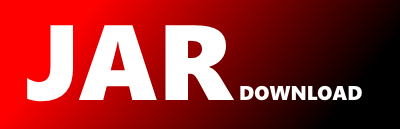
org.drmcrop.AbsoluteTiming Maven / Gradle / Ivy
//
// This file was generated by the JavaTM Architecture for XML Binding(JAXB) Reference Implementation, v2.2.6
// See http://java.sun.com/xml/jaxb
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2014.10.22 at 06:25:25 PM CEST
//
package org.drmcrop;
import java.math.BigDecimal;
import java.util.ArrayList;
import java.util.Collection;
import java.util.List;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlID;
import javax.xml.bind.annotation.XmlSchemaType;
import javax.xml.bind.annotation.XmlType;
import javax.xml.bind.annotation.adapters.CollapsedStringAdapter;
import javax.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
import javax.xml.datatype.XMLGregorianCalendar;
import org.jvnet.jaxb2_commons.lang.JAXBToStringStrategy;
import org.jvnet.jaxb2_commons.lang.ToString;
import org.jvnet.jaxb2_commons.lang.ToStringStrategy;
import org.jvnet.jaxb2_commons.locator.ObjectLocator;
/**
* Definition.
* A time period during which a particular Operation is planned to be, or was, executed following a certain specification.
*
* Remark.
* One Operation can be executed in several time periods. It is possible to assign an urgency or timeliness value to each period.
*
* Apart from AbsoluteTiming it is possible to specify a RelativeTiming. One can specify for example that a certain fertilizer gift has to be done three to two weeks before planting.
*
* Apart from AbsoluteTiming it is possible for Task's and Job's to specify one or more TimeFrame's. AbsoluteTiming has a more agronomical meaning, while TimeFrame has a more (machinery) management meaning.
*
* Java class for AbsoluteTiming complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="AbsoluteTiming">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="AbsoluteTimingID" type="{http://www.w3.org/2001/XMLSchema}ID"/>
* <element name="BeginDateTime" type="{http://www.w3.org/2001/XMLSchema}dateTime"/>
* <element name="EndDateTime" type="{http://www.w3.org/2001/XMLSchema}dateTime"/>
* <element name="AbsoluteTimingStatus" type="{http://www.drmCrop.org/schemas/Enumerations}StatusEnumeration"/>
* <element name="TimelinesValue" type="{http://www.w3.org/2001/XMLSchema}decimal" minOccurs="0"/>
* <element name="Allocation" type="{http://www.drmCrop.org/schemas/drmCrop}Allocation" maxOccurs="unbounded" minOccurs="0"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "AbsoluteTiming", propOrder = {
"absoluteTimingID",
"beginDateTime",
"endDateTime",
"absoluteTimingStatus",
"timelinesValue",
"allocation"
})
public class AbsoluteTiming
implements ToString
{
@XmlElement(name = "AbsoluteTimingID", required = true)
@XmlJavaTypeAdapter(CollapsedStringAdapter.class)
@XmlID
@XmlSchemaType(name = "ID")
protected String absoluteTimingID;
@XmlElement(name = "BeginDateTime", required = true)
@XmlSchemaType(name = "dateTime")
protected XMLGregorianCalendar beginDateTime;
@XmlElement(name = "EndDateTime", required = true)
@XmlSchemaType(name = "dateTime")
protected XMLGregorianCalendar endDateTime;
@XmlElement(name = "AbsoluteTimingStatus", required = true)
protected StatusEnumeration absoluteTimingStatus;
@XmlElement(name = "TimelinesValue")
protected BigDecimal timelinesValue;
@XmlElement(name = "Allocation")
protected List allocation;
/**
* Gets the value of the absoluteTimingID property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getAbsoluteTimingID() {
return absoluteTimingID;
}
/**
* Sets the value of the absoluteTimingID property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setAbsoluteTimingID(String value) {
this.absoluteTimingID = value;
}
/**
* Gets the value of the beginDateTime property.
*
* @return
* possible object is
* {@link XMLGregorianCalendar }
*
*/
public XMLGregorianCalendar getBeginDateTime() {
return beginDateTime;
}
/**
* Sets the value of the beginDateTime property.
*
* @param value
* allowed object is
* {@link XMLGregorianCalendar }
*
*/
public void setBeginDateTime(XMLGregorianCalendar value) {
this.beginDateTime = value;
}
/**
* Gets the value of the endDateTime property.
