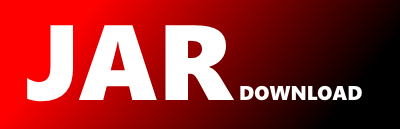
org.drmcrop.crp.CropProductionUnit Maven / Gradle / Ivy
//
// This file was generated by the JavaTM Architecture for XML Binding(JAXB) Reference Implementation, v2.2.6
// See http://java.sun.com/xml/jaxb
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2015.02.07 at 09:47:09 AM CET
//
package org.drmcrop.crp;
import java.math.BigInteger;
import java.util.ArrayList;
import java.util.Collection;
import java.util.List;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlID;
import javax.xml.bind.annotation.XmlSchemaType;
import javax.xml.bind.annotation.XmlType;
import javax.xml.bind.annotation.adapters.CollapsedStringAdapter;
import javax.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
import org.drmcrop.crpdt.CodeType;
import org.drmcrop.crpdt.GlobalUniqueIdentifierType;
import org.jvnet.jaxb2_commons.lang.JAXBToStringStrategy;
import org.jvnet.jaxb2_commons.lang.ToString;
import org.jvnet.jaxb2_commons.lang.ToStringStrategy;
import org.jvnet.jaxb2_commons.locator.ObjectLocator;
/**
* Definition.
* The cultivation of one CropClass on one or more CropField's which is seen by the Farm enterprise as one unit of production which should be distinguished from other CropProductionUnits's.
*
* Remarks.
* Reasons to separate the same CropClass in different CropProductionUnit's are determined by the farm enterprise. In many cases a different variety will lead to different CropProductionUnit's, but also the purpose for which crops are grown can be a reason ( seed production versus consumption) or in case of seed production the differences in classification can be a reason.
*
* CropField is used to specify the time period during which the CropProductionUnit occupies a certain area of a Field and the status (planned, realised, ...) .
*
* Operations are planned and executed on an ActivityField which can be the same surface as a CropField, but also a combination of more CropFields or only a part of a CropField.
*
* Activities on a CropProductionUnit can also include those which are not executed on a ActivityField like sorting of planting material and storage of the Produce.
*
* An organisation can decide to start and stop the CropProductionUnit when field activities start and finish, and see Batch'es of Product (the seed) or Produce (what is harvested) as a unit of production.
*
* Java class for CropProductionUnit complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="CropProductionUnit">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="CropProductionUnitID" type="{http://www.w3.org/2001/XMLSchema}ID"/>
* <element name="CropProductionUnitGUID" type="{http://www.drmCrop.org/schemas/DataTypes}GlobalUniqueIdentifierType"/>
* <element name="CropProductionUnitDesignator" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="CropProductionUnitYear" type="{http://www.w3.org/2001/XMLSchema}integer"/>
* <element name="CropTypeCode" type="{http://www.drmCrop.org/schemas/DataTypes}CodeType"/>
* <element name="VarietyCode" type="{http://www.drmCrop.org/schemas/DataTypes}CodeType"/>
* <element name="CropProductionPurposeCode" type="{http://www.drmCrop.org/schemas/DataTypes}CodeType"/>
* <element name="ProductionTypeCode" type="{http://www.drmCrop.org/schemas/DataTypes}CodeType"/>
* <element name="CropClass" type="{http://www.drmCrop.org/schemas/drmCrop}CropClass"/>
* <element name="Variety" type="{http://www.drmCrop.org/schemas/drmCrop}Variety" maxOccurs="unbounded"/>
* <element name="CropField" type="{http://www.drmCrop.org/schemas/drmCrop}CropField" maxOccurs="unbounded" minOccurs="0"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "CropProductionUnit", propOrder = {
"cropProductionUnitID",
"cropProductionUnitGUID",
"cropProductionUnitDesignator",
"cropProductionUnitYear",
"cropTypeCode",
"varietyCode",
"cropProductionPurposeCode",
"productionTypeCode",
"cropClass",
"variety",
"cropField"
})
public class CropProductionUnit
implements ToString
{
@XmlElement(name = "CropProductionUnitID", required = true)
@XmlJavaTypeAdapter(CollapsedStringAdapter.class)
@XmlID
@XmlSchemaType(name = "ID")
protected String cropProductionUnitID;
@XmlElement(name = "CropProductionUnitGUID", required = true)
protected GlobalUniqueIdentifierType cropProductionUnitGUID;
@XmlElement(name = "CropProductionUnitDesignator", required = true)
protected String cropProductionUnitDesignator;
@XmlElement(name = "CropProductionUnitYear", required = true)
protected BigInteger cropProductionUnitYear;
@XmlElement(name = "CropTypeCode", required = true)
protected CodeType cropTypeCode;
@XmlElement(name = "VarietyCode", required = true)
protected CodeType varietyCode;
@XmlElement(name = "CropProductionPurposeCode", required = true)
protected CodeType cropProductionPurposeCode;
@XmlElement(name = "ProductionTypeCode", required = true)
protected CodeType productionTypeCode;
@XmlElement(name = "CropClass", required = true)
protected CropClass cropClass;
@XmlElement(name = "Variety", required = true)
protected List variety;
@XmlElement(name = "CropField")
protected List cropField;
/**
* Gets the value of the cropProductionUnitID property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getCropProductionUnitID() {
return cropProductionUnitID;
}
/**
* Sets the value of the cropProductionUnitID property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setCropProductionUnitID(String value) {
this.cropProductionUnitID = value;
}
/**
* Gets the value of the cropProductionUnitGUID property.
*
* @return
* possible object is
* {@link GlobalUniqueIdentifierType }
*
*/
public GlobalUniqueIdentifierType getCropProductionUnitGUID() {
return cropProductionUnitGUID;
}
/**
* Sets the value of the cropProductionUnitGUID property.
*
* @param value
* allowed object is
* {@link GlobalUniqueIdentifierType }
*
*/
public void setCropProductionUnitGUID(GlobalUniqueIdentifierType value) {
this.cropProductionUnitGUID = value;
}
/**
* Gets the value of the cropProductionUnitDesignator property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getCropProductionUnitDesignator() {
return cropProductionUnitDesignator;
}
/**
* Sets the value of the cropProductionUnitDesignator property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setCropProductionUnitDesignator(String value) {
this.cropProductionUnitDesignator = value;
}
/**
* Gets the value of the cropProductionUnitYear property.
*
* @return
* possible object is
* {@link BigInteger }
*
*/
public BigInteger getCropProductionUnitYear() {
return cropProductionUnitYear;
}
/**
* Sets the value of the cropProductionUnitYear property.
*
* @param value
* allowed object is
* {@link BigInteger }
*
*/
public void setCropProductionUnitYear(BigInteger value) {
this.cropProductionUnitYear = value;
}
/**
* Gets the value of the cropTypeCode property.
*
* @return
* possible object is
* {@link CodeType }
*
*/
public CodeType getCropTypeCode() {
return cropTypeCode;
}
/**
* Sets the value of the cropTypeCode property.
*
* @param value
* allowed object is
* {@link CodeType }
*
*/
public void setCropTypeCode(CodeType value) {
this.cropTypeCode = value;
}
/**
* Gets the value of the varietyCode property.
*
* @return
* possible object is
* {@link CodeType }
*
*/
public CodeType getVarietyCode() {
return varietyCode;
}
/**
* Sets the value of the varietyCode property.
*
* @param value
* allowed object is
* {@link CodeType }
*
*/
public void setVarietyCode(CodeType value) {
this.varietyCode = value;
}
/**
* Gets the value of the cropProductionPurposeCode property.
*
* @return
* possible object is
* {@link CodeType }
*
*/
public CodeType getCropProductionPurposeCode() {
return cropProductionPurposeCode;
}
/**
* Sets the value of the cropProductionPurposeCode property.
*
* @param value
* allowed object is
* {@link CodeType }
*
*/
public void setCropProductionPurposeCode(CodeType value) {
this.cropProductionPurposeCode = value;
}
/**
* Gets the value of the productionTypeCode property.
*
* @return
* possible object is
* {@link CodeType }
*
*/
public CodeType getProductionTypeCode() {
return productionTypeCode;
}
/**
* Sets the value of the productionTypeCode property.
*
* @param value
* allowed object is
* {@link CodeType }
*
*/
public void setProductionTypeCode(CodeType value) {
this.productionTypeCode = value;
}
/**
* Gets the value of the cropClass property.
*
* @return
* possible object is
* {@link CropClass }
*
*/
public CropClass getCropClass() {
return cropClass;
}
/**
* Sets the value of the cropClass property.
*
* @param value
* allowed object is
* {@link CropClass }
*
*/
public void setCropClass(CropClass value) {
this.cropClass = value;
}
/**
* Gets the value of the variety property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the variety property.
*
*
* For example, to add a new item, do as follows:
*
* getVariety().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Variety }
*
*
*/
public List getVariety() {
if (variety == null) {
variety = new ArrayList();
}
return this.variety;
}
/**
* Gets the value of the cropField property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the cropField property.
*
*
* For example, to add a new item, do as follows:
*
* getCropField().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link CropField }
*
*
*/
public List getCropField() {
if (cropField == null) {
cropField = new ArrayList();
}
return this.cropField;
}
public String toString() {
final ToStringStrategy strategy = JAXBToStringStrategy.INSTANCE;
final StringBuilder buffer = new StringBuilder();
append(null, buffer, strategy);
return buffer.toString();
}
public StringBuilder append(ObjectLocator locator, StringBuilder buffer, ToStringStrategy strategy) {
strategy.appendStart(locator, this, buffer);
appendFields(locator, buffer, strategy);
strategy.appendEnd(locator, this, buffer);
return buffer;
}
public StringBuilder appendFields(ObjectLocator locator, StringBuilder buffer, ToStringStrategy strategy) {
{
String theCropProductionUnitID;
theCropProductionUnitID = this.getCropProductionUnitID();
strategy.appendField(locator, this, "cropProductionUnitID", buffer, theCropProductionUnitID);
}
{
GlobalUniqueIdentifierType theCropProductionUnitGUID;
theCropProductionUnitGUID = this.getCropProductionUnitGUID();
strategy.appendField(locator, this, "cropProductionUnitGUID", buffer, theCropProductionUnitGUID);
}
{
String theCropProductionUnitDesignator;
theCropProductionUnitDesignator = this.getCropProductionUnitDesignator();
strategy.appendField(locator, this, "cropProductionUnitDesignator", buffer, theCropProductionUnitDesignator);
}
{
BigInteger theCropProductionUnitYear;
theCropProductionUnitYear = this.getCropProductionUnitYear();
strategy.appendField(locator, this, "cropProductionUnitYear", buffer, theCropProductionUnitYear);
}
{
CodeType theCropTypeCode;
theCropTypeCode = this.getCropTypeCode();
strategy.appendField(locator, this, "cropTypeCode", buffer, theCropTypeCode);
}
{
CodeType theVarietyCode;
theVarietyCode = this.getVarietyCode();
strategy.appendField(locator, this, "varietyCode", buffer, theVarietyCode);
}
{
CodeType theCropProductionPurposeCode;
theCropProductionPurposeCode = this.getCropProductionPurposeCode();
strategy.appendField(locator, this, "cropProductionPurposeCode", buffer, theCropProductionPurposeCode);
}
{
CodeType theProductionTypeCode;
theProductionTypeCode = this.getProductionTypeCode();
strategy.appendField(locator, this, "productionTypeCode", buffer, theProductionTypeCode);
}
{
CropClass theCropClass;
theCropClass = this.getCropClass();
strategy.appendField(locator, this, "cropClass", buffer, theCropClass);
}
{
List theVariety;
theVariety = (((this.variety!= null)&&(!this.variety.isEmpty()))?this.getVariety():null);
strategy.appendField(locator, this, "variety", buffer, theVariety);
}
{
List theCropField;
theCropField = (((this.cropField!= null)&&(!this.cropField.isEmpty()))?this.getCropField():null);
strategy.appendField(locator, this, "cropField", buffer, theCropField);
}
return buffer;
}
public CropProductionUnit withCropProductionUnitID(String value) {
setCropProductionUnitID(value);
return this;
}
public CropProductionUnit withCropProductionUnitGUID(GlobalUniqueIdentifierType value) {
setCropProductionUnitGUID(value);
return this;
}
public CropProductionUnit withCropProductionUnitDesignator(String value) {
setCropProductionUnitDesignator(value);
return this;
}
public CropProductionUnit withCropProductionUnitYear(BigInteger value) {
setCropProductionUnitYear(value);
return this;
}
public CropProductionUnit withCropTypeCode(CodeType value) {
setCropTypeCode(value);
return this;
}
public CropProductionUnit withVarietyCode(CodeType value) {
setVarietyCode(value);
return this;
}
public CropProductionUnit withCropProductionPurposeCode(CodeType value) {
setCropProductionPurposeCode(value);
return this;
}
public CropProductionUnit withProductionTypeCode(CodeType value) {
setProductionTypeCode(value);
return this;
}
public CropProductionUnit withCropClass(CropClass value) {
setCropClass(value);
return this;
}
public CropProductionUnit withVariety(Variety... values) {
if (values!= null) {
for (Variety value: values) {
getVariety().add(value);
}
}
return this;
}
public CropProductionUnit withVariety(Collection values) {
if (values!= null) {
getVariety().addAll(values);
}
return this;
}
public CropProductionUnit withCropField(CropField... values) {
if (values!= null) {
for (CropField value: values) {
getCropField().add(value);
}
}
return this;
}
public CropProductionUnit withCropField(Collection values) {
if (values!= null) {
getCropField().addAll(values);
}
return this;
}
}