligthEffectiviness;
@XmlElement(name = "NewGrowthEffectiviness", required = true)
protected NumberValueType newGrowthEffectiviness;
@XmlElement(name = "StemBlightEffectiviness", required = true)
protected NumberValueType stemBlightEffectiviness;
@XmlElement(name = "TuberBlightEffectiviness", required = true)
protected NumberValueType tuberBlightEffectiviness;
@XmlElement(name = "Protectant", required = true)
protected NumberValueType protectant;
@XmlElement(name = "Curative", required = true)
protected NumberValueType curative;
@XmlElement(name = "AntiSporulant", required = true)
protected NumberValueType antiSporulant;
@XmlElement(name = "Rainfastness", required = true)
protected NumberValueType rainfastness;
@XmlElement(name = "MobilityInThePlant", required = true)
protected String mobilityInThePlant;
@XmlElement(name = "PhytophthoraProtectionCategory", required = true)
protected PhytophthoraProtectionCategory phytophthoraProtectionCategory;
/**
* Gets the value of the recommendedDose property.
*
* @return
* possible object is
* {@link RateType }
*
*/
public RateType getRecommendedDose() {
return recommendedDose;
}
/**
* Sets the value of the recommendedDose property.
*
* @param value
* allowed object is
* {@link RateType }
*
*/
public void setRecommendedDose(RateType value) {
this.recommendedDose = value;
}
/**
* Gets the value of the leafBligthEffectiviness property.
*
* @return
* possible object is
* {@link NumberValueType }
*
*/
public NumberValueType getLeafBligthEffectiviness() {
return leafBligthEffectiviness;
}
/**
* Sets the value of the leafBligthEffectiviness property.
*
* @param value
* allowed object is
* {@link NumberValueType }
*
*/
public void setLeafBligthEffectiviness(NumberValueType value) {
this.leafBligthEffectiviness = value;
}
/**
* Gets the value of the newGrowthEffectiviness property.
*
* @return
* possible object is
* {@link NumberValueType }
*
*/
public NumberValueType getNewGrowthEffectiviness() {
return newGrowthEffectiviness;
}
/**
* Sets the value of the newGrowthEffectiviness property.
*
* @param value
* allowed object is
* {@link NumberValueType }
*
*/
public void setNewGrowthEffectiviness(NumberValueType value) {
this.newGrowthEffectiviness = value;
}
/**
* Gets the value of the stemBlightEffectiviness property.
*
* @return
* possible object is
* {@link NumberValueType }
*
*/
public NumberValueType getStemBlightEffectiviness() {
return stemBlightEffectiviness;
}
/**
* Sets the value of the stemBlightEffectiviness property.
*
* @param value
* allowed object is
* {@link NumberValueType }
*
*/
public void setStemBlightEffectiviness(NumberValueType value) {
this.stemBlightEffectiviness = value;
}
/**
* Gets the value of the tuberBlightEffectiviness property.
*
* @return
* possible object is
* {@link NumberValueType }
*
*/
public NumberValueType getTuberBlightEffectiviness() {
return tuberBlightEffectiviness;
}
/**
* Sets the value of the tuberBlightEffectiviness property.
*
* @param value
* allowed object is
* {@link NumberValueType }
*
*/
public void setTuberBlightEffectiviness(NumberValueType value) {
this.tuberBlightEffectiviness = value;
}
/**
* Gets the value of the protectant property.
*
* @return
* possible object is
* {@link NumberValueType }
*
*/
public NumberValueType getProtectant() {
return protectant;
}
/**
* Sets the value of the protectant property.
*
* @param value
* allowed object is
* {@link NumberValueType }
*
*/
public void setProtectant(NumberValueType value) {
this.protectant = value;
}
/**
* Gets the value of the curative property.
*
* @return
* possible object is
* {@link NumberValueType }
*
*/
public NumberValueType getCurative() {
return curative;
}
/**
* Sets the value of the curative property.
*
* @param value
* allowed object is
* {@link NumberValueType }
*
*/
public void setCurative(NumberValueType value) {
this.curative = value;
}
/**
* Gets the value of the antiSporulant property.
*
* @return
* possible object is
* {@link NumberValueType }
*
*/
public NumberValueType getAntiSporulant() {
return antiSporulant;
}
/**
* Sets the value of the antiSporulant property.
*
* @param value
* allowed object is
* {@link NumberValueType }
*
*/
public void setAntiSporulant(NumberValueType value) {
this.antiSporulant = value;
}
/**
* Gets the value of the rainfastness property.
*
* @return
* possible object is
* {@link NumberValueType }
*
*/
public NumberValueType getRainfastness() {
return rainfastness;
}
/**
* Sets the value of the rainfastness property.
*
* @param value
* allowed object is
* {@link NumberValueType }
*
*/
public void setRainfastness(NumberValueType value) {
this.rainfastness = value;
}
/**
* Gets the value of the mobilityInThePlant property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getMobilityInThePlant() {
return mobilityInThePlant;
}
/**
* Sets the value of the mobilityInThePlant property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setMobilityInThePlant(String value) {
this.mobilityInThePlant = value;
}
/**
* Gets the value of the phytophthoraProtectionCategory property.
*
* @return
* possible object is
* {@link PhytophthoraProtectionCategory }
*
*/
public PhytophthoraProtectionCategory getPhytophthoraProtectionCategory() {
return phytophthoraProtectionCategory;
}
/**
* Sets the value of the phytophthoraProtectionCategory property.
*
* @param value
* allowed object is
* {@link PhytophthoraProtectionCategory }
*
*/
public void setPhytophthoraProtectionCategory(PhytophthoraProtectionCategory value) {
this.phytophthoraProtectionCategory = value;
}
public String toString() {
final ToStringStrategy strategy = JAXBToStringStrategy.INSTANCE;
final StringBuilder buffer = new StringBuilder();
append(null, buffer, strategy);
return buffer.toString();
}
public StringBuilder append(ObjectLocator locator, StringBuilder buffer, ToStringStrategy strategy) {
strategy.appendStart(locator, this, buffer);
appendFields(locator, buffer, strategy);
strategy.appendEnd(locator, this, buffer);
return buffer;
}
public StringBuilder appendFields(ObjectLocator locator, StringBuilder buffer, ToStringStrategy strategy) {
super.appendFields(locator, buffer, strategy);
{
RateType theRecommendedDose;
theRecommendedDose = this.getRecommendedDose();
strategy.appendField(locator, this, "recommendedDose", buffer, theRecommendedDose);
}
{
NumberValueType theLeafBligthEffectiviness;
theLeafBligthEffectiviness = this.getLeafBligthEffectiviness();
strategy.appendField(locator, this, "leafBligthEffectiviness", buffer, theLeafBligthEffectiviness);
}
{
NumberValueType theNewGrowthEffectiviness;
theNewGrowthEffectiviness = this.getNewGrowthEffectiviness();
strategy.appendField(locator, this, "newGrowthEffectiviness", buffer, theNewGrowthEffectiviness);
}
{
NumberValueType theStemBlightEffectiviness;
theStemBlightEffectiviness = this.getStemBlightEffectiviness();
strategy.appendField(locator, this, "stemBlightEffectiviness", buffer, theStemBlightEffectiviness);
}
{
NumberValueType theTuberBlightEffectiviness;
theTuberBlightEffectiviness = this.getTuberBlightEffectiviness();
strategy.appendField(locator, this, "tuberBlightEffectiviness", buffer, theTuberBlightEffectiviness);
}
{
NumberValueType theProtectant;
theProtectant = this.getProtectant();
strategy.appendField(locator, this, "protectant", buffer, theProtectant);
}
{
NumberValueType theCurative;
theCurative = this.getCurative();
strategy.appendField(locator, this, "curative", buffer, theCurative);
}
{
NumberValueType theAntiSporulant;
theAntiSporulant = this.getAntiSporulant();
strategy.appendField(locator, this, "antiSporulant", buffer, theAntiSporulant);
}
{
NumberValueType theRainfastness;
theRainfastness = this.getRainfastness();
strategy.appendField(locator, this, "rainfastness", buffer, theRainfastness);
}
{
String theMobilityInThePlant;
theMobilityInThePlant = this.getMobilityInThePlant();
strategy.appendField(locator, this, "mobilityInThePlant", buffer, theMobilityInThePlant);
}
{
PhytophthoraProtectionCategory thePhytophthoraProtectionCategory;
thePhytophthoraProtectionCategory = this.getPhytophthoraProtectionCategory();
strategy.appendField(locator, this, "phytophthoraProtectionCategory", buffer, thePhytophthoraProtectionCategory);
}
return buffer;
}
public PhytophthoraFungicide withRecommendedDose(RateType value) {
setRecommendedDose(value);
return this;
}
public PhytophthoraFungicide withLeafBligthEffectiviness(NumberValueType value) {
setLeafBligthEffectiviness(value);
return this;
}
public PhytophthoraFungicide withNewGrowthEffectiviness(NumberValueType value) {
setNewGrowthEffectiviness(value);
return this;
}
public PhytophthoraFungicide withStemBlightEffectiviness(NumberValueType value) {
setStemBlightEffectiviness(value);
return this;
}
public PhytophthoraFungicide withTuberBlightEffectiviness(NumberValueType value) {
setTuberBlightEffectiviness(value);
return this;
}
public PhytophthoraFungicide withProtectant(NumberValueType value) {
setProtectant(value);
return this;
}
public PhytophthoraFungicide withCurative(NumberValueType value) {
setCurative(value);
return this;
}
public PhytophthoraFungicide withAntiSporulant(NumberValueType value) {
setAntiSporulant(value);
return this;
}
public PhytophthoraFungicide withRainfastness(NumberValueType value) {
setRainfastness(value);
return this;
}
public PhytophthoraFungicide withMobilityInThePlant(String value) {
setMobilityInThePlant(value);
return this;
}
public PhytophthoraFungicide withPhytophthoraProtectionCategory(PhytophthoraProtectionCategory value) {
setPhytophthoraProtectionCategory(value);
return this;
}
@Override
public PhytophthoraFungicide withPPProductFunction(CodeType value) {
setPPProductFunction(value);
return this;
}
@Override
public PhytophthoraFungicide withPPProductOtherFunction(String value) {
setPPProductOtherFunction(value);
return this;
}
@Override
public PhytophthoraFungicide withFormulationCode(String value) {
setFormulationCode(value);
return this;
}
@Override
public PhytophthoraFungicide withFormulationTypeCode(CodeType value) {
setFormulationTypeCode(value);
return this;
}
@Override
public PhytophthoraFungicide withTypeOfUser(TypeOfPPPUserEnumeration value) {
setTypeOfUser(value);
return this;
}
@Override
public PhytophthoraFungicide withProductID(String value) {
setProductID(value);
return this;
}
@Override
public PhytophthoraFungicide withProductCode(CodeType value) {
setProductCode(value);
return this;
}
@Override
public PhytophthoraFungicide withProductDesignator(String value) {
setProductDesignator(value);
return this;
}
@Override
public PhytophthoraFungicide withApplicationRateUnit(RateUnitType value) {
setApplicationRateUnit(value);
return this;
}
@Override
public PhytophthoraFungicide withProductForm(ProductFormEnumeration value) {
setProductForm(value);
return this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy