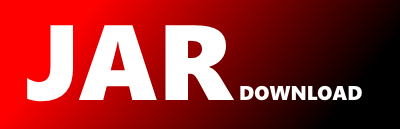
org.drmcrop.PropertyVariable Maven / Gradle / Ivy
//
// This file was generated by the JavaTM Architecture for XML Binding(JAXB) Reference Implementation, v2.2.6
// See http://java.sun.com/xml/jaxb
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2014.10.22 at 06:25:25 PM CEST
//
package org.drmcrop;
import java.math.BigDecimal;
import java.util.ArrayList;
import java.util.Collection;
import java.util.List;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlID;
import javax.xml.bind.annotation.XmlSchemaType;
import javax.xml.bind.annotation.XmlType;
import javax.xml.bind.annotation.adapters.CollapsedStringAdapter;
import javax.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
import org.jvnet.jaxb2_commons.lang.JAXBToStringStrategy;
import org.jvnet.jaxb2_commons.lang.ToString;
import org.jvnet.jaxb2_commons.lang.ToStringStrategy;
import org.jvnet.jaxb2_commons.locator.ObjectLocator;
/**
* Description.
* The PropertyVariable defines a variable which can be measured by a sensor (Operation) , is the result of a (laboratory) analyses or the result of a calculation (DataProcess) . It can be used to describe properties of an Object. Objects can be Field, PartField, PropertyZone, VerticalLayer, (Batch of)Product, (Batch of)Produce, etc.
*
* Remark.
* Lists of PropertyVariables are maintained by a standardization organization. AgroConnect is such an organization and maintains lists for several PropertyCategory's. For Some Propertycategory's use is made of standards maintained by other standardization organizations like UN/CEFACT. These organizations are referenced in the PropertyCategory.
*
* Java class for PropertyVariable complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="PropertyVariable">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="PropertyVariableID" type="{http://www.w3.org/2001/XMLSchema}ID"/>
* <element name="PropertyVariableCode" type="{http://www.drmCrop.org/schemas/DataTypes}DrmCropCodeType" maxOccurs="unbounded"/>
* <element name="PropertyVariableDesignator" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="ExtendedDesignator" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="MinimumValue" type="{http://www.w3.org/2001/XMLSchema}decimal" minOccurs="0"/>
* <element name="MaximumValue" type="{http://www.w3.org/2001/XMLSchema}decimal" minOccurs="0"/>
* <element name="Definition" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="ValueEnumerator" type="{http://www.drmCrop.org/schemas/Enumerations}ValueEnumeration"/>
* <element name="NumeratorUom" type="{http://www.drmCrop.org/schemas/DataTypes}DrmCropCodeType" minOccurs="0"/>
* <element name="DenumeratorUom" type="{http://www.drmCrop.org/schemas/DataTypes}DrmCropCodeType" minOccurs="0"/>
* <element name="SecondDenumeratorUom" type="{http://www.drmCrop.org/schemas/DataTypes}DrmCropCodeType" minOccurs="0"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "PropertyVariable", propOrder = {
"propertyVariableID",
"propertyVariableCode",
"propertyVariableDesignator",
"extendedDesignator",
"minimumValue",
"maximumValue",
"definition",
"valueEnumerator",
"numeratorUom",
"denumeratorUom",
"secondDenumeratorUom"
})
public class PropertyVariable
implements ToString
{
@XmlElement(name = "PropertyVariableID", required = true)
@XmlJavaTypeAdapter(CollapsedStringAdapter.class)
@XmlID
@XmlSchemaType(name = "ID")
protected String propertyVariableID;
@XmlElement(name = "PropertyVariableCode", required = true)
protected List propertyVariableCode;
@XmlElement(name = "PropertyVariableDesignator", required = true)
protected String propertyVariableDesignator;
@XmlElement(name = "ExtendedDesignator", required = true)
protected String extendedDesignator;
@XmlElement(name = "MinimumValue")
protected BigDecimal minimumValue;
@XmlElement(name = "MaximumValue")
protected BigDecimal maximumValue;
@XmlElement(name = "Definition", required = true)
protected String definition;
@XmlElement(name = "ValueEnumerator", required = true)
protected ValueEnumeration valueEnumerator;
@XmlElement(name = "NumeratorUom")
protected DrmCropCodeType numeratorUom;
@XmlElement(name = "DenumeratorUom")
protected DrmCropCodeType denumeratorUom;
@XmlElement(name = "SecondDenumeratorUom")
protected DrmCropCodeType secondDenumeratorUom;
/**
* Gets the value of the propertyVariableID property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getPropertyVariableID() {
return propertyVariableID;
}
/**
* Sets the value of the propertyVariableID property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setPropertyVariableID(String value) {
this.propertyVariableID = value;
}
/**
* Gets the value of the propertyVariableCode property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the propertyVariableCode property.
*
*
* For example, to add a new item, do as follows:
*
* getPropertyVariableCode().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link DrmCropCodeType }
*
*
*/
public List getPropertyVariableCode() {
if (propertyVariableCode == null) {
propertyVariableCode = new ArrayList();
}
return this.propertyVariableCode;
}
/**
* Gets the value of the propertyVariableDesignator property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getPropertyVariableDesignator() {
return propertyVariableDesignator;
}
/**
* Sets the value of the propertyVariableDesignator property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setPropertyVariableDesignator(String value) {
this.propertyVariableDesignator = value;
}
/**
* Gets the value of the extendedDesignator property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getExtendedDesignator() {
return extendedDesignator;
}
/**
* Sets the value of the extendedDesignator property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setExtendedDesignator(String value) {
this.extendedDesignator = value;
}
/**
* Gets the value of the minimumValue property.
*
* @return
* possible object is
* {@link BigDecimal }
*
*/
public BigDecimal getMinimumValue() {
return minimumValue;
}
/**
* Sets the value of the minimumValue property.
*
* @param value
* allowed object is
* {@link BigDecimal }
*
*/
public void setMinimumValue(BigDecimal value) {
this.minimumValue = value;
}
/**
* Gets the value of the maximumValue property.
*
* @return
* possible object is
* {@link BigDecimal }
*
*/
public BigDecimal getMaximumValue() {
return maximumValue;
}
/**
* Sets the value of the maximumValue property.
*
* @param value
* allowed object is
* {@link BigDecimal }
*
*/
public void setMaximumValue(BigDecimal value) {
this.maximumValue = value;
}
/**
* Gets the value of the definition property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getDefinition() {
return definition;
}
/**
* Sets the value of the definition property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setDefinition(String value) {
this.definition = value;
}
/**
* Gets the value of the valueEnumerator property.
*
* @return
* possible object is
* {@link ValueEnumeration }
*
*/
public ValueEnumeration getValueEnumerator() {
return valueEnumerator;
}
/**
* Sets the value of the valueEnumerator property.
*
* @param value
* allowed object is
* {@link ValueEnumeration }
*
*/
public void setValueEnumerator(ValueEnumeration value) {
this.valueEnumerator = value;
}
/**
* Gets the value of the numeratorUom property.
*
* @return
* possible object is
* {@link DrmCropCodeType }
*
*/
public DrmCropCodeType getNumeratorUom() {
return numeratorUom;
}
/**
* Sets the value of the numeratorUom property.
*
* @param value
* allowed object is
* {@link DrmCropCodeType }
*
*/
public void setNumeratorUom(DrmCropCodeType value) {
this.numeratorUom = value;
}
/**
* Gets the value of the denumeratorUom property.
*
* @return
* possible object is
* {@link DrmCropCodeType }
*
*/
public DrmCropCodeType getDenumeratorUom() {
return denumeratorUom;
}
/**
* Sets the value of the denumeratorUom property.
*
* @param value
* allowed object is
* {@link DrmCropCodeType }
*
*/
public void setDenumeratorUom(DrmCropCodeType value) {
this.denumeratorUom = value;
}
/**
* Gets the value of the secondDenumeratorUom property.
*
* @return
* possible object is
* {@link DrmCropCodeType }
*
*/
public DrmCropCodeType getSecondDenumeratorUom() {
return secondDenumeratorUom;
}
/**
* Sets the value of the secondDenumeratorUom property.
*
* @param value
* allowed object is
* {@link DrmCropCodeType }
*
*/
public void setSecondDenumeratorUom(DrmCropCodeType value) {
this.secondDenumeratorUom = value;
}
public String toString() {
final ToStringStrategy strategy = JAXBToStringStrategy.INSTANCE;
final StringBuilder buffer = new StringBuilder();
append(null, buffer, strategy);
return buffer.toString();
}
public StringBuilder append(ObjectLocator locator, StringBuilder buffer, ToStringStrategy strategy) {
strategy.appendStart(locator, this, buffer);
appendFields(locator, buffer, strategy);
strategy.appendEnd(locator, this, buffer);
return buffer;
}
public StringBuilder appendFields(ObjectLocator locator, StringBuilder buffer, ToStringStrategy strategy) {
{
String thePropertyVariableID;
thePropertyVariableID = this.getPropertyVariableID();
strategy.appendField(locator, this, "propertyVariableID", buffer, thePropertyVariableID);
}
{
List thePropertyVariableCode;
thePropertyVariableCode = (((this.propertyVariableCode!= null)&&(!this.propertyVariableCode.isEmpty()))?this.getPropertyVariableCode():null);
strategy.appendField(locator, this, "propertyVariableCode", buffer, thePropertyVariableCode);
}
{
String thePropertyVariableDesignator;
thePropertyVariableDesignator = this.getPropertyVariableDesignator();
strategy.appendField(locator, this, "propertyVariableDesignator", buffer, thePropertyVariableDesignator);
}
{
String theExtendedDesignator;
theExtendedDesignator = this.getExtendedDesignator();
strategy.appendField(locator, this, "extendedDesignator", buffer, theExtendedDesignator);
}
{
BigDecimal theMinimumValue;
theMinimumValue = this.getMinimumValue();
strategy.appendField(locator, this, "minimumValue", buffer, theMinimumValue);
}
{
BigDecimal theMaximumValue;
theMaximumValue = this.getMaximumValue();
strategy.appendField(locator, this, "maximumValue", buffer, theMaximumValue);
}
{
String theDefinition;
theDefinition = this.getDefinition();
strategy.appendField(locator, this, "definition", buffer, theDefinition);
}
{
ValueEnumeration theValueEnumerator;
theValueEnumerator = this.getValueEnumerator();
strategy.appendField(locator, this, "valueEnumerator", buffer, theValueEnumerator);
}
{
DrmCropCodeType theNumeratorUom;
theNumeratorUom = this.getNumeratorUom();
strategy.appendField(locator, this, "numeratorUom", buffer, theNumeratorUom);
}
{
DrmCropCodeType theDenumeratorUom;
theDenumeratorUom = this.getDenumeratorUom();
strategy.appendField(locator, this, "denumeratorUom", buffer, theDenumeratorUom);
}
{
DrmCropCodeType theSecondDenumeratorUom;
theSecondDenumeratorUom = this.getSecondDenumeratorUom();
strategy.appendField(locator, this, "secondDenumeratorUom", buffer, theSecondDenumeratorUom);
}
return buffer;
}
public PropertyVariable withPropertyVariableID(String value) {
setPropertyVariableID(value);
return this;
}
public PropertyVariable withPropertyVariableCode(DrmCropCodeType... values) {
if (values!= null) {
for (DrmCropCodeType value: values) {
getPropertyVariableCode().add(value);
}
}
return this;
}
public PropertyVariable withPropertyVariableCode(Collection values) {
if (values!= null) {
getPropertyVariableCode().addAll(values);
}
return this;
}
public PropertyVariable withPropertyVariableDesignator(String value) {
setPropertyVariableDesignator(value);
return this;
}
public PropertyVariable withExtendedDesignator(String value) {
setExtendedDesignator(value);
return this;
}
public PropertyVariable withMinimumValue(BigDecimal value) {
setMinimumValue(value);
return this;
}
public PropertyVariable withMaximumValue(BigDecimal value) {
setMaximumValue(value);
return this;
}
public PropertyVariable withDefinition(String value) {
setDefinition(value);
return this;
}
public PropertyVariable withValueEnumerator(ValueEnumeration value) {
setValueEnumerator(value);
return this;
}
public PropertyVariable withNumeratorUom(DrmCropCodeType value) {
setNumeratorUom(value);
return this;
}
public PropertyVariable withDenumeratorUom(DrmCropCodeType value) {
setDenumeratorUom(value);
return this;
}
public PropertyVariable withSecondDenumeratorUom(DrmCropCodeType value) {
setSecondDenumeratorUom(value);
return this;
}
}