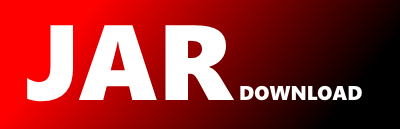
org.drmcrop.crp.Worker Maven / Gradle / Ivy
The newest version!
//
// This file was generated by the JavaTM Architecture for XML Binding(JAXB) Reference Implementation, v2.2.6
// See http://java.sun.com/xml/jaxb
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2015.02.07 at 09:47:09 AM CET
//
package org.drmcrop.crp;
import java.util.Collection;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlSchemaType;
import javax.xml.bind.annotation.XmlType;
import javax.xml.bind.annotation.adapters.NormalizedStringAdapter;
import javax.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
import javax.xml.datatype.XMLGregorianCalendar;
import org.drmcrop.crpdt.AddressType;
import org.drmcrop.crpdt.DeliveryAddressType;
import org.drmcrop.crpdt.GlobalUniqueIdentifierType;
import org.drmcrop.crpdt.PartyRoleType;
import org.drmcrop.crpdt.PhoneNumberType;
import org.drmcrop.crpe.GenderEnumeration;
import org.drmcrop.crpe.PartyCategoryEnumeration;
import org.jvnet.jaxb2_commons.lang.JAXBToStringStrategy;
import org.jvnet.jaxb2_commons.lang.ToString;
import org.jvnet.jaxb2_commons.lang.ToStringStrategy;
import org.jvnet.jaxb2_commons.locator.ObjectLocator;
/**
* Description.
* A Worker is a person that performs activities (Task's) for an Organization.
*
* Java class for Worker complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="Worker">
* <complexContent>
* <extension base="{http://www.drmCrop.org/schemas/drmCrop}Person">
* <sequence>
* <element name="WorkPhone" type="{http://www.drmCrop.org/schemas/DataTypes}PhoneNumberType"/>
* <element name="WorkMobilePhone" type="{http://www.drmCrop.org/schemas/DataTypes}PhoneNumberType"/>
* <element name="WorkE-Mail" type="{http://www.w3.org/2001/XMLSchema}anyURI"/>
* <element name="JobTitle" type="{http://www.w3.org/2001/XMLSchema}normalizedString"/>
* </sequence>
* </extension>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "Worker", propOrder = {
"workPhone",
"workMobilePhone",
"workEMail",
"jobTitle"
})
public class Worker
extends Person
implements ToString
{
@XmlElement(name = "WorkPhone", required = true)
protected PhoneNumberType workPhone;
@XmlElement(name = "WorkMobilePhone", required = true)
protected PhoneNumberType workMobilePhone;
@XmlElement(name = "WorkE-Mail", required = true)
@XmlSchemaType(name = "anyURI")
protected String workEMail;
@XmlElement(name = "JobTitle", required = true)
@XmlJavaTypeAdapter(NormalizedStringAdapter.class)
@XmlSchemaType(name = "normalizedString")
protected String jobTitle;
/**
* Gets the value of the workPhone property.
*
* @return
* possible object is
* {@link PhoneNumberType }
*
*/
public PhoneNumberType getWorkPhone() {
return workPhone;
}
/**
* Sets the value of the workPhone property.
*
* @param value
* allowed object is
* {@link PhoneNumberType }
*
*/
public void setWorkPhone(PhoneNumberType value) {
this.workPhone = value;
}
/**
* Gets the value of the workMobilePhone property.
*
* @return
* possible object is
* {@link PhoneNumberType }
*
*/
public PhoneNumberType getWorkMobilePhone() {
return workMobilePhone;
}
/**
* Sets the value of the workMobilePhone property.
