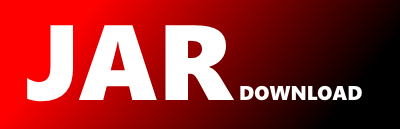
org.drmcrop.CropClass Maven / Gradle / Ivy
//
// This file was generated by the JavaTM Architecture for XML Binding(JAXB) Reference Implementation, v2.2.6
// See http://java.sun.com/xml/jaxb
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2014.10.22 at 06:25:25 PM CEST
//
package org.drmcrop;
import java.util.ArrayList;
import java.util.Collection;
import java.util.List;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlID;
import javax.xml.bind.annotation.XmlSchemaType;
import javax.xml.bind.annotation.XmlType;
import javax.xml.bind.annotation.adapters.CollapsedStringAdapter;
import javax.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
import org.jvnet.jaxb2_commons.lang.JAXBToStringStrategy;
import org.jvnet.jaxb2_commons.lang.ToString;
import org.jvnet.jaxb2_commons.lang.ToStringStrategy;
import org.jvnet.jaxb2_commons.locator.ObjectLocator;
/**
* Description.
* The Classification of crops that are distinguished within an organization.
*
* Remark.
* Organizations might have different levels of detail by which CropClasses are distinguished. For some organizations "potatoes" might be sufficient, but other want to distinguish between "seed potatoes', "starch potatoes" and "consumption potatoes", while others will separate the consumption potatoes in "table potatoes" and "Potatoes for french fries".
* In the Netherlands the EDI-Teelt coding list and their designators are used for communication to other parties, but organizations are free to make their own subdivision.
*
* Java class for CropClass complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="CropClass">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="CropClassID" type="{http://www.w3.org/2001/XMLSchema}ID"/>
* <element name="CropClassCode" type="{http://www.drmCrop.org/schemas/DataTypes}DrmCropCodeType"/>
* <element name="CropClassDesignator" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="ScientificName" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="FamilyName" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="Variety" type="{http://www.drmCrop.org/schemas/drmCrop}Variety" maxOccurs="unbounded"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "CropClass", propOrder = {
"cropClassID",
"cropClassCode",
"cropClassDesignator",
"scientificName",
"familyName",
"variety"
})
public class CropClass
implements ToString
{
@XmlElement(name = "CropClassID", required = true)
@XmlJavaTypeAdapter(CollapsedStringAdapter.class)
@XmlID
@XmlSchemaType(name = "ID")
protected String cropClassID;
@XmlElement(name = "CropClassCode", required = true)
protected DrmCropCodeType cropClassCode;
@XmlElement(name = "CropClassDesignator", required = true)
protected String cropClassDesignator;
@XmlElement(name = "ScientificName", required = true)
protected String scientificName;
@XmlElement(name = "FamilyName", required = true)
protected String familyName;
@XmlElement(name = "Variety", required = true)
protected List variety;
/**
* Gets the value of the cropClassID property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getCropClassID() {
return cropClassID;
}
/**
* Sets the value of the cropClassID property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setCropClassID(String value) {
this.cropClassID = value;
}
/**
* Gets the value of the cropClassCode property.
*
* @return
* possible object is
* {@link DrmCropCodeType }
*
*/
public DrmCropCodeType getCropClassCode() {
return cropClassCode;
}
/**
* Sets the value of the cropClassCode property.
*
* @param value
* allowed object is
* {@link DrmCropCodeType }
*
*/
public void setCropClassCode(DrmCropCodeType value) {
this.cropClassCode = value;
}
/**
* Gets the value of the cropClassDesignator property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getCropClassDesignator() {
return cropClassDesignator;
}
/**
* Sets the value of the cropClassDesignator property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setCropClassDesignator(String value) {
this.cropClassDesignator = value;
}
/**
* Gets the value of the scientificName property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getScientificName() {
return scientificName;
}
/**
* Sets the value of the scientificName property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setScientificName(String value) {
this.scientificName = value;
}
/**
* Gets the value of the familyName property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getFamilyName() {
return familyName;
}
/**
* Sets the value of the familyName property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setFamilyName(String value) {
this.familyName = value;
}
/**
* Gets the value of the variety property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the variety property.
*
*
* For example, to add a new item, do as follows:
*
* getVariety().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Variety }
*
*
*/
public List getVariety() {
if (variety == null) {
variety = new ArrayList();
}
return this.variety;
}
public String toString() {
final ToStringStrategy strategy = JAXBToStringStrategy.INSTANCE;
final StringBuilder buffer = new StringBuilder();
append(null, buffer, strategy);
return buffer.toString();
}
public StringBuilder append(ObjectLocator locator, StringBuilder buffer, ToStringStrategy strategy) {
strategy.appendStart(locator, this, buffer);
appendFields(locator, buffer, strategy);
strategy.appendEnd(locator, this, buffer);
return buffer;
}
public StringBuilder appendFields(ObjectLocator locator, StringBuilder buffer, ToStringStrategy strategy) {
{
String theCropClassID;
theCropClassID = this.getCropClassID();
strategy.appendField(locator, this, "cropClassID", buffer, theCropClassID);
}
{
DrmCropCodeType theCropClassCode;
theCropClassCode = this.getCropClassCode();
strategy.appendField(locator, this, "cropClassCode", buffer, theCropClassCode);
}
{
String theCropClassDesignator;
theCropClassDesignator = this.getCropClassDesignator();
strategy.appendField(locator, this, "cropClassDesignator", buffer, theCropClassDesignator);
}
{
String theScientificName;
theScientificName = this.getScientificName();
strategy.appendField(locator, this, "scientificName", buffer, theScientificName);
}
{
String theFamilyName;
theFamilyName = this.getFamilyName();
strategy.appendField(locator, this, "familyName", buffer, theFamilyName);
}
{
List theVariety;
theVariety = (((this.variety!= null)&&(!this.variety.isEmpty()))?this.getVariety():null);
strategy.appendField(locator, this, "variety", buffer, theVariety);
}
return buffer;
}
public CropClass withCropClassID(String value) {
setCropClassID(value);
return this;
}
public CropClass withCropClassCode(DrmCropCodeType value) {
setCropClassCode(value);
return this;
}
public CropClass withCropClassDesignator(String value) {
setCropClassDesignator(value);
return this;
}
public CropClass withScientificName(String value) {
setScientificName(value);
return this;
}
public CropClass withFamilyName(String value) {
setFamilyName(value);
return this;
}
public CropClass withVariety(Variety... values) {
if (values!= null) {
for (Variety value: values) {
getVariety().add(value);
}
}
return this;
}
public CropClass withVariety(Collection values) {
if (values!= null) {
getVariety().addAll(values);
}
return this;
}
}