*
* @return
* possible object is
* {@link XMLGregorianCalendar }
*
*/
public XMLGregorianCalendar getEndDateTime() {
return endDateTime;
}
/**
* Sets the value of the endDateTime property.
*
* @param value
* allowed object is
* {@link XMLGregorianCalendar }
*
*/
public void setEndDateTime(XMLGregorianCalendar value) {
this.endDateTime = value;
}
/**
* Gets the value of the absoluteTimingStatus property.
*
* @return
* possible object is
* {@link StatusEnumeration }
*
*/
public StatusEnumeration getAbsoluteTimingStatus() {
return absoluteTimingStatus;
}
/**
* Sets the value of the absoluteTimingStatus property.
*
* @param value
* allowed object is
* {@link StatusEnumeration }
*
*/
public void setAbsoluteTimingStatus(StatusEnumeration value) {
this.absoluteTimingStatus = value;
}
/**
* Gets the value of the timelinesValue property.
*
* @return
* possible object is
* {@link BigDecimal }
*
*/
public BigDecimal getTimelinesValue() {
return timelinesValue;
}
/**
* Sets the value of the timelinesValue property.
*
* @param value
* allowed object is
* {@link BigDecimal }
*
*/
public void setTimelinesValue(BigDecimal value) {
this.timelinesValue = value;
}
/**
* Gets the value of the allocation property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the allocation property.
*
*
* For example, to add a new item, do as follows:
*
* getAllocation().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Allocation }
*
*
*/
public List getAllocation() {
if (allocation == null) {
allocation = new ArrayList();
}
return this.allocation;
}
public String toString() {
final ToStringStrategy strategy = JAXBToStringStrategy.INSTANCE;
final StringBuilder buffer = new StringBuilder();
append(null, buffer, strategy);
return buffer.toString();
}
public StringBuilder append(ObjectLocator locator, StringBuilder buffer, ToStringStrategy strategy) {
strategy.appendStart(locator, this, buffer);
appendFields(locator, buffer, strategy);
strategy.appendEnd(locator, this, buffer);
return buffer;
}
public StringBuilder appendFields(ObjectLocator locator, StringBuilder buffer, ToStringStrategy strategy) {
{
String theAbsoluteTimingID;
theAbsoluteTimingID = this.getAbsoluteTimingID();
strategy.appendField(locator, this, "absoluteTimingID", buffer, theAbsoluteTimingID);
}
{
XMLGregorianCalendar theBeginDateTime;
theBeginDateTime = this.getBeginDateTime();
strategy.appendField(locator, this, "beginDateTime", buffer, theBeginDateTime);
}
{
XMLGregorianCalendar theEndDateTime;
theEndDateTime = this.getEndDateTime();
strategy.appendField(locator, this, "endDateTime", buffer, theEndDateTime);
}
{
StatusEnumeration theAbsoluteTimingStatus;
theAbsoluteTimingStatus = this.getAbsoluteTimingStatus();
strategy.appendField(locator, this, "absoluteTimingStatus", buffer, theAbsoluteTimingStatus);
}
{
BigDecimal theTimelinesValue;
theTimelinesValue = this.getTimelinesValue();
strategy.appendField(locator, this, "timelinesValue", buffer, theTimelinesValue);
}
{
List theAllocation;
theAllocation = (((this.allocation!= null)&&(!this.allocation.isEmpty()))?this.getAllocation():null);
strategy.appendField(locator, this, "allocation", buffer, theAllocation);
}
return buffer;
}
public AbsoluteTiming withAbsoluteTimingID(String value) {
setAbsoluteTimingID(value);
return this;
}
public AbsoluteTiming withBeginDateTime(XMLGregorianCalendar value) {
setBeginDateTime(value);
return this;
}
public AbsoluteTiming withEndDateTime(XMLGregorianCalendar value) {
setEndDateTime(value);
return this;
}
public AbsoluteTiming withAbsoluteTimingStatus(StatusEnumeration value) {
setAbsoluteTimingStatus(value);
return this;
}
public AbsoluteTiming withTimelinesValue(BigDecimal value) {
setTimelinesValue(value);
return this;
}
public AbsoluteTiming withAllocation(Allocation... values) {
if (values!= null) {
for (Allocation value: values) {
getAllocation().add(value);
}
}
return this;
}
public AbsoluteTiming withAllocation(Collection values) {
if (values!= null) {
getAllocation().addAll(values);
}
return this;
}
}