*
* @param value
* allowed object is
* {@link PhoneNumberType }
*
*/
public void setWorkMobilePhone(PhoneNumberType value) {
this.workMobilePhone = value;
}
/**
* Gets the value of the workEMail property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getWorkEMail() {
return workEMail;
}
/**
* Sets the value of the workEMail property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setWorkEMail(String value) {
this.workEMail = value;
}
/**
* Gets the value of the jobTitle property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getJobTitle() {
return jobTitle;
}
/**
* Sets the value of the jobTitle property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setJobTitle(String value) {
this.jobTitle = value;
}
public String toString() {
final ToStringStrategy strategy = JAXBToStringStrategy.INSTANCE;
final StringBuilder buffer = new StringBuilder();
append(null, buffer, strategy);
return buffer.toString();
}
public StringBuilder append(ObjectLocator locator, StringBuilder buffer, ToStringStrategy strategy) {
strategy.appendStart(locator, this, buffer);
appendFields(locator, buffer, strategy);
strategy.appendEnd(locator, this, buffer);
return buffer;
}
public StringBuilder appendFields(ObjectLocator locator, StringBuilder buffer, ToStringStrategy strategy) {
super.appendFields(locator, buffer, strategy);
{
PhoneNumberType theWorkPhone;
theWorkPhone = this.getWorkPhone();
strategy.appendField(locator, this, "workPhone", buffer, theWorkPhone);
}
{
PhoneNumberType theWorkMobilePhone;
theWorkMobilePhone = this.getWorkMobilePhone();
strategy.appendField(locator, this, "workMobilePhone", buffer, theWorkMobilePhone);
}
{
String theWorkEMail;
theWorkEMail = this.getWorkEMail();
strategy.appendField(locator, this, "workEMail", buffer, theWorkEMail);
}
{
String theJobTitle;
theJobTitle = this.getJobTitle();
strategy.appendField(locator, this, "jobTitle", buffer, theJobTitle);
}
return buffer;
}
public Worker withWorkPhone(PhoneNumberType value) {
setWorkPhone(value);
return this;
}
public Worker withWorkMobilePhone(PhoneNumberType value) {
setWorkMobilePhone(value);
return this;
}
public Worker withWorkEMail(String value) {
setWorkEMail(value);
return this;
}
public Worker withJobTitle(String value) {
setJobTitle(value);
return this;
}
@Override
public Worker withFirstName(String value) {
setFirstName(value);
return this;
}
@Override
public Worker withMiddleName(String value) {
setMiddleName(value);
return this;
}
@Override
public Worker withLastName(String value) {
setLastName(value);
return this;
}
@Override
public Worker withBirthDate(XMLGregorianCalendar value) {
setBirthDate(value);
return this;
}
@Override
public Worker withGender(GenderEnumeration value) {
setGender(value);
return this;
}
@Override
public Worker withTitle(String value) {
setTitle(value);
return this;
}
@Override
public Worker withPrivatePhone(PhoneNumberType value) {
setPrivatePhone(value);
return this;
}
@Override
public Worker withPrivateMobilePhone(PhoneNumberType value) {
setPrivateMobilePhone(value);
return this;
}
@Override
public Worker withPrivateEMail(String value) {
setPrivateEMail(value);
return this;
}
@Override
public Worker withSalution(String value) {
setSalution(value);
return this;
}
@Override
public Worker withPartyID(String value) {
setPartyID(value);
return this;
}
@Override
public Worker withPartyGUID(GlobalUniqueIdentifierType value) {
setPartyGUID(value);
return this;
}
@Override
public Worker withThirdPartyGUID(GlobalUniqueIdentifierType value) {
setThirdPartyGUID(value);
return this;
}
@Override
public Worker withDesignator(String value) {
setDesignator(value);
return this;
}
@Override
public Worker withPartyCategory(PartyCategoryEnumeration value) {
setPartyCategory(value);
return this;
}
@Override
public Worker withPartyRole(PartyRoleType... values) {
if (values!= null) {
for (PartyRoleType value: values) {
getPartyRole().add(value);
}
}
return this;
}
@Override
public Worker withPartyRole(Collection values) {
if (values!= null) {
getPartyRole().addAll(values);
}
return this;
}
@Override
public Worker withDeliveryAddress(DeliveryAddressType value) {
setDeliveryAddress(value);
return this;
}
@Override
public Worker withPostalAddress(AddressType value) {
setPostalAddress(value);
return this;
}
@Override
public Worker withVisitingAddress(AddressType value) {
setVisitingAddress(value);
return this;